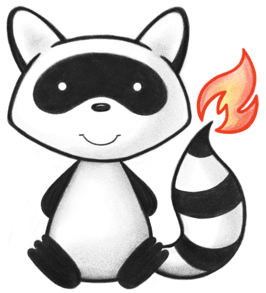
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum RestfulInteraction { 041 042 /** 043 * Read the current state of the resource. 044 */ 045 READ, 046 /** 047 * Read the state of a specific version of the resource. 048 */ 049 VREAD, 050 /** 051 * Update an existing resource by its id (or create it if it is new). 052 */ 053 UPDATE, 054 /** 055 * Update an existing resource by posting a set of changes to it. 056 */ 057 PATCH, 058 /** 059 * Delete a resource. 060 */ 061 DELETE, 062 /** 063 * Retrieve the change history for a particular resource, type of resource, or the entire system. 064 */ 065 HISTORY, 066 /** 067 * Retrieve the change history for a particular resource. 068 */ 069 HISTORYINSTANCE, 070 /** 071 * Retrieve the change history for all resources of a particular type. 072 */ 073 HISTORYTYPE, 074 /** 075 * Retrieve the change history for all resources on a system. 076 */ 077 HISTORYSYSTEM, 078 /** 079 * Create a new resource with a server assigned id. 080 */ 081 CREATE, 082 /** 083 * Search a resource type or all resources based on some filter criteria. 084 */ 085 SEARCH, 086 /** 087 * Search all resources of the specified type based on some filter criteria. 088 */ 089 SEARCHTYPE, 090 /** 091 * Search all resources based on some filter criteria. 092 */ 093 SEARCHSYSTEM, 094 /** 095 * Get a Capability Statement for the system. 096 */ 097 CAPABILITIES, 098 /** 099 * Update, create or delete a set of resources as a single transaction. 100 */ 101 TRANSACTION, 102 /** 103 * perform a set of a separate interactions in a single http operation 104 */ 105 BATCH, 106 /** 107 * Perform an operation as defined by an OperationDefinition. 108 */ 109 OPERATION, 110 /** 111 * added to help the parsers 112 */ 113 NULL; 114 public static RestfulInteraction fromCode(String codeString) throws FHIRException { 115 if (codeString == null || "".equals(codeString)) 116 return null; 117 if ("read".equals(codeString)) 118 return READ; 119 if ("vread".equals(codeString)) 120 return VREAD; 121 if ("update".equals(codeString)) 122 return UPDATE; 123 if ("patch".equals(codeString)) 124 return PATCH; 125 if ("delete".equals(codeString)) 126 return DELETE; 127 if ("history".equals(codeString)) 128 return HISTORY; 129 if ("history-instance".equals(codeString)) 130 return HISTORYINSTANCE; 131 if ("history-type".equals(codeString)) 132 return HISTORYTYPE; 133 if ("history-system".equals(codeString)) 134 return HISTORYSYSTEM; 135 if ("create".equals(codeString)) 136 return CREATE; 137 if ("search".equals(codeString)) 138 return SEARCH; 139 if ("search-type".equals(codeString)) 140 return SEARCHTYPE; 141 if ("search-system".equals(codeString)) 142 return SEARCHSYSTEM; 143 if ("capabilities".equals(codeString)) 144 return CAPABILITIES; 145 if ("transaction".equals(codeString)) 146 return TRANSACTION; 147 if ("batch".equals(codeString)) 148 return BATCH; 149 if ("operation".equals(codeString)) 150 return OPERATION; 151 throw new FHIRException("Unknown RestfulInteraction code '"+codeString+"'"); 152 } 153 public String toCode() { 154 switch (this) { 155 case READ: return "read"; 156 case VREAD: return "vread"; 157 case UPDATE: return "update"; 158 case PATCH: return "patch"; 159 case DELETE: return "delete"; 160 case HISTORY: return "history"; 161 case HISTORYINSTANCE: return "history-instance"; 162 case HISTORYTYPE: return "history-type"; 163 case HISTORYSYSTEM: return "history-system"; 164 case CREATE: return "create"; 165 case SEARCH: return "search"; 166 case SEARCHTYPE: return "search-type"; 167 case SEARCHSYSTEM: return "search-system"; 168 case CAPABILITIES: return "capabilities"; 169 case TRANSACTION: return "transaction"; 170 case BATCH: return "batch"; 171 case OPERATION: return "operation"; 172 case NULL: return null; 173 default: return "?"; 174 } 175 } 176 public String getSystem() { 177 return "http://hl7.org/fhir/restful-interaction"; 178 } 179 public String getDefinition() { 180 switch (this) { 181 case READ: return "Read the current state of the resource."; 182 case VREAD: return "Read the state of a specific version of the resource."; 183 case UPDATE: return "Update an existing resource by its id (or create it if it is new)."; 184 case PATCH: return "Update an existing resource by posting a set of changes to it."; 185 case DELETE: return "Delete a resource."; 186 case HISTORY: return "Retrieve the change history for a particular resource, type of resource, or the entire system."; 187 case HISTORYINSTANCE: return "Retrieve the change history for a particular resource."; 188 case HISTORYTYPE: return "Retrieve the change history for all resources of a particular type."; 189 case HISTORYSYSTEM: return "Retrieve the change history for all resources on a system."; 190 case CREATE: return "Create a new resource with a server assigned id."; 191 case SEARCH: return "Search a resource type or all resources based on some filter criteria."; 192 case SEARCHTYPE: return "Search all resources of the specified type based on some filter criteria."; 193 case SEARCHSYSTEM: return "Search all resources based on some filter criteria."; 194 case CAPABILITIES: return "Get a Capability Statement for the system."; 195 case TRANSACTION: return "Update, create or delete a set of resources as a single transaction."; 196 case BATCH: return "perform a set of a separate interactions in a single http operation"; 197 case OPERATION: return "Perform an operation as defined by an OperationDefinition."; 198 case NULL: return null; 199 default: return "?"; 200 } 201 } 202 public String getDisplay() { 203 switch (this) { 204 case READ: return "read"; 205 case VREAD: return "vread"; 206 case UPDATE: return "update"; 207 case PATCH: return "patch"; 208 case DELETE: return "delete"; 209 case HISTORY: return "history"; 210 case HISTORYINSTANCE: return "history-instance"; 211 case HISTORYTYPE: return "history-type"; 212 case HISTORYSYSTEM: return "history-system"; 213 case CREATE: return "create"; 214 case SEARCH: return "search"; 215 case SEARCHTYPE: return "search-type"; 216 case SEARCHSYSTEM: return "search-system"; 217 case CAPABILITIES: return "capabilities"; 218 case TRANSACTION: return "transaction"; 219 case BATCH: return "batch"; 220 case OPERATION: return "operation"; 221 case NULL: return null; 222 default: return "?"; 223 } 224 } 225 226 227}