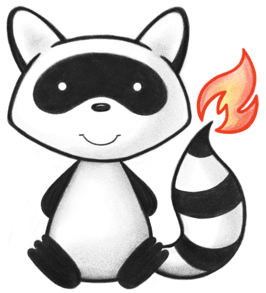
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum OrganizationType { 041 042 /** 043 * An organization that provides healthcare services. 044 */ 045 PROV, 046 /** 047 * A department or ward within a hospital (Generally is not applicable to top level organizations) 048 */ 049 DEPT, 050 /** 051 * An organizational team is usually a grouping of practitioners that perform a specific function within an organization (which could be a top level organization, or a department). 052 */ 053 TEAM, 054 /** 055 * A political body, often used when including organization records for government bodies such as a Federal Government, State or Local Government. 056 */ 057 GOVT, 058 /** 059 * A company that provides insurance to its subscribers that may include healthcare related policies. 060 */ 061 INS, 062 /** 063 * An educational institution that provides education or research facilities. 064 */ 065 EDU, 066 /** 067 * An organization that is identified as a part of a religious institution. 068 */ 069 RELI, 070 /** 071 * An organization that is identified as a Pharmaceutical/Clinical Research Sponsor. 072 */ 073 CRS, 074 /** 075 * An un-incorporated community group. 076 */ 077 CG, 078 /** 079 * An organization that is a registered business or corporation but not identified by other types. 080 */ 081 BUS, 082 /** 083 * Other type of organization not already specified. 084 */ 085 OTHER, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 public static OrganizationType fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("prov".equals(codeString)) 094 return PROV; 095 if ("dept".equals(codeString)) 096 return DEPT; 097 if ("team".equals(codeString)) 098 return TEAM; 099 if ("govt".equals(codeString)) 100 return GOVT; 101 if ("ins".equals(codeString)) 102 return INS; 103 if ("edu".equals(codeString)) 104 return EDU; 105 if ("reli".equals(codeString)) 106 return RELI; 107 if ("crs".equals(codeString)) 108 return CRS; 109 if ("cg".equals(codeString)) 110 return CG; 111 if ("bus".equals(codeString)) 112 return BUS; 113 if ("other".equals(codeString)) 114 return OTHER; 115 throw new FHIRException("Unknown OrganizationType code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case PROV: return "prov"; 120 case DEPT: return "dept"; 121 case TEAM: return "team"; 122 case GOVT: return "govt"; 123 case INS: return "ins"; 124 case EDU: return "edu"; 125 case RELI: return "reli"; 126 case CRS: return "crs"; 127 case CG: return "cg"; 128 case BUS: return "bus"; 129 case OTHER: return "other"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getSystem() { 135 return "http://hl7.org/fhir/organization-type"; 136 } 137 public String getDefinition() { 138 switch (this) { 139 case PROV: return "An organization that provides healthcare services."; 140 case DEPT: return "A department or ward within a hospital (Generally is not applicable to top level organizations)"; 141 case TEAM: return "An organizational team is usually a grouping of practitioners that perform a specific function within an organization (which could be a top level organization, or a department)."; 142 case GOVT: return "A political body, often used when including organization records for government bodies such as a Federal Government, State or Local Government."; 143 case INS: return "A company that provides insurance to its subscribers that may include healthcare related policies."; 144 case EDU: return "An educational institution that provides education or research facilities."; 145 case RELI: return "An organization that is identified as a part of a religious institution."; 146 case CRS: return "An organization that is identified as a Pharmaceutical/Clinical Research Sponsor."; 147 case CG: return "An un-incorporated community group."; 148 case BUS: return "An organization that is a registered business or corporation but not identified by other types."; 149 case OTHER: return "Other type of organization not already specified."; 150 case NULL: return null; 151 default: return "?"; 152 } 153 } 154 public String getDisplay() { 155 switch (this) { 156 case PROV: return "Healthcare Provider"; 157 case DEPT: return "Hospital Department"; 158 case TEAM: return "Organizational team"; 159 case GOVT: return "Government"; 160 case INS: return "Insurance Company"; 161 case EDU: return "Educational Institute"; 162 case RELI: return "Religious Institution"; 163 case CRS: return "Clinical Research Sponsor"; 164 case CG: return "Community Group"; 165 case BUS: return "Non-Healthcare Business or Corporation"; 166 case OTHER: return "Other"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 172 173}