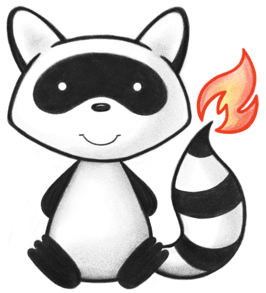
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Teeth { 041 042 /** 043 * Upper Right Tooth 1 from the central axis, permanent dentition. 044 */ 045 _11, 046 /** 047 * Upper Right Tooth 2 from the central axis, permanent dentition. 048 */ 049 _12, 050 /** 051 * Upper Right Tooth 3 from the central axis, permanent dentition. 052 */ 053 _13, 054 /** 055 * Upper Right Tooth 4 from the central axis, permanent dentition. 056 */ 057 _14, 058 /** 059 * Upper Right Tooth 5 from the central axis, permanent dentition. 060 */ 061 _15, 062 /** 063 * Upper Right Tooth 6 from the central axis, permanent dentition. 064 */ 065 _16, 066 /** 067 * Upper Right Tooth 7 from the central axis, permanent dentition. 068 */ 069 _17, 070 /** 071 * Upper Right Tooth 8 from the central axis, permanent dentition. 072 */ 073 _18, 074 /** 075 * Upper Left Tooth 1 from the central axis, permanent dentition. 076 */ 077 _21, 078 /** 079 * Upper Left Tooth 2 from the central axis, permanent dentition. 080 */ 081 _22, 082 /** 083 * Upper Left Tooth 3 from the central axis, permanent dentition. 084 */ 085 _23, 086 /** 087 * Upper Left Tooth 4 from the central axis, permanent dentition. 088 */ 089 _24, 090 /** 091 * Upper Left Tooth 5 from the central axis, permanent dentition. 092 */ 093 _25, 094 /** 095 * Upper Left Tooth 6 from the central axis, permanent dentition. 096 */ 097 _26, 098 /** 099 * Upper Left Tooth 7 from the central axis, permanent dentition. 100 */ 101 _27, 102 /** 103 * Upper Left Tooth 8 from the central axis, permanent dentition. 104 */ 105 _28, 106 /** 107 * Lower Left Tooth 1 from the central axis, permanent dentition. 108 */ 109 _31, 110 /** 111 * Lower Left Tooth 2 from the central axis, permanent dentition. 112 */ 113 _32, 114 /** 115 * Lower Left Tooth 3 from the central axis, permanent dentition. 116 */ 117 _33, 118 /** 119 * Lower Left Tooth 4 from the central axis, permanent dentition. 120 */ 121 _34, 122 /** 123 * Lower Left Tooth 5 from the central axis, permanent dentition. 124 */ 125 _35, 126 /** 127 * Lower Left Tooth 6 from the central axis, permanent dentition. 128 */ 129 _36, 130 /** 131 * Lower Left Tooth 7 from the central axis, permanent dentition. 132 */ 133 _37, 134 /** 135 * Lower Left Tooth 8 from the central axis, permanent dentition. 136 */ 137 _38, 138 /** 139 * Lower Right Tooth 1 from the central axis, permanent dentition. 140 */ 141 _41, 142 /** 143 * Lower Right Tooth 2 from the central axis, permanent dentition. 144 */ 145 _42, 146 /** 147 * Lower Right Tooth 3 from the central axis, permanent dentition. 148 */ 149 _43, 150 /** 151 * Lower Right Tooth 4 from the central axis, permanent dentition. 152 */ 153 _44, 154 /** 155 * Lower Right Tooth 5 from the central axis, permanent dentition. 156 */ 157 _45, 158 /** 159 * Lower Right Tooth 6 from the central axis, permanent dentition. 160 */ 161 _46, 162 /** 163 * Lower Right Tooth 7 from the central axis, permanent dentition. 164 */ 165 _47, 166 /** 167 * Lower Right Tooth 8 from the central axis, permanent dentition. 168 */ 169 _48, 170 /** 171 * added to help the parsers 172 */ 173 NULL; 174 public static Teeth fromCode(String codeString) throws FHIRException { 175 if (codeString == null || "".equals(codeString)) 176 return null; 177 if ("11".equals(codeString)) 178 return _11; 179 if ("12".equals(codeString)) 180 return _12; 181 if ("13".equals(codeString)) 182 return _13; 183 if ("14".equals(codeString)) 184 return _14; 185 if ("15".equals(codeString)) 186 return _15; 187 if ("16".equals(codeString)) 188 return _16; 189 if ("17".equals(codeString)) 190 return _17; 191 if ("18".equals(codeString)) 192 return _18; 193 if ("21".equals(codeString)) 194 return _21; 195 if ("22".equals(codeString)) 196 return _22; 197 if ("23".equals(codeString)) 198 return _23; 199 if ("24".equals(codeString)) 200 return _24; 201 if ("25".equals(codeString)) 202 return _25; 203 if ("26".equals(codeString)) 204 return _26; 205 if ("27".equals(codeString)) 206 return _27; 207 if ("28".equals(codeString)) 208 return _28; 209 if ("31".equals(codeString)) 210 return _31; 211 if ("32".equals(codeString)) 212 return _32; 213 if ("33".equals(codeString)) 214 return _33; 215 if ("34".equals(codeString)) 216 return _34; 217 if ("35".equals(codeString)) 218 return _35; 219 if ("36".equals(codeString)) 220 return _36; 221 if ("37".equals(codeString)) 222 return _37; 223 if ("38".equals(codeString)) 224 return _38; 225 if ("41".equals(codeString)) 226 return _41; 227 if ("42".equals(codeString)) 228 return _42; 229 if ("43".equals(codeString)) 230 return _43; 231 if ("44".equals(codeString)) 232 return _44; 233 if ("45".equals(codeString)) 234 return _45; 235 if ("46".equals(codeString)) 236 return _46; 237 if ("47".equals(codeString)) 238 return _47; 239 if ("48".equals(codeString)) 240 return _48; 241 throw new FHIRException("Unknown Teeth code '"+codeString+"'"); 242 } 243 public String toCode() { 244 switch (this) { 245 case _11: return "11"; 246 case _12: return "12"; 247 case _13: return "13"; 248 case _14: return "14"; 249 case _15: return "15"; 250 case _16: return "16"; 251 case _17: return "17"; 252 case _18: return "18"; 253 case _21: return "21"; 254 case _22: return "22"; 255 case _23: return "23"; 256 case _24: return "24"; 257 case _25: return "25"; 258 case _26: return "26"; 259 case _27: return "27"; 260 case _28: return "28"; 261 case _31: return "31"; 262 case _32: return "32"; 263 case _33: return "33"; 264 case _34: return "34"; 265 case _35: return "35"; 266 case _36: return "36"; 267 case _37: return "37"; 268 case _38: return "38"; 269 case _41: return "41"; 270 case _42: return "42"; 271 case _43: return "43"; 272 case _44: return "44"; 273 case _45: return "45"; 274 case _46: return "46"; 275 case _47: return "47"; 276 case _48: return "48"; 277 case NULL: return null; 278 default: return "?"; 279 } 280 } 281 public String getSystem() { 282 return "http://hl7.org/fhir/ex-fdi"; 283 } 284 public String getDefinition() { 285 switch (this) { 286 case _11: return "Upper Right Tooth 1 from the central axis, permanent dentition."; 287 case _12: return "Upper Right Tooth 2 from the central axis, permanent dentition."; 288 case _13: return "Upper Right Tooth 3 from the central axis, permanent dentition."; 289 case _14: return "Upper Right Tooth 4 from the central axis, permanent dentition."; 290 case _15: return "Upper Right Tooth 5 from the central axis, permanent dentition."; 291 case _16: return "Upper Right Tooth 6 from the central axis, permanent dentition."; 292 case _17: return "Upper Right Tooth 7 from the central axis, permanent dentition."; 293 case _18: return "Upper Right Tooth 8 from the central axis, permanent dentition."; 294 case _21: return "Upper Left Tooth 1 from the central axis, permanent dentition."; 295 case _22: return "Upper Left Tooth 2 from the central axis, permanent dentition."; 296 case _23: return "Upper Left Tooth 3 from the central axis, permanent dentition."; 297 case _24: return "Upper Left Tooth 4 from the central axis, permanent dentition."; 298 case _25: return "Upper Left Tooth 5 from the central axis, permanent dentition."; 299 case _26: return "Upper Left Tooth 6 from the central axis, permanent dentition."; 300 case _27: return "Upper Left Tooth 7 from the central axis, permanent dentition."; 301 case _28: return "Upper Left Tooth 8 from the central axis, permanent dentition."; 302 case _31: return "Lower Left Tooth 1 from the central axis, permanent dentition."; 303 case _32: return "Lower Left Tooth 2 from the central axis, permanent dentition."; 304 case _33: return "Lower Left Tooth 3 from the central axis, permanent dentition."; 305 case _34: return "Lower Left Tooth 4 from the central axis, permanent dentition."; 306 case _35: return "Lower Left Tooth 5 from the central axis, permanent dentition."; 307 case _36: return "Lower Left Tooth 6 from the central axis, permanent dentition."; 308 case _37: return "Lower Left Tooth 7 from the central axis, permanent dentition."; 309 case _38: return "Lower Left Tooth 8 from the central axis, permanent dentition."; 310 case _41: return "Lower Right Tooth 1 from the central axis, permanent dentition."; 311 case _42: return "Lower Right Tooth 2 from the central axis, permanent dentition."; 312 case _43: return "Lower Right Tooth 3 from the central axis, permanent dentition."; 313 case _44: return "Lower Right Tooth 4 from the central axis, permanent dentition."; 314 case _45: return "Lower Right Tooth 5 from the central axis, permanent dentition."; 315 case _46: return "Lower Right Tooth 6 from the central axis, permanent dentition."; 316 case _47: return "Lower Right Tooth 7 from the central axis, permanent dentition."; 317 case _48: return "Lower Right Tooth 8 from the central axis, permanent dentition."; 318 case NULL: return null; 319 default: return "?"; 320 } 321 } 322 public String getDisplay() { 323 switch (this) { 324 case _11: return "11"; 325 case _12: return "12"; 326 case _13: return "13"; 327 case _14: return "14"; 328 case _15: return "15"; 329 case _16: return "16"; 330 case _17: return "17"; 331 case _18: return "18"; 332 case _21: return "21"; 333 case _22: return "22"; 334 case _23: return "23"; 335 case _24: return "24"; 336 case _25: return "25"; 337 case _26: return "26"; 338 case _27: return "27"; 339 case _28: return "28"; 340 case _31: return "31"; 341 case _32: return "32"; 342 case _33: return "33"; 343 case _34: return "34"; 344 case _35: return "35"; 345 case _36: return "36"; 346 case _37: return "37"; 347 case _38: return "38"; 348 case _41: return "41"; 349 case _42: return "42"; 350 case _43: return "43"; 351 case _44: return "44"; 352 case _45: return "45"; 353 case _46: return "46"; 354 case _47: return "47"; 355 case _48: return "48"; 356 case NULL: return null; 357 default: return "?"; 358 } 359 } 360 361 362}