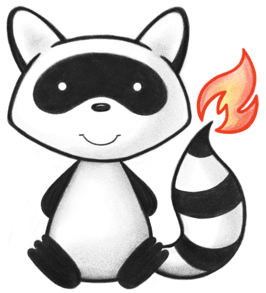
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Participantrequired { 041 042 /** 043 * The participant is required to attend the appointment. 044 */ 045 REQUIRED, 046 /** 047 * The participant may optionally attend the appointment. 048 */ 049 OPTIONAL, 050 /** 051 * The participant is excluded from the appointment, and may not be informed of the appointment taking place. (Appointment is about them, not for them - such as 2 doctors discussing results about a patient's test). 052 */ 053 INFORMATIONONLY, 054 /** 055 * added to help the parsers 056 */ 057 NULL; 058 public static Participantrequired fromCode(String codeString) throws FHIRException { 059 if (codeString == null || "".equals(codeString)) 060 return null; 061 if ("required".equals(codeString)) 062 return REQUIRED; 063 if ("optional".equals(codeString)) 064 return OPTIONAL; 065 if ("information-only".equals(codeString)) 066 return INFORMATIONONLY; 067 throw new FHIRException("Unknown Participantrequired code '"+codeString+"'"); 068 } 069 public String toCode() { 070 switch (this) { 071 case REQUIRED: return "required"; 072 case OPTIONAL: return "optional"; 073 case INFORMATIONONLY: return "information-only"; 074 case NULL: return null; 075 default: return "?"; 076 } 077 } 078 public String getSystem() { 079 return "http://hl7.org/fhir/participantrequired"; 080 } 081 public String getDefinition() { 082 switch (this) { 083 case REQUIRED: return "The participant is required to attend the appointment."; 084 case OPTIONAL: return "The participant may optionally attend the appointment."; 085 case INFORMATIONONLY: return "The participant is excluded from the appointment, and may not be informed of the appointment taking place. (Appointment is about them, not for them - such as 2 doctors discussing results about a patient's test)."; 086 case NULL: return null; 087 default: return "?"; 088 } 089 } 090 public String getDisplay() { 091 switch (this) { 092 case REQUIRED: return "Required"; 093 case OPTIONAL: return "Optional"; 094 case INFORMATIONONLY: return "Information Only"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 100 101}