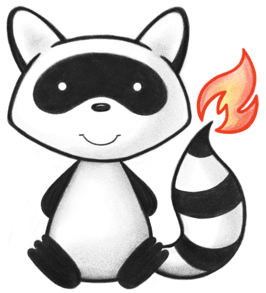
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ObjectRole { 041 042 /** 043 * This object is the patient that is the subject of care related to this event. It is identifiable by patient ID or equivalent. The patient may be either human or animal. 044 */ 045 _1, 046 /** 047 * This is a location identified as related to the event. This is usually the location where the event took place. Note that for shipping, the usual events are arrival at a location or departure from a location. 048 */ 049 _2, 050 /** 051 * This object is any kind of persistent document created as a result of the event. This could be a paper report, film, electronic report, DICOM Study, etc. Issues related to medical records life cycle management are conveyed elsewhere. 052 */ 053 _3, 054 /** 055 * A logical object related to a health record event. This is any healthcare specific resource (object) not restricted to FHIR defined Resources. 056 */ 057 _4, 058 /** 059 * This is any configurable file used to control creation of documents. Examples include the objects maintained by the HL7 Master File transactions, Value Sets, etc. 060 */ 061 _5, 062 /** 063 * A human participant not otherwise identified by some other category. 064 */ 065 _6, 066 /** 067 * (deprecated) 068 */ 069 _7, 070 /** 071 * Typically a licensed person who is providing or performing care related to the event, generally a physician. The key distinction between doctor and practitioner is with regards to their role, not the licensing. The doctor is the human who actually performed the work. The practitioner is the human or organization that is responsible for the work. 072 */ 073 _8, 074 /** 075 * A person or system that is being notified as part of the event. This is relevant in situations where automated systems provide notifications to other parties when an event took place. 076 */ 077 _9, 078 /** 079 * Insurance company, or any other organization who accepts responsibility for paying for the healthcare event. 080 */ 081 _10, 082 /** 083 * A person or active system object involved in the event with a security role. 084 */ 085 _11, 086 /** 087 * A person or system object involved in the event with the authority to modify security roles of other objects. 088 */ 089 _12, 090 /** 091 * A passive object, such as a role table, that is relevant to the event. 092 */ 093 _13, 094 /** 095 * (deprecated) Relevant to certain RBAC security methodologies. 096 */ 097 _14, 098 /** 099 * Any person or organization responsible for providing care. This encompasses all forms of care, licensed or otherwise, and all sorts of teams and care groups. Note the distinction between practitioner and the doctor that actually provided the care to the patient. 100 */ 101 _15, 102 /** 103 * The source or destination for data transfer, when it does not match some other role. 104 */ 105 _16, 106 /** 107 * A source or destination for data transfer that acts as an archive, database, or similar role. 108 */ 109 _17, 110 /** 111 * An object that holds schedule information. This could be an appointment book, availability information, etc. 112 */ 113 _18, 114 /** 115 * An organization or person that is the recipient of services. This could be an organization that is buying services for a patient, or a person that is buying services for an animal. 116 */ 117 _19, 118 /** 119 * An order, task, work item, procedure step, or other description of work to be performed; e.g. a particular instance of an MPPS. 120 */ 121 _20, 122 /** 123 * A list of jobs or a system that provides lists of jobs; e.g. an MWL SCP. 124 */ 125 _21, 126 /** 127 * (Deprecated) 128 */ 129 _22, 130 /** 131 * An object that specifies or controls the routing or delivery of items. For example, a distribution list is the routing criteria for mail. The items delivered may be documents, jobs, or other objects. 132 */ 133 _23, 134 /** 135 * The contents of a query. This is used to capture the contents of any kind of query. For security surveillance purposes knowing the queries being made is very important. 136 */ 137 _24, 138 /** 139 * added to help the parsers 140 */ 141 NULL; 142 public static ObjectRole fromCode(String codeString) throws FHIRException { 143 if (codeString == null || "".equals(codeString)) 144 return null; 145 if ("1".equals(codeString)) 146 return _1; 147 if ("2".equals(codeString)) 148 return _2; 149 if ("3".equals(codeString)) 150 return _3; 151 if ("4".equals(codeString)) 152 return _4; 153 if ("5".equals(codeString)) 154 return _5; 155 if ("6".equals(codeString)) 156 return _6; 157 if ("7".equals(codeString)) 158 return _7; 159 if ("8".equals(codeString)) 160 return _8; 161 if ("9".equals(codeString)) 162 return _9; 163 if ("10".equals(codeString)) 164 return _10; 165 if ("11".equals(codeString)) 166 return _11; 167 if ("12".equals(codeString)) 168 return _12; 169 if ("13".equals(codeString)) 170 return _13; 171 if ("14".equals(codeString)) 172 return _14; 173 if ("15".equals(codeString)) 174 return _15; 175 if ("16".equals(codeString)) 176 return _16; 177 if ("17".equals(codeString)) 178 return _17; 179 if ("18".equals(codeString)) 180 return _18; 181 if ("19".equals(codeString)) 182 return _19; 183 if ("20".equals(codeString)) 184 return _20; 185 if ("21".equals(codeString)) 186 return _21; 187 if ("22".equals(codeString)) 188 return _22; 189 if ("23".equals(codeString)) 190 return _23; 191 if ("24".equals(codeString)) 192 return _24; 193 throw new FHIRException("Unknown ObjectRole code '"+codeString+"'"); 194 } 195 public String toCode() { 196 switch (this) { 197 case _1: return "1"; 198 case _2: return "2"; 199 case _3: return "3"; 200 case _4: return "4"; 201 case _5: return "5"; 202 case _6: return "6"; 203 case _7: return "7"; 204 case _8: return "8"; 205 case _9: return "9"; 206 case _10: return "10"; 207 case _11: return "11"; 208 case _12: return "12"; 209 case _13: return "13"; 210 case _14: return "14"; 211 case _15: return "15"; 212 case _16: return "16"; 213 case _17: return "17"; 214 case _18: return "18"; 215 case _19: return "19"; 216 case _20: return "20"; 217 case _21: return "21"; 218 case _22: return "22"; 219 case _23: return "23"; 220 case _24: return "24"; 221 case NULL: return null; 222 default: return "?"; 223 } 224 } 225 public String getSystem() { 226 return "http://hl7.org/fhir/object-role"; 227 } 228 public String getDefinition() { 229 switch (this) { 230 case _1: return "This object is the patient that is the subject of care related to this event. It is identifiable by patient ID or equivalent. The patient may be either human or animal."; 231 case _2: return "This is a location identified as related to the event. This is usually the location where the event took place. Note that for shipping, the usual events are arrival at a location or departure from a location."; 232 case _3: return "This object is any kind of persistent document created as a result of the event. This could be a paper report, film, electronic report, DICOM Study, etc. Issues related to medical records life cycle management are conveyed elsewhere."; 233 case _4: return "A logical object related to a health record event. This is any healthcare specific resource (object) not restricted to FHIR defined Resources."; 234 case _5: return "This is any configurable file used to control creation of documents. Examples include the objects maintained by the HL7 Master File transactions, Value Sets, etc."; 235 case _6: return "A human participant not otherwise identified by some other category."; 236 case _7: return "(deprecated)"; 237 case _8: return "Typically a licensed person who is providing or performing care related to the event, generally a physician. The key distinction between doctor and practitioner is with regards to their role, not the licensing. The doctor is the human who actually performed the work. The practitioner is the human or organization that is responsible for the work."; 238 case _9: return "A person or system that is being notified as part of the event. This is relevant in situations where automated systems provide notifications to other parties when an event took place."; 239 case _10: return "Insurance company, or any other organization who accepts responsibility for paying for the healthcare event."; 240 case _11: return "A person or active system object involved in the event with a security role."; 241 case _12: return "A person or system object involved in the event with the authority to modify security roles of other objects."; 242 case _13: return "A passive object, such as a role table, that is relevant to the event."; 243 case _14: return "(deprecated) Relevant to certain RBAC security methodologies."; 244 case _15: return "Any person or organization responsible for providing care. This encompasses all forms of care, licensed or otherwise, and all sorts of teams and care groups. Note the distinction between practitioner and the doctor that actually provided the care to the patient."; 245 case _16: return "The source or destination for data transfer, when it does not match some other role."; 246 case _17: return "A source or destination for data transfer that acts as an archive, database, or similar role."; 247 case _18: return "An object that holds schedule information. This could be an appointment book, availability information, etc."; 248 case _19: return "An organization or person that is the recipient of services. This could be an organization that is buying services for a patient, or a person that is buying services for an animal."; 249 case _20: return "An order, task, work item, procedure step, or other description of work to be performed; e.g. a particular instance of an MPPS."; 250 case _21: return "A list of jobs or a system that provides lists of jobs; e.g. an MWL SCP."; 251 case _22: return "(Deprecated)"; 252 case _23: return "An object that specifies or controls the routing or delivery of items. For example, a distribution list is the routing criteria for mail. The items delivered may be documents, jobs, or other objects."; 253 case _24: return "The contents of a query. This is used to capture the contents of any kind of query. For security surveillance purposes knowing the queries being made is very important."; 254 case NULL: return null; 255 default: return "?"; 256 } 257 } 258 public String getDisplay() { 259 switch (this) { 260 case _1: return "Patient"; 261 case _2: return "Location"; 262 case _3: return "Report"; 263 case _4: return "Domain Resource"; 264 case _5: return "Master file"; 265 case _6: return "User"; 266 case _7: return "List"; 267 case _8: return "Doctor"; 268 case _9: return "Subscriber"; 269 case _10: return "Guarantor"; 270 case _11: return "Security User Entity"; 271 case _12: return "Security User Group"; 272 case _13: return "Security Resource"; 273 case _14: return "Security Granularity Definition"; 274 case _15: return "Practitioner"; 275 case _16: return "Data Destination"; 276 case _17: return "Data Repository"; 277 case _18: return "Schedule"; 278 case _19: return "Customer"; 279 case _20: return "Job"; 280 case _21: return "Job Stream"; 281 case _22: return "Table"; 282 case _23: return "Routing Criteria"; 283 case _24: return "Query"; 284 case NULL: return null; 285 default: return "?"; 286 } 287 } 288 289 290}