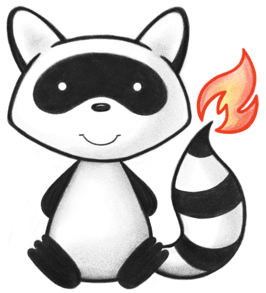
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ReferencerangeMeaning { 041 042 /** 043 * General types of reference range. 044 */ 045 TYPE, 046 /** 047 * Based on 95th percentile for the relevant control population. 048 */ 049 NORMAL, 050 /** 051 * The range that is recommended by a relevant professional body. 052 */ 053 RECOMMENDED, 054 /** 055 * The range at which treatment would/should be considered. 056 */ 057 TREATMENT, 058 /** 059 * The optimal range for best therapeutic outcomes. 060 */ 061 THERAPEUTIC, 062 /** 063 * The optimal range for best therapeutic outcomes for a specimen taken immediately before administration. 064 */ 065 PRE, 066 /** 067 * The optimal range for best therapeutic outcomes for a specimen taken immediately after administration. 068 */ 069 POST, 070 /** 071 * Endocrine related states that change the expected value. 072 */ 073 ENDOCRINE, 074 /** 075 * An expected range in an individual prior to puberty. 076 */ 077 PREPUBERTY, 078 /** 079 * An expected range in an individual during the follicular stage of the cycle. 080 */ 081 FOLLICULAR, 082 /** 083 * An expected range in an individual during the follicular stage of the cycle. 084 */ 085 MIDCYCLE, 086 /** 087 * An expected range in an individual during the luteal stage of the cycle. 088 */ 089 LUTEAL, 090 /** 091 * An expected range in an individual post-menopause. 092 */ 093 POSTMEOPAUSAL, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 public static ReferencerangeMeaning fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("type".equals(codeString)) 102 return TYPE; 103 if ("normal".equals(codeString)) 104 return NORMAL; 105 if ("recommended".equals(codeString)) 106 return RECOMMENDED; 107 if ("treatment".equals(codeString)) 108 return TREATMENT; 109 if ("therapeutic".equals(codeString)) 110 return THERAPEUTIC; 111 if ("pre".equals(codeString)) 112 return PRE; 113 if ("post".equals(codeString)) 114 return POST; 115 if ("endocrine".equals(codeString)) 116 return ENDOCRINE; 117 if ("pre-puberty".equals(codeString)) 118 return PREPUBERTY; 119 if ("follicular".equals(codeString)) 120 return FOLLICULAR; 121 if ("midcycle".equals(codeString)) 122 return MIDCYCLE; 123 if ("luteal".equals(codeString)) 124 return LUTEAL; 125 if ("postmeopausal".equals(codeString)) 126 return POSTMEOPAUSAL; 127 throw new FHIRException("Unknown ReferencerangeMeaning code '"+codeString+"'"); 128 } 129 public String toCode() { 130 switch (this) { 131 case TYPE: return "type"; 132 case NORMAL: return "normal"; 133 case RECOMMENDED: return "recommended"; 134 case TREATMENT: return "treatment"; 135 case THERAPEUTIC: return "therapeutic"; 136 case PRE: return "pre"; 137 case POST: return "post"; 138 case ENDOCRINE: return "endocrine"; 139 case PREPUBERTY: return "pre-puberty"; 140 case FOLLICULAR: return "follicular"; 141 case MIDCYCLE: return "midcycle"; 142 case LUTEAL: return "luteal"; 143 case POSTMEOPAUSAL: return "postmeopausal"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getSystem() { 149 return "http://hl7.org/fhir/referencerange-meaning"; 150 } 151 public String getDefinition() { 152 switch (this) { 153 case TYPE: return "General types of reference range."; 154 case NORMAL: return "Based on 95th percentile for the relevant control population."; 155 case RECOMMENDED: return "The range that is recommended by a relevant professional body."; 156 case TREATMENT: return "The range at which treatment would/should be considered."; 157 case THERAPEUTIC: return "The optimal range for best therapeutic outcomes."; 158 case PRE: return "The optimal range for best therapeutic outcomes for a specimen taken immediately before administration."; 159 case POST: return "The optimal range for best therapeutic outcomes for a specimen taken immediately after administration."; 160 case ENDOCRINE: return "Endocrine related states that change the expected value."; 161 case PREPUBERTY: return "An expected range in an individual prior to puberty."; 162 case FOLLICULAR: return "An expected range in an individual during the follicular stage of the cycle."; 163 case MIDCYCLE: return "An expected range in an individual during the follicular stage of the cycle."; 164 case LUTEAL: return "An expected range in an individual during the luteal stage of the cycle."; 165 case POSTMEOPAUSAL: return "An expected range in an individual post-menopause."; 166 case NULL: return null; 167 default: return "?"; 168 } 169 } 170 public String getDisplay() { 171 switch (this) { 172 case TYPE: return "Type"; 173 case NORMAL: return "Normal Range"; 174 case RECOMMENDED: return "Recommended Range"; 175 case TREATMENT: return "Treatment Range"; 176 case THERAPEUTIC: return "Therapeutic Desired Level"; 177 case PRE: return "Pre Therapeutic Desired Level"; 178 case POST: return "Post Therapeutic Desired Level"; 179 case ENDOCRINE: return "Endocrine"; 180 case PREPUBERTY: return "Pre-Puberty"; 181 case FOLLICULAR: return "Follicular Stage"; 182 case MIDCYCLE: return "MidCycle"; 183 case LUTEAL: return "Luteal"; 184 case POSTMEOPAUSAL: return "Post-Menopause"; 185 case NULL: return null; 186 default: return "?"; 187 } 188 } 189 190 191}