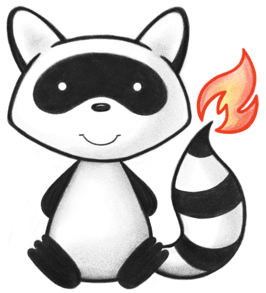
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3AcknowledgementDetailCode { 041 042 /** 043 * Refelects rejections because elements of the communication are not supported in the current context. 044 */ 045 _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE, 046 /** 047 * The interaction (or: this version of the interaction) is not supported. 048 */ 049 NS200, 050 /** 051 * The Processing ID is not supported. 052 */ 053 NS202, 054 /** 055 * The Version ID is not supported. 056 */ 057 NS203, 058 /** 059 * The processing mode is not supported. 060 */ 061 NS250, 062 /** 063 * The Device.id of the sender is unknown. 064 */ 065 NS260, 066 /** 067 * The receiver requires information in the attentionLine classes for routing purposes. 068 */ 069 NS261, 070 /** 071 * An internal software component (database, application, queue mechanism, etc.) has failed, leading to inability to process the message. 072 */ 073 INTERR, 074 /** 075 * Rejection: The message can't be stored by the receiver due to an unspecified internal application issue. The message was neither processed nor stored by the receiving application. 076 */ 077 NOSTORE, 078 /** 079 * Error: The destination of this message is known to the receiving application. Messages have been successfully routed to that destination in the past. The link to the destination application or an intermediate application is unavailable. 080 */ 081 RTEDEST, 082 /** 083 * The destination of this message is unknown to the receiving application. The receiving application in the message does not match the application which received the message. The message was neither routed, processed nor stored by the receiving application. 084 */ 085 RTUDEST, 086 /** 087 * Warning: The destination of this message is known to the receiving application. Messages have been successfully routed to that destination in the past. The link to the destination application or an intermediate application is (temporarily) unavailable. The receiving application will forward the message as soon as the destination can be reached again. 088 */ 089 RTWDEST, 090 /** 091 * Reflects errors in the syntax or structure of the communication. 092 */ 093 SYN, 094 /** 095 * The attribute contained data of the wrong data type, e.g. a numeric attribute contained "FOO". 096 */ 097 SYN102, 098 /** 099 * Description: Required association or attribute missing in message; or the sequence of the classes is different than required by the standard or one of the conformance profiles identified in the message. 100 */ 101 SYN105, 102 /** 103 * Required association missing in message; or the sequence of the classes is different than required by the standard or one of the conformance profiles identified in the message. 104 */ 105 SYN100, 106 /** 107 * A required attribute is missing in a class. 108 */ 109 SYN101, 110 /** 111 * Description: The number of repetitions of a group of association or attributes is less than the required minimum for the standard or of one of the conformance profiles or templates identified in the message. 112 */ 113 SYN114, 114 /** 115 * Description: A coded attribute or datatype property violates one of the terminology constraints specified in the standard or one of the conformance profiles or templates declared by the instance. 116 */ 117 SYN106, 118 /** 119 * An attribute value was compared against the corresponding code system, and no match was found. 120 */ 121 SYN103, 122 /** 123 * An attribute value referenced a code system that is not valid for an attribute constrained to CNE. 124 */ 125 SYN104, 126 /** 127 * Description: A coded attribute is referencing a code that has been deprecated by the owning code system. 128 */ 129 SYN107, 130 /** 131 * Description: The number of repetitions of a (group of) association(s) or attribute(s) exceeds the limits of the standard or of one of the conformance profiles or templates identified in the message. 132 */ 133 SYN108, 134 /** 135 * The number of repetitions of a (group of) association(s) exceeds the limits of the standard or of one of the conformance profiles identified in the message. 136 */ 137 SYN110, 138 /** 139 * The number of repetitions of an attribute exceeds the limits of the standard or of one of the conformance profiles identified in the message. 140 */ 141 SYN112, 142 /** 143 * Description: An attribute or association identified as mandatory in a specification or declared conformance profile or template has been specified with a null flavor. 144 */ 145 SYN109, 146 /** 147 * Description: The value of an attribute or property differs from the fixed value asserted in the standard or one of the conformance profiles or templates declared in the message. 148 */ 149 SYN111, 150 /** 151 * Description: A formal constraint asserted in the standard or one of the conformance profiles or templates declared in the message has been violated. 152 */ 153 SYN113, 154 /** 155 * added to help the parsers 156 */ 157 NULL; 158 public static V3AcknowledgementDetailCode fromCode(String codeString) throws FHIRException { 159 if (codeString == null || "".equals(codeString)) 160 return null; 161 if ("_AcknowledgementDetailNotSupportedCode".equals(codeString)) 162 return _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE; 163 if ("NS200".equals(codeString)) 164 return NS200; 165 if ("NS202".equals(codeString)) 166 return NS202; 167 if ("NS203".equals(codeString)) 168 return NS203; 169 if ("NS250".equals(codeString)) 170 return NS250; 171 if ("NS260".equals(codeString)) 172 return NS260; 173 if ("NS261".equals(codeString)) 174 return NS261; 175 if ("INTERR".equals(codeString)) 176 return INTERR; 177 if ("NOSTORE".equals(codeString)) 178 return NOSTORE; 179 if ("RTEDEST".equals(codeString)) 180 return RTEDEST; 181 if ("RTUDEST".equals(codeString)) 182 return RTUDEST; 183 if ("RTWDEST".equals(codeString)) 184 return RTWDEST; 185 if ("SYN".equals(codeString)) 186 return SYN; 187 if ("SYN102".equals(codeString)) 188 return SYN102; 189 if ("SYN105".equals(codeString)) 190 return SYN105; 191 if ("SYN100".equals(codeString)) 192 return SYN100; 193 if ("SYN101".equals(codeString)) 194 return SYN101; 195 if ("SYN114".equals(codeString)) 196 return SYN114; 197 if ("SYN106".equals(codeString)) 198 return SYN106; 199 if ("SYN103".equals(codeString)) 200 return SYN103; 201 if ("SYN104".equals(codeString)) 202 return SYN104; 203 if ("SYN107".equals(codeString)) 204 return SYN107; 205 if ("SYN108".equals(codeString)) 206 return SYN108; 207 if ("SYN110".equals(codeString)) 208 return SYN110; 209 if ("SYN112".equals(codeString)) 210 return SYN112; 211 if ("SYN109".equals(codeString)) 212 return SYN109; 213 if ("SYN111".equals(codeString)) 214 return SYN111; 215 if ("SYN113".equals(codeString)) 216 return SYN113; 217 throw new FHIRException("Unknown V3AcknowledgementDetailCode code '"+codeString+"'"); 218 } 219 public String toCode() { 220 switch (this) { 221 case _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE: return "_AcknowledgementDetailNotSupportedCode"; 222 case NS200: return "NS200"; 223 case NS202: return "NS202"; 224 case NS203: return "NS203"; 225 case NS250: return "NS250"; 226 case NS260: return "NS260"; 227 case NS261: return "NS261"; 228 case INTERR: return "INTERR"; 229 case NOSTORE: return "NOSTORE"; 230 case RTEDEST: return "RTEDEST"; 231 case RTUDEST: return "RTUDEST"; 232 case RTWDEST: return "RTWDEST"; 233 case SYN: return "SYN"; 234 case SYN102: return "SYN102"; 235 case SYN105: return "SYN105"; 236 case SYN100: return "SYN100"; 237 case SYN101: return "SYN101"; 238 case SYN114: return "SYN114"; 239 case SYN106: return "SYN106"; 240 case SYN103: return "SYN103"; 241 case SYN104: return "SYN104"; 242 case SYN107: return "SYN107"; 243 case SYN108: return "SYN108"; 244 case SYN110: return "SYN110"; 245 case SYN112: return "SYN112"; 246 case SYN109: return "SYN109"; 247 case SYN111: return "SYN111"; 248 case SYN113: return "SYN113"; 249 case NULL: return null; 250 default: return "?"; 251 } 252 } 253 public String getSystem() { 254 return "http://hl7.org/fhir/v3/AcknowledgementDetailCode"; 255 } 256 public String getDefinition() { 257 switch (this) { 258 case _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE: return "Refelects rejections because elements of the communication are not supported in the current context."; 259 case NS200: return "The interaction (or: this version of the interaction) is not supported."; 260 case NS202: return "The Processing ID is not supported."; 261 case NS203: return "The Version ID is not supported."; 262 case NS250: return "The processing mode is not supported."; 263 case NS260: return "The Device.id of the sender is unknown."; 264 case NS261: return "The receiver requires information in the attentionLine classes for routing purposes."; 265 case INTERR: return "An internal software component (database, application, queue mechanism, etc.) has failed, leading to inability to process the message."; 266 case NOSTORE: return "Rejection: The message can't be stored by the receiver due to an unspecified internal application issue. The message was neither processed nor stored by the receiving application."; 267 case RTEDEST: return "Error: The destination of this message is known to the receiving application. Messages have been successfully routed to that destination in the past. The link to the destination application or an intermediate application is unavailable."; 268 case RTUDEST: return "The destination of this message is unknown to the receiving application. The receiving application in the message does not match the application which received the message. The message was neither routed, processed nor stored by the receiving application."; 269 case RTWDEST: return "Warning: The destination of this message is known to the receiving application. Messages have been successfully routed to that destination in the past. The link to the destination application or an intermediate application is (temporarily) unavailable. The receiving application will forward the message as soon as the destination can be reached again."; 270 case SYN: return "Reflects errors in the syntax or structure of the communication."; 271 case SYN102: return "The attribute contained data of the wrong data type, e.g. a numeric attribute contained \"FOO\"."; 272 case SYN105: return "Description: Required association or attribute missing in message; or the sequence of the classes is different than required by the standard or one of the conformance profiles identified in the message."; 273 case SYN100: return "Required association missing in message; or the sequence of the classes is different than required by the standard or one of the conformance profiles identified in the message."; 274 case SYN101: return "A required attribute is missing in a class."; 275 case SYN114: return "Description: The number of repetitions of a group of association or attributes is less than the required minimum for the standard or of one of the conformance profiles or templates identified in the message."; 276 case SYN106: return "Description: A coded attribute or datatype property violates one of the terminology constraints specified in the standard or one of the conformance profiles or templates declared by the instance."; 277 case SYN103: return "An attribute value was compared against the corresponding code system, and no match was found."; 278 case SYN104: return "An attribute value referenced a code system that is not valid for an attribute constrained to CNE."; 279 case SYN107: return "Description: A coded attribute is referencing a code that has been deprecated by the owning code system."; 280 case SYN108: return "Description: The number of repetitions of a (group of) association(s) or attribute(s) exceeds the limits of the standard or of one of the conformance profiles or templates identified in the message."; 281 case SYN110: return "The number of repetitions of a (group of) association(s) exceeds the limits of the standard or of one of the conformance profiles identified in the message."; 282 case SYN112: return "The number of repetitions of an attribute exceeds the limits of the standard or of one of the conformance profiles identified in the message."; 283 case SYN109: return "Description: An attribute or association identified as mandatory in a specification or declared conformance profile or template has been specified with a null flavor."; 284 case SYN111: return "Description: The value of an attribute or property differs from the fixed value asserted in the standard or one of the conformance profiles or templates declared in the message."; 285 case SYN113: return "Description: A formal constraint asserted in the standard or one of the conformance profiles or templates declared in the message has been violated."; 286 case NULL: return null; 287 default: return "?"; 288 } 289 } 290 public String getDisplay() { 291 switch (this) { 292 case _ACKNOWLEDGEMENTDETAILNOTSUPPORTEDCODE: return "AcknowledgementDetailNotSupportedCode"; 293 case NS200: return "Unsupported interaction"; 294 case NS202: return "Unsupported processing id"; 295 case NS203: return "Unsupported version id"; 296 case NS250: return "Unsupported processing Mode"; 297 case NS260: return "Unknown sender"; 298 case NS261: return "Unrecognized attentionline"; 299 case INTERR: return "Internal system error"; 300 case NOSTORE: return "No storage space for message."; 301 case RTEDEST: return "Message routing error, destination unreachable."; 302 case RTUDEST: return "Error: Message routing error, unknown destination."; 303 case RTWDEST: return "Message routing warning, destination unreachable."; 304 case SYN: return "Syntax error"; 305 case SYN102: return "Data type error"; 306 case SYN105: return "Required element missing"; 307 case SYN100: return "Required association missing"; 308 case SYN101: return "Required attribute missing"; 309 case SYN114: return "Insufficient repetitions"; 310 case SYN106: return "Terminology error"; 311 case SYN103: return "Value not found in code system"; 312 case SYN104: return "Invalid code system in CNE"; 313 case SYN107: return "Deprecated code"; 314 case SYN108: return "Number of repetitions exceeds limit"; 315 case SYN110: return "Number of association repetitions exceeds limit"; 316 case SYN112: return "Number of attribute repetitions exceeds limit"; 317 case SYN109: return "Mandatory element with null value"; 318 case SYN111: return "Value does not match fixed value"; 319 case SYN113: return "Formal constraint violation"; 320 case NULL: return null; 321 default: return "?"; 322 } 323 } 324 325 326}