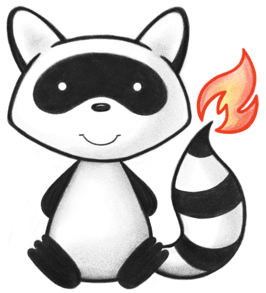
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Surface { 041 042 /** 043 * The surface of a tooth that is closest to the midline (middle) of the face. 044 */ 045 M, 046 /** 047 * The chewing surface of posterior teeth. 048 */ 049 O, 050 /** 051 * The biting edge of anterior teeth. 052 */ 053 I, 054 /** 055 * The surface of a tooth that faces away from the midline of the face. 056 */ 057 D, 058 /** 059 * The surface of a posterior tooth facing the cheeks. 060 */ 061 B, 062 /** 063 * The surface of a tooth facing the lips. 064 */ 065 V, 066 /** 067 * The surface of a tooth facing the tongue. 068 */ 069 L, 070 /** 071 * The Mesioclusal surfaces of a tooth. 072 */ 073 MO, 074 /** 075 * The Distoclusal surfaces of a tooth. 076 */ 077 DO, 078 /** 079 * The Distoincisal surfaces of a tooth. 080 */ 081 DI, 082 /** 083 * The Mesioclusodistal surfaces of a tooth. 084 */ 085 MOD, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 public static Surface fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("M".equals(codeString)) 094 return M; 095 if ("O".equals(codeString)) 096 return O; 097 if ("I".equals(codeString)) 098 return I; 099 if ("D".equals(codeString)) 100 return D; 101 if ("B".equals(codeString)) 102 return B; 103 if ("V".equals(codeString)) 104 return V; 105 if ("L".equals(codeString)) 106 return L; 107 if ("MO".equals(codeString)) 108 return MO; 109 if ("DO".equals(codeString)) 110 return DO; 111 if ("DI".equals(codeString)) 112 return DI; 113 if ("MOD".equals(codeString)) 114 return MOD; 115 throw new FHIRException("Unknown Surface code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case M: return "M"; 120 case O: return "O"; 121 case I: return "I"; 122 case D: return "D"; 123 case B: return "B"; 124 case V: return "V"; 125 case L: return "L"; 126 case MO: return "MO"; 127 case DO: return "DO"; 128 case DI: return "DI"; 129 case MOD: return "MOD"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getSystem() { 135 return "http://hl7.org/fhir/FDI-surface"; 136 } 137 public String getDefinition() { 138 switch (this) { 139 case M: return "The surface of a tooth that is closest to the midline (middle) of the face."; 140 case O: return "The chewing surface of posterior teeth."; 141 case I: return "The biting edge of anterior teeth."; 142 case D: return "The surface of a tooth that faces away from the midline of the face."; 143 case B: return "The surface of a posterior tooth facing the cheeks."; 144 case V: return "The surface of a tooth facing the lips."; 145 case L: return "The surface of a tooth facing the tongue."; 146 case MO: return "The Mesioclusal surfaces of a tooth."; 147 case DO: return "The Distoclusal surfaces of a tooth."; 148 case DI: return "The Distoincisal surfaces of a tooth."; 149 case MOD: return "The Mesioclusodistal surfaces of a tooth."; 150 case NULL: return null; 151 default: return "?"; 152 } 153 } 154 public String getDisplay() { 155 switch (this) { 156 case M: return "Mesial"; 157 case O: return "Occlusal"; 158 case I: return "Incisal"; 159 case D: return "Distal"; 160 case B: return "Buccal"; 161 case V: return "Ventral"; 162 case L: return "Lingual"; 163 case MO: return "Mesioclusal"; 164 case DO: return "Distoclusal"; 165 case DI: return "Distoincisal"; 166 case MOD: return "Mesioclusodistal"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 172 173}