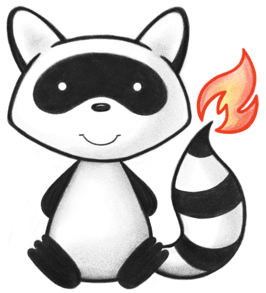
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum RelatedArtifactType { 041 042 /** 043 * Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness 044 */ 045 DOCUMENTATION, 046 /** 047 * A summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource 048 */ 049 JUSTIFICATION, 050 /** 051 * Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource 052 */ 053 CITATION, 054 /** 055 * The previous version of the knowledge resource 056 */ 057 PREDECESSOR, 058 /** 059 * The next version of the knowledge resource 060 */ 061 SUCCESSOR, 062 /** 063 * The knowledge resource is derived from the related artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting 064 */ 065 DERIVEDFROM, 066 /** 067 * The knowledge resource depends on the given related artifact 068 */ 069 DEPENDSON, 070 /** 071 * The knowledge resource is composed of the given related artifact 072 */ 073 COMPOSEDOF, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 public static RelatedArtifactType fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("documentation".equals(codeString)) 082 return DOCUMENTATION; 083 if ("justification".equals(codeString)) 084 return JUSTIFICATION; 085 if ("citation".equals(codeString)) 086 return CITATION; 087 if ("predecessor".equals(codeString)) 088 return PREDECESSOR; 089 if ("successor".equals(codeString)) 090 return SUCCESSOR; 091 if ("derived-from".equals(codeString)) 092 return DERIVEDFROM; 093 if ("depends-on".equals(codeString)) 094 return DEPENDSON; 095 if ("composed-of".equals(codeString)) 096 return COMPOSEDOF; 097 throw new FHIRException("Unknown RelatedArtifactType code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case DOCUMENTATION: return "documentation"; 102 case JUSTIFICATION: return "justification"; 103 case CITATION: return "citation"; 104 case PREDECESSOR: return "predecessor"; 105 case SUCCESSOR: return "successor"; 106 case DERIVEDFROM: return "derived-from"; 107 case DEPENDSON: return "depends-on"; 108 case COMPOSEDOF: return "composed-of"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getSystem() { 114 return "http://hl7.org/fhir/related-artifact-type"; 115 } 116 public String getDefinition() { 117 switch (this) { 118 case DOCUMENTATION: return "Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness"; 119 case JUSTIFICATION: return "A summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource"; 120 case CITATION: return "Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource"; 121 case PREDECESSOR: return "The previous version of the knowledge resource"; 122 case SUCCESSOR: return "The next version of the knowledge resource"; 123 case DERIVEDFROM: return "The knowledge resource is derived from the related artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting"; 124 case DEPENDSON: return "The knowledge resource depends on the given related artifact"; 125 case COMPOSEDOF: return "The knowledge resource is composed of the given related artifact"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case DOCUMENTATION: return "Documentation"; 133 case JUSTIFICATION: return "Justification"; 134 case CITATION: return "Citation"; 135 case PREDECESSOR: return "Predecessor"; 136 case SUCCESSOR: return "Successor"; 137 case DERIVEDFROM: return "Derived From"; 138 case DEPENDSON: return "Depends On"; 139 case COMPOSEDOF: return "Composed Of"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 145 146}