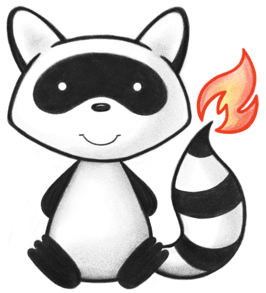
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum MedicationRequestStatus { 041 042 /** 043 * The prescription is 'actionable', but not all actions that are implied by it have occurred yet. 044 */ 045 ACTIVE, 046 /** 047 * Actions implied by the prescription are to be temporarily halted, but are expected to continue later. May also be called "suspended". 048 */ 049 ONHOLD, 050 /** 051 * The prescription has been withdrawn. 052 */ 053 CANCELLED, 054 /** 055 * All actions that are implied by the prescription have occurred. 056 */ 057 COMPLETED, 058 /** 059 * The prescription was entered in error. 060 */ 061 ENTEREDINERROR, 062 /** 063 * Actions implied by the prescription are to be permanently halted, before all of them occurred. 064 */ 065 STOPPED, 066 /** 067 * The prescription is not yet 'actionable', i.e. it is a work in progress, requires sign-off or verification, and needs to be run through decision support process. 068 */ 069 DRAFT, 070 /** 071 * The authoring system does not know which of the status values currently applies for this request 072 */ 073 UNKNOWN, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 public static MedicationRequestStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("active".equals(codeString)) 082 return ACTIVE; 083 if ("on-hold".equals(codeString)) 084 return ONHOLD; 085 if ("cancelled".equals(codeString)) 086 return CANCELLED; 087 if ("completed".equals(codeString)) 088 return COMPLETED; 089 if ("entered-in-error".equals(codeString)) 090 return ENTEREDINERROR; 091 if ("stopped".equals(codeString)) 092 return STOPPED; 093 if ("draft".equals(codeString)) 094 return DRAFT; 095 if ("unknown".equals(codeString)) 096 return UNKNOWN; 097 throw new FHIRException("Unknown MedicationRequestStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case ACTIVE: return "active"; 102 case ONHOLD: return "on-hold"; 103 case CANCELLED: return "cancelled"; 104 case COMPLETED: return "completed"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case STOPPED: return "stopped"; 107 case DRAFT: return "draft"; 108 case UNKNOWN: return "unknown"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getSystem() { 114 return "http://hl7.org/fhir/medication-request-status"; 115 } 116 public String getDefinition() { 117 switch (this) { 118 case ACTIVE: return "The prescription is 'actionable', but not all actions that are implied by it have occurred yet."; 119 case ONHOLD: return "Actions implied by the prescription are to be temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 120 case CANCELLED: return "The prescription has been withdrawn."; 121 case COMPLETED: return "All actions that are implied by the prescription have occurred."; 122 case ENTEREDINERROR: return "The prescription was entered in error."; 123 case STOPPED: return "Actions implied by the prescription are to be permanently halted, before all of them occurred."; 124 case DRAFT: return "The prescription is not yet 'actionable', i.e. it is a work in progress, requires sign-off or verification, and needs to be run through decision support process."; 125 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case ACTIVE: return "Active"; 133 case ONHOLD: return "On Hold"; 134 case CANCELLED: return "Cancelled"; 135 case COMPLETED: return "Completed"; 136 case ENTEREDINERROR: return "Entered In Error"; 137 case STOPPED: return "Stopped"; 138 case DRAFT: return "Draft"; 139 case UNKNOWN: return "Unknown"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 145 146}