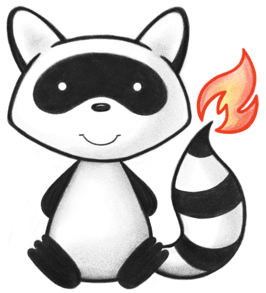
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum TestscriptOperationCodes { 041 042 /** 043 * Read the current state of the resource. 044 */ 045 READ, 046 /** 047 * Read the state of a specific version of the resource. 048 */ 049 VREAD, 050 /** 051 * Update an existing resource by its id. 052 */ 053 UPDATE, 054 /** 055 * Update an existing resource by its id (or create it if it is new). 056 */ 057 UPDATECREATE, 058 /** 059 * Delete a resource. 060 */ 061 DELETE, 062 /** 063 * Conditionally delete a single resource based on search parameters. 064 */ 065 DELETECONDSINGLE, 066 /** 067 * Conditionally delete one or more resources based on search parameters. 068 */ 069 DELETECONDMULTIPLE, 070 /** 071 * Retrieve the change history for a particular resource or resource type. 072 */ 073 HISTORY, 074 /** 075 * Create a new resource with a server assigned id. 076 */ 077 CREATE, 078 /** 079 * Search based on some filter criteria. 080 */ 081 SEARCH, 082 /** 083 * Update, create or delete a set of resources as independent actions. 084 */ 085 BATCH, 086 /** 087 * Update, create or delete a set of resources as a single transaction. 088 */ 089 TRANSACTION, 090 /** 091 * Get a capability statement for the system. 092 */ 093 CAPABILITIES, 094 /** 095 * Realize a definition in a specific context 096 */ 097 APPLY, 098 /** 099 * Cancel Task 100 */ 101 CANCEL, 102 /** 103 * Closure Table Maintenance 104 */ 105 CLOSURE, 106 /** 107 * Code Composition based on supplied properties 108 */ 109 COMPOSE, 110 /** 111 * Test if a server implements a client's required operations 112 */ 113 CONFORMS, 114 /** 115 * Aggregates and return the parameters and data requirements as a single module definition library 116 */ 117 DATAREQUIREMENTS, 118 /** 119 * Generate a Document 120 */ 121 DOCUMENT, 122 /** 123 * Evaluate DecisionSupportRule / DecisionSupportServiceModule 124 */ 125 EVALUATE, 126 /** 127 * Evaluate Measure 128 */ 129 EVALUATEMEASURE, 130 /** 131 * Fetch Encounter/Patient Record 132 */ 133 EVERYTHING, 134 /** 135 * Value Set Expansion 136 */ 137 EXPAND, 138 /** 139 * Fail Task 140 */ 141 FAIL, 142 /** 143 * Find a functional list 144 */ 145 FIND, 146 /** 147 * Finish Task 148 */ 149 FINISH, 150 /** 151 * Test if a server implements a client's required operations 152 */ 153 IMPLEMENTS, 154 /** 155 * Concept Look Up 156 */ 157 LOOKUP, 158 /** 159 * Find patient matches using MPI based logic 160 */ 161 MATCH, 162 /** 163 * Access a list of profiles, tags, and security labels 164 */ 165 META, 166 /** 167 * Add profiles, tags, and security labels to a resource 168 */ 169 METAADD, 170 /** 171 * Delete profiles, tags, and security labels for a resource 172 */ 173 METADELETE, 174 /** 175 * Populate Questionnaire 176 */ 177 POPULATE, 178 /** 179 * Generate HTML for Questionnaire 180 */ 181 POPULATEHTML, 182 /** 183 * Generate a link to a Questionnaire completion webpage 184 */ 185 POPULATELINK, 186 /** 187 * Process Message 188 */ 189 PROCESSMESSAGE, 190 /** 191 * Build Questionnaire 192 */ 193 QUESTIONNAIRE, 194 /** 195 * Release Task 196 */ 197 RELEASE, 198 /** 199 * Reserve Task 200 */ 201 RESERVE, 202 /** 203 * Resume Task 204 */ 205 RESUME, 206 /** 207 * Set Task Input 208 */ 209 SETINPUT, 210 /** 211 * Set Task Output 212 */ 213 SETOUTPUT, 214 /** 215 * Start Task 216 */ 217 START, 218 /** 219 * Observation Statistics 220 */ 221 STATS, 222 /** 223 * Stop Task 224 */ 225 STOP, 226 /** 227 * Fetch a subset of the CapabilityStatement resource 228 */ 229 SUBSET, 230 /** 231 * Determine if code A is subsumed by code B 232 */ 233 SUBSUMES, 234 /** 235 * Suspend Task 236 */ 237 SUSPEND, 238 /** 239 * Model Instance Transformation 240 */ 241 TRANSFORM, 242 /** 243 * Concept Translation 244 */ 245 TRANSLATE, 246 /** 247 * Validate a resource 248 */ 249 VALIDATE, 250 /** 251 * Value Set based Validation 252 */ 253 VALIDATECODE, 254 /** 255 * added to help the parsers 256 */ 257 NULL; 258 public static TestscriptOperationCodes fromCode(String codeString) throws FHIRException { 259 if (codeString == null || "".equals(codeString)) 260 return null; 261 if ("read".equals(codeString)) 262 return READ; 263 if ("vread".equals(codeString)) 264 return VREAD; 265 if ("update".equals(codeString)) 266 return UPDATE; 267 if ("updateCreate".equals(codeString)) 268 return UPDATECREATE; 269 if ("delete".equals(codeString)) 270 return DELETE; 271 if ("deleteCondSingle".equals(codeString)) 272 return DELETECONDSINGLE; 273 if ("deleteCondMultiple".equals(codeString)) 274 return DELETECONDMULTIPLE; 275 if ("history".equals(codeString)) 276 return HISTORY; 277 if ("create".equals(codeString)) 278 return CREATE; 279 if ("search".equals(codeString)) 280 return SEARCH; 281 if ("batch".equals(codeString)) 282 return BATCH; 283 if ("transaction".equals(codeString)) 284 return TRANSACTION; 285 if ("capabilities".equals(codeString)) 286 return CAPABILITIES; 287 if ("apply".equals(codeString)) 288 return APPLY; 289 if ("cancel".equals(codeString)) 290 return CANCEL; 291 if ("closure".equals(codeString)) 292 return CLOSURE; 293 if ("compose".equals(codeString)) 294 return COMPOSE; 295 if ("conforms".equals(codeString)) 296 return CONFORMS; 297 if ("data-requirements".equals(codeString)) 298 return DATAREQUIREMENTS; 299 if ("document".equals(codeString)) 300 return DOCUMENT; 301 if ("evaluate".equals(codeString)) 302 return EVALUATE; 303 if ("evaluate-measure".equals(codeString)) 304 return EVALUATEMEASURE; 305 if ("everything".equals(codeString)) 306 return EVERYTHING; 307 if ("expand".equals(codeString)) 308 return EXPAND; 309 if ("fail".equals(codeString)) 310 return FAIL; 311 if ("find".equals(codeString)) 312 return FIND; 313 if ("finish".equals(codeString)) 314 return FINISH; 315 if ("implements".equals(codeString)) 316 return IMPLEMENTS; 317 if ("lookup".equals(codeString)) 318 return LOOKUP; 319 if ("match".equals(codeString)) 320 return MATCH; 321 if ("meta".equals(codeString)) 322 return META; 323 if ("meta-add".equals(codeString)) 324 return METAADD; 325 if ("meta-delete".equals(codeString)) 326 return METADELETE; 327 if ("populate".equals(codeString)) 328 return POPULATE; 329 if ("populatehtml".equals(codeString)) 330 return POPULATEHTML; 331 if ("populatelink".equals(codeString)) 332 return POPULATELINK; 333 if ("process-message".equals(codeString)) 334 return PROCESSMESSAGE; 335 if ("questionnaire".equals(codeString)) 336 return QUESTIONNAIRE; 337 if ("release".equals(codeString)) 338 return RELEASE; 339 if ("reserve".equals(codeString)) 340 return RESERVE; 341 if ("resume".equals(codeString)) 342 return RESUME; 343 if ("set-input".equals(codeString)) 344 return SETINPUT; 345 if ("set-output".equals(codeString)) 346 return SETOUTPUT; 347 if ("start".equals(codeString)) 348 return START; 349 if ("stats".equals(codeString)) 350 return STATS; 351 if ("stop".equals(codeString)) 352 return STOP; 353 if ("subset".equals(codeString)) 354 return SUBSET; 355 if ("subsumes".equals(codeString)) 356 return SUBSUMES; 357 if ("suspend".equals(codeString)) 358 return SUSPEND; 359 if ("transform".equals(codeString)) 360 return TRANSFORM; 361 if ("translate".equals(codeString)) 362 return TRANSLATE; 363 if ("validate".equals(codeString)) 364 return VALIDATE; 365 if ("validate-code".equals(codeString)) 366 return VALIDATECODE; 367 throw new FHIRException("Unknown TestscriptOperationCodes code '"+codeString+"'"); 368 } 369 public String toCode() { 370 switch (this) { 371 case READ: return "read"; 372 case VREAD: return "vread"; 373 case UPDATE: return "update"; 374 case UPDATECREATE: return "updateCreate"; 375 case DELETE: return "delete"; 376 case DELETECONDSINGLE: return "deleteCondSingle"; 377 case DELETECONDMULTIPLE: return "deleteCondMultiple"; 378 case HISTORY: return "history"; 379 case CREATE: return "create"; 380 case SEARCH: return "search"; 381 case BATCH: return "batch"; 382 case TRANSACTION: return "transaction"; 383 case CAPABILITIES: return "capabilities"; 384 case APPLY: return "apply"; 385 case CANCEL: return "cancel"; 386 case CLOSURE: return "closure"; 387 case COMPOSE: return "compose"; 388 case CONFORMS: return "conforms"; 389 case DATAREQUIREMENTS: return "data-requirements"; 390 case DOCUMENT: return "document"; 391 case EVALUATE: return "evaluate"; 392 case EVALUATEMEASURE: return "evaluate-measure"; 393 case EVERYTHING: return "everything"; 394 case EXPAND: return "expand"; 395 case FAIL: return "fail"; 396 case FIND: return "find"; 397 case FINISH: return "finish"; 398 case IMPLEMENTS: return "implements"; 399 case LOOKUP: return "lookup"; 400 case MATCH: return "match"; 401 case META: return "meta"; 402 case METAADD: return "meta-add"; 403 case METADELETE: return "meta-delete"; 404 case POPULATE: return "populate"; 405 case POPULATEHTML: return "populatehtml"; 406 case POPULATELINK: return "populatelink"; 407 case PROCESSMESSAGE: return "process-message"; 408 case QUESTIONNAIRE: return "questionnaire"; 409 case RELEASE: return "release"; 410 case RESERVE: return "reserve"; 411 case RESUME: return "resume"; 412 case SETINPUT: return "set-input"; 413 case SETOUTPUT: return "set-output"; 414 case START: return "start"; 415 case STATS: return "stats"; 416 case STOP: return "stop"; 417 case SUBSET: return "subset"; 418 case SUBSUMES: return "subsumes"; 419 case SUSPEND: return "suspend"; 420 case TRANSFORM: return "transform"; 421 case TRANSLATE: return "translate"; 422 case VALIDATE: return "validate"; 423 case VALIDATECODE: return "validate-code"; 424 case NULL: return null; 425 default: return "?"; 426 } 427 } 428 public String getSystem() { 429 return "http://hl7.org/fhir/testscript-operation-codes"; 430 } 431 public String getDefinition() { 432 switch (this) { 433 case READ: return "Read the current state of the resource."; 434 case VREAD: return "Read the state of a specific version of the resource."; 435 case UPDATE: return "Update an existing resource by its id."; 436 case UPDATECREATE: return "Update an existing resource by its id (or create it if it is new)."; 437 case DELETE: return "Delete a resource."; 438 case DELETECONDSINGLE: return "Conditionally delete a single resource based on search parameters."; 439 case DELETECONDMULTIPLE: return "Conditionally delete one or more resources based on search parameters."; 440 case HISTORY: return "Retrieve the change history for a particular resource or resource type."; 441 case CREATE: return "Create a new resource with a server assigned id."; 442 case SEARCH: return "Search based on some filter criteria."; 443 case BATCH: return "Update, create or delete a set of resources as independent actions."; 444 case TRANSACTION: return "Update, create or delete a set of resources as a single transaction."; 445 case CAPABILITIES: return "Get a capability statement for the system."; 446 case APPLY: return "Realize a definition in a specific context"; 447 case CANCEL: return "Cancel Task"; 448 case CLOSURE: return "Closure Table Maintenance"; 449 case COMPOSE: return "Code Composition based on supplied properties"; 450 case CONFORMS: return "Test if a server implements a client's required operations"; 451 case DATAREQUIREMENTS: return "Aggregates and return the parameters and data requirements as a single module definition library"; 452 case DOCUMENT: return "Generate a Document"; 453 case EVALUATE: return "Evaluate DecisionSupportRule / DecisionSupportServiceModule"; 454 case EVALUATEMEASURE: return "Evaluate Measure"; 455 case EVERYTHING: return "Fetch Encounter/Patient Record"; 456 case EXPAND: return "Value Set Expansion"; 457 case FAIL: return "Fail Task"; 458 case FIND: return "Find a functional list"; 459 case FINISH: return "Finish Task"; 460 case IMPLEMENTS: return "Test if a server implements a client's required operations"; 461 case LOOKUP: return "Concept Look Up"; 462 case MATCH: return "Find patient matches using MPI based logic"; 463 case META: return "Access a list of profiles, tags, and security labels"; 464 case METAADD: return "Add profiles, tags, and security labels to a resource"; 465 case METADELETE: return "Delete profiles, tags, and security labels for a resource"; 466 case POPULATE: return "Populate Questionnaire"; 467 case POPULATEHTML: return "Generate HTML for Questionnaire"; 468 case POPULATELINK: return "Generate a link to a Questionnaire completion webpage"; 469 case PROCESSMESSAGE: return "Process Message"; 470 case QUESTIONNAIRE: return "Build Questionnaire"; 471 case RELEASE: return "Release Task"; 472 case RESERVE: return "Reserve Task"; 473 case RESUME: return "Resume Task"; 474 case SETINPUT: return "Set Task Input"; 475 case SETOUTPUT: return "Set Task Output"; 476 case START: return "Start Task"; 477 case STATS: return "Observation Statistics"; 478 case STOP: return "Stop Task"; 479 case SUBSET: return "Fetch a subset of the CapabilityStatement resource"; 480 case SUBSUMES: return "Determine if code A is subsumed by code B"; 481 case SUSPEND: return "Suspend Task"; 482 case TRANSFORM: return "Model Instance Transformation"; 483 case TRANSLATE: return "Concept Translation"; 484 case VALIDATE: return "Validate a resource"; 485 case VALIDATECODE: return "Value Set based Validation"; 486 case NULL: return null; 487 default: return "?"; 488 } 489 } 490 public String getDisplay() { 491 switch (this) { 492 case READ: return "Read"; 493 case VREAD: return "Version Read"; 494 case UPDATE: return "Update"; 495 case UPDATECREATE: return "Create using Update"; 496 case DELETE: return "Delete"; 497 case DELETECONDSINGLE: return "Conditional Delete Single"; 498 case DELETECONDMULTIPLE: return "Conditional Delete Multiple"; 499 case HISTORY: return "History"; 500 case CREATE: return "Create"; 501 case SEARCH: return "Search"; 502 case BATCH: return "Batch"; 503 case TRANSACTION: return "Transaction"; 504 case CAPABILITIES: return "Capabilities"; 505 case APPLY: return "$apply"; 506 case CANCEL: return "$cancel"; 507 case CLOSURE: return "$closure"; 508 case COMPOSE: return "$compose"; 509 case CONFORMS: return "$conforms"; 510 case DATAREQUIREMENTS: return "$data-requirements"; 511 case DOCUMENT: return "$document"; 512 case EVALUATE: return "$evaluate"; 513 case EVALUATEMEASURE: return "$evaluate-measure"; 514 case EVERYTHING: return "$everything"; 515 case EXPAND: return "$expand"; 516 case FAIL: return "$fail"; 517 case FIND: return "$find"; 518 case FINISH: return "$finish"; 519 case IMPLEMENTS: return "$implements"; 520 case LOOKUP: return "$lookup"; 521 case MATCH: return "$match"; 522 case META: return "$meta"; 523 case METAADD: return "$meta-add"; 524 case METADELETE: return "$meta-delete"; 525 case POPULATE: return "$populate"; 526 case POPULATEHTML: return "$populatehtml"; 527 case POPULATELINK: return "$populatelink"; 528 case PROCESSMESSAGE: return "$process-message"; 529 case QUESTIONNAIRE: return "$questionnaire"; 530 case RELEASE: return "$release"; 531 case RESERVE: return "$reserve"; 532 case RESUME: return "$resume"; 533 case SETINPUT: return "$set-input"; 534 case SETOUTPUT: return "$set-output"; 535 case START: return "$start"; 536 case STATS: return "$stats"; 537 case STOP: return "$stop"; 538 case SUBSET: return "$subset"; 539 case SUBSUMES: return "$subsumes"; 540 case SUSPEND: return "$suspend"; 541 case TRANSFORM: return "$transform"; 542 case TRANSLATE: return "$translate"; 543 case VALIDATE: return "$validate"; 544 case VALIDATECODE: return "$validate-code"; 545 case NULL: return null; 546 default: return "?"; 547 } 548 } 549 550 551}