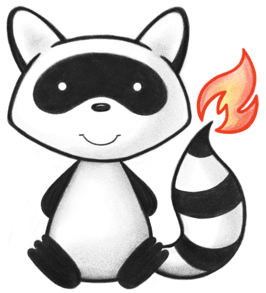
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum RequestIntent { 041 042 /** 043 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 044 */ 045 PROPOSAL, 046 /** 047 * The request represents an intension to ensure something occurs without providing an authorization for others to act 048 */ 049 PLAN, 050 /** 051 * The request represents a request/demand and authorization for action 052 */ 053 ORDER, 054 /** 055 * The request represents an original authorization for action 056 */ 057 ORIGINALORDER, 058 /** 059 * The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization 060 */ 061 REFLEXORDER, 062 /** 063 * The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order 064 */ 065 FILLERORDER, 066 /** 067 * An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug. 068 */ 069 INSTANCEORDER, 070 /** 071 * The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. 072 073Refer to [[[RequestGroup]]] for additional information on how this status is used 074 */ 075 OPTION, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 public static RequestIntent fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("proposal".equals(codeString)) 084 return PROPOSAL; 085 if ("plan".equals(codeString)) 086 return PLAN; 087 if ("order".equals(codeString)) 088 return ORDER; 089 if ("original-order".equals(codeString)) 090 return ORIGINALORDER; 091 if ("reflex-order".equals(codeString)) 092 return REFLEXORDER; 093 if ("filler-order".equals(codeString)) 094 return FILLERORDER; 095 if ("instance-order".equals(codeString)) 096 return INSTANCEORDER; 097 if ("option".equals(codeString)) 098 return OPTION; 099 throw new FHIRException("Unknown RequestIntent code '"+codeString+"'"); 100 } 101 public String toCode() { 102 switch (this) { 103 case PROPOSAL: return "proposal"; 104 case PLAN: return "plan"; 105 case ORDER: return "order"; 106 case ORIGINALORDER: return "original-order"; 107 case REFLEXORDER: return "reflex-order"; 108 case FILLERORDER: return "filler-order"; 109 case INSTANCEORDER: return "instance-order"; 110 case OPTION: return "option"; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getSystem() { 116 return "http://hl7.org/fhir/request-intent"; 117 } 118 public String getDefinition() { 119 switch (this) { 120 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 121 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 122 case ORDER: return "The request represents a request/demand and authorization for action"; 123 case ORIGINALORDER: return "The request represents an original authorization for action"; 124 case REFLEXORDER: return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization"; 125 case FILLERORDER: return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order"; 126 case INSTANCEORDER: return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 127 case OPTION: return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests.\n\nRefer to [[[RequestGroup]]] for additional information on how this status is used"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case PROPOSAL: return "Proposal"; 135 case PLAN: return "Plan"; 136 case ORDER: return "Order"; 137 case ORIGINALORDER: return "Original Order"; 138 case REFLEXORDER: return "Reflex Order"; 139 case FILLERORDER: return "Filler Order"; 140 case INSTANCEORDER: return "Instance Order"; 141 case OPTION: return "Option"; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 147 148}