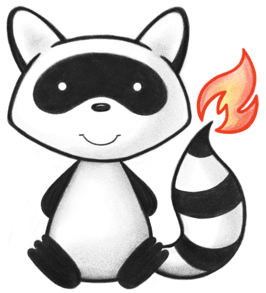
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ServicePlace { 041 042 /** 043 * A facility or location where drugs and other medically related items and services are sold, dispensed, or otherwise provided directly to patients. 044 */ 045 _01, 046 /** 047 * A facility whose primary purpose is education. 048 */ 049 _03, 050 /** 051 * A facility or location whose primary purpose is to provide temporary housing to homeless individuals (e.g., emergency shelters, individual or family shelters). 052 */ 053 _04, 054 /** 055 * A facility or location, owned and operated by the Indian Health Service, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to American Indians and Alaska Natives who do not require hospitalization. 056 */ 057 _05, 058 /** 059 * A facility or location, owned and operated by the Indian Health Service, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services rendered by, or under the supervision of, physicians to American Indians and Alaska Natives admitted as inpatients or outpatients. 060 */ 061 _06, 062 /** 063 * A facility or location owned and operated by a federally recognized American Indian or Alaska Native tribe or tribal organization under a 638 agreement, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to tribal members who do not require hospitalization. 064 */ 065 _07, 066 /** 067 * A facility or location owned and operated by a federally recognized American Indian or Alaska Native tribe or tribal organization under a 638 agreement, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to tribal members admitted as inpatients or outpatients. 068 */ 069 _08, 070 /** 071 * A prison, jail, reformatory, work farm, detention center, or any other similar facility maintained by either Federal, State or local authorities for the purpose of confinement or rehabilitation of adult or juvenile criminal offenders. 072 */ 073 _09, 074 /** 075 * Location, other than a hospital, skilled nursing facility (SNF), military treatment facility, community health center, State or local public health clinic, or intermediate care facility (ICF), where the health professional routinely provides health examinations, diagnosis, and treatment of illness or injury on an ambulatory basis. 076 */ 077 _11, 078 /** 079 * Location, other than a hospital or other facility, where the patient receives care in a private residence. 080 */ 081 _12, 082 /** 083 * Congregate residential facility with self-contained living units providing assessment of each resident's needs and on-site support 24 hours a day, 7 days a week, with the capacity to deliver or arrange for services including some health care and other services. 084 */ 085 _13, 086 /** 087 * A residence, with shared living areas, where clients receive supervision and other services such as social and/or behavioral services, custodial service, and minimal services (e.g., medication administration). 088 */ 089 _14, 090 /** 091 * A facility/unit that moves from place-to-place equipped to provide preventive, screening, diagnostic, and/or treatment services. 092 */ 093 _15, 094 /** 095 * portion of an off-campus hospital provider based department which provides diagnostic, therapeutic (both surgical and nonsurgical), and rehabilitation services to sick or injured persons who do not require hospitalization or institutionalization. 096 */ 097 _19, 098 /** 099 * Location, distinct from a hospital emergency room, an office, or a clinic, whose purpose is to diagnose and treat illness or injury for unscheduled, ambulatory patients seeking immediate medical attention. 100 */ 101 _20, 102 /** 103 * A facility, other than psychiatric, which primarily provides diagnostic, therapeutic (both surgical and nonsurgical), and rehabilitation services by, or under, the supervision of physicians to patients admitted for a variety of medical conditions. 104 */ 105 _21, 106 /** 107 * A land vehicle specifically designed, equipped and staffed for lifesaving and transporting the sick or injured. 108 */ 109 _41, 110 /** 111 * added to help the parsers 112 */ 113 NULL; 114 public static ServicePlace fromCode(String codeString) throws FHIRException { 115 if (codeString == null || "".equals(codeString)) 116 return null; 117 if ("01".equals(codeString)) 118 return _01; 119 if ("03".equals(codeString)) 120 return _03; 121 if ("04".equals(codeString)) 122 return _04; 123 if ("05".equals(codeString)) 124 return _05; 125 if ("06".equals(codeString)) 126 return _06; 127 if ("07".equals(codeString)) 128 return _07; 129 if ("08".equals(codeString)) 130 return _08; 131 if ("09".equals(codeString)) 132 return _09; 133 if ("11".equals(codeString)) 134 return _11; 135 if ("12".equals(codeString)) 136 return _12; 137 if ("13".equals(codeString)) 138 return _13; 139 if ("14".equals(codeString)) 140 return _14; 141 if ("15".equals(codeString)) 142 return _15; 143 if ("19".equals(codeString)) 144 return _19; 145 if ("20".equals(codeString)) 146 return _20; 147 if ("21".equals(codeString)) 148 return _21; 149 if ("41".equals(codeString)) 150 return _41; 151 throw new FHIRException("Unknown ServicePlace code '"+codeString+"'"); 152 } 153 public String toCode() { 154 switch (this) { 155 case _01: return "01"; 156 case _03: return "03"; 157 case _04: return "04"; 158 case _05: return "05"; 159 case _06: return "06"; 160 case _07: return "07"; 161 case _08: return "08"; 162 case _09: return "09"; 163 case _11: return "11"; 164 case _12: return "12"; 165 case _13: return "13"; 166 case _14: return "14"; 167 case _15: return "15"; 168 case _19: return "19"; 169 case _20: return "20"; 170 case _21: return "21"; 171 case _41: return "41"; 172 case NULL: return null; 173 default: return "?"; 174 } 175 } 176 public String getSystem() { 177 return "http://hl7.org/fhir/ex-serviceplace"; 178 } 179 public String getDefinition() { 180 switch (this) { 181 case _01: return "A facility or location where drugs and other medically related items and services are sold, dispensed, or otherwise provided directly to patients."; 182 case _03: return "A facility whose primary purpose is education."; 183 case _04: return "A facility or location whose primary purpose is to provide temporary housing to homeless individuals (e.g., emergency shelters, individual or family shelters)."; 184 case _05: return "A facility or location, owned and operated by the Indian Health Service, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to American Indians and Alaska Natives who do not require hospitalization."; 185 case _06: return "A facility or location, owned and operated by the Indian Health Service, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services rendered by, or under the supervision of, physicians to American Indians and Alaska Natives admitted as inpatients or outpatients."; 186 case _07: return "A facility or location owned and operated by a federally recognized American Indian or Alaska Native tribe or tribal organization under a 638 agreement, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to tribal members who do not require hospitalization."; 187 case _08: return "A facility or location owned and operated by a federally recognized American Indian or Alaska Native tribe or tribal organization under a 638 agreement, which provides diagnostic, therapeutic (surgical and nonsurgical), and rehabilitation services to tribal members admitted as inpatients or outpatients."; 188 case _09: return "A prison, jail, reformatory, work farm, detention center, or any other similar facility maintained by either Federal, State or local authorities for the purpose of confinement or rehabilitation of adult or juvenile criminal offenders."; 189 case _11: return "Location, other than a hospital, skilled nursing facility (SNF), military treatment facility, community health center, State or local public health clinic, or intermediate care facility (ICF), where the health professional routinely provides health examinations, diagnosis, and treatment of illness or injury on an ambulatory basis."; 190 case _12: return "Location, other than a hospital or other facility, where the patient receives care in a private residence."; 191 case _13: return "Congregate residential facility with self-contained living units providing assessment of each resident's needs and on-site support 24 hours a day, 7 days a week, with the capacity to deliver or arrange for services including some health care and other services."; 192 case _14: return "A residence, with shared living areas, where clients receive supervision and other services such as social and/or behavioral services, custodial service, and minimal services (e.g., medication administration)."; 193 case _15: return "A facility/unit that moves from place-to-place equipped to provide preventive, screening, diagnostic, and/or treatment services."; 194 case _19: return "portion of an off-campus hospital provider based department which provides diagnostic, therapeutic (both surgical and nonsurgical), and rehabilitation services to sick or injured persons who do not require hospitalization or institutionalization."; 195 case _20: return "Location, distinct from a hospital emergency room, an office, or a clinic, whose purpose is to diagnose and treat illness or injury for unscheduled, ambulatory patients seeking immediate medical attention."; 196 case _21: return "A facility, other than psychiatric, which primarily provides diagnostic, therapeutic (both surgical and nonsurgical), and rehabilitation services by, or under, the supervision of physicians to patients admitted for a variety of medical conditions."; 197 case _41: return "A land vehicle specifically designed, equipped and staffed for lifesaving and transporting the sick or injured."; 198 case NULL: return null; 199 default: return "?"; 200 } 201 } 202 public String getDisplay() { 203 switch (this) { 204 case _01: return "Pharmacy"; 205 case _03: return "School"; 206 case _04: return "Homeless Shelter"; 207 case _05: return "Indian Health Service Free-standing Facility"; 208 case _06: return "Indian Health Service Provider-based Facility"; 209 case _07: return "Tribal 638 Free-Standing Facility"; 210 case _08: return "Tribal 638 Provider-Based Facility"; 211 case _09: return "Prison/Correctional Facility"; 212 case _11: return "Office"; 213 case _12: return "Home"; 214 case _13: return "Assisted Living Fa"; 215 case _14: return "Group Home"; 216 case _15: return "Mobile Unit"; 217 case _19: return "Off Campus-Outpatient Hospital"; 218 case _20: return "Urgent Care Facility"; 219 case _21: return "Inpatient Hospital"; 220 case _41: return "Ambulance?Land"; 221 case NULL: return null; 222 default: return "?"; 223 } 224 } 225 226 227}