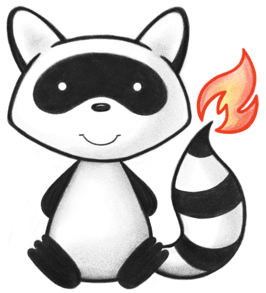
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Thu, Feb 9, 2017 08:03-0500 for FHIR v1.9.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ProvenanceAgentRole { 041 042 /** 043 * A person entering the data into the originating system 044 */ 045 ENTERER, 046 /** 047 * A person, animal, organization or device that who actually and principally carries out the activity 048 */ 049 PERFORMER, 050 /** 051 * A party that originates the resource and therefore has responsibility for the information given in the resource and ownership of this resource 052 */ 053 AUTHOR, 054 /** 055 * A person who verifies the correctness and appropriateness of activity 056 */ 057 VERIFIER, 058 /** 059 * The person authenticated the content and accepted legal responsibility for its content 060 */ 061 LEGAL, 062 /** 063 * A verifier who attests to the accuracy of the resource 064 */ 065 ATTESTER, 066 /** 067 * A person who reported information that contributed to the resource 068 */ 069 INFORMANT, 070 /** 071 * The entity that is accountable for maintaining a true an accurate copy of the original record 072 */ 073 CUSTODIAN, 074 /** 075 * A device that operates independently of an author on custodian's algorithms for data extraction of existing information for purpose of generating a new artifact. 076 */ 077 ASSEMBLER, 078 /** 079 * A device used by an author to record new information, which may also be used by the author to select existing information for aggregation with newly recorded information for the purpose of generating a new artifact. 080 */ 081 COMPOSER, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 public static ProvenanceAgentRole fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("enterer".equals(codeString)) 090 return ENTERER; 091 if ("performer".equals(codeString)) 092 return PERFORMER; 093 if ("author".equals(codeString)) 094 return AUTHOR; 095 if ("verifier".equals(codeString)) 096 return VERIFIER; 097 if ("legal".equals(codeString)) 098 return LEGAL; 099 if ("attester".equals(codeString)) 100 return ATTESTER; 101 if ("informant".equals(codeString)) 102 return INFORMANT; 103 if ("custodian".equals(codeString)) 104 return CUSTODIAN; 105 if ("assembler".equals(codeString)) 106 return ASSEMBLER; 107 if ("composer".equals(codeString)) 108 return COMPOSER; 109 throw new FHIRException("Unknown ProvenanceAgentRole code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case ENTERER: return "enterer"; 114 case PERFORMER: return "performer"; 115 case AUTHOR: return "author"; 116 case VERIFIER: return "verifier"; 117 case LEGAL: return "legal"; 118 case ATTESTER: return "attester"; 119 case INFORMANT: return "informant"; 120 case CUSTODIAN: return "custodian"; 121 case ASSEMBLER: return "assembler"; 122 case COMPOSER: return "composer"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getSystem() { 128 return "http://hl7.org/fhir/provenance-participant-role"; 129 } 130 public String getDefinition() { 131 switch (this) { 132 case ENTERER: return "A person entering the data into the originating system"; 133 case PERFORMER: return "A person, animal, organization or device that who actually and principally carries out the activity"; 134 case AUTHOR: return "A party that originates the resource and therefore has responsibility for the information given in the resource and ownership of this resource"; 135 case VERIFIER: return "A person who verifies the correctness and appropriateness of activity"; 136 case LEGAL: return "The person authenticated the content and accepted legal responsibility for its content"; 137 case ATTESTER: return "A verifier who attests to the accuracy of the resource"; 138 case INFORMANT: return "A person who reported information that contributed to the resource"; 139 case CUSTODIAN: return "The entity that is accountable for maintaining a true an accurate copy of the original record"; 140 case ASSEMBLER: return "A device that operates independently of an author on custodian's algorithms for data extraction of existing information for purpose of generating a new artifact."; 141 case COMPOSER: return "A device used by an author to record new information, which may also be used by the author to select existing information for aggregation with newly recorded information for the purpose of generating a new artifact."; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDisplay() { 147 switch (this) { 148 case ENTERER: return "Enterer"; 149 case PERFORMER: return "Performer"; 150 case AUTHOR: return "Author"; 151 case VERIFIER: return "Verifier"; 152 case LEGAL: return "Legal Authenticator"; 153 case ATTESTER: return "Attester"; 154 case INFORMANT: return "Informant"; 155 case CUSTODIAN: return "Custodian"; 156 case ASSEMBLER: return "Assembler"; 157 case COMPOSER: return "Composer"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 163 164}