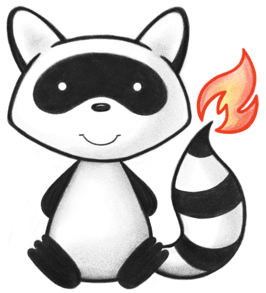
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ObservationStatistics { 041 042 /** 043 * The [mean](https://en.wikipedia.org/wiki/Arithmetic_mean) of N measurements over the stated period 044 */ 045 AVERAGE, 046 /** 047 * The [maximum](https://en.wikipedia.org/wiki/Maximal_element) value of N measurements over the stated period 048 */ 049 MAXIMUM, 050 /** 051 * The [minimum](https://en.wikipedia.org/wiki/Minimal_element) value of N measurements over the stated period 052 */ 053 MINIMUM, 054 /** 055 * The [number] of valid measurements over the stated period that contributed to the other statistical outputs 056 */ 057 COUNT, 058 /** 059 * The total [number] of valid measurements over the stated period, including observations that were ignored because they did not contain valid result values 060 */ 061 TOTALCOUNT, 062 /** 063 * The [median](https://en.wikipedia.org/wiki/Median) of N measurements over the stated period 064 */ 065 MEDIAN, 066 /** 067 * The [standard deviation](https://en.wikipedia.org/wiki/Standard_deviation) of N measurements over the stated period 068 */ 069 STDDEV, 070 /** 071 * The [sum](https://en.wikipedia.org/wiki/Summation) of N measurements over the stated period 072 */ 073 SUM, 074 /** 075 * The [variance](https://en.wikipedia.org/wiki/Variance) of N measurements over the stated period 076 */ 077 VARIANCE, 078 /** 079 * The 20th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N measurements over the stated period 080 */ 081 _20PERCENT, 082 /** 083 * The 80th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N measurements over the stated period 084 */ 085 _80PERCENT, 086 /** 087 * The lower [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N measurements over the stated period 088 */ 089 _4LOWER, 090 /** 091 * The upper [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N measurements over the stated period 092 */ 093 _4UPPER, 094 /** 095 * The difference between the upper and lower [Quartiles](https://en.wikipedia.org/wiki/Quartile) is called the Interquartile range. (IQR = Q3-Q1) Quartile deviation or Semi-interquartile range is one-half the difference between the first and the third quartiles. 096 */ 097 _4DEV, 098 /** 099 * The lowest of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population 100 */ 101 _51, 102 /** 103 * The second of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population 104 */ 105 _52, 106 /** 107 * The third of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population 108 */ 109 _53, 110 /** 111 * The fourth of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population 112 */ 113 _54, 114 /** 115 * Skewness is a measure of the asymmetry of the probability distribution of a real-valued random variable about its mean. The skewness value can be positive or negative, or even undefined. Source: [Wikipedia](https://en.wikipedia.org/wiki/Skewness) 116 */ 117 SKEW, 118 /** 119 * Kurtosis is a measure of the "tailedness" of the probability distribution of a real-valued random variable. Source: [Wikipedia](https://en.wikipedia.org/wiki/Kurtosis) 120 */ 121 KURTOSIS, 122 /** 123 * Linear regression is an approach for modeling two-dimensional sample points with one independent variable and one dependent variable (conventionally, the x and y coordinates in a Cartesian coordinate system) and finds a linear function (a non-vertical straight line) that, as accurately as possible, predicts the dependent variable values as a function of the independent variables. Source: [Wikipedia](https://en.wikipedia.org/wiki/Simple_linear_regression) This Statistic code will return both a gradient and an intercept value. 124 */ 125 REGRESSION, 126 /** 127 * added to help the parsers 128 */ 129 NULL; 130 public static ObservationStatistics fromCode(String codeString) throws FHIRException { 131 if (codeString == null || "".equals(codeString)) 132 return null; 133 if ("average".equals(codeString)) 134 return AVERAGE; 135 if ("maximum".equals(codeString)) 136 return MAXIMUM; 137 if ("minimum".equals(codeString)) 138 return MINIMUM; 139 if ("count".equals(codeString)) 140 return COUNT; 141 if ("totalcount".equals(codeString)) 142 return TOTALCOUNT; 143 if ("median".equals(codeString)) 144 return MEDIAN; 145 if ("std-dev".equals(codeString)) 146 return STDDEV; 147 if ("sum".equals(codeString)) 148 return SUM; 149 if ("variance".equals(codeString)) 150 return VARIANCE; 151 if ("20-percent".equals(codeString)) 152 return _20PERCENT; 153 if ("80-percent".equals(codeString)) 154 return _80PERCENT; 155 if ("4-lower".equals(codeString)) 156 return _4LOWER; 157 if ("4-upper".equals(codeString)) 158 return _4UPPER; 159 if ("4-dev".equals(codeString)) 160 return _4DEV; 161 if ("5-1".equals(codeString)) 162 return _51; 163 if ("5-2".equals(codeString)) 164 return _52; 165 if ("5-3".equals(codeString)) 166 return _53; 167 if ("5-4".equals(codeString)) 168 return _54; 169 if ("skew".equals(codeString)) 170 return SKEW; 171 if ("kurtosis".equals(codeString)) 172 return KURTOSIS; 173 if ("regression".equals(codeString)) 174 return REGRESSION; 175 throw new FHIRException("Unknown ObservationStatistics code '"+codeString+"'"); 176 } 177 public String toCode() { 178 switch (this) { 179 case AVERAGE: return "average"; 180 case MAXIMUM: return "maximum"; 181 case MINIMUM: return "minimum"; 182 case COUNT: return "count"; 183 case TOTALCOUNT: return "totalcount"; 184 case MEDIAN: return "median"; 185 case STDDEV: return "std-dev"; 186 case SUM: return "sum"; 187 case VARIANCE: return "variance"; 188 case _20PERCENT: return "20-percent"; 189 case _80PERCENT: return "80-percent"; 190 case _4LOWER: return "4-lower"; 191 case _4UPPER: return "4-upper"; 192 case _4DEV: return "4-dev"; 193 case _51: return "5-1"; 194 case _52: return "5-2"; 195 case _53: return "5-3"; 196 case _54: return "5-4"; 197 case SKEW: return "skew"; 198 case KURTOSIS: return "kurtosis"; 199 case REGRESSION: return "regression"; 200 case NULL: return null; 201 default: return "?"; 202 } 203 } 204 public String getSystem() { 205 return "http://hl7.org/fhir/observation-statistics"; 206 } 207 public String getDefinition() { 208 switch (this) { 209 case AVERAGE: return "The [mean](https://en.wikipedia.org/wiki/Arithmetic_mean) of N measurements over the stated period"; 210 case MAXIMUM: return "The [maximum](https://en.wikipedia.org/wiki/Maximal_element) value of N measurements over the stated period"; 211 case MINIMUM: return "The [minimum](https://en.wikipedia.org/wiki/Minimal_element) value of N measurements over the stated period"; 212 case COUNT: return "The [number] of valid measurements over the stated period that contributed to the other statistical outputs"; 213 case TOTALCOUNT: return "The total [number] of valid measurements over the stated period, including observations that were ignored because they did not contain valid result values"; 214 case MEDIAN: return "The [median](https://en.wikipedia.org/wiki/Median) of N measurements over the stated period"; 215 case STDDEV: return "The [standard deviation](https://en.wikipedia.org/wiki/Standard_deviation) of N measurements over the stated period"; 216 case SUM: return "The [sum](https://en.wikipedia.org/wiki/Summation) of N measurements over the stated period"; 217 case VARIANCE: return "The [variance](https://en.wikipedia.org/wiki/Variance) of N measurements over the stated period"; 218 case _20PERCENT: return "The 20th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N measurements over the stated period"; 219 case _80PERCENT: return "The 80th [Percentile](https://en.wikipedia.org/wiki/Percentile) of N measurements over the stated period"; 220 case _4LOWER: return "The lower [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N measurements over the stated period"; 221 case _4UPPER: return "The upper [Quartile](https://en.wikipedia.org/wiki/Quartile) Boundary of N measurements over the stated period"; 222 case _4DEV: return "The difference between the upper and lower [Quartiles](https://en.wikipedia.org/wiki/Quartile) is called the Interquartile range. (IQR = Q3-Q1) Quartile deviation or Semi-interquartile range is one-half the difference between the first and the third quartiles."; 223 case _51: return "The lowest of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population"; 224 case _52: return "The second of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population"; 225 case _53: return "The third of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population"; 226 case _54: return "The fourth of four values that divide the N measurements into a frequency distribution of five classes with each containing one fifth of the total population"; 227 case SKEW: return "Skewness is a measure of the asymmetry of the probability distribution of a real-valued random variable about its mean. The skewness value can be positive or negative, or even undefined. Source: [Wikipedia](https://en.wikipedia.org/wiki/Skewness)"; 228 case KURTOSIS: return "Kurtosis is a measure of the \"tailedness\" of the probability distribution of a real-valued random variable. Source: [Wikipedia](https://en.wikipedia.org/wiki/Kurtosis)"; 229 case REGRESSION: return "Linear regression is an approach for modeling two-dimensional sample points with one independent variable and one dependent variable (conventionally, the x and y coordinates in a Cartesian coordinate system) and finds a linear function (a non-vertical straight line) that, as accurately as possible, predicts the dependent variable values as a function of the independent variables. Source: [Wikipedia](https://en.wikipedia.org/wiki/Simple_linear_regression) This Statistic code will return both a gradient and an intercept value."; 230 case NULL: return null; 231 default: return "?"; 232 } 233 } 234 public String getDisplay() { 235 switch (this) { 236 case AVERAGE: return "Average"; 237 case MAXIMUM: return "Maximum"; 238 case MINIMUM: return "Minimum"; 239 case COUNT: return "Count"; 240 case TOTALCOUNT: return "Total Count"; 241 case MEDIAN: return "Median"; 242 case STDDEV: return "Standard Deviation"; 243 case SUM: return "Sum"; 244 case VARIANCE: return "Variance"; 245 case _20PERCENT: return "20th Percentile"; 246 case _80PERCENT: return "80th Percentile"; 247 case _4LOWER: return "Lower Quartile"; 248 case _4UPPER: return "Upper Quartile"; 249 case _4DEV: return "Quartile Deviation"; 250 case _51: return "1st Quintile"; 251 case _52: return "2nd Quintile"; 252 case _53: return "3rd Quintile"; 253 case _54: return "4th Quintile"; 254 case SKEW: return "Skew"; 255 case KURTOSIS: return "Kurtosis"; 256 case REGRESSION: return "Regression"; 257 case NULL: return null; 258 default: return "?"; 259 } 260 } 261 262 263}