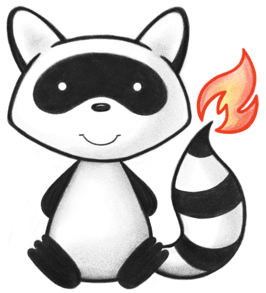
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum SearchComparator { 041 042 /** 043 * the value for the parameter in the resource is equal to the provided value 044 */ 045 EQ, 046 /** 047 * the value for the parameter in the resource is not equal to the provided value 048 */ 049 NE, 050 /** 051 * the value for the parameter in the resource is greater than the provided value 052 */ 053 GT, 054 /** 055 * the value for the parameter in the resource is less than the provided value 056 */ 057 LT, 058 /** 059 * the value for the parameter in the resource is greater or equal to the provided value 060 */ 061 GE, 062 /** 063 * the value for the parameter in the resource is less or equal to the provided value 064 */ 065 LE, 066 /** 067 * the value for the parameter in the resource starts after the provided value 068 */ 069 SA, 070 /** 071 * the value for the parameter in the resource ends before the provided value 072 */ 073 EB, 074 /** 075 * the value for the parameter in the resource is approximately the same to the provided value. 076 */ 077 AP, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 public static SearchComparator fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("eq".equals(codeString)) 086 return EQ; 087 if ("ne".equals(codeString)) 088 return NE; 089 if ("gt".equals(codeString)) 090 return GT; 091 if ("lt".equals(codeString)) 092 return LT; 093 if ("ge".equals(codeString)) 094 return GE; 095 if ("le".equals(codeString)) 096 return LE; 097 if ("sa".equals(codeString)) 098 return SA; 099 if ("eb".equals(codeString)) 100 return EB; 101 if ("ap".equals(codeString)) 102 return AP; 103 throw new FHIRException("Unknown SearchComparator code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case EQ: return "eq"; 108 case NE: return "ne"; 109 case GT: return "gt"; 110 case LT: return "lt"; 111 case GE: return "ge"; 112 case LE: return "le"; 113 case SA: return "sa"; 114 case EB: return "eb"; 115 case AP: return "ap"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 return "http://hl7.org/fhir/search-comparator"; 122 } 123 public String getDefinition() { 124 switch (this) { 125 case EQ: return "the value for the parameter in the resource is equal to the provided value"; 126 case NE: return "the value for the parameter in the resource is not equal to the provided value"; 127 case GT: return "the value for the parameter in the resource is greater than the provided value"; 128 case LT: return "the value for the parameter in the resource is less than the provided value"; 129 case GE: return "the value for the parameter in the resource is greater or equal to the provided value"; 130 case LE: return "the value for the parameter in the resource is less or equal to the provided value"; 131 case SA: return "the value for the parameter in the resource starts after the provided value"; 132 case EB: return "the value for the parameter in the resource ends before the provided value"; 133 case AP: return "the value for the parameter in the resource is approximately the same to the provided value."; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case EQ: return "Equals"; 141 case NE: return "Not Equals"; 142 case GT: return "Greater Than"; 143 case LT: return "Less Then"; 144 case GE: return "Greater or Equals"; 145 case LE: return "Less of Equal"; 146 case SA: return "Starts After"; 147 case EB: return "Ends Before"; 148 case AP: return "Approximately"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 154 155}