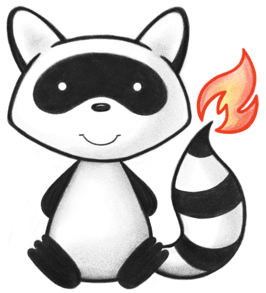
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum TaskStatus { 041 042 /** 043 * The task is not yet ready to be acted upon. 044 */ 045 DRAFT, 046 /** 047 * The task is ready to be acted upon and action is sought. 048 */ 049 REQUESTED, 050 /** 051 * A potential performer has claimed ownership of the task and is evaluating whether to perform it. 052 */ 053 RECEIVED, 054 /** 055 * The potential performer has agreed to execute the task but has not yet started work. 056 */ 057 ACCEPTED, 058 /** 059 * The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action. 060 */ 061 REJECTED, 062 /** 063 * Task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given. 064 */ 065 READY, 066 /** 067 * The task was not completed. 068 */ 069 CANCELLED, 070 /** 071 * Task has been started but is not yet complete. 072 */ 073 INPROGRESS, 074 /** 075 * Task has been started but work has been paused. 076 */ 077 ONHOLD, 078 /** 079 * The task was attempted but could not be completed due to some error. 080 */ 081 FAILED, 082 /** 083 * The task has been completed. 084 */ 085 COMPLETED, 086 /** 087 * The task should never have existed and is retained only because of the possibility it may have used. 088 */ 089 ENTEREDINERROR, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 public static TaskStatus fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("draft".equals(codeString)) 098 return DRAFT; 099 if ("requested".equals(codeString)) 100 return REQUESTED; 101 if ("received".equals(codeString)) 102 return RECEIVED; 103 if ("accepted".equals(codeString)) 104 return ACCEPTED; 105 if ("rejected".equals(codeString)) 106 return REJECTED; 107 if ("ready".equals(codeString)) 108 return READY; 109 if ("cancelled".equals(codeString)) 110 return CANCELLED; 111 if ("in-progress".equals(codeString)) 112 return INPROGRESS; 113 if ("on-hold".equals(codeString)) 114 return ONHOLD; 115 if ("failed".equals(codeString)) 116 return FAILED; 117 if ("completed".equals(codeString)) 118 return COMPLETED; 119 if ("entered-in-error".equals(codeString)) 120 return ENTEREDINERROR; 121 throw new FHIRException("Unknown TaskStatus code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case DRAFT: return "draft"; 126 case REQUESTED: return "requested"; 127 case RECEIVED: return "received"; 128 case ACCEPTED: return "accepted"; 129 case REJECTED: return "rejected"; 130 case READY: return "ready"; 131 case CANCELLED: return "cancelled"; 132 case INPROGRESS: return "in-progress"; 133 case ONHOLD: return "on-hold"; 134 case FAILED: return "failed"; 135 case COMPLETED: return "completed"; 136 case ENTEREDINERROR: return "entered-in-error"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getSystem() { 142 return "http://hl7.org/fhir/task-status"; 143 } 144 public String getDefinition() { 145 switch (this) { 146 case DRAFT: return "The task is not yet ready to be acted upon."; 147 case REQUESTED: return "The task is ready to be acted upon and action is sought."; 148 case RECEIVED: return "A potential performer has claimed ownership of the task and is evaluating whether to perform it."; 149 case ACCEPTED: return "The potential performer has agreed to execute the task but has not yet started work."; 150 case REJECTED: return "The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action."; 151 case READY: return "Task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given."; 152 case CANCELLED: return "The task was not completed."; 153 case INPROGRESS: return "Task has been started but is not yet complete."; 154 case ONHOLD: return "Task has been started but work has been paused."; 155 case FAILED: return "The task was attempted but could not be completed due to some error."; 156 case COMPLETED: return "The task has been completed."; 157 case ENTEREDINERROR: return "The task should never have existed and is retained only because of the possibility it may have used."; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getDisplay() { 163 switch (this) { 164 case DRAFT: return "Draft"; 165 case REQUESTED: return "Requested"; 166 case RECEIVED: return "Received"; 167 case ACCEPTED: return "Accepted"; 168 case REJECTED: return "Rejected"; 169 case READY: return "Ready"; 170 case CANCELLED: return "Cancelled"; 171 case INPROGRESS: return "In Progress"; 172 case ONHOLD: return "On Hold"; 173 case FAILED: return "Failed"; 174 case COMPLETED: return "Completed"; 175 case ENTEREDINERROR: return "Entered in Error"; 176 case NULL: return null; 177 default: return "?"; 178 } 179 } 180 181 182}