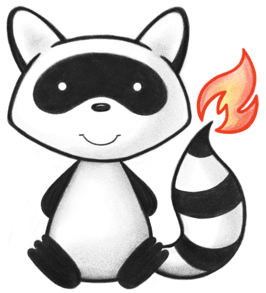
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum NameUse { 041 042 /** 043 * Known as/conventional/the one you normally use 044 */ 045 USUAL, 046 /** 047 * The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called "legal name". 048 */ 049 OFFICIAL, 050 /** 051 * A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations. 052 */ 053 TEMP, 054 /** 055 * A name that is used to address the person in an informal manner, but is not part of their formal or usual name 056 */ 057 NICKNAME, 058 /** 059 * Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons) 060 */ 061 ANONYMOUS, 062 /** 063 * This name is no longer in use (or was never correct, but retained for records) 064 */ 065 OLD, 066 /** 067 * A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name 068 */ 069 MAIDEN, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static NameUse fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("usual".equals(codeString)) 078 return USUAL; 079 if ("official".equals(codeString)) 080 return OFFICIAL; 081 if ("temp".equals(codeString)) 082 return TEMP; 083 if ("nickname".equals(codeString)) 084 return NICKNAME; 085 if ("anonymous".equals(codeString)) 086 return ANONYMOUS; 087 if ("old".equals(codeString)) 088 return OLD; 089 if ("maiden".equals(codeString)) 090 return MAIDEN; 091 throw new FHIRException("Unknown NameUse code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case USUAL: return "usual"; 096 case OFFICIAL: return "official"; 097 case TEMP: return "temp"; 098 case NICKNAME: return "nickname"; 099 case ANONYMOUS: return "anonymous"; 100 case OLD: return "old"; 101 case MAIDEN: return "maiden"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/name-use"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case USUAL: return "Known as/conventional/the one you normally use"; 112 case OFFICIAL: return "The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\"."; 113 case TEMP: return "A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 114 case NICKNAME: return "A name that is used to address the person in an informal manner, but is not part of their formal or usual name"; 115 case ANONYMOUS: return "Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons)"; 116 case OLD: return "This name is no longer in use (or was never correct, but retained for records)"; 117 case MAIDEN: return "A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case USUAL: return "Usual"; 125 case OFFICIAL: return "Official"; 126 case TEMP: return "Temp"; 127 case NICKNAME: return "Nickname"; 128 case ANONYMOUS: return "Anonymous"; 129 case OLD: return "Old"; 130 case MAIDEN: return "Name changed for Marriage"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}