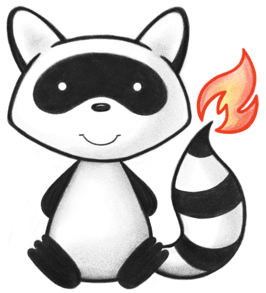
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum MessageEvents { 041 042 /** 043 * The definition of a code system is used to create a simple collection of codes suitable for use for data entry or validation. An expanded code system will be returned, or an error message. 044 */ 045 CODESYSTEMEXPAND, 046 /** 047 * Change the status of a Medication Administration to show that it is complete. 048 */ 049 MEDICATIONADMINISTRATIONCOMPLETE, 050 /** 051 * Someone wishes to record that the record of administration of a medication is in error and should be ignored. 052 */ 053 MEDICATIONADMINISTRATIONNULLIFICATION, 054 /** 055 * Indicates that a medication has been recorded against the patient's record. 056 */ 057 MEDICATIONADMINISTRATIONRECORDING, 058 /** 059 * Update a Medication Administration record. 060 */ 061 MEDICATIONADMINISTRATIONUPDATE, 062 /** 063 * Notification of a change to an administrative resource (either create or update). Note that there is no delete, though some administrative resources have status or period elements for this use. 064 */ 065 ADMINNOTIFY, 066 /** 067 * Notification to convey information. 068 */ 069 COMMUNICATIONREQUEST, 070 /** 071 * Provide a diagnostic report, or update a previously provided diagnostic report. 072 */ 073 DIAGNOSTICREPORTPROVIDE, 074 /** 075 * Provide a simple observation or update a previously provided simple observation. 076 */ 077 OBSERVATIONPROVIDE, 078 /** 079 * Notification that two patient records actually identify the same patient. 080 */ 081 PATIENTLINK, 082 /** 083 * Notification that previous advice that two patient records concern the same patient is now considered incorrect. 084 */ 085 PATIENTUNLINK, 086 /** 087 * The definition of a value set is used to create a simple collection of codes suitable for use for data entry or validation. An expanded value set will be returned, or an error message. 088 */ 089 VALUESETEXPAND, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 public static MessageEvents fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("CodeSystem-expand".equals(codeString)) 098 return CODESYSTEMEXPAND; 099 if ("MedicationAdministration-Complete".equals(codeString)) 100 return MEDICATIONADMINISTRATIONCOMPLETE; 101 if ("MedicationAdministration-Nullification".equals(codeString)) 102 return MEDICATIONADMINISTRATIONNULLIFICATION; 103 if ("MedicationAdministration-Recording".equals(codeString)) 104 return MEDICATIONADMINISTRATIONRECORDING; 105 if ("MedicationAdministration-Update".equals(codeString)) 106 return MEDICATIONADMINISTRATIONUPDATE; 107 if ("admin-notify".equals(codeString)) 108 return ADMINNOTIFY; 109 if ("communication-request".equals(codeString)) 110 return COMMUNICATIONREQUEST; 111 if ("diagnosticreport-provide".equals(codeString)) 112 return DIAGNOSTICREPORTPROVIDE; 113 if ("observation-provide".equals(codeString)) 114 return OBSERVATIONPROVIDE; 115 if ("patient-link".equals(codeString)) 116 return PATIENTLINK; 117 if ("patient-unlink".equals(codeString)) 118 return PATIENTUNLINK; 119 if ("valueset-expand".equals(codeString)) 120 return VALUESETEXPAND; 121 throw new FHIRException("Unknown MessageEvents code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case CODESYSTEMEXPAND: return "CodeSystem-expand"; 126 case MEDICATIONADMINISTRATIONCOMPLETE: return "MedicationAdministration-Complete"; 127 case MEDICATIONADMINISTRATIONNULLIFICATION: return "MedicationAdministration-Nullification"; 128 case MEDICATIONADMINISTRATIONRECORDING: return "MedicationAdministration-Recording"; 129 case MEDICATIONADMINISTRATIONUPDATE: return "MedicationAdministration-Update"; 130 case ADMINNOTIFY: return "admin-notify"; 131 case COMMUNICATIONREQUEST: return "communication-request"; 132 case DIAGNOSTICREPORTPROVIDE: return "diagnosticreport-provide"; 133 case OBSERVATIONPROVIDE: return "observation-provide"; 134 case PATIENTLINK: return "patient-link"; 135 case PATIENTUNLINK: return "patient-unlink"; 136 case VALUESETEXPAND: return "valueset-expand"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getSystem() { 142 return "http://hl7.org/fhir/message-events"; 143 } 144 public String getDefinition() { 145 switch (this) { 146 case CODESYSTEMEXPAND: return "The definition of a code system is used to create a simple collection of codes suitable for use for data entry or validation. An expanded code system will be returned, or an error message."; 147 case MEDICATIONADMINISTRATIONCOMPLETE: return "Change the status of a Medication Administration to show that it is complete."; 148 case MEDICATIONADMINISTRATIONNULLIFICATION: return "Someone wishes to record that the record of administration of a medication is in error and should be ignored."; 149 case MEDICATIONADMINISTRATIONRECORDING: return "Indicates that a medication has been recorded against the patient's record."; 150 case MEDICATIONADMINISTRATIONUPDATE: return "Update a Medication Administration record."; 151 case ADMINNOTIFY: return "Notification of a change to an administrative resource (either create or update). Note that there is no delete, though some administrative resources have status or period elements for this use."; 152 case COMMUNICATIONREQUEST: return "Notification to convey information."; 153 case DIAGNOSTICREPORTPROVIDE: return "Provide a diagnostic report, or update a previously provided diagnostic report."; 154 case OBSERVATIONPROVIDE: return "Provide a simple observation or update a previously provided simple observation."; 155 case PATIENTLINK: return "Notification that two patient records actually identify the same patient."; 156 case PATIENTUNLINK: return "Notification that previous advice that two patient records concern the same patient is now considered incorrect."; 157 case VALUESETEXPAND: return "The definition of a value set is used to create a simple collection of codes suitable for use for data entry or validation. An expanded value set will be returned, or an error message."; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getDisplay() { 163 switch (this) { 164 case CODESYSTEMEXPAND: return "CodeSystem-expand"; 165 case MEDICATIONADMINISTRATIONCOMPLETE: return "MedicationAdministration-Complete"; 166 case MEDICATIONADMINISTRATIONNULLIFICATION: return "MedicationAdministration-Nullification"; 167 case MEDICATIONADMINISTRATIONRECORDING: return "MedicationAdministration-Recording"; 168 case MEDICATIONADMINISTRATIONUPDATE: return "MedicationAdministration-Update"; 169 case ADMINNOTIFY: return "admin-notify"; 170 case COMMUNICATIONREQUEST: return "communication-request"; 171 case DIAGNOSTICREPORTPROVIDE: return "diagnosticreport-provide"; 172 case OBSERVATIONPROVIDE: return "observation-provide"; 173 case PATIENTLINK: return "patient-link"; 174 case PATIENTUNLINK: return "patient-unlink"; 175 case VALUESETEXPAND: return "valueset-expand"; 176 case NULL: return null; 177 default: return "?"; 178 } 179 } 180 181 182}