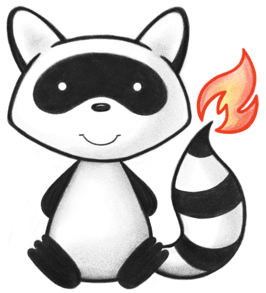
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum SpecificationType { 041 042 /** 043 * Unspecified Production Specification - MDC_ID_PROD_SPEC_UNSPECIFIED 044 */ 045 UNSPECIFIED, 046 /** 047 * Serial Number - MDC_ID_PROD_SPEC_SERIAL 048 */ 049 SERIALNUMBER, 050 /** 051 * Part Number - MDC_ID_PROD_SPEC_PART 052 */ 053 PARTNUMBER, 054 /** 055 * Hardware Revision - MDC_ID_PROD_SPEC_HW 056 */ 057 HARDWAREREVISION, 058 /** 059 * Software Revision - MDC_ID_PROD_SPEC_SW 060 */ 061 SOFTWAREREVISION, 062 /** 063 * Firmware Revision - MDC_ID_PROD_SPEC_FW 064 */ 065 FIRMWAREREVISION, 066 /** 067 * Protocol Revision - MDC_ID_PROD_SPEC_PROTOCOL 068 */ 069 PROTOCOLREVISION, 070 /** 071 * GMDN - MDC_ID_PROD_SPEC_GMDN 072 */ 073 GMDN, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 public static SpecificationType fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("unspecified".equals(codeString)) 082 return UNSPECIFIED; 083 if ("serial-number".equals(codeString)) 084 return SERIALNUMBER; 085 if ("part-number".equals(codeString)) 086 return PARTNUMBER; 087 if ("hardware-revision".equals(codeString)) 088 return HARDWAREREVISION; 089 if ("software-revision".equals(codeString)) 090 return SOFTWAREREVISION; 091 if ("firmware-revision".equals(codeString)) 092 return FIRMWAREREVISION; 093 if ("protocol-revision".equals(codeString)) 094 return PROTOCOLREVISION; 095 if ("gmdn".equals(codeString)) 096 return GMDN; 097 throw new FHIRException("Unknown SpecificationType code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case UNSPECIFIED: return "unspecified"; 102 case SERIALNUMBER: return "serial-number"; 103 case PARTNUMBER: return "part-number"; 104 case HARDWAREREVISION: return "hardware-revision"; 105 case SOFTWAREREVISION: return "software-revision"; 106 case FIRMWAREREVISION: return "firmware-revision"; 107 case PROTOCOLREVISION: return "protocol-revision"; 108 case GMDN: return "gmdn"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getSystem() { 114 return "http://hl7.org/fhir/specification-type"; 115 } 116 public String getDefinition() { 117 switch (this) { 118 case UNSPECIFIED: return "Unspecified Production Specification - MDC_ID_PROD_SPEC_UNSPECIFIED"; 119 case SERIALNUMBER: return "Serial Number - MDC_ID_PROD_SPEC_SERIAL"; 120 case PARTNUMBER: return "Part Number - MDC_ID_PROD_SPEC_PART"; 121 case HARDWAREREVISION: return "Hardware Revision - MDC_ID_PROD_SPEC_HW"; 122 case SOFTWAREREVISION: return "Software Revision - MDC_ID_PROD_SPEC_SW"; 123 case FIRMWAREREVISION: return "Firmware Revision - MDC_ID_PROD_SPEC_FW"; 124 case PROTOCOLREVISION: return "Protocol Revision - MDC_ID_PROD_SPEC_PROTOCOL"; 125 case GMDN: return "GMDN - MDC_ID_PROD_SPEC_GMDN"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case UNSPECIFIED: return "Unspecified Production Specification"; 133 case SERIALNUMBER: return "Serial Number"; 134 case PARTNUMBER: return "Part Number"; 135 case HARDWAREREVISION: return "Hardware Revision"; 136 case SOFTWAREREVISION: return "Software Revision"; 137 case FIRMWAREREVISION: return "Firmware Revision"; 138 case PROTOCOLREVISION: return "Protocol Revision"; 139 case GMDN: return "GMDN"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 145 146}