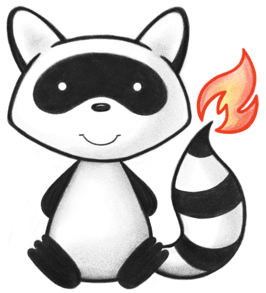
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 035 036 037import org.hl7.fhir.dstu3.model.EnumFactory; 038 039public class MapTransformEnumFactory implements EnumFactory<MapTransform> { 040 041 public MapTransform fromCode(String codeString) throws IllegalArgumentException { 042 if (codeString == null || "".equals(codeString)) 043 return null; 044 if ("create".equals(codeString)) 045 return MapTransform.CREATE; 046 if ("copy".equals(codeString)) 047 return MapTransform.COPY; 048 if ("truncate".equals(codeString)) 049 return MapTransform.TRUNCATE; 050 if ("escapeUrlParam".equals(codeString)) 051 return MapTransform.ESCAPE; 052 if ("cast".equals(codeString)) 053 return MapTransform.CAST; 054 if ("append".equals(codeString)) 055 return MapTransform.APPEND; 056 if ("translate".equals(codeString)) 057 return MapTransform.TRANSLATE; 058 if ("reference".equals(codeString)) 059 return MapTransform.REFERENCE; 060 if ("dateOp".equals(codeString)) 061 return MapTransform.DATEOP; 062 if ("uuid".equals(codeString)) 063 return MapTransform.UUID; 064 if ("pointer".equals(codeString)) 065 return MapTransform.POINTER; 066 if ("evaluate".equals(codeString)) 067 return MapTransform.EVALUATE; 068 if ("cc".equals(codeString)) 069 return MapTransform.CC; 070 if ("c".equals(codeString)) 071 return MapTransform.C; 072 if ("qty".equals(codeString)) 073 return MapTransform.QTY; 074 if ("id".equals(codeString)) 075 return MapTransform.ID; 076 if ("cp".equals(codeString)) 077 return MapTransform.CP; 078 throw new IllegalArgumentException("Unknown MapTransform code '"+codeString+"'"); 079 } 080 081 public String toCode(MapTransform code) { 082 if (code == MapTransform.NULL) 083 return null; 084 if (code == MapTransform.CREATE) 085 return "create"; 086 if (code == MapTransform.COPY) 087 return "copy"; 088 if (code == MapTransform.TRUNCATE) 089 return "truncate"; 090 if (code == MapTransform.ESCAPE) 091 return "escapeUrlParam"; 092 if (code == MapTransform.CAST) 093 return "cast"; 094 if (code == MapTransform.APPEND) 095 return "append"; 096 if (code == MapTransform.TRANSLATE) 097 return "translate"; 098 if (code == MapTransform.REFERENCE) 099 return "reference"; 100 if (code == MapTransform.DATEOP) 101 return "dateOp"; 102 if (code == MapTransform.UUID) 103 return "uuid"; 104 if (code == MapTransform.POINTER) 105 return "pointer"; 106 if (code == MapTransform.EVALUATE) 107 return "evaluate"; 108 if (code == MapTransform.CC) 109 return "cc"; 110 if (code == MapTransform.C) 111 return "c"; 112 if (code == MapTransform.QTY) 113 return "qty"; 114 if (code == MapTransform.ID) 115 return "id"; 116 if (code == MapTransform.CP) 117 return "cp"; 118 return "?"; 119 } 120 121 public String toSystem(MapTransform code) { 122 return code.getSystem(); 123 } 124 125}