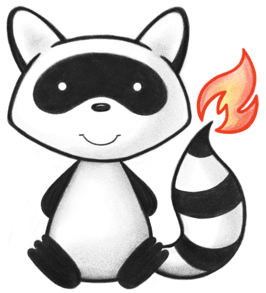
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum Tooth { 041 042 /** 043 * Oral cavity. 044 */ 045 _0, 046 /** 047 * Permanent teeth Maxillary right. 048 */ 049 _1, 050 /** 051 * Permanent teeth Maxillary left. 052 */ 053 _2, 054 /** 055 * Permanent teeth Mandibular right. 056 */ 057 _3, 058 /** 059 * Permanent teeth Mandibular left. 060 */ 061 _4, 062 /** 063 * Deciduous teeth Maxillary right. 064 */ 065 _5, 066 /** 067 * Deciduous teeth Maxillary left. 068 */ 069 _6, 070 /** 071 * Deciduous teeth Mandibular right. 072 */ 073 _7, 074 /** 075 * Deciduous teeth Mandibular left. 076 */ 077 _8, 078 /** 079 * Upper Right Tooth 1 from the central axis, permanent dentition. 080 */ 081 _11, 082 /** 083 * Upper Right Tooth 2 from the central axis, permanent dentition. 084 */ 085 _12, 086 /** 087 * Upper Right Tooth 3 from the central axis, permanent dentition. 088 */ 089 _13, 090 /** 091 * Upper Right Tooth 4 from the central axis, permanent dentition. 092 */ 093 _14, 094 /** 095 * Upper Right Tooth 5 from the central axis, permanent dentition. 096 */ 097 _15, 098 /** 099 * Upper Right Tooth 6 from the central axis, permanent dentition. 100 */ 101 _16, 102 /** 103 * Upper Right Tooth 7 from the central axis, permanent dentition. 104 */ 105 _17, 106 /** 107 * Upper Right Tooth 8 from the central axis, permanent dentition. 108 */ 109 _18, 110 /** 111 * Upper Left Tooth 1 from the central axis, permanent dentition. 112 */ 113 _21, 114 /** 115 * Upper Left Tooth 2 from the central axis, permanent dentition. 116 */ 117 _22, 118 /** 119 * Upper Left Tooth 3 from the central axis, permanent dentition. 120 */ 121 _23, 122 /** 123 * Upper Left Tooth 4 from the central axis, permanent dentition. 124 */ 125 _24, 126 /** 127 * Upper Left Tooth 5 from the central axis, permanent dentition. 128 */ 129 _25, 130 /** 131 * Upper Left Tooth 6 from the central axis, permanent dentition. 132 */ 133 _26, 134 /** 135 * Upper Left Tooth 7 from the central axis, permanent dentition. 136 */ 137 _27, 138 /** 139 * Upper Left Tooth 8 from the central axis, permanent dentition. 140 */ 141 _28, 142 /** 143 * Lower Left Tooth 1 from the central axis, permanent dentition. 144 */ 145 _31, 146 /** 147 * Lower Left Tooth 2 from the central axis, permanent dentition. 148 */ 149 _32, 150 /** 151 * Lower Left Tooth 3 from the central axis, permanent dentition. 152 */ 153 _33, 154 /** 155 * Lower Left Tooth 4 from the central axis, permanent dentition. 156 */ 157 _34, 158 /** 159 * Lower Left Tooth 5 from the central axis, permanent dentition. 160 */ 161 _35, 162 /** 163 * Lower Left Tooth 6 from the central axis, permanent dentition. 164 */ 165 _36, 166 /** 167 * Lower Left Tooth 7 from the central axis, permanent dentition. 168 */ 169 _37, 170 /** 171 * Lower Left Tooth 8 from the central axis, permanent dentition. 172 */ 173 _38, 174 /** 175 * Lower Right Tooth 1 from the central axis, permanent dentition. 176 */ 177 _41, 178 /** 179 * Lower Right Tooth 2 from the central axis, permanent dentition. 180 */ 181 _42, 182 /** 183 * Lower Right Tooth 3 from the central axis, permanent dentition. 184 */ 185 _43, 186 /** 187 * Lower Right Tooth 4 from the central axis, permanent dentition. 188 */ 189 _44, 190 /** 191 * Lower Right Tooth 5 from the central axis, permanent dentition. 192 */ 193 _45, 194 /** 195 * Lower Right Tooth 6 from the central axis, permanent dentition. 196 */ 197 _46, 198 /** 199 * Lower Right Tooth 7 from the central axis, permanent dentition. 200 */ 201 _47, 202 /** 203 * Lower Right Tooth 8 from the central axis, permanent dentition. 204 */ 205 _48, 206 /** 207 * added to help the parsers 208 */ 209 NULL; 210 public static Tooth fromCode(String codeString) throws FHIRException { 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("0".equals(codeString)) 214 return _0; 215 if ("1".equals(codeString)) 216 return _1; 217 if ("2".equals(codeString)) 218 return _2; 219 if ("3".equals(codeString)) 220 return _3; 221 if ("4".equals(codeString)) 222 return _4; 223 if ("5".equals(codeString)) 224 return _5; 225 if ("6".equals(codeString)) 226 return _6; 227 if ("7".equals(codeString)) 228 return _7; 229 if ("8".equals(codeString)) 230 return _8; 231 if ("11".equals(codeString)) 232 return _11; 233 if ("12".equals(codeString)) 234 return _12; 235 if ("13".equals(codeString)) 236 return _13; 237 if ("14".equals(codeString)) 238 return _14; 239 if ("15".equals(codeString)) 240 return _15; 241 if ("16".equals(codeString)) 242 return _16; 243 if ("17".equals(codeString)) 244 return _17; 245 if ("18".equals(codeString)) 246 return _18; 247 if ("21".equals(codeString)) 248 return _21; 249 if ("22".equals(codeString)) 250 return _22; 251 if ("23".equals(codeString)) 252 return _23; 253 if ("24".equals(codeString)) 254 return _24; 255 if ("25".equals(codeString)) 256 return _25; 257 if ("26".equals(codeString)) 258 return _26; 259 if ("27".equals(codeString)) 260 return _27; 261 if ("28".equals(codeString)) 262 return _28; 263 if ("31".equals(codeString)) 264 return _31; 265 if ("32".equals(codeString)) 266 return _32; 267 if ("33".equals(codeString)) 268 return _33; 269 if ("34".equals(codeString)) 270 return _34; 271 if ("35".equals(codeString)) 272 return _35; 273 if ("36".equals(codeString)) 274 return _36; 275 if ("37".equals(codeString)) 276 return _37; 277 if ("38".equals(codeString)) 278 return _38; 279 if ("41".equals(codeString)) 280 return _41; 281 if ("42".equals(codeString)) 282 return _42; 283 if ("43".equals(codeString)) 284 return _43; 285 if ("44".equals(codeString)) 286 return _44; 287 if ("45".equals(codeString)) 288 return _45; 289 if ("46".equals(codeString)) 290 return _46; 291 if ("47".equals(codeString)) 292 return _47; 293 if ("48".equals(codeString)) 294 return _48; 295 throw new FHIRException("Unknown Tooth code '"+codeString+"'"); 296 } 297 public String toCode() { 298 switch (this) { 299 case _0: return "0"; 300 case _1: return "1"; 301 case _2: return "2"; 302 case _3: return "3"; 303 case _4: return "4"; 304 case _5: return "5"; 305 case _6: return "6"; 306 case _7: return "7"; 307 case _8: return "8"; 308 case _11: return "11"; 309 case _12: return "12"; 310 case _13: return "13"; 311 case _14: return "14"; 312 case _15: return "15"; 313 case _16: return "16"; 314 case _17: return "17"; 315 case _18: return "18"; 316 case _21: return "21"; 317 case _22: return "22"; 318 case _23: return "23"; 319 case _24: return "24"; 320 case _25: return "25"; 321 case _26: return "26"; 322 case _27: return "27"; 323 case _28: return "28"; 324 case _31: return "31"; 325 case _32: return "32"; 326 case _33: return "33"; 327 case _34: return "34"; 328 case _35: return "35"; 329 case _36: return "36"; 330 case _37: return "37"; 331 case _38: return "38"; 332 case _41: return "41"; 333 case _42: return "42"; 334 case _43: return "43"; 335 case _44: return "44"; 336 case _45: return "45"; 337 case _46: return "46"; 338 case _47: return "47"; 339 case _48: return "48"; 340 case NULL: return null; 341 default: return "?"; 342 } 343 } 344 public String getSystem() { 345 return "http://hl7.org/fhir/ex-tooth"; 346 } 347 public String getDefinition() { 348 switch (this) { 349 case _0: return "Oral cavity."; 350 case _1: return "Permanent teeth Maxillary right."; 351 case _2: return "Permanent teeth Maxillary left."; 352 case _3: return "Permanent teeth Mandibular right."; 353 case _4: return "Permanent teeth Mandibular left."; 354 case _5: return "Deciduous teeth Maxillary right."; 355 case _6: return "Deciduous teeth Maxillary left."; 356 case _7: return "Deciduous teeth Mandibular right."; 357 case _8: return "Deciduous teeth Mandibular left."; 358 case _11: return "Upper Right Tooth 1 from the central axis, permanent dentition."; 359 case _12: return "Upper Right Tooth 2 from the central axis, permanent dentition."; 360 case _13: return "Upper Right Tooth 3 from the central axis, permanent dentition."; 361 case _14: return "Upper Right Tooth 4 from the central axis, permanent dentition."; 362 case _15: return "Upper Right Tooth 5 from the central axis, permanent dentition."; 363 case _16: return "Upper Right Tooth 6 from the central axis, permanent dentition."; 364 case _17: return "Upper Right Tooth 7 from the central axis, permanent dentition."; 365 case _18: return "Upper Right Tooth 8 from the central axis, permanent dentition."; 366 case _21: return "Upper Left Tooth 1 from the central axis, permanent dentition."; 367 case _22: return "Upper Left Tooth 2 from the central axis, permanent dentition."; 368 case _23: return "Upper Left Tooth 3 from the central axis, permanent dentition."; 369 case _24: return "Upper Left Tooth 4 from the central axis, permanent dentition."; 370 case _25: return "Upper Left Tooth 5 from the central axis, permanent dentition."; 371 case _26: return "Upper Left Tooth 6 from the central axis, permanent dentition."; 372 case _27: return "Upper Left Tooth 7 from the central axis, permanent dentition."; 373 case _28: return "Upper Left Tooth 8 from the central axis, permanent dentition."; 374 case _31: return "Lower Left Tooth 1 from the central axis, permanent dentition."; 375 case _32: return "Lower Left Tooth 2 from the central axis, permanent dentition."; 376 case _33: return "Lower Left Tooth 3 from the central axis, permanent dentition."; 377 case _34: return "Lower Left Tooth 4 from the central axis, permanent dentition."; 378 case _35: return "Lower Left Tooth 5 from the central axis, permanent dentition."; 379 case _36: return "Lower Left Tooth 6 from the central axis, permanent dentition."; 380 case _37: return "Lower Left Tooth 7 from the central axis, permanent dentition."; 381 case _38: return "Lower Left Tooth 8 from the central axis, permanent dentition."; 382 case _41: return "Lower Right Tooth 1 from the central axis, permanent dentition."; 383 case _42: return "Lower Right Tooth 2 from the central axis, permanent dentition."; 384 case _43: return "Lower Right Tooth 3 from the central axis, permanent dentition."; 385 case _44: return "Lower Right Tooth 4 from the central axis, permanent dentition."; 386 case _45: return "Lower Right Tooth 5 from the central axis, permanent dentition."; 387 case _46: return "Lower Right Tooth 6 from the central axis, permanent dentition."; 388 case _47: return "Lower Right Tooth 7 from the central axis, permanent dentition."; 389 case _48: return "Lower Right Tooth 8 from the central axis, permanent dentition."; 390 case NULL: return null; 391 default: return "?"; 392 } 393 } 394 public String getDisplay() { 395 switch (this) { 396 case _0: return "Oral cavity"; 397 case _1: return "1"; 398 case _2: return "2"; 399 case _3: return "3"; 400 case _4: return "4"; 401 case _5: return "5"; 402 case _6: return "6"; 403 case _7: return "7"; 404 case _8: return "8"; 405 case _11: return "11"; 406 case _12: return "12"; 407 case _13: return "13"; 408 case _14: return "14"; 409 case _15: return "15"; 410 case _16: return "16"; 411 case _17: return "17"; 412 case _18: return "18"; 413 case _21: return "21"; 414 case _22: return "22"; 415 case _23: return "23"; 416 case _24: return "24"; 417 case _25: return "25"; 418 case _26: return "26"; 419 case _27: return "27"; 420 case _28: return "28"; 421 case _31: return "31"; 422 case _32: return "32"; 423 case _33: return "33"; 424 case _34: return "34"; 425 case _35: return "35"; 426 case _36: return "36"; 427 case _37: return "37"; 428 case _38: return "38"; 429 case _41: return "41"; 430 case _42: return "42"; 431 case _43: return "43"; 432 case _44: return "44"; 433 case _45: return "45"; 434 case _46: return "46"; 435 case _47: return "47"; 436 case _48: return "48"; 437 case NULL: return null; 438 default: return "?"; 439 } 440 } 441 442 443}