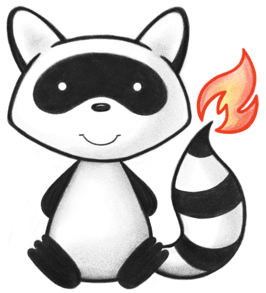
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ProvenanceEntityRole { 041 042 /** 043 * A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a preexisting entity. 044 */ 045 DERIVATION, 046 /** 047 * A derivation for which the resulting entity is a revised version of some original. 048 */ 049 REVISION, 050 /** 051 * The repeat of (some or all of) an entity, such as text or image, by someone who may or may not be its original author. 052 */ 053 QUOTATION, 054 /** 055 * A primary source for a topic refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight. 056 */ 057 SOURCE, 058 /** 059 * A derivation for which the entity is removed from accessibility usually through the use of the Delete operation. 060 */ 061 REMOVAL, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 public static ProvenanceEntityRole fromCode(String codeString) throws FHIRException { 067 if (codeString == null || "".equals(codeString)) 068 return null; 069 if ("derivation".equals(codeString)) 070 return DERIVATION; 071 if ("revision".equals(codeString)) 072 return REVISION; 073 if ("quotation".equals(codeString)) 074 return QUOTATION; 075 if ("source".equals(codeString)) 076 return SOURCE; 077 if ("removal".equals(codeString)) 078 return REMOVAL; 079 throw new FHIRException("Unknown ProvenanceEntityRole code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case DERIVATION: return "derivation"; 084 case REVISION: return "revision"; 085 case QUOTATION: return "quotation"; 086 case SOURCE: return "source"; 087 case REMOVAL: return "removal"; 088 case NULL: return null; 089 default: return "?"; 090 } 091 } 092 public String getSystem() { 093 return "http://hl7.org/fhir/provenance-entity-role"; 094 } 095 public String getDefinition() { 096 switch (this) { 097 case DERIVATION: return "A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a preexisting entity."; 098 case REVISION: return "A derivation for which the resulting entity is a revised version of some original."; 099 case QUOTATION: return "The repeat of (some or all of) an entity, such as text or image, by someone who may or may not be its original author."; 100 case SOURCE: return "A primary source for a topic refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight."; 101 case REMOVAL: return "A derivation for which the entity is removed from accessibility usually through the use of the Delete operation."; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getDisplay() { 107 switch (this) { 108 case DERIVATION: return "Derivation"; 109 case REVISION: return "Revision"; 110 case QUOTATION: return "Quotation"; 111 case SOURCE: return "Source"; 112 case REMOVAL: return "Removal"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 118 119}