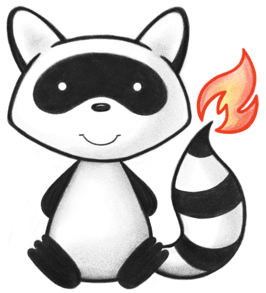
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ParameterGroup { 041 042 /** 043 * Haemodynamic Parameter Group - MDC_PGRP_HEMO 044 */ 045 HAEMODYNAMIC, 046 /** 047 * ECG Parameter Group - MDC_PGRP_ECG 048 */ 049 ECG, 050 /** 051 * Respiratory Parameter Group - MDC_PGRP_RESP 052 */ 053 RESPIRATORY, 054 /** 055 * Ventilation Parameter Group - MDC_PGRP_VENT 056 */ 057 VENTILATION, 058 /** 059 * Neurological Parameter Group - MDC_PGRP_NEURO 060 */ 061 NEUROLOGICAL, 062 /** 063 * Drug Delivery Parameter Group - MDC_PGRP_DRUG 064 */ 065 DRUGDELIVERY, 066 /** 067 * Fluid Chemistry Parameter Group - MDC_PGRP_FLUID 068 */ 069 FLUIDCHEMISTRY, 070 /** 071 * Blood Chemistry Parameter Group - MDC_PGRP_BLOOD_CHEM 072 */ 073 BLOODCHEMISTRY, 074 /** 075 * Miscellaneous Parameter Group - MDC_PGRP_MISC 076 */ 077 MISCELLANEOUS, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 public static ParameterGroup fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("haemodynamic".equals(codeString)) 086 return HAEMODYNAMIC; 087 if ("ecg".equals(codeString)) 088 return ECG; 089 if ("respiratory".equals(codeString)) 090 return RESPIRATORY; 091 if ("ventilation".equals(codeString)) 092 return VENTILATION; 093 if ("neurological".equals(codeString)) 094 return NEUROLOGICAL; 095 if ("drug-delivery".equals(codeString)) 096 return DRUGDELIVERY; 097 if ("fluid-chemistry".equals(codeString)) 098 return FLUIDCHEMISTRY; 099 if ("blood-chemistry".equals(codeString)) 100 return BLOODCHEMISTRY; 101 if ("miscellaneous".equals(codeString)) 102 return MISCELLANEOUS; 103 throw new FHIRException("Unknown ParameterGroup code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case HAEMODYNAMIC: return "haemodynamic"; 108 case ECG: return "ecg"; 109 case RESPIRATORY: return "respiratory"; 110 case VENTILATION: return "ventilation"; 111 case NEUROLOGICAL: return "neurological"; 112 case DRUGDELIVERY: return "drug-delivery"; 113 case FLUIDCHEMISTRY: return "fluid-chemistry"; 114 case BLOODCHEMISTRY: return "blood-chemistry"; 115 case MISCELLANEOUS: return "miscellaneous"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 return "http://hl7.org/fhir/parameter-group"; 122 } 123 public String getDefinition() { 124 switch (this) { 125 case HAEMODYNAMIC: return "Haemodynamic Parameter Group - MDC_PGRP_HEMO"; 126 case ECG: return "ECG Parameter Group - MDC_PGRP_ECG"; 127 case RESPIRATORY: return "Respiratory Parameter Group - MDC_PGRP_RESP"; 128 case VENTILATION: return "Ventilation Parameter Group - MDC_PGRP_VENT"; 129 case NEUROLOGICAL: return "Neurological Parameter Group - MDC_PGRP_NEURO"; 130 case DRUGDELIVERY: return "Drug Delivery Parameter Group - MDC_PGRP_DRUG"; 131 case FLUIDCHEMISTRY: return "Fluid Chemistry Parameter Group - MDC_PGRP_FLUID"; 132 case BLOODCHEMISTRY: return "Blood Chemistry Parameter Group - MDC_PGRP_BLOOD_CHEM"; 133 case MISCELLANEOUS: return "Miscellaneous Parameter Group - MDC_PGRP_MISC"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case HAEMODYNAMIC: return "Haemodynamic Parameter Group"; 141 case ECG: return "ECG Parameter Group"; 142 case RESPIRATORY: return "Respiratory Parameter Group"; 143 case VENTILATION: return "Ventilation Parameter Group"; 144 case NEUROLOGICAL: return "Neurological Parameter Group"; 145 case DRUGDELIVERY: return "Drug Delivery Parameter Group"; 146 case FLUIDCHEMISTRY: return "Fluid Chemistry Parameter Group"; 147 case BLOODCHEMISTRY: return "Blood Chemistry Parameter Group"; 148 case MISCELLANEOUS: return "Miscellaneous Parameter Group"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 154 155}