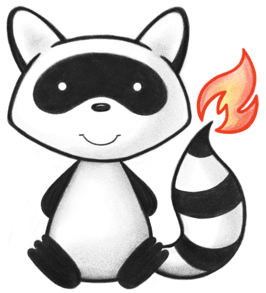
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum QuestionnaireItemControl { 041 042 /** 043 * UI controls relevant to organizing groups of questions 044 */ 045 GROUP, 046 /** 047 * Questions within the group should be listed sequentially 048 */ 049 LIST, 050 /** 051 * Questions within the group are rows in the table with possible answers as columns 052 */ 053 TABLE, 054 /** 055 * The group is to be continuously visible at the top of the questionnaire 056 */ 057 HEADER, 058 /** 059 * The group is to be continuously visible at the bottom of the questionnaire 060 */ 061 FOOTER, 062 /** 063 * UI controls relevant to rendering questionnaire text items 064 */ 065 TEXT, 066 /** 067 * Text is displayed as a paragraph in a sequential position between sibling items (default behavior) 068 */ 069 INLINE, 070 /** 071 * Text is displayed immediately below or within the answer-entry area of the containing question item (typically as a guide for what to enter) 072 */ 073 PROMPT, 074 /** 075 * Text is displayed adjacent (horizontally or vertically) to the answer space for the parent question, typically to indicate a unit of measure 076 */ 077 UNIT, 078 /** 079 * Text is displayed to the left of the set of answer choices or a scaling control for the parent question item to indicate the meaning of the 'lower' bound. E.g. 'Strongly disagree' 080 */ 081 LOWER, 082 /** 083 * Text is displayed to the right of the set of answer choices or a scaling control for the parent question item to indicate the meaning of the 'upper' bound. E.g. 'Strongly agree' 084 */ 085 UPPER, 086 /** 087 * Text is temporarily visible over top of an item if the mouse is positioned over top of the text for the containing item 088 */ 089 FLYOVER, 090 /** 091 * Text is displayed in a dialog box or similar control if invoked by pushing a button or some other UI-appropriate action to request 'help' for a question, group or the questionnaire as a whole (depending what the text is nested within) 092 */ 093 HELP, 094 /** 095 * UI controls relevant to capturing question data 096 */ 097 QUESTION, 098 /** 099 * A control which provides a list of potential matches based on text entered into a control. Used for large choice sets where text-matching is an appropriate discovery mechanism. 100 */ 101 AUTOCOMPLETE, 102 /** 103 * A control where an item (or multiple items) can be selected from a list that is only displayed when the user is editing the field. 104 */ 105 DROPDOWN, 106 /** 107 * A control where choices are listed with a box beside them. The box can be toggled to select or de-select a given choice. Multiple selections may be possible. 108 */ 109 CHECKBOX, 110 /** 111 * A control where editing an item spawns a separate dialog box or screen permitting a user to navigate, filter or otherwise discover an appropriate match. Useful for large choice sets where text matching is not an appropriate discovery mechanism. Such screens must generally be tuned for the specific choice list structure. 112 */ 113 LOOKUP, 114 /** 115 * A control where choices are listed with a button beside them. The button can be toggled to select or de-select a given choice. Selecting one item deselects all others. 116 */ 117 RADIOBUTTON, 118 /** 119 * A control where an axis is displayed between the high and low values and the control can be visually manipulated to select a value anywhere on the axis. 120 */ 121 SLIDER, 122 /** 123 * A control where a list of numeric or other ordered values can be scrolled through via arrows. 124 */ 125 SPINNER, 126 /** 127 * A control where a user can type in their answer freely. 128 */ 129 TEXTBOX, 130 /** 131 * added to help the parsers 132 */ 133 NULL; 134 public static QuestionnaireItemControl fromCode(String codeString) throws FHIRException { 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("group".equals(codeString)) 138 return GROUP; 139 if ("list".equals(codeString)) 140 return LIST; 141 if ("table".equals(codeString)) 142 return TABLE; 143 if ("header".equals(codeString)) 144 return HEADER; 145 if ("footer".equals(codeString)) 146 return FOOTER; 147 if ("text".equals(codeString)) 148 return TEXT; 149 if ("inline".equals(codeString)) 150 return INLINE; 151 if ("prompt".equals(codeString)) 152 return PROMPT; 153 if ("unit".equals(codeString)) 154 return UNIT; 155 if ("lower".equals(codeString)) 156 return LOWER; 157 if ("upper".equals(codeString)) 158 return UPPER; 159 if ("flyover".equals(codeString)) 160 return FLYOVER; 161 if ("help".equals(codeString)) 162 return HELP; 163 if ("question".equals(codeString)) 164 return QUESTION; 165 if ("autocomplete".equals(codeString)) 166 return AUTOCOMPLETE; 167 if ("drop-down".equals(codeString)) 168 return DROPDOWN; 169 if ("check-box".equals(codeString)) 170 return CHECKBOX; 171 if ("lookup".equals(codeString)) 172 return LOOKUP; 173 if ("radio-button".equals(codeString)) 174 return RADIOBUTTON; 175 if ("slider".equals(codeString)) 176 return SLIDER; 177 if ("spinner".equals(codeString)) 178 return SPINNER; 179 if ("text-box".equals(codeString)) 180 return TEXTBOX; 181 throw new FHIRException("Unknown QuestionnaireItemControl code '"+codeString+"'"); 182 } 183 public String toCode() { 184 switch (this) { 185 case GROUP: return "group"; 186 case LIST: return "list"; 187 case TABLE: return "table"; 188 case HEADER: return "header"; 189 case FOOTER: return "footer"; 190 case TEXT: return "text"; 191 case INLINE: return "inline"; 192 case PROMPT: return "prompt"; 193 case UNIT: return "unit"; 194 case LOWER: return "lower"; 195 case UPPER: return "upper"; 196 case FLYOVER: return "flyover"; 197 case HELP: return "help"; 198 case QUESTION: return "question"; 199 case AUTOCOMPLETE: return "autocomplete"; 200 case DROPDOWN: return "drop-down"; 201 case CHECKBOX: return "check-box"; 202 case LOOKUP: return "lookup"; 203 case RADIOBUTTON: return "radio-button"; 204 case SLIDER: return "slider"; 205 case SPINNER: return "spinner"; 206 case TEXTBOX: return "text-box"; 207 case NULL: return null; 208 default: return "?"; 209 } 210 } 211 public String getSystem() { 212 return "http://hl7.org/fhir/questionnaire-item-control"; 213 } 214 public String getDefinition() { 215 switch (this) { 216 case GROUP: return "UI controls relevant to organizing groups of questions"; 217 case LIST: return "Questions within the group should be listed sequentially"; 218 case TABLE: return "Questions within the group are rows in the table with possible answers as columns"; 219 case HEADER: return "The group is to be continuously visible at the top of the questionnaire"; 220 case FOOTER: return "The group is to be continuously visible at the bottom of the questionnaire"; 221 case TEXT: return "UI controls relevant to rendering questionnaire text items"; 222 case INLINE: return "Text is displayed as a paragraph in a sequential position between sibling items (default behavior)"; 223 case PROMPT: return "Text is displayed immediately below or within the answer-entry area of the containing question item (typically as a guide for what to enter)"; 224 case UNIT: return "Text is displayed adjacent (horizontally or vertically) to the answer space for the parent question, typically to indicate a unit of measure"; 225 case LOWER: return "Text is displayed to the left of the set of answer choices or a scaling control for the parent question item to indicate the meaning of the 'lower' bound. E.g. 'Strongly disagree'"; 226 case UPPER: return "Text is displayed to the right of the set of answer choices or a scaling control for the parent question item to indicate the meaning of the 'upper' bound. E.g. 'Strongly agree'"; 227 case FLYOVER: return "Text is temporarily visible over top of an item if the mouse is positioned over top of the text for the containing item"; 228 case HELP: return "Text is displayed in a dialog box or similar control if invoked by pushing a button or some other UI-appropriate action to request 'help' for a question, group or the questionnaire as a whole (depending what the text is nested within)"; 229 case QUESTION: return "UI controls relevant to capturing question data"; 230 case AUTOCOMPLETE: return "A control which provides a list of potential matches based on text entered into a control. Used for large choice sets where text-matching is an appropriate discovery mechanism."; 231 case DROPDOWN: return "A control where an item (or multiple items) can be selected from a list that is only displayed when the user is editing the field."; 232 case CHECKBOX: return "A control where choices are listed with a box beside them. The box can be toggled to select or de-select a given choice. Multiple selections may be possible."; 233 case LOOKUP: return "A control where editing an item spawns a separate dialog box or screen permitting a user to navigate, filter or otherwise discover an appropriate match. Useful for large choice sets where text matching is not an appropriate discovery mechanism. Such screens must generally be tuned for the specific choice list structure."; 234 case RADIOBUTTON: return "A control where choices are listed with a button beside them. The button can be toggled to select or de-select a given choice. Selecting one item deselects all others."; 235 case SLIDER: return "A control where an axis is displayed between the high and low values and the control can be visually manipulated to select a value anywhere on the axis."; 236 case SPINNER: return "A control where a list of numeric or other ordered values can be scrolled through via arrows."; 237 case TEXTBOX: return "A control where a user can type in their answer freely."; 238 case NULL: return null; 239 default: return "?"; 240 } 241 } 242 public String getDisplay() { 243 switch (this) { 244 case GROUP: return "group"; 245 case LIST: return "List"; 246 case TABLE: return "Table"; 247 case HEADER: return "Header"; 248 case FOOTER: return "Footer"; 249 case TEXT: return "text"; 250 case INLINE: return "In-line"; 251 case PROMPT: return "Prompt"; 252 case UNIT: return "Unit"; 253 case LOWER: return "Lower-bound"; 254 case UPPER: return "Upper-bound"; 255 case FLYOVER: return "Fly-over"; 256 case HELP: return "Help-Button"; 257 case QUESTION: return "question"; 258 case AUTOCOMPLETE: return "Auto-complete"; 259 case DROPDOWN: return "Drop down"; 260 case CHECKBOX: return "Check-box"; 261 case LOOKUP: return "Lookup"; 262 case RADIOBUTTON: return "Radio Button"; 263 case SLIDER: return "Slider"; 264 case SPINNER: return "Spinner"; 265 case TEXTBOX: return "Text Box"; 266 case NULL: return null; 267 default: return "?"; 268 } 269 } 270 271 272}