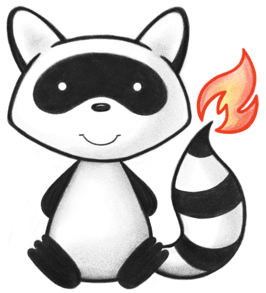
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ServiceCategory { 041 042 /** 043 * Adoption 044 */ 045 _1, 046 /** 047 * Aged Care 048 */ 049 _2, 050 /** 051 * Allied Health 052 */ 053 _34, 054 /** 055 * Alternative & Complementary Therapies 056 */ 057 _3, 058 /** 059 * Child Care and/or Kindergarten 060 */ 061 _4, 062 /** 063 * Child Development 064 */ 065 _5, 066 /** 067 * Child Protection & Family Services 068 */ 069 _6, 070 /** 071 * Community Health Care 072 */ 073 _7, 074 /** 075 * Counselling 076 */ 077 _8, 078 /** 079 * Crisis Line (GPAH use only) 080 */ 081 _36, 082 /** 083 * Death Services 084 */ 085 _9, 086 /** 087 * Dental 088 */ 089 _10, 090 /** 091 * Disability Support 092 */ 093 _11, 094 /** 095 * Drug/Alcohol 096 */ 097 _12, 098 /** 099 * Education & Learning 100 */ 101 _13, 102 /** 103 * Emergency Department 104 */ 105 _14, 106 /** 107 * Employment 108 */ 109 _15, 110 /** 111 * Financial & Material aid 112 */ 113 _16, 114 /** 115 * General Practice/GP (doctor) 116 */ 117 _17, 118 /** 119 * Hospital 120 */ 121 _35, 122 /** 123 * Housing/Homelessness 124 */ 125 _18, 126 /** 127 * Interpreting 128 */ 129 _19, 130 /** 131 * Justice 132 */ 133 _20, 134 /** 135 * Legal 136 */ 137 _21, 138 /** 139 * Mental Health 140 */ 141 _22, 142 /** 143 * NDIA 144 */ 145 _38, 146 /** 147 * Physical Activity & Recreation 148 */ 149 _23, 150 /** 151 * Regulation 152 */ 153 _24, 154 /** 155 * Respite/Carer Support 156 */ 157 _25, 158 /** 159 * Specialist Clinical Pathology - requires referral 160 */ 161 _26, 162 /** 163 * Specialist Medical - requires referral 164 */ 165 _27, 166 /** 167 * Specialist Obstetrics & Gynaecology - requires referral 168 */ 169 _28, 170 /** 171 * Specialist Paediatric - requires referral 172 */ 173 _29, 174 /** 175 * Specialist Radiology/Imaging - requires referral 176 */ 177 _30, 178 /** 179 * Specialist Surgical - requires referral 180 */ 181 _31, 182 /** 183 * Support group/s 184 */ 185 _32, 186 /** 187 * Test Message (HSD admin use only) 188 */ 189 _37, 190 /** 191 * Transport 192 */ 193 _33, 194 /** 195 * added to help the parsers 196 */ 197 NULL; 198 public static ServiceCategory fromCode(String codeString) throws FHIRException { 199 if (codeString == null || "".equals(codeString)) 200 return null; 201 if ("1".equals(codeString)) 202 return _1; 203 if ("2".equals(codeString)) 204 return _2; 205 if ("34".equals(codeString)) 206 return _34; 207 if ("3".equals(codeString)) 208 return _3; 209 if ("4".equals(codeString)) 210 return _4; 211 if ("5".equals(codeString)) 212 return _5; 213 if ("6".equals(codeString)) 214 return _6; 215 if ("7".equals(codeString)) 216 return _7; 217 if ("8".equals(codeString)) 218 return _8; 219 if ("36".equals(codeString)) 220 return _36; 221 if ("9".equals(codeString)) 222 return _9; 223 if ("10".equals(codeString)) 224 return _10; 225 if ("11".equals(codeString)) 226 return _11; 227 if ("12".equals(codeString)) 228 return _12; 229 if ("13".equals(codeString)) 230 return _13; 231 if ("14".equals(codeString)) 232 return _14; 233 if ("15".equals(codeString)) 234 return _15; 235 if ("16".equals(codeString)) 236 return _16; 237 if ("17".equals(codeString)) 238 return _17; 239 if ("35".equals(codeString)) 240 return _35; 241 if ("18".equals(codeString)) 242 return _18; 243 if ("19".equals(codeString)) 244 return _19; 245 if ("20".equals(codeString)) 246 return _20; 247 if ("21".equals(codeString)) 248 return _21; 249 if ("22".equals(codeString)) 250 return _22; 251 if ("38".equals(codeString)) 252 return _38; 253 if ("23".equals(codeString)) 254 return _23; 255 if ("24".equals(codeString)) 256 return _24; 257 if ("25".equals(codeString)) 258 return _25; 259 if ("26".equals(codeString)) 260 return _26; 261 if ("27".equals(codeString)) 262 return _27; 263 if ("28".equals(codeString)) 264 return _28; 265 if ("29".equals(codeString)) 266 return _29; 267 if ("30".equals(codeString)) 268 return _30; 269 if ("31".equals(codeString)) 270 return _31; 271 if ("32".equals(codeString)) 272 return _32; 273 if ("37".equals(codeString)) 274 return _37; 275 if ("33".equals(codeString)) 276 return _33; 277 throw new FHIRException("Unknown ServiceCategory code '"+codeString+"'"); 278 } 279 public String toCode() { 280 switch (this) { 281 case _1: return "1"; 282 case _2: return "2"; 283 case _34: return "34"; 284 case _3: return "3"; 285 case _4: return "4"; 286 case _5: return "5"; 287 case _6: return "6"; 288 case _7: return "7"; 289 case _8: return "8"; 290 case _36: return "36"; 291 case _9: return "9"; 292 case _10: return "10"; 293 case _11: return "11"; 294 case _12: return "12"; 295 case _13: return "13"; 296 case _14: return "14"; 297 case _15: return "15"; 298 case _16: return "16"; 299 case _17: return "17"; 300 case _35: return "35"; 301 case _18: return "18"; 302 case _19: return "19"; 303 case _20: return "20"; 304 case _21: return "21"; 305 case _22: return "22"; 306 case _38: return "38"; 307 case _23: return "23"; 308 case _24: return "24"; 309 case _25: return "25"; 310 case _26: return "26"; 311 case _27: return "27"; 312 case _28: return "28"; 313 case _29: return "29"; 314 case _30: return "30"; 315 case _31: return "31"; 316 case _32: return "32"; 317 case _37: return "37"; 318 case _33: return "33"; 319 case NULL: return null; 320 default: return "?"; 321 } 322 } 323 public String getSystem() { 324 return "http://hl7.org/fhir/service-category"; 325 } 326 public String getDefinition() { 327 switch (this) { 328 case _1: return "Adoption"; 329 case _2: return "Aged Care"; 330 case _34: return "Allied Health"; 331 case _3: return "Alternative & Complementary Therapies"; 332 case _4: return "Child Care and/or Kindergarten"; 333 case _5: return "Child Development"; 334 case _6: return "Child Protection & Family Services"; 335 case _7: return "Community Health Care"; 336 case _8: return "Counselling"; 337 case _36: return "Crisis Line (GPAH use only)"; 338 case _9: return "Death Services"; 339 case _10: return "Dental"; 340 case _11: return "Disability Support"; 341 case _12: return "Drug/Alcohol"; 342 case _13: return "Education & Learning"; 343 case _14: return "Emergency Department"; 344 case _15: return "Employment"; 345 case _16: return "Financial & Material aid"; 346 case _17: return "General Practice/GP (doctor)"; 347 case _35: return "Hospital"; 348 case _18: return "Housing/Homelessness"; 349 case _19: return "Interpreting"; 350 case _20: return "Justice"; 351 case _21: return "Legal"; 352 case _22: return "Mental Health"; 353 case _38: return "NDIA"; 354 case _23: return "Physical Activity & Recreation"; 355 case _24: return "Regulation"; 356 case _25: return "Respite/Carer Support"; 357 case _26: return "Specialist Clinical Pathology - requires referral"; 358 case _27: return "Specialist Medical - requires referral"; 359 case _28: return "Specialist Obstetrics & Gynaecology - requires referral"; 360 case _29: return "Specialist Paediatric - requires referral"; 361 case _30: return "Specialist Radiology/Imaging - requires referral"; 362 case _31: return "Specialist Surgical - requires referral"; 363 case _32: return "Support group/s"; 364 case _37: return "Test Message (HSD admin use only)"; 365 case _33: return "Transport"; 366 case NULL: return null; 367 default: return "?"; 368 } 369 } 370 public String getDisplay() { 371 switch (this) { 372 case _1: return "Adoption"; 373 case _2: return "Aged Care"; 374 case _34: return "Allied Health"; 375 case _3: return "Alternative/Complementary Therapies"; 376 case _4: return "Child Care /Kindergarten"; 377 case _5: return "Child Development"; 378 case _6: return "Child Protection & Family Services"; 379 case _7: return "Community Health Care"; 380 case _8: return "Counselling"; 381 case _36: return "Crisis Line (GPAH use only)"; 382 case _9: return "Death Services"; 383 case _10: return "Dental"; 384 case _11: return "Disability Support"; 385 case _12: return "Drug/Alcohol"; 386 case _13: return "Education & Learning"; 387 case _14: return "Emergency Department"; 388 case _15: return "Employment"; 389 case _16: return "Financial & Material Aid"; 390 case _17: return "General Practice"; 391 case _35: return "Hospital"; 392 case _18: return "Housing/Homelessness"; 393 case _19: return "Interpreting"; 394 case _20: return "Justice"; 395 case _21: return "Legal"; 396 case _22: return "Mental Health"; 397 case _38: return "NDIA"; 398 case _23: return "Physical Activity & Recreation"; 399 case _24: return "Regulation"; 400 case _25: return "Respite/Carer Support"; 401 case _26: return "Specialist Clinical Pathology"; 402 case _27: return "Specialist Medical"; 403 case _28: return "Specialist Obstetrics & Gynaecology"; 404 case _29: return "Specialist Paediatric"; 405 case _30: return "Specialist Radiology/Imaging"; 406 case _31: return "Specialist Surgical"; 407 case _32: return "Support Group/s"; 408 case _37: return "Test Message (HSD admin)"; 409 case _33: return "Transport"; 410 case NULL: return null; 411 default: return "?"; 412 } 413 } 414 415 416}