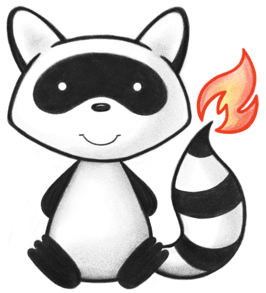
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum ServiceUscls { 041 042 /** 043 * Exam, comp, primary 044 */ 045 _1101, 046 /** 047 * Exam, comp, mixed 048 */ 049 _1102, 050 /** 051 * Exam, comp, permanent 052 */ 053 _1103, 054 /** 055 * Exam, recall 056 */ 057 _1201, 058 /** 059 * Exam, emergency 060 */ 061 _1205, 062 /** 063 * Radiograph, series (12) 064 */ 065 _2101, 066 /** 067 * Radiograph, series (16) 068 */ 069 _2102, 070 /** 071 * Radiograph, bytewing 072 */ 073 _2141, 074 /** 075 * Radiograph, panoramic 076 */ 077 _2601, 078 /** 079 * Polishing, 1 unit 080 */ 081 _11101, 082 /** 083 * Polishing, 2 unit 084 */ 085 _11102, 086 /** 087 * Polishing, 3 unit 088 */ 089 _11103, 090 /** 091 * Polishing, 4 unit 092 */ 093 _11104, 094 /** 095 * Amalgam, 1 surface 096 */ 097 _21211, 098 /** 099 * Amalgam, 2 surface 100 */ 101 _21212, 102 /** 103 * Crown, PFM 104 */ 105 _27211, 106 /** 107 * Maryland Bridge 108 */ 109 _67211, 110 /** 111 * Lab, commercial 112 */ 113 _99111, 114 /** 115 * Lab, in office 116 */ 117 _99333, 118 /** 119 * Expense 120 */ 121 _99555, 122 /** 123 * added to help the parsers 124 */ 125 NULL; 126 public static ServiceUscls fromCode(String codeString) throws FHIRException { 127 if (codeString == null || "".equals(codeString)) 128 return null; 129 if ("1101".equals(codeString)) 130 return _1101; 131 if ("1102".equals(codeString)) 132 return _1102; 133 if ("1103".equals(codeString)) 134 return _1103; 135 if ("1201".equals(codeString)) 136 return _1201; 137 if ("1205".equals(codeString)) 138 return _1205; 139 if ("2101".equals(codeString)) 140 return _2101; 141 if ("2102".equals(codeString)) 142 return _2102; 143 if ("2141".equals(codeString)) 144 return _2141; 145 if ("2601".equals(codeString)) 146 return _2601; 147 if ("11101".equals(codeString)) 148 return _11101; 149 if ("11102".equals(codeString)) 150 return _11102; 151 if ("11103".equals(codeString)) 152 return _11103; 153 if ("11104".equals(codeString)) 154 return _11104; 155 if ("21211".equals(codeString)) 156 return _21211; 157 if ("21212".equals(codeString)) 158 return _21212; 159 if ("27211".equals(codeString)) 160 return _27211; 161 if ("67211".equals(codeString)) 162 return _67211; 163 if ("99111".equals(codeString)) 164 return _99111; 165 if ("99333".equals(codeString)) 166 return _99333; 167 if ("99555".equals(codeString)) 168 return _99555; 169 throw new FHIRException("Unknown ServiceUscls code '"+codeString+"'"); 170 } 171 public String toCode() { 172 switch (this) { 173 case _1101: return "1101"; 174 case _1102: return "1102"; 175 case _1103: return "1103"; 176 case _1201: return "1201"; 177 case _1205: return "1205"; 178 case _2101: return "2101"; 179 case _2102: return "2102"; 180 case _2141: return "2141"; 181 case _2601: return "2601"; 182 case _11101: return "11101"; 183 case _11102: return "11102"; 184 case _11103: return "11103"; 185 case _11104: return "11104"; 186 case _21211: return "21211"; 187 case _21212: return "21212"; 188 case _27211: return "27211"; 189 case _67211: return "67211"; 190 case _99111: return "99111"; 191 case _99333: return "99333"; 192 case _99555: return "99555"; 193 case NULL: return null; 194 default: return "?"; 195 } 196 } 197 public String getSystem() { 198 return "http://hl7.org/fhir/ex-USCLS"; 199 } 200 public String getDefinition() { 201 switch (this) { 202 case _1101: return "Exam, comp, primary"; 203 case _1102: return "Exam, comp, mixed"; 204 case _1103: return "Exam, comp, permanent"; 205 case _1201: return "Exam, recall"; 206 case _1205: return "Exam, emergency"; 207 case _2101: return "Radiograph, series (12)"; 208 case _2102: return "Radiograph, series (16)"; 209 case _2141: return "Radiograph, bytewing"; 210 case _2601: return "Radiograph, panoramic"; 211 case _11101: return "Polishing, 1 unit"; 212 case _11102: return "Polishing, 2 unit"; 213 case _11103: return "Polishing, 3 unit"; 214 case _11104: return "Polishing, 4 unit"; 215 case _21211: return "Amalgam, 1 surface"; 216 case _21212: return "Amalgam, 2 surface"; 217 case _27211: return "Crown, PFM"; 218 case _67211: return "Maryland Bridge"; 219 case _99111: return "Lab, commercial"; 220 case _99333: return "Lab, in office"; 221 case _99555: return "Expense"; 222 case NULL: return null; 223 default: return "?"; 224 } 225 } 226 public String getDisplay() { 227 switch (this) { 228 case _1101: return "Exam, comp, primary"; 229 case _1102: return "Exam, comp, mixed"; 230 case _1103: return "Exam, comp, permanent"; 231 case _1201: return "Exam, recall"; 232 case _1205: return "Exam, emergency"; 233 case _2101: return "Radiograph, series (12)"; 234 case _2102: return "Radiograph, series (16)"; 235 case _2141: return "Radiograph, bytewing"; 236 case _2601: return "Radiograph, panoramic"; 237 case _11101: return "Polishing, 1 unit"; 238 case _11102: return "Polishing, 2 unit"; 239 case _11103: return "Polishing, 3 unit"; 240 case _11104: return "Polishing, 4 unit"; 241 case _21211: return "Amalgam, 1 surface"; 242 case _21212: return "Amalgam, 2 surface"; 243 case _27211: return "Crown, PFM"; 244 case _67211: return "Maryland Bridge"; 245 case _99111: return "Lab, commercial"; 246 case _99333: return "Lab, in office"; 247 case _99555: return "Expense"; 248 case NULL: return null; 249 default: return "?"; 250 } 251 } 252 253 254}