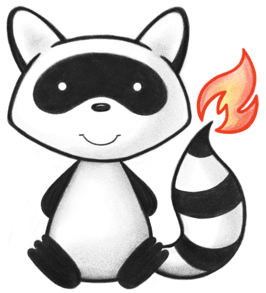
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.dstu3.model.EnumFactory; 039 040public class ServiceTypeEnumFactory implements EnumFactory<ServiceType> { 041 042 public ServiceType fromCode(String codeString) throws IllegalArgumentException { 043 if (codeString == null || "".equals(codeString)) 044 return null; 045 if ("1".equals(codeString)) 046 return ServiceType._1; 047 if ("2".equals(codeString)) 048 return ServiceType._2; 049 if ("3".equals(codeString)) 050 return ServiceType._3; 051 if ("4".equals(codeString)) 052 return ServiceType._4; 053 if ("5".equals(codeString)) 054 return ServiceType._5; 055 if ("6".equals(codeString)) 056 return ServiceType._6; 057 if ("7".equals(codeString)) 058 return ServiceType._7; 059 if ("8".equals(codeString)) 060 return ServiceType._8; 061 if ("9".equals(codeString)) 062 return ServiceType._9; 063 if ("10".equals(codeString)) 064 return ServiceType._10; 065 if ("11".equals(codeString)) 066 return ServiceType._11; 067 if ("12".equals(codeString)) 068 return ServiceType._12; 069 if ("13".equals(codeString)) 070 return ServiceType._13; 071 if ("14".equals(codeString)) 072 return ServiceType._14; 073 if ("15".equals(codeString)) 074 return ServiceType._15; 075 if ("16".equals(codeString)) 076 return ServiceType._16; 077 if ("17".equals(codeString)) 078 return ServiceType._17; 079 if ("18".equals(codeString)) 080 return ServiceType._18; 081 if ("19".equals(codeString)) 082 return ServiceType._19; 083 if ("20".equals(codeString)) 084 return ServiceType._20; 085 if ("21".equals(codeString)) 086 return ServiceType._21; 087 if ("22".equals(codeString)) 088 return ServiceType._22; 089 if ("23".equals(codeString)) 090 return ServiceType._23; 091 if ("24".equals(codeString)) 092 return ServiceType._24; 093 if ("25".equals(codeString)) 094 return ServiceType._25; 095 if ("26".equals(codeString)) 096 return ServiceType._26; 097 if ("27".equals(codeString)) 098 return ServiceType._27; 099 if ("28".equals(codeString)) 100 return ServiceType._28; 101 if ("29".equals(codeString)) 102 return ServiceType._29; 103 if ("30".equals(codeString)) 104 return ServiceType._30; 105 if ("31".equals(codeString)) 106 return ServiceType._31; 107 if ("32".equals(codeString)) 108 return ServiceType._32; 109 if ("33".equals(codeString)) 110 return ServiceType._33; 111 if ("34".equals(codeString)) 112 return ServiceType._34; 113 if ("35".equals(codeString)) 114 return ServiceType._35; 115 if ("36".equals(codeString)) 116 return ServiceType._36; 117 if ("37".equals(codeString)) 118 return ServiceType._37; 119 if ("38".equals(codeString)) 120 return ServiceType._38; 121 if ("39".equals(codeString)) 122 return ServiceType._39; 123 if ("40".equals(codeString)) 124 return ServiceType._40; 125 if ("41".equals(codeString)) 126 return ServiceType._41; 127 if ("42".equals(codeString)) 128 return ServiceType._42; 129 if ("43".equals(codeString)) 130 return ServiceType._43; 131 if ("44".equals(codeString)) 132 return ServiceType._44; 133 if ("45".equals(codeString)) 134 return ServiceType._45; 135 if ("46".equals(codeString)) 136 return ServiceType._46; 137 if ("47".equals(codeString)) 138 return ServiceType._47; 139 if ("48".equals(codeString)) 140 return ServiceType._48; 141 if ("49".equals(codeString)) 142 return ServiceType._49; 143 if ("50".equals(codeString)) 144 return ServiceType._50; 145 if ("51".equals(codeString)) 146 return ServiceType._51; 147 if ("52".equals(codeString)) 148 return ServiceType._52; 149 if ("53".equals(codeString)) 150 return ServiceType._53; 151 if ("54".equals(codeString)) 152 return ServiceType._54; 153 if ("55".equals(codeString)) 154 return ServiceType._55; 155 if ("56".equals(codeString)) 156 return ServiceType._56; 157 if ("57".equals(codeString)) 158 return ServiceType._57; 159 if ("58".equals(codeString)) 160 return ServiceType._58; 161 if ("59".equals(codeString)) 162 return ServiceType._59; 163 if ("60".equals(codeString)) 164 return ServiceType._60; 165 if ("61".equals(codeString)) 166 return ServiceType._61; 167 if ("62".equals(codeString)) 168 return ServiceType._62; 169 if ("63".equals(codeString)) 170 return ServiceType._63; 171 if ("64".equals(codeString)) 172 return ServiceType._64; 173 if ("65".equals(codeString)) 174 return ServiceType._65; 175 if ("66".equals(codeString)) 176 return ServiceType._66; 177 if ("67".equals(codeString)) 178 return ServiceType._67; 179 if ("68".equals(codeString)) 180 return ServiceType._68; 181 if ("69".equals(codeString)) 182 return ServiceType._69; 183 if ("70".equals(codeString)) 184 return ServiceType._70; 185 if ("71".equals(codeString)) 186 return ServiceType._71; 187 if ("72".equals(codeString)) 188 return ServiceType._72; 189 if ("73".equals(codeString)) 190 return ServiceType._73; 191 if ("74".equals(codeString)) 192 return ServiceType._74; 193 if ("75".equals(codeString)) 194 return ServiceType._75; 195 if ("76".equals(codeString)) 196 return ServiceType._76; 197 if ("77".equals(codeString)) 198 return ServiceType._77; 199 if ("78".equals(codeString)) 200 return ServiceType._78; 201 if ("79".equals(codeString)) 202 return ServiceType._79; 203 if ("80".equals(codeString)) 204 return ServiceType._80; 205 if ("81".equals(codeString)) 206 return ServiceType._81; 207 if ("82".equals(codeString)) 208 return ServiceType._82; 209 if ("83".equals(codeString)) 210 return ServiceType._83; 211 if ("84".equals(codeString)) 212 return ServiceType._84; 213 if ("85".equals(codeString)) 214 return ServiceType._85; 215 if ("86".equals(codeString)) 216 return ServiceType._86; 217 if ("87".equals(codeString)) 218 return ServiceType._87; 219 if ("88".equals(codeString)) 220 return ServiceType._88; 221 if ("89".equals(codeString)) 222 return ServiceType._89; 223 if ("90".equals(codeString)) 224 return ServiceType._90; 225 if ("91".equals(codeString)) 226 return ServiceType._91; 227 if ("92".equals(codeString)) 228 return ServiceType._92; 229 if ("93".equals(codeString)) 230 return ServiceType._93; 231 if ("94".equals(codeString)) 232 return ServiceType._94; 233 if ("95".equals(codeString)) 234 return ServiceType._95; 235 if ("96".equals(codeString)) 236 return ServiceType._96; 237 if ("97".equals(codeString)) 238 return ServiceType._97; 239 if ("98".equals(codeString)) 240 return ServiceType._98; 241 if ("99".equals(codeString)) 242 return ServiceType._99; 243 if ("100".equals(codeString)) 244 return ServiceType._100; 245 if ("101".equals(codeString)) 246 return ServiceType._101; 247 if ("102".equals(codeString)) 248 return ServiceType._102; 249 if ("103".equals(codeString)) 250 return ServiceType._103; 251 if ("104".equals(codeString)) 252 return ServiceType._104; 253 if ("105".equals(codeString)) 254 return ServiceType._105; 255 if ("106".equals(codeString)) 256 return ServiceType._106; 257 if ("107".equals(codeString)) 258 return ServiceType._107; 259 if ("108".equals(codeString)) 260 return ServiceType._108; 261 if ("109".equals(codeString)) 262 return ServiceType._109; 263 if ("110".equals(codeString)) 264 return ServiceType._110; 265 if ("111".equals(codeString)) 266 return ServiceType._111; 267 if ("112".equals(codeString)) 268 return ServiceType._112; 269 if ("113".equals(codeString)) 270 return ServiceType._113; 271 if ("114".equals(codeString)) 272 return ServiceType._114; 273 if ("115".equals(codeString)) 274 return ServiceType._115; 275 if ("116".equals(codeString)) 276 return ServiceType._116; 277 if ("117".equals(codeString)) 278 return ServiceType._117; 279 if ("118".equals(codeString)) 280 return ServiceType._118; 281 if ("119".equals(codeString)) 282 return ServiceType._119; 283 if ("120".equals(codeString)) 284 return ServiceType._120; 285 if ("121".equals(codeString)) 286 return ServiceType._121; 287 if ("122".equals(codeString)) 288 return ServiceType._122; 289 if ("123".equals(codeString)) 290 return ServiceType._123; 291 if ("124".equals(codeString)) 292 return ServiceType._124; 293 if ("125".equals(codeString)) 294 return ServiceType._125; 295 if ("126".equals(codeString)) 296 return ServiceType._126; 297 if ("127".equals(codeString)) 298 return ServiceType._127; 299 if ("128".equals(codeString)) 300 return ServiceType._128; 301 if ("129".equals(codeString)) 302 return ServiceType._129; 303 if ("130".equals(codeString)) 304 return ServiceType._130; 305 if ("131".equals(codeString)) 306 return ServiceType._131; 307 if ("132".equals(codeString)) 308 return ServiceType._132; 309 if ("133".equals(codeString)) 310 return ServiceType._133; 311 if ("134".equals(codeString)) 312 return ServiceType._134; 313 if ("135".equals(codeString)) 314 return ServiceType._135; 315 if ("136".equals(codeString)) 316 return ServiceType._136; 317 if ("137".equals(codeString)) 318 return ServiceType._137; 319 if ("138".equals(codeString)) 320 return ServiceType._138; 321 if ("139".equals(codeString)) 322 return ServiceType._139; 323 if ("140".equals(codeString)) 324 return ServiceType._140; 325 if ("141".equals(codeString)) 326 return ServiceType._141; 327 if ("142".equals(codeString)) 328 return ServiceType._142; 329 if ("143".equals(codeString)) 330 return ServiceType._143; 331 if ("144".equals(codeString)) 332 return ServiceType._144; 333 if ("145".equals(codeString)) 334 return ServiceType._145; 335 if ("146".equals(codeString)) 336 return ServiceType._146; 337 if ("147".equals(codeString)) 338 return ServiceType._147; 339 if ("148".equals(codeString)) 340 return ServiceType._148; 341 if ("149".equals(codeString)) 342 return ServiceType._149; 343 if ("150".equals(codeString)) 344 return ServiceType._150; 345 if ("151".equals(codeString)) 346 return ServiceType._151; 347 if ("152".equals(codeString)) 348 return ServiceType._152; 349 if ("153".equals(codeString)) 350 return ServiceType._153; 351 if ("154".equals(codeString)) 352 return ServiceType._154; 353 if ("155".equals(codeString)) 354 return ServiceType._155; 355 if ("156".equals(codeString)) 356 return ServiceType._156; 357 if ("157".equals(codeString)) 358 return ServiceType._157; 359 if ("158".equals(codeString)) 360 return ServiceType._158; 361 if ("159".equals(codeString)) 362 return ServiceType._159; 363 if ("160".equals(codeString)) 364 return ServiceType._160; 365 if ("161".equals(codeString)) 366 return ServiceType._161; 367 if ("162".equals(codeString)) 368 return ServiceType._162; 369 if ("163".equals(codeString)) 370 return ServiceType._163; 371 if ("164".equals(codeString)) 372 return ServiceType._164; 373 if ("165".equals(codeString)) 374 return ServiceType._165; 375 if ("166".equals(codeString)) 376 return ServiceType._166; 377 if ("167".equals(codeString)) 378 return ServiceType._167; 379 if ("168".equals(codeString)) 380 return ServiceType._168; 381 if ("169".equals(codeString)) 382 return ServiceType._169; 383 if ("170".equals(codeString)) 384 return ServiceType._170; 385 if ("171".equals(codeString)) 386 return ServiceType._171; 387 if ("172".equals(codeString)) 388 return ServiceType._172; 389 if ("173".equals(codeString)) 390 return ServiceType._173; 391 if ("174".equals(codeString)) 392 return ServiceType._174; 393 if ("175".equals(codeString)) 394 return ServiceType._175; 395 if ("176".equals(codeString)) 396 return ServiceType._176; 397 if ("177".equals(codeString)) 398 return ServiceType._177; 399 if ("178".equals(codeString)) 400 return ServiceType._178; 401 if ("179".equals(codeString)) 402 return ServiceType._179; 403 if ("180".equals(codeString)) 404 return ServiceType._180; 405 if ("181".equals(codeString)) 406 return ServiceType._181; 407 if ("182".equals(codeString)) 408 return ServiceType._182; 409 if ("183".equals(codeString)) 410 return ServiceType._183; 411 if ("184".equals(codeString)) 412 return ServiceType._184; 413 if ("185".equals(codeString)) 414 return ServiceType._185; 415 if ("186".equals(codeString)) 416 return ServiceType._186; 417 if ("187".equals(codeString)) 418 return ServiceType._187; 419 if ("188".equals(codeString)) 420 return ServiceType._188; 421 if ("189".equals(codeString)) 422 return ServiceType._189; 423 if ("190".equals(codeString)) 424 return ServiceType._190; 425 if ("191".equals(codeString)) 426 return ServiceType._191; 427 if ("192".equals(codeString)) 428 return ServiceType._192; 429 if ("193".equals(codeString)) 430 return ServiceType._193; 431 if ("194".equals(codeString)) 432 return ServiceType._194; 433 if ("195".equals(codeString)) 434 return ServiceType._195; 435 if ("196".equals(codeString)) 436 return ServiceType._196; 437 if ("197".equals(codeString)) 438 return ServiceType._197; 439 if ("198".equals(codeString)) 440 return ServiceType._198; 441 if ("199".equals(codeString)) 442 return ServiceType._199; 443 if ("200".equals(codeString)) 444 return ServiceType._200; 445 if ("201".equals(codeString)) 446 return ServiceType._201; 447 if ("202".equals(codeString)) 448 return ServiceType._202; 449 if ("203".equals(codeString)) 450 return ServiceType._203; 451 if ("204".equals(codeString)) 452 return ServiceType._204; 453 if ("205".equals(codeString)) 454 return ServiceType._205; 455 if ("206".equals(codeString)) 456 return ServiceType._206; 457 if ("207".equals(codeString)) 458 return ServiceType._207; 459 if ("208".equals(codeString)) 460 return ServiceType._208; 461 if ("209".equals(codeString)) 462 return ServiceType._209; 463 if ("210".equals(codeString)) 464 return ServiceType._210; 465 if ("211".equals(codeString)) 466 return ServiceType._211; 467 if ("212".equals(codeString)) 468 return ServiceType._212; 469 if ("213".equals(codeString)) 470 return ServiceType._213; 471 if ("214".equals(codeString)) 472 return ServiceType._214; 473 if ("215".equals(codeString)) 474 return ServiceType._215; 475 if ("216".equals(codeString)) 476 return ServiceType._216; 477 if ("217".equals(codeString)) 478 return ServiceType._217; 479 if ("218".equals(codeString)) 480 return ServiceType._218; 481 if ("219".equals(codeString)) 482 return ServiceType._219; 483 if ("220".equals(codeString)) 484 return ServiceType._220; 485 if ("221".equals(codeString)) 486 return ServiceType._221; 487 if ("222".equals(codeString)) 488 return ServiceType._222; 489 if ("223".equals(codeString)) 490 return ServiceType._223; 491 if ("224".equals(codeString)) 492 return ServiceType._224; 493 if ("225".equals(codeString)) 494 return ServiceType._225; 495 if ("226".equals(codeString)) 496 return ServiceType._226; 497 if ("227".equals(codeString)) 498 return ServiceType._227; 499 if ("228".equals(codeString)) 500 return ServiceType._228; 501 if ("229".equals(codeString)) 502 return ServiceType._229; 503 if ("230".equals(codeString)) 504 return ServiceType._230; 505 if ("231".equals(codeString)) 506 return ServiceType._231; 507 if ("232".equals(codeString)) 508 return ServiceType._232; 509 if ("233".equals(codeString)) 510 return ServiceType._233; 511 if ("234".equals(codeString)) 512 return ServiceType._234; 513 if ("235".equals(codeString)) 514 return ServiceType._235; 515 if ("236".equals(codeString)) 516 return ServiceType._236; 517 if ("237".equals(codeString)) 518 return ServiceType._237; 519 if ("238".equals(codeString)) 520 return ServiceType._238; 521 if ("239".equals(codeString)) 522 return ServiceType._239; 523 if ("240".equals(codeString)) 524 return ServiceType._240; 525 if ("241".equals(codeString)) 526 return ServiceType._241; 527 if ("242".equals(codeString)) 528 return ServiceType._242; 529 if ("243".equals(codeString)) 530 return ServiceType._243; 531 if ("244".equals(codeString)) 532 return ServiceType._244; 533 if ("245".equals(codeString)) 534 return ServiceType._245; 535 if ("246".equals(codeString)) 536 return ServiceType._246; 537 if ("247".equals(codeString)) 538 return ServiceType._247; 539 if ("248".equals(codeString)) 540 return ServiceType._248; 541 if ("249".equals(codeString)) 542 return ServiceType._249; 543 if ("250".equals(codeString)) 544 return ServiceType._250; 545 if ("251".equals(codeString)) 546 return ServiceType._251; 547 if ("252".equals(codeString)) 548 return ServiceType._252; 549 if ("253".equals(codeString)) 550 return ServiceType._253; 551 if ("254".equals(codeString)) 552 return ServiceType._254; 553 if ("255".equals(codeString)) 554 return ServiceType._255; 555 if ("256".equals(codeString)) 556 return ServiceType._256; 557 if ("257".equals(codeString)) 558 return ServiceType._257; 559 if ("258".equals(codeString)) 560 return ServiceType._258; 561 if ("259".equals(codeString)) 562 return ServiceType._259; 563 if ("260".equals(codeString)) 564 return ServiceType._260; 565 if ("261".equals(codeString)) 566 return ServiceType._261; 567 if ("262".equals(codeString)) 568 return ServiceType._262; 569 if ("263".equals(codeString)) 570 return ServiceType._263; 571 if ("264".equals(codeString)) 572 return ServiceType._264; 573 if ("265".equals(codeString)) 574 return ServiceType._265; 575 if ("266".equals(codeString)) 576 return ServiceType._266; 577 if ("267".equals(codeString)) 578 return ServiceType._267; 579 if ("268".equals(codeString)) 580 return ServiceType._268; 581 if ("269".equals(codeString)) 582 return ServiceType._269; 583 if ("270".equals(codeString)) 584 return ServiceType._270; 585 if ("271".equals(codeString)) 586 return ServiceType._271; 587 if ("272".equals(codeString)) 588 return ServiceType._272; 589 if ("273".equals(codeString)) 590 return ServiceType._273; 591 if ("274".equals(codeString)) 592 return ServiceType._274; 593 if ("275".equals(codeString)) 594 return ServiceType._275; 595 if ("276".equals(codeString)) 596 return ServiceType._276; 597 if ("277".equals(codeString)) 598 return ServiceType._277; 599 if ("278".equals(codeString)) 600 return ServiceType._278; 601 if ("279".equals(codeString)) 602 return ServiceType._279; 603 if ("280".equals(codeString)) 604 return ServiceType._280; 605 if ("281".equals(codeString)) 606 return ServiceType._281; 607 if ("282".equals(codeString)) 608 return ServiceType._282; 609 if ("283".equals(codeString)) 610 return ServiceType._283; 611 if ("284".equals(codeString)) 612 return ServiceType._284; 613 if ("285".equals(codeString)) 614 return ServiceType._285; 615 if ("286".equals(codeString)) 616 return ServiceType._286; 617 if ("287".equals(codeString)) 618 return ServiceType._287; 619 if ("288".equals(codeString)) 620 return ServiceType._288; 621 if ("289".equals(codeString)) 622 return ServiceType._289; 623 if ("290".equals(codeString)) 624 return ServiceType._290; 625 if ("291".equals(codeString)) 626 return ServiceType._291; 627 if ("292".equals(codeString)) 628 return ServiceType._292; 629 if ("293".equals(codeString)) 630 return ServiceType._293; 631 if ("294".equals(codeString)) 632 return ServiceType._294; 633 if ("295".equals(codeString)) 634 return ServiceType._295; 635 if ("296".equals(codeString)) 636 return ServiceType._296; 637 if ("297".equals(codeString)) 638 return ServiceType._297; 639 if ("298".equals(codeString)) 640 return ServiceType._298; 641 if ("299".equals(codeString)) 642 return ServiceType._299; 643 if ("300".equals(codeString)) 644 return ServiceType._300; 645 if ("301".equals(codeString)) 646 return ServiceType._301; 647 if ("302".equals(codeString)) 648 return ServiceType._302; 649 if ("303".equals(codeString)) 650 return ServiceType._303; 651 if ("304".equals(codeString)) 652 return ServiceType._304; 653 if ("305".equals(codeString)) 654 return ServiceType._305; 655 if ("306".equals(codeString)) 656 return ServiceType._306; 657 if ("307".equals(codeString)) 658 return ServiceType._307; 659 if ("308".equals(codeString)) 660 return ServiceType._308; 661 if ("309".equals(codeString)) 662 return ServiceType._309; 663 if ("310".equals(codeString)) 664 return ServiceType._310; 665 if ("311".equals(codeString)) 666 return ServiceType._311; 667 if ("312".equals(codeString)) 668 return ServiceType._312; 669 if ("313".equals(codeString)) 670 return ServiceType._313; 671 if ("314".equals(codeString)) 672 return ServiceType._314; 673 if ("315".equals(codeString)) 674 return ServiceType._315; 675 if ("316".equals(codeString)) 676 return ServiceType._316; 677 if ("317".equals(codeString)) 678 return ServiceType._317; 679 if ("318".equals(codeString)) 680 return ServiceType._318; 681 if ("319".equals(codeString)) 682 return ServiceType._319; 683 if ("320".equals(codeString)) 684 return ServiceType._320; 685 if ("321".equals(codeString)) 686 return ServiceType._321; 687 if ("322".equals(codeString)) 688 return ServiceType._322; 689 if ("323".equals(codeString)) 690 return ServiceType._323; 691 if ("324".equals(codeString)) 692 return ServiceType._324; 693 if ("325".equals(codeString)) 694 return ServiceType._325; 695 if ("326".equals(codeString)) 696 return ServiceType._326; 697 if ("327".equals(codeString)) 698 return ServiceType._327; 699 if ("328".equals(codeString)) 700 return ServiceType._328; 701 if ("330".equals(codeString)) 702 return ServiceType._330; 703 if ("331".equals(codeString)) 704 return ServiceType._331; 705 if ("332".equals(codeString)) 706 return ServiceType._332; 707 if ("333".equals(codeString)) 708 return ServiceType._333; 709 if ("334".equals(codeString)) 710 return ServiceType._334; 711 if ("335".equals(codeString)) 712 return ServiceType._335; 713 if ("336".equals(codeString)) 714 return ServiceType._336; 715 if ("337".equals(codeString)) 716 return ServiceType._337; 717 if ("338".equals(codeString)) 718 return ServiceType._338; 719 if ("339".equals(codeString)) 720 return ServiceType._339; 721 if ("340".equals(codeString)) 722 return ServiceType._340; 723 if ("341".equals(codeString)) 724 return ServiceType._341; 725 if ("342".equals(codeString)) 726 return ServiceType._342; 727 if ("343".equals(codeString)) 728 return ServiceType._343; 729 if ("344".equals(codeString)) 730 return ServiceType._344; 731 if ("345".equals(codeString)) 732 return ServiceType._345; 733 if ("346".equals(codeString)) 734 return ServiceType._346; 735 if ("347".equals(codeString)) 736 return ServiceType._347; 737 if ("348".equals(codeString)) 738 return ServiceType._348; 739 if ("349".equals(codeString)) 740 return ServiceType._349; 741 if ("350".equals(codeString)) 742 return ServiceType._350; 743 if ("351".equals(codeString)) 744 return ServiceType._351; 745 if ("352".equals(codeString)) 746 return ServiceType._352; 747 if ("353".equals(codeString)) 748 return ServiceType._353; 749 if ("354".equals(codeString)) 750 return ServiceType._354; 751 if ("355".equals(codeString)) 752 return ServiceType._355; 753 if ("356".equals(codeString)) 754 return ServiceType._356; 755 if ("357".equals(codeString)) 756 return ServiceType._357; 757 if ("358".equals(codeString)) 758 return ServiceType._358; 759 if ("359".equals(codeString)) 760 return ServiceType._359; 761 if ("360".equals(codeString)) 762 return ServiceType._360; 763 if ("361".equals(codeString)) 764 return ServiceType._361; 765 if ("362".equals(codeString)) 766 return ServiceType._362; 767 if ("364".equals(codeString)) 768 return ServiceType._364; 769 if ("365".equals(codeString)) 770 return ServiceType._365; 771 if ("366".equals(codeString)) 772 return ServiceType._366; 773 if ("367".equals(codeString)) 774 return ServiceType._367; 775 if ("368".equals(codeString)) 776 return ServiceType._368; 777 if ("369".equals(codeString)) 778 return ServiceType._369; 779 if ("370".equals(codeString)) 780 return ServiceType._370; 781 if ("371".equals(codeString)) 782 return ServiceType._371; 783 if ("372".equals(codeString)) 784 return ServiceType._372; 785 if ("373".equals(codeString)) 786 return ServiceType._373; 787 if ("374".equals(codeString)) 788 return ServiceType._374; 789 if ("375".equals(codeString)) 790 return ServiceType._375; 791 if ("376".equals(codeString)) 792 return ServiceType._376; 793 if ("377".equals(codeString)) 794 return ServiceType._377; 795 if ("378".equals(codeString)) 796 return ServiceType._378; 797 if ("379".equals(codeString)) 798 return ServiceType._379; 799 if ("380".equals(codeString)) 800 return ServiceType._380; 801 if ("381".equals(codeString)) 802 return ServiceType._381; 803 if ("382".equals(codeString)) 804 return ServiceType._382; 805 if ("383".equals(codeString)) 806 return ServiceType._383; 807 if ("384".equals(codeString)) 808 return ServiceType._384; 809 if ("385".equals(codeString)) 810 return ServiceType._385; 811 if ("386".equals(codeString)) 812 return ServiceType._386; 813 if ("387".equals(codeString)) 814 return ServiceType._387; 815 if ("388".equals(codeString)) 816 return ServiceType._388; 817 if ("389".equals(codeString)) 818 return ServiceType._389; 819 if ("390".equals(codeString)) 820 return ServiceType._390; 821 if ("391".equals(codeString)) 822 return ServiceType._391; 823 if ("392".equals(codeString)) 824 return ServiceType._392; 825 if ("393".equals(codeString)) 826 return ServiceType._393; 827 if ("394".equals(codeString)) 828 return ServiceType._394; 829 if ("395".equals(codeString)) 830 return ServiceType._395; 831 if ("396".equals(codeString)) 832 return ServiceType._396; 833 if ("397".equals(codeString)) 834 return ServiceType._397; 835 if ("398".equals(codeString)) 836 return ServiceType._398; 837 if ("399".equals(codeString)) 838 return ServiceType._399; 839 if ("400".equals(codeString)) 840 return ServiceType._400; 841 if ("401".equals(codeString)) 842 return ServiceType._401; 843 if ("402".equals(codeString)) 844 return ServiceType._402; 845 if ("403".equals(codeString)) 846 return ServiceType._403; 847 if ("404".equals(codeString)) 848 return ServiceType._404; 849 if ("405".equals(codeString)) 850 return ServiceType._405; 851 if ("406".equals(codeString)) 852 return ServiceType._406; 853 if ("407".equals(codeString)) 854 return ServiceType._407; 855 if ("408".equals(codeString)) 856 return ServiceType._408; 857 if ("409".equals(codeString)) 858 return ServiceType._409; 859 if ("410".equals(codeString)) 860 return ServiceType._410; 861 if ("411".equals(codeString)) 862 return ServiceType._411; 863 if ("412".equals(codeString)) 864 return ServiceType._412; 865 if ("413".equals(codeString)) 866 return ServiceType._413; 867 if ("414".equals(codeString)) 868 return ServiceType._414; 869 if ("415".equals(codeString)) 870 return ServiceType._415; 871 if ("416".equals(codeString)) 872 return ServiceType._416; 873 if ("417".equals(codeString)) 874 return ServiceType._417; 875 if ("418".equals(codeString)) 876 return ServiceType._418; 877 if ("419".equals(codeString)) 878 return ServiceType._419; 879 if ("420".equals(codeString)) 880 return ServiceType._420; 881 if ("421".equals(codeString)) 882 return ServiceType._421; 883 if ("422".equals(codeString)) 884 return ServiceType._422; 885 if ("423".equals(codeString)) 886 return ServiceType._423; 887 if ("424".equals(codeString)) 888 return ServiceType._424; 889 if ("425".equals(codeString)) 890 return ServiceType._425; 891 if ("426".equals(codeString)) 892 return ServiceType._426; 893 if ("427".equals(codeString)) 894 return ServiceType._427; 895 if ("428".equals(codeString)) 896 return ServiceType._428; 897 if ("429".equals(codeString)) 898 return ServiceType._429; 899 if ("430".equals(codeString)) 900 return ServiceType._430; 901 if ("431".equals(codeString)) 902 return ServiceType._431; 903 if ("432".equals(codeString)) 904 return ServiceType._432; 905 if ("433".equals(codeString)) 906 return ServiceType._433; 907 if ("434".equals(codeString)) 908 return ServiceType._434; 909 if ("435".equals(codeString)) 910 return ServiceType._435; 911 if ("436".equals(codeString)) 912 return ServiceType._436; 913 if ("437".equals(codeString)) 914 return ServiceType._437; 915 if ("438".equals(codeString)) 916 return ServiceType._438; 917 if ("439".equals(codeString)) 918 return ServiceType._439; 919 if ("440".equals(codeString)) 920 return ServiceType._440; 921 if ("441".equals(codeString)) 922 return ServiceType._441; 923 if ("442".equals(codeString)) 924 return ServiceType._442; 925 if ("443".equals(codeString)) 926 return ServiceType._443; 927 if ("444".equals(codeString)) 928 return ServiceType._444; 929 if ("445".equals(codeString)) 930 return ServiceType._445; 931 if ("446".equals(codeString)) 932 return ServiceType._446; 933 if ("447".equals(codeString)) 934 return ServiceType._447; 935 if ("448".equals(codeString)) 936 return ServiceType._448; 937 if ("449".equals(codeString)) 938 return ServiceType._449; 939 if ("450".equals(codeString)) 940 return ServiceType._450; 941 if ("451".equals(codeString)) 942 return ServiceType._451; 943 if ("452".equals(codeString)) 944 return ServiceType._452; 945 if ("453".equals(codeString)) 946 return ServiceType._453; 947 if ("454".equals(codeString)) 948 return ServiceType._454; 949 if ("455".equals(codeString)) 950 return ServiceType._455; 951 if ("456".equals(codeString)) 952 return ServiceType._456; 953 if ("457".equals(codeString)) 954 return ServiceType._457; 955 if ("458".equals(codeString)) 956 return ServiceType._458; 957 if ("459".equals(codeString)) 958 return ServiceType._459; 959 if ("460".equals(codeString)) 960 return ServiceType._460; 961 if ("461".equals(codeString)) 962 return ServiceType._461; 963 if ("462".equals(codeString)) 964 return ServiceType._462; 965 if ("463".equals(codeString)) 966 return ServiceType._463; 967 if ("464".equals(codeString)) 968 return ServiceType._464; 969 if ("465".equals(codeString)) 970 return ServiceType._465; 971 if ("466".equals(codeString)) 972 return ServiceType._466; 973 if ("467".equals(codeString)) 974 return ServiceType._467; 975 if ("468".equals(codeString)) 976 return ServiceType._468; 977 if ("469".equals(codeString)) 978 return ServiceType._469; 979 if ("470".equals(codeString)) 980 return ServiceType._470; 981 if ("471".equals(codeString)) 982 return ServiceType._471; 983 if ("472".equals(codeString)) 984 return ServiceType._472; 985 if ("473".equals(codeString)) 986 return ServiceType._473; 987 if ("474".equals(codeString)) 988 return ServiceType._474; 989 if ("475".equals(codeString)) 990 return ServiceType._475; 991 if ("476".equals(codeString)) 992 return ServiceType._476; 993 if ("477".equals(codeString)) 994 return ServiceType._477; 995 if ("478".equals(codeString)) 996 return ServiceType._478; 997 if ("479".equals(codeString)) 998 return ServiceType._479; 999 if ("480".equals(codeString)) 1000 return ServiceType._480; 1001 if ("481".equals(codeString)) 1002 return ServiceType._481; 1003 if ("482".equals(codeString)) 1004 return ServiceType._482; 1005 if ("483".equals(codeString)) 1006 return ServiceType._483; 1007 if ("484".equals(codeString)) 1008 return ServiceType._484; 1009 if ("485".equals(codeString)) 1010 return ServiceType._485; 1011 if ("486".equals(codeString)) 1012 return ServiceType._486; 1013 if ("488".equals(codeString)) 1014 return ServiceType._488; 1015 if ("489".equals(codeString)) 1016 return ServiceType._489; 1017 if ("490".equals(codeString)) 1018 return ServiceType._490; 1019 if ("491".equals(codeString)) 1020 return ServiceType._491; 1021 if ("492".equals(codeString)) 1022 return ServiceType._492; 1023 if ("493".equals(codeString)) 1024 return ServiceType._493; 1025 if ("494".equals(codeString)) 1026 return ServiceType._494; 1027 if ("495".equals(codeString)) 1028 return ServiceType._495; 1029 if ("496".equals(codeString)) 1030 return ServiceType._496; 1031 if ("497".equals(codeString)) 1032 return ServiceType._497; 1033 if ("498".equals(codeString)) 1034 return ServiceType._498; 1035 if ("500".equals(codeString)) 1036 return ServiceType._500; 1037 if ("501".equals(codeString)) 1038 return ServiceType._501; 1039 if ("502".equals(codeString)) 1040 return ServiceType._502; 1041 if ("503".equals(codeString)) 1042 return ServiceType._503; 1043 if ("504".equals(codeString)) 1044 return ServiceType._504; 1045 if ("505".equals(codeString)) 1046 return ServiceType._505; 1047 if ("506".equals(codeString)) 1048 return ServiceType._506; 1049 if ("507".equals(codeString)) 1050 return ServiceType._507; 1051 if ("508".equals(codeString)) 1052 return ServiceType._508; 1053 if ("509".equals(codeString)) 1054 return ServiceType._509; 1055 if ("510".equals(codeString)) 1056 return ServiceType._510; 1057 if ("513".equals(codeString)) 1058 return ServiceType._513; 1059 if ("514".equals(codeString)) 1060 return ServiceType._514; 1061 if ("530".equals(codeString)) 1062 return ServiceType._530; 1063 if ("531".equals(codeString)) 1064 return ServiceType._531; 1065 if ("532".equals(codeString)) 1066 return ServiceType._532; 1067 if ("533".equals(codeString)) 1068 return ServiceType._533; 1069 if ("534".equals(codeString)) 1070 return ServiceType._534; 1071 if ("535".equals(codeString)) 1072 return ServiceType._535; 1073 if ("536".equals(codeString)) 1074 return ServiceType._536; 1075 if ("537".equals(codeString)) 1076 return ServiceType._537; 1077 if ("538".equals(codeString)) 1078 return ServiceType._538; 1079 if ("539".equals(codeString)) 1080 return ServiceType._539; 1081 if ("540".equals(codeString)) 1082 return ServiceType._540; 1083 if ("541".equals(codeString)) 1084 return ServiceType._541; 1085 if ("542".equals(codeString)) 1086 return ServiceType._542; 1087 if ("543".equals(codeString)) 1088 return ServiceType._543; 1089 if ("544".equals(codeString)) 1090 return ServiceType._544; 1091 if ("545".equals(codeString)) 1092 return ServiceType._545; 1093 if ("546".equals(codeString)) 1094 return ServiceType._546; 1095 if ("547".equals(codeString)) 1096 return ServiceType._547; 1097 if ("548".equals(codeString)) 1098 return ServiceType._548; 1099 if ("550".equals(codeString)) 1100 return ServiceType._550; 1101 if ("551".equals(codeString)) 1102 return ServiceType._551; 1103 if ("552".equals(codeString)) 1104 return ServiceType._552; 1105 if ("553".equals(codeString)) 1106 return ServiceType._553; 1107 if ("554".equals(codeString)) 1108 return ServiceType._554; 1109 if ("555".equals(codeString)) 1110 return ServiceType._555; 1111 if ("556".equals(codeString)) 1112 return ServiceType._556; 1113 if ("557".equals(codeString)) 1114 return ServiceType._557; 1115 if ("558".equals(codeString)) 1116 return ServiceType._558; 1117 if ("559".equals(codeString)) 1118 return ServiceType._559; 1119 if ("560".equals(codeString)) 1120 return ServiceType._560; 1121 if ("561".equals(codeString)) 1122 return ServiceType._561; 1123 if ("562".equals(codeString)) 1124 return ServiceType._562; 1125 if ("563".equals(codeString)) 1126 return ServiceType._563; 1127 if ("564".equals(codeString)) 1128 return ServiceType._564; 1129 if ("565".equals(codeString)) 1130 return ServiceType._565; 1131 if ("566".equals(codeString)) 1132 return ServiceType._566; 1133 if ("567".equals(codeString)) 1134 return ServiceType._567; 1135 if ("568".equals(codeString)) 1136 return ServiceType._568; 1137 if ("569".equals(codeString)) 1138 return ServiceType._569; 1139 if ("570".equals(codeString)) 1140 return ServiceType._570; 1141 if ("571".equals(codeString)) 1142 return ServiceType._571; 1143 if ("572".equals(codeString)) 1144 return ServiceType._572; 1145 if ("573".equals(codeString)) 1146 return ServiceType._573; 1147 if ("574".equals(codeString)) 1148 return ServiceType._574; 1149 if ("575".equals(codeString)) 1150 return ServiceType._575; 1151 if ("576".equals(codeString)) 1152 return ServiceType._576; 1153 if ("577".equals(codeString)) 1154 return ServiceType._577; 1155 if ("580".equals(codeString)) 1156 return ServiceType._580; 1157 if ("581".equals(codeString)) 1158 return ServiceType._581; 1159 if ("582".equals(codeString)) 1160 return ServiceType._582; 1161 if ("583".equals(codeString)) 1162 return ServiceType._583; 1163 if ("584".equals(codeString)) 1164 return ServiceType._584; 1165 if ("585".equals(codeString)) 1166 return ServiceType._585; 1167 if ("589".equals(codeString)) 1168 return ServiceType._589; 1169 if ("590".equals(codeString)) 1170 return ServiceType._590; 1171 if ("591".equals(codeString)) 1172 return ServiceType._591; 1173 if ("593".equals(codeString)) 1174 return ServiceType._593; 1175 if ("599".equals(codeString)) 1176 return ServiceType._599; 1177 if ("600".equals(codeString)) 1178 return ServiceType._600; 1179 if ("601".equals(codeString)) 1180 return ServiceType._601; 1181 if ("602".equals(codeString)) 1182 return ServiceType._602; 1183 if ("603".equals(codeString)) 1184 return ServiceType._603; 1185 if ("604".equals(codeString)) 1186 return ServiceType._604; 1187 if ("605".equals(codeString)) 1188 return ServiceType._605; 1189 if ("606".equals(codeString)) 1190 return ServiceType._606; 1191 if ("607".equals(codeString)) 1192 return ServiceType._607; 1193 if ("608".equals(codeString)) 1194 return ServiceType._608; 1195 if ("609".equals(codeString)) 1196 return ServiceType._609; 1197 if ("610".equals(codeString)) 1198 return ServiceType._610; 1199 if ("611".equals(codeString)) 1200 return ServiceType._611; 1201 if ("612".equals(codeString)) 1202 return ServiceType._612; 1203 if ("613".equals(codeString)) 1204 return ServiceType._613; 1205 if ("614".equals(codeString)) 1206 return ServiceType._614; 1207 if ("615".equals(codeString)) 1208 return ServiceType._615; 1209 if ("616".equals(codeString)) 1210 return ServiceType._616; 1211 if ("617".equals(codeString)) 1212 return ServiceType._617; 1213 if ("618".equals(codeString)) 1214 return ServiceType._618; 1215 if ("619".equals(codeString)) 1216 return ServiceType._619; 1217 if ("620".equals(codeString)) 1218 return ServiceType._620; 1219 if ("621".equals(codeString)) 1220 return ServiceType._621; 1221 if ("622".equals(codeString)) 1222 return ServiceType._622; 1223 if ("623".equals(codeString)) 1224 return ServiceType._623; 1225 if ("624".equals(codeString)) 1226 return ServiceType._624; 1227 if ("625".equals(codeString)) 1228 return ServiceType._625; 1229 if ("626".equals(codeString)) 1230 return ServiceType._626; 1231 if ("627".equals(codeString)) 1232 return ServiceType._627; 1233 if ("628".equals(codeString)) 1234 return ServiceType._628; 1235 if ("629".equals(codeString)) 1236 return ServiceType._629; 1237 throw new IllegalArgumentException("Unknown ServiceType code '"+codeString+"'"); 1238 } 1239 1240 public String toCode(ServiceType code) { 1241 if (code == ServiceType.NULL) 1242 return null; 1243 if (code == ServiceType._1) 1244 return "1"; 1245 if (code == ServiceType._2) 1246 return "2"; 1247 if (code == ServiceType._3) 1248 return "3"; 1249 if (code == ServiceType._4) 1250 return "4"; 1251 if (code == ServiceType._5) 1252 return "5"; 1253 if (code == ServiceType._6) 1254 return "6"; 1255 if (code == ServiceType._7) 1256 return "7"; 1257 if (code == ServiceType._8) 1258 return "8"; 1259 if (code == ServiceType._9) 1260 return "9"; 1261 if (code == ServiceType._10) 1262 return "10"; 1263 if (code == ServiceType._11) 1264 return "11"; 1265 if (code == ServiceType._12) 1266 return "12"; 1267 if (code == ServiceType._13) 1268 return "13"; 1269 if (code == ServiceType._14) 1270 return "14"; 1271 if (code == ServiceType._15) 1272 return "15"; 1273 if (code == ServiceType._16) 1274 return "16"; 1275 if (code == ServiceType._17) 1276 return "17"; 1277 if (code == ServiceType._18) 1278 return "18"; 1279 if (code == ServiceType._19) 1280 return "19"; 1281 if (code == ServiceType._20) 1282 return "20"; 1283 if (code == ServiceType._21) 1284 return "21"; 1285 if (code == ServiceType._22) 1286 return "22"; 1287 if (code == ServiceType._23) 1288 return "23"; 1289 if (code == ServiceType._24) 1290 return "24"; 1291 if (code == ServiceType._25) 1292 return "25"; 1293 if (code == ServiceType._26) 1294 return "26"; 1295 if (code == ServiceType._27) 1296 return "27"; 1297 if (code == ServiceType._28) 1298 return "28"; 1299 if (code == ServiceType._29) 1300 return "29"; 1301 if (code == ServiceType._30) 1302 return "30"; 1303 if (code == ServiceType._31) 1304 return "31"; 1305 if (code == ServiceType._32) 1306 return "32"; 1307 if (code == ServiceType._33) 1308 return "33"; 1309 if (code == ServiceType._34) 1310 return "34"; 1311 if (code == ServiceType._35) 1312 return "35"; 1313 if (code == ServiceType._36) 1314 return "36"; 1315 if (code == ServiceType._37) 1316 return "37"; 1317 if (code == ServiceType._38) 1318 return "38"; 1319 if (code == ServiceType._39) 1320 return "39"; 1321 if (code == ServiceType._40) 1322 return "40"; 1323 if (code == ServiceType._41) 1324 return "41"; 1325 if (code == ServiceType._42) 1326 return "42"; 1327 if (code == ServiceType._43) 1328 return "43"; 1329 if (code == ServiceType._44) 1330 return "44"; 1331 if (code == ServiceType._45) 1332 return "45"; 1333 if (code == ServiceType._46) 1334 return "46"; 1335 if (code == ServiceType._47) 1336 return "47"; 1337 if (code == ServiceType._48) 1338 return "48"; 1339 if (code == ServiceType._49) 1340 return "49"; 1341 if (code == ServiceType._50) 1342 return "50"; 1343 if (code == ServiceType._51) 1344 return "51"; 1345 if (code == ServiceType._52) 1346 return "52"; 1347 if (code == ServiceType._53) 1348 return "53"; 1349 if (code == ServiceType._54) 1350 return "54"; 1351 if (code == ServiceType._55) 1352 return "55"; 1353 if (code == ServiceType._56) 1354 return "56"; 1355 if (code == ServiceType._57) 1356 return "57"; 1357 if (code == ServiceType._58) 1358 return "58"; 1359 if (code == ServiceType._59) 1360 return "59"; 1361 if (code == ServiceType._60) 1362 return "60"; 1363 if (code == ServiceType._61) 1364 return "61"; 1365 if (code == ServiceType._62) 1366 return "62"; 1367 if (code == ServiceType._63) 1368 return "63"; 1369 if (code == ServiceType._64) 1370 return "64"; 1371 if (code == ServiceType._65) 1372 return "65"; 1373 if (code == ServiceType._66) 1374 return "66"; 1375 if (code == ServiceType._67) 1376 return "67"; 1377 if (code == ServiceType._68) 1378 return "68"; 1379 if (code == ServiceType._69) 1380 return "69"; 1381 if (code == ServiceType._70) 1382 return "70"; 1383 if (code == ServiceType._71) 1384 return "71"; 1385 if (code == ServiceType._72) 1386 return "72"; 1387 if (code == ServiceType._73) 1388 return "73"; 1389 if (code == ServiceType._74) 1390 return "74"; 1391 if (code == ServiceType._75) 1392 return "75"; 1393 if (code == ServiceType._76) 1394 return "76"; 1395 if (code == ServiceType._77) 1396 return "77"; 1397 if (code == ServiceType._78) 1398 return "78"; 1399 if (code == ServiceType._79) 1400 return "79"; 1401 if (code == ServiceType._80) 1402 return "80"; 1403 if (code == ServiceType._81) 1404 return "81"; 1405 if (code == ServiceType._82) 1406 return "82"; 1407 if (code == ServiceType._83) 1408 return "83"; 1409 if (code == ServiceType._84) 1410 return "84"; 1411 if (code == ServiceType._85) 1412 return "85"; 1413 if (code == ServiceType._86) 1414 return "86"; 1415 if (code == ServiceType._87) 1416 return "87"; 1417 if (code == ServiceType._88) 1418 return "88"; 1419 if (code == ServiceType._89) 1420 return "89"; 1421 if (code == ServiceType._90) 1422 return "90"; 1423 if (code == ServiceType._91) 1424 return "91"; 1425 if (code == ServiceType._92) 1426 return "92"; 1427 if (code == ServiceType._93) 1428 return "93"; 1429 if (code == ServiceType._94) 1430 return "94"; 1431 if (code == ServiceType._95) 1432 return "95"; 1433 if (code == ServiceType._96) 1434 return "96"; 1435 if (code == ServiceType._97) 1436 return "97"; 1437 if (code == ServiceType._98) 1438 return "98"; 1439 if (code == ServiceType._99) 1440 return "99"; 1441 if (code == ServiceType._100) 1442 return "100"; 1443 if (code == ServiceType._101) 1444 return "101"; 1445 if (code == ServiceType._102) 1446 return "102"; 1447 if (code == ServiceType._103) 1448 return "103"; 1449 if (code == ServiceType._104) 1450 return "104"; 1451 if (code == ServiceType._105) 1452 return "105"; 1453 if (code == ServiceType._106) 1454 return "106"; 1455 if (code == ServiceType._107) 1456 return "107"; 1457 if (code == ServiceType._108) 1458 return "108"; 1459 if (code == ServiceType._109) 1460 return "109"; 1461 if (code == ServiceType._110) 1462 return "110"; 1463 if (code == ServiceType._111) 1464 return "111"; 1465 if (code == ServiceType._112) 1466 return "112"; 1467 if (code == ServiceType._113) 1468 return "113"; 1469 if (code == ServiceType._114) 1470 return "114"; 1471 if (code == ServiceType._115) 1472 return "115"; 1473 if (code == ServiceType._116) 1474 return "116"; 1475 if (code == ServiceType._117) 1476 return "117"; 1477 if (code == ServiceType._118) 1478 return "118"; 1479 if (code == ServiceType._119) 1480 return "119"; 1481 if (code == ServiceType._120) 1482 return "120"; 1483 if (code == ServiceType._121) 1484 return "121"; 1485 if (code == ServiceType._122) 1486 return "122"; 1487 if (code == ServiceType._123) 1488 return "123"; 1489 if (code == ServiceType._124) 1490 return "124"; 1491 if (code == ServiceType._125) 1492 return "125"; 1493 if (code == ServiceType._126) 1494 return "126"; 1495 if (code == ServiceType._127) 1496 return "127"; 1497 if (code == ServiceType._128) 1498 return "128"; 1499 if (code == ServiceType._129) 1500 return "129"; 1501 if (code == ServiceType._130) 1502 return "130"; 1503 if (code == ServiceType._131) 1504 return "131"; 1505 if (code == ServiceType._132) 1506 return "132"; 1507 if (code == ServiceType._133) 1508 return "133"; 1509 if (code == ServiceType._134) 1510 return "134"; 1511 if (code == ServiceType._135) 1512 return "135"; 1513 if (code == ServiceType._136) 1514 return "136"; 1515 if (code == ServiceType._137) 1516 return "137"; 1517 if (code == ServiceType._138) 1518 return "138"; 1519 if (code == ServiceType._139) 1520 return "139"; 1521 if (code == ServiceType._140) 1522 return "140"; 1523 if (code == ServiceType._141) 1524 return "141"; 1525 if (code == ServiceType._142) 1526 return "142"; 1527 if (code == ServiceType._143) 1528 return "143"; 1529 if (code == ServiceType._144) 1530 return "144"; 1531 if (code == ServiceType._145) 1532 return "145"; 1533 if (code == ServiceType._146) 1534 return "146"; 1535 if (code == ServiceType._147) 1536 return "147"; 1537 if (code == ServiceType._148) 1538 return "148"; 1539 if (code == ServiceType._149) 1540 return "149"; 1541 if (code == ServiceType._150) 1542 return "150"; 1543 if (code == ServiceType._151) 1544 return "151"; 1545 if (code == ServiceType._152) 1546 return "152"; 1547 if (code == ServiceType._153) 1548 return "153"; 1549 if (code == ServiceType._154) 1550 return "154"; 1551 if (code == ServiceType._155) 1552 return "155"; 1553 if (code == ServiceType._156) 1554 return "156"; 1555 if (code == ServiceType._157) 1556 return "157"; 1557 if (code == ServiceType._158) 1558 return "158"; 1559 if (code == ServiceType._159) 1560 return "159"; 1561 if (code == ServiceType._160) 1562 return "160"; 1563 if (code == ServiceType._161) 1564 return "161"; 1565 if (code == ServiceType._162) 1566 return "162"; 1567 if (code == ServiceType._163) 1568 return "163"; 1569 if (code == ServiceType._164) 1570 return "164"; 1571 if (code == ServiceType._165) 1572 return "165"; 1573 if (code == ServiceType._166) 1574 return "166"; 1575 if (code == ServiceType._167) 1576 return "167"; 1577 if (code == ServiceType._168) 1578 return "168"; 1579 if (code == ServiceType._169) 1580 return "169"; 1581 if (code == ServiceType._170) 1582 return "170"; 1583 if (code == ServiceType._171) 1584 return "171"; 1585 if (code == ServiceType._172) 1586 return "172"; 1587 if (code == ServiceType._173) 1588 return "173"; 1589 if (code == ServiceType._174) 1590 return "174"; 1591 if (code == ServiceType._175) 1592 return "175"; 1593 if (code == ServiceType._176) 1594 return "176"; 1595 if (code == ServiceType._177) 1596 return "177"; 1597 if (code == ServiceType._178) 1598 return "178"; 1599 if (code == ServiceType._179) 1600 return "179"; 1601 if (code == ServiceType._180) 1602 return "180"; 1603 if (code == ServiceType._181) 1604 return "181"; 1605 if (code == ServiceType._182) 1606 return "182"; 1607 if (code == ServiceType._183) 1608 return "183"; 1609 if (code == ServiceType._184) 1610 return "184"; 1611 if (code == ServiceType._185) 1612 return "185"; 1613 if (code == ServiceType._186) 1614 return "186"; 1615 if (code == ServiceType._187) 1616 return "187"; 1617 if (code == ServiceType._188) 1618 return "188"; 1619 if (code == ServiceType._189) 1620 return "189"; 1621 if (code == ServiceType._190) 1622 return "190"; 1623 if (code == ServiceType._191) 1624 return "191"; 1625 if (code == ServiceType._192) 1626 return "192"; 1627 if (code == ServiceType._193) 1628 return "193"; 1629 if (code == ServiceType._194) 1630 return "194"; 1631 if (code == ServiceType._195) 1632 return "195"; 1633 if (code == ServiceType._196) 1634 return "196"; 1635 if (code == ServiceType._197) 1636 return "197"; 1637 if (code == ServiceType._198) 1638 return "198"; 1639 if (code == ServiceType._199) 1640 return "199"; 1641 if (code == ServiceType._200) 1642 return "200"; 1643 if (code == ServiceType._201) 1644 return "201"; 1645 if (code == ServiceType._202) 1646 return "202"; 1647 if (code == ServiceType._203) 1648 return "203"; 1649 if (code == ServiceType._204) 1650 return "204"; 1651 if (code == ServiceType._205) 1652 return "205"; 1653 if (code == ServiceType._206) 1654 return "206"; 1655 if (code == ServiceType._207) 1656 return "207"; 1657 if (code == ServiceType._208) 1658 return "208"; 1659 if (code == ServiceType._209) 1660 return "209"; 1661 if (code == ServiceType._210) 1662 return "210"; 1663 if (code == ServiceType._211) 1664 return "211"; 1665 if (code == ServiceType._212) 1666 return "212"; 1667 if (code == ServiceType._213) 1668 return "213"; 1669 if (code == ServiceType._214) 1670 return "214"; 1671 if (code == ServiceType._215) 1672 return "215"; 1673 if (code == ServiceType._216) 1674 return "216"; 1675 if (code == ServiceType._217) 1676 return "217"; 1677 if (code == ServiceType._218) 1678 return "218"; 1679 if (code == ServiceType._219) 1680 return "219"; 1681 if (code == ServiceType._220) 1682 return "220"; 1683 if (code == ServiceType._221) 1684 return "221"; 1685 if (code == ServiceType._222) 1686 return "222"; 1687 if (code == ServiceType._223) 1688 return "223"; 1689 if (code == ServiceType._224) 1690 return "224"; 1691 if (code == ServiceType._225) 1692 return "225"; 1693 if (code == ServiceType._226) 1694 return "226"; 1695 if (code == ServiceType._227) 1696 return "227"; 1697 if (code == ServiceType._228) 1698 return "228"; 1699 if (code == ServiceType._229) 1700 return "229"; 1701 if (code == ServiceType._230) 1702 return "230"; 1703 if (code == ServiceType._231) 1704 return "231"; 1705 if (code == ServiceType._232) 1706 return "232"; 1707 if (code == ServiceType._233) 1708 return "233"; 1709 if (code == ServiceType._234) 1710 return "234"; 1711 if (code == ServiceType._235) 1712 return "235"; 1713 if (code == ServiceType._236) 1714 return "236"; 1715 if (code == ServiceType._237) 1716 return "237"; 1717 if (code == ServiceType._238) 1718 return "238"; 1719 if (code == ServiceType._239) 1720 return "239"; 1721 if (code == ServiceType._240) 1722 return "240"; 1723 if (code == ServiceType._241) 1724 return "241"; 1725 if (code == ServiceType._242) 1726 return "242"; 1727 if (code == ServiceType._243) 1728 return "243"; 1729 if (code == ServiceType._244) 1730 return "244"; 1731 if (code == ServiceType._245) 1732 return "245"; 1733 if (code == ServiceType._246) 1734 return "246"; 1735 if (code == ServiceType._247) 1736 return "247"; 1737 if (code == ServiceType._248) 1738 return "248"; 1739 if (code == ServiceType._249) 1740 return "249"; 1741 if (code == ServiceType._250) 1742 return "250"; 1743 if (code == ServiceType._251) 1744 return "251"; 1745 if (code == ServiceType._252) 1746 return "252"; 1747 if (code == ServiceType._253) 1748 return "253"; 1749 if (code == ServiceType._254) 1750 return "254"; 1751 if (code == ServiceType._255) 1752 return "255"; 1753 if (code == ServiceType._256) 1754 return "256"; 1755 if (code == ServiceType._257) 1756 return "257"; 1757 if (code == ServiceType._258) 1758 return "258"; 1759 if (code == ServiceType._259) 1760 return "259"; 1761 if (code == ServiceType._260) 1762 return "260"; 1763 if (code == ServiceType._261) 1764 return "261"; 1765 if (code == ServiceType._262) 1766 return "262"; 1767 if (code == ServiceType._263) 1768 return "263"; 1769 if (code == ServiceType._264) 1770 return "264"; 1771 if (code == ServiceType._265) 1772 return "265"; 1773 if (code == ServiceType._266) 1774 return "266"; 1775 if (code == ServiceType._267) 1776 return "267"; 1777 if (code == ServiceType._268) 1778 return "268"; 1779 if (code == ServiceType._269) 1780 return "269"; 1781 if (code == ServiceType._270) 1782 return "270"; 1783 if (code == ServiceType._271) 1784 return "271"; 1785 if (code == ServiceType._272) 1786 return "272"; 1787 if (code == ServiceType._273) 1788 return "273"; 1789 if (code == ServiceType._274) 1790 return "274"; 1791 if (code == ServiceType._275) 1792 return "275"; 1793 if (code == ServiceType._276) 1794 return "276"; 1795 if (code == ServiceType._277) 1796 return "277"; 1797 if (code == ServiceType._278) 1798 return "278"; 1799 if (code == ServiceType._279) 1800 return "279"; 1801 if (code == ServiceType._280) 1802 return "280"; 1803 if (code == ServiceType._281) 1804 return "281"; 1805 if (code == ServiceType._282) 1806 return "282"; 1807 if (code == ServiceType._283) 1808 return "283"; 1809 if (code == ServiceType._284) 1810 return "284"; 1811 if (code == ServiceType._285) 1812 return "285"; 1813 if (code == ServiceType._286) 1814 return "286"; 1815 if (code == ServiceType._287) 1816 return "287"; 1817 if (code == ServiceType._288) 1818 return "288"; 1819 if (code == ServiceType._289) 1820 return "289"; 1821 if (code == ServiceType._290) 1822 return "290"; 1823 if (code == ServiceType._291) 1824 return "291"; 1825 if (code == ServiceType._292) 1826 return "292"; 1827 if (code == ServiceType._293) 1828 return "293"; 1829 if (code == ServiceType._294) 1830 return "294"; 1831 if (code == ServiceType._295) 1832 return "295"; 1833 if (code == ServiceType._296) 1834 return "296"; 1835 if (code == ServiceType._297) 1836 return "297"; 1837 if (code == ServiceType._298) 1838 return "298"; 1839 if (code == ServiceType._299) 1840 return "299"; 1841 if (code == ServiceType._300) 1842 return "300"; 1843 if (code == ServiceType._301) 1844 return "301"; 1845 if (code == ServiceType._302) 1846 return "302"; 1847 if (code == ServiceType._303) 1848 return "303"; 1849 if (code == ServiceType._304) 1850 return "304"; 1851 if (code == ServiceType._305) 1852 return "305"; 1853 if (code == ServiceType._306) 1854 return "306"; 1855 if (code == ServiceType._307) 1856 return "307"; 1857 if (code == ServiceType._308) 1858 return "308"; 1859 if (code == ServiceType._309) 1860 return "309"; 1861 if (code == ServiceType._310) 1862 return "310"; 1863 if (code == ServiceType._311) 1864 return "311"; 1865 if (code == ServiceType._312) 1866 return "312"; 1867 if (code == ServiceType._313) 1868 return "313"; 1869 if (code == ServiceType._314) 1870 return "314"; 1871 if (code == ServiceType._315) 1872 return "315"; 1873 if (code == ServiceType._316) 1874 return "316"; 1875 if (code == ServiceType._317) 1876 return "317"; 1877 if (code == ServiceType._318) 1878 return "318"; 1879 if (code == ServiceType._319) 1880 return "319"; 1881 if (code == ServiceType._320) 1882 return "320"; 1883 if (code == ServiceType._321) 1884 return "321"; 1885 if (code == ServiceType._322) 1886 return "322"; 1887 if (code == ServiceType._323) 1888 return "323"; 1889 if (code == ServiceType._324) 1890 return "324"; 1891 if (code == ServiceType._325) 1892 return "325"; 1893 if (code == ServiceType._326) 1894 return "326"; 1895 if (code == ServiceType._327) 1896 return "327"; 1897 if (code == ServiceType._328) 1898 return "328"; 1899 if (code == ServiceType._330) 1900 return "330"; 1901 if (code == ServiceType._331) 1902 return "331"; 1903 if (code == ServiceType._332) 1904 return "332"; 1905 if (code == ServiceType._333) 1906 return "333"; 1907 if (code == ServiceType._334) 1908 return "334"; 1909 if (code == ServiceType._335) 1910 return "335"; 1911 if (code == ServiceType._336) 1912 return "336"; 1913 if (code == ServiceType._337) 1914 return "337"; 1915 if (code == ServiceType._338) 1916 return "338"; 1917 if (code == ServiceType._339) 1918 return "339"; 1919 if (code == ServiceType._340) 1920 return "340"; 1921 if (code == ServiceType._341) 1922 return "341"; 1923 if (code == ServiceType._342) 1924 return "342"; 1925 if (code == ServiceType._343) 1926 return "343"; 1927 if (code == ServiceType._344) 1928 return "344"; 1929 if (code == ServiceType._345) 1930 return "345"; 1931 if (code == ServiceType._346) 1932 return "346"; 1933 if (code == ServiceType._347) 1934 return "347"; 1935 if (code == ServiceType._348) 1936 return "348"; 1937 if (code == ServiceType._349) 1938 return "349"; 1939 if (code == ServiceType._350) 1940 return "350"; 1941 if (code == ServiceType._351) 1942 return "351"; 1943 if (code == ServiceType._352) 1944 return "352"; 1945 if (code == ServiceType._353) 1946 return "353"; 1947 if (code == ServiceType._354) 1948 return "354"; 1949 if (code == ServiceType._355) 1950 return "355"; 1951 if (code == ServiceType._356) 1952 return "356"; 1953 if (code == ServiceType._357) 1954 return "357"; 1955 if (code == ServiceType._358) 1956 return "358"; 1957 if (code == ServiceType._359) 1958 return "359"; 1959 if (code == ServiceType._360) 1960 return "360"; 1961 if (code == ServiceType._361) 1962 return "361"; 1963 if (code == ServiceType._362) 1964 return "362"; 1965 if (code == ServiceType._364) 1966 return "364"; 1967 if (code == ServiceType._365) 1968 return "365"; 1969 if (code == ServiceType._366) 1970 return "366"; 1971 if (code == ServiceType._367) 1972 return "367"; 1973 if (code == ServiceType._368) 1974 return "368"; 1975 if (code == ServiceType._369) 1976 return "369"; 1977 if (code == ServiceType._370) 1978 return "370"; 1979 if (code == ServiceType._371) 1980 return "371"; 1981 if (code == ServiceType._372) 1982 return "372"; 1983 if (code == ServiceType._373) 1984 return "373"; 1985 if (code == ServiceType._374) 1986 return "374"; 1987 if (code == ServiceType._375) 1988 return "375"; 1989 if (code == ServiceType._376) 1990 return "376"; 1991 if (code == ServiceType._377) 1992 return "377"; 1993 if (code == ServiceType._378) 1994 return "378"; 1995 if (code == ServiceType._379) 1996 return "379"; 1997 if (code == ServiceType._380) 1998 return "380"; 1999 if (code == ServiceType._381) 2000 return "381"; 2001 if (code == ServiceType._382) 2002 return "382"; 2003 if (code == ServiceType._383) 2004 return "383"; 2005 if (code == ServiceType._384) 2006 return "384"; 2007 if (code == ServiceType._385) 2008 return "385"; 2009 if (code == ServiceType._386) 2010 return "386"; 2011 if (code == ServiceType._387) 2012 return "387"; 2013 if (code == ServiceType._388) 2014 return "388"; 2015 if (code == ServiceType._389) 2016 return "389"; 2017 if (code == ServiceType._390) 2018 return "390"; 2019 if (code == ServiceType._391) 2020 return "391"; 2021 if (code == ServiceType._392) 2022 return "392"; 2023 if (code == ServiceType._393) 2024 return "393"; 2025 if (code == ServiceType._394) 2026 return "394"; 2027 if (code == ServiceType._395) 2028 return "395"; 2029 if (code == ServiceType._396) 2030 return "396"; 2031 if (code == ServiceType._397) 2032 return "397"; 2033 if (code == ServiceType._398) 2034 return "398"; 2035 if (code == ServiceType._399) 2036 return "399"; 2037 if (code == ServiceType._400) 2038 return "400"; 2039 if (code == ServiceType._401) 2040 return "401"; 2041 if (code == ServiceType._402) 2042 return "402"; 2043 if (code == ServiceType._403) 2044 return "403"; 2045 if (code == ServiceType._404) 2046 return "404"; 2047 if (code == ServiceType._405) 2048 return "405"; 2049 if (code == ServiceType._406) 2050 return "406"; 2051 if (code == ServiceType._407) 2052 return "407"; 2053 if (code == ServiceType._408) 2054 return "408"; 2055 if (code == ServiceType._409) 2056 return "409"; 2057 if (code == ServiceType._410) 2058 return "410"; 2059 if (code == ServiceType._411) 2060 return "411"; 2061 if (code == ServiceType._412) 2062 return "412"; 2063 if (code == ServiceType._413) 2064 return "413"; 2065 if (code == ServiceType._414) 2066 return "414"; 2067 if (code == ServiceType._415) 2068 return "415"; 2069 if (code == ServiceType._416) 2070 return "416"; 2071 if (code == ServiceType._417) 2072 return "417"; 2073 if (code == ServiceType._418) 2074 return "418"; 2075 if (code == ServiceType._419) 2076 return "419"; 2077 if (code == ServiceType._420) 2078 return "420"; 2079 if (code == ServiceType._421) 2080 return "421"; 2081 if (code == ServiceType._422) 2082 return "422"; 2083 if (code == ServiceType._423) 2084 return "423"; 2085 if (code == ServiceType._424) 2086 return "424"; 2087 if (code == ServiceType._425) 2088 return "425"; 2089 if (code == ServiceType._426) 2090 return "426"; 2091 if (code == ServiceType._427) 2092 return "427"; 2093 if (code == ServiceType._428) 2094 return "428"; 2095 if (code == ServiceType._429) 2096 return "429"; 2097 if (code == ServiceType._430) 2098 return "430"; 2099 if (code == ServiceType._431) 2100 return "431"; 2101 if (code == ServiceType._432) 2102 return "432"; 2103 if (code == ServiceType._433) 2104 return "433"; 2105 if (code == ServiceType._434) 2106 return "434"; 2107 if (code == ServiceType._435) 2108 return "435"; 2109 if (code == ServiceType._436) 2110 return "436"; 2111 if (code == ServiceType._437) 2112 return "437"; 2113 if (code == ServiceType._438) 2114 return "438"; 2115 if (code == ServiceType._439) 2116 return "439"; 2117 if (code == ServiceType._440) 2118 return "440"; 2119 if (code == ServiceType._441) 2120 return "441"; 2121 if (code == ServiceType._442) 2122 return "442"; 2123 if (code == ServiceType._443) 2124 return "443"; 2125 if (code == ServiceType._444) 2126 return "444"; 2127 if (code == ServiceType._445) 2128 return "445"; 2129 if (code == ServiceType._446) 2130 return "446"; 2131 if (code == ServiceType._447) 2132 return "447"; 2133 if (code == ServiceType._448) 2134 return "448"; 2135 if (code == ServiceType._449) 2136 return "449"; 2137 if (code == ServiceType._450) 2138 return "450"; 2139 if (code == ServiceType._451) 2140 return "451"; 2141 if (code == ServiceType._452) 2142 return "452"; 2143 if (code == ServiceType._453) 2144 return "453"; 2145 if (code == ServiceType._454) 2146 return "454"; 2147 if (code == ServiceType._455) 2148 return "455"; 2149 if (code == ServiceType._456) 2150 return "456"; 2151 if (code == ServiceType._457) 2152 return "457"; 2153 if (code == ServiceType._458) 2154 return "458"; 2155 if (code == ServiceType._459) 2156 return "459"; 2157 if (code == ServiceType._460) 2158 return "460"; 2159 if (code == ServiceType._461) 2160 return "461"; 2161 if (code == ServiceType._462) 2162 return "462"; 2163 if (code == ServiceType._463) 2164 return "463"; 2165 if (code == ServiceType._464) 2166 return "464"; 2167 if (code == ServiceType._465) 2168 return "465"; 2169 if (code == ServiceType._466) 2170 return "466"; 2171 if (code == ServiceType._467) 2172 return "467"; 2173 if (code == ServiceType._468) 2174 return "468"; 2175 if (code == ServiceType._469) 2176 return "469"; 2177 if (code == ServiceType._470) 2178 return "470"; 2179 if (code == ServiceType._471) 2180 return "471"; 2181 if (code == ServiceType._472) 2182 return "472"; 2183 if (code == ServiceType._473) 2184 return "473"; 2185 if (code == ServiceType._474) 2186 return "474"; 2187 if (code == ServiceType._475) 2188 return "475"; 2189 if (code == ServiceType._476) 2190 return "476"; 2191 if (code == ServiceType._477) 2192 return "477"; 2193 if (code == ServiceType._478) 2194 return "478"; 2195 if (code == ServiceType._479) 2196 return "479"; 2197 if (code == ServiceType._480) 2198 return "480"; 2199 if (code == ServiceType._481) 2200 return "481"; 2201 if (code == ServiceType._482) 2202 return "482"; 2203 if (code == ServiceType._483) 2204 return "483"; 2205 if (code == ServiceType._484) 2206 return "484"; 2207 if (code == ServiceType._485) 2208 return "485"; 2209 if (code == ServiceType._486) 2210 return "486"; 2211 if (code == ServiceType._488) 2212 return "488"; 2213 if (code == ServiceType._489) 2214 return "489"; 2215 if (code == ServiceType._490) 2216 return "490"; 2217 if (code == ServiceType._491) 2218 return "491"; 2219 if (code == ServiceType._492) 2220 return "492"; 2221 if (code == ServiceType._493) 2222 return "493"; 2223 if (code == ServiceType._494) 2224 return "494"; 2225 if (code == ServiceType._495) 2226 return "495"; 2227 if (code == ServiceType._496) 2228 return "496"; 2229 if (code == ServiceType._497) 2230 return "497"; 2231 if (code == ServiceType._498) 2232 return "498"; 2233 if (code == ServiceType._500) 2234 return "500"; 2235 if (code == ServiceType._501) 2236 return "501"; 2237 if (code == ServiceType._502) 2238 return "502"; 2239 if (code == ServiceType._503) 2240 return "503"; 2241 if (code == ServiceType._504) 2242 return "504"; 2243 if (code == ServiceType._505) 2244 return "505"; 2245 if (code == ServiceType._506) 2246 return "506"; 2247 if (code == ServiceType._507) 2248 return "507"; 2249 if (code == ServiceType._508) 2250 return "508"; 2251 if (code == ServiceType._509) 2252 return "509"; 2253 if (code == ServiceType._510) 2254 return "510"; 2255 if (code == ServiceType._513) 2256 return "513"; 2257 if (code == ServiceType._514) 2258 return "514"; 2259 if (code == ServiceType._530) 2260 return "530"; 2261 if (code == ServiceType._531) 2262 return "531"; 2263 if (code == ServiceType._532) 2264 return "532"; 2265 if (code == ServiceType._533) 2266 return "533"; 2267 if (code == ServiceType._534) 2268 return "534"; 2269 if (code == ServiceType._535) 2270 return "535"; 2271 if (code == ServiceType._536) 2272 return "536"; 2273 if (code == ServiceType._537) 2274 return "537"; 2275 if (code == ServiceType._538) 2276 return "538"; 2277 if (code == ServiceType._539) 2278 return "539"; 2279 if (code == ServiceType._540) 2280 return "540"; 2281 if (code == ServiceType._541) 2282 return "541"; 2283 if (code == ServiceType._542) 2284 return "542"; 2285 if (code == ServiceType._543) 2286 return "543"; 2287 if (code == ServiceType._544) 2288 return "544"; 2289 if (code == ServiceType._545) 2290 return "545"; 2291 if (code == ServiceType._546) 2292 return "546"; 2293 if (code == ServiceType._547) 2294 return "547"; 2295 if (code == ServiceType._548) 2296 return "548"; 2297 if (code == ServiceType._550) 2298 return "550"; 2299 if (code == ServiceType._551) 2300 return "551"; 2301 if (code == ServiceType._552) 2302 return "552"; 2303 if (code == ServiceType._553) 2304 return "553"; 2305 if (code == ServiceType._554) 2306 return "554"; 2307 if (code == ServiceType._555) 2308 return "555"; 2309 if (code == ServiceType._556) 2310 return "556"; 2311 if (code == ServiceType._557) 2312 return "557"; 2313 if (code == ServiceType._558) 2314 return "558"; 2315 if (code == ServiceType._559) 2316 return "559"; 2317 if (code == ServiceType._560) 2318 return "560"; 2319 if (code == ServiceType._561) 2320 return "561"; 2321 if (code == ServiceType._562) 2322 return "562"; 2323 if (code == ServiceType._563) 2324 return "563"; 2325 if (code == ServiceType._564) 2326 return "564"; 2327 if (code == ServiceType._565) 2328 return "565"; 2329 if (code == ServiceType._566) 2330 return "566"; 2331 if (code == ServiceType._567) 2332 return "567"; 2333 if (code == ServiceType._568) 2334 return "568"; 2335 if (code == ServiceType._569) 2336 return "569"; 2337 if (code == ServiceType._570) 2338 return "570"; 2339 if (code == ServiceType._571) 2340 return "571"; 2341 if (code == ServiceType._572) 2342 return "572"; 2343 if (code == ServiceType._573) 2344 return "573"; 2345 if (code == ServiceType._574) 2346 return "574"; 2347 if (code == ServiceType._575) 2348 return "575"; 2349 if (code == ServiceType._576) 2350 return "576"; 2351 if (code == ServiceType._577) 2352 return "577"; 2353 if (code == ServiceType._580) 2354 return "580"; 2355 if (code == ServiceType._581) 2356 return "581"; 2357 if (code == ServiceType._582) 2358 return "582"; 2359 if (code == ServiceType._583) 2360 return "583"; 2361 if (code == ServiceType._584) 2362 return "584"; 2363 if (code == ServiceType._585) 2364 return "585"; 2365 if (code == ServiceType._589) 2366 return "589"; 2367 if (code == ServiceType._590) 2368 return "590"; 2369 if (code == ServiceType._591) 2370 return "591"; 2371 if (code == ServiceType._593) 2372 return "593"; 2373 if (code == ServiceType._599) 2374 return "599"; 2375 if (code == ServiceType._600) 2376 return "600"; 2377 if (code == ServiceType._601) 2378 return "601"; 2379 if (code == ServiceType._602) 2380 return "602"; 2381 if (code == ServiceType._603) 2382 return "603"; 2383 if (code == ServiceType._604) 2384 return "604"; 2385 if (code == ServiceType._605) 2386 return "605"; 2387 if (code == ServiceType._606) 2388 return "606"; 2389 if (code == ServiceType._607) 2390 return "607"; 2391 if (code == ServiceType._608) 2392 return "608"; 2393 if (code == ServiceType._609) 2394 return "609"; 2395 if (code == ServiceType._610) 2396 return "610"; 2397 if (code == ServiceType._611) 2398 return "611"; 2399 if (code == ServiceType._612) 2400 return "612"; 2401 if (code == ServiceType._613) 2402 return "613"; 2403 if (code == ServiceType._614) 2404 return "614"; 2405 if (code == ServiceType._615) 2406 return "615"; 2407 if (code == ServiceType._616) 2408 return "616"; 2409 if (code == ServiceType._617) 2410 return "617"; 2411 if (code == ServiceType._618) 2412 return "618"; 2413 if (code == ServiceType._619) 2414 return "619"; 2415 if (code == ServiceType._620) 2416 return "620"; 2417 if (code == ServiceType._621) 2418 return "621"; 2419 if (code == ServiceType._622) 2420 return "622"; 2421 if (code == ServiceType._623) 2422 return "623"; 2423 if (code == ServiceType._624) 2424 return "624"; 2425 if (code == ServiceType._625) 2426 return "625"; 2427 if (code == ServiceType._626) 2428 return "626"; 2429 if (code == ServiceType._627) 2430 return "627"; 2431 if (code == ServiceType._628) 2432 return "628"; 2433 if (code == ServiceType._629) 2434 return "629"; 2435 return "?"; 2436 } 2437 2438 public String toSystem(ServiceType code) { 2439 return code.getSystem(); 2440 } 2441 2442}