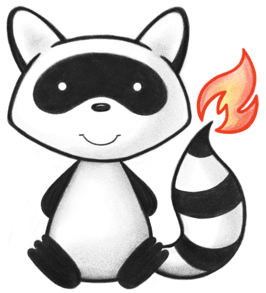
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3ActPriority { 041 042 /** 043 * As soon as possible, next highest priority after stat. 044 */ 045 A, 046 /** 047 * Filler should contact the placer as soon as results are available, even for preliminary results. (Was "C" in HL7 version 2.3's reporting priority.) 048 */ 049 CR, 050 /** 051 * Filler should contact the placer (or target) to schedule the service. (Was "C" in HL7 version 2.3's TQ-priority component.) 052 */ 053 CS, 054 /** 055 * Filler should contact the placer to schedule the service. (Was "C" in HL7 version 2.3's TQ-priority component.) 056 */ 057 CSP, 058 /** 059 * Filler should contact the service recipient (target) to schedule the service. (Was "C" in HL7 version 2.3's TQ-priority component.) 060 */ 061 CSR, 062 /** 063 * Beneficial to the patient but not essential for survival. 064 */ 065 EL, 066 /** 067 * An unforeseen combination of circumstances or the resulting state that calls for immediate action. 068 */ 069 EM, 070 /** 071 * Used to indicate that a service is to be performed prior to a scheduled surgery. When ordering a service and using the pre-op priority, a check is done to see the amount of time that must be allowed for performance of the service. When the order is placed, a message can be generated indicating the time needed for the service so that it is not ordered in conflict with a scheduled operation. 072 */ 073 P, 074 /** 075 * An "as needed" order should be accompanied by a description of what constitutes a need. This description is represented by an observation service predicate as a precondition. 076 */ 077 PRN, 078 /** 079 * Routine service, do at usual work hours. 080 */ 081 R, 082 /** 083 * A report should be prepared and sent as quickly as possible. 084 */ 085 RR, 086 /** 087 * With highest priority (e.g., emergency). 088 */ 089 S, 090 /** 091 * It is critical to come as close as possible to the requested time (e.g., for a through antimicrobial level). 092 */ 093 T, 094 /** 095 * Drug is to be used as directed by the prescriber. 096 */ 097 UD, 098 /** 099 * Calls for prompt action. 100 */ 101 UR, 102 /** 103 * added to help the parsers 104 */ 105 NULL; 106 public static V3ActPriority fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("A".equals(codeString)) 110 return A; 111 if ("CR".equals(codeString)) 112 return CR; 113 if ("CS".equals(codeString)) 114 return CS; 115 if ("CSP".equals(codeString)) 116 return CSP; 117 if ("CSR".equals(codeString)) 118 return CSR; 119 if ("EL".equals(codeString)) 120 return EL; 121 if ("EM".equals(codeString)) 122 return EM; 123 if ("P".equals(codeString)) 124 return P; 125 if ("PRN".equals(codeString)) 126 return PRN; 127 if ("R".equals(codeString)) 128 return R; 129 if ("RR".equals(codeString)) 130 return RR; 131 if ("S".equals(codeString)) 132 return S; 133 if ("T".equals(codeString)) 134 return T; 135 if ("UD".equals(codeString)) 136 return UD; 137 if ("UR".equals(codeString)) 138 return UR; 139 throw new FHIRException("Unknown V3ActPriority code '"+codeString+"'"); 140 } 141 public String toCode() { 142 switch (this) { 143 case A: return "A"; 144 case CR: return "CR"; 145 case CS: return "CS"; 146 case CSP: return "CSP"; 147 case CSR: return "CSR"; 148 case EL: return "EL"; 149 case EM: return "EM"; 150 case P: return "P"; 151 case PRN: return "PRN"; 152 case R: return "R"; 153 case RR: return "RR"; 154 case S: return "S"; 155 case T: return "T"; 156 case UD: return "UD"; 157 case UR: return "UR"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getSystem() { 163 return "http://hl7.org/fhir/v3/ActPriority"; 164 } 165 public String getDefinition() { 166 switch (this) { 167 case A: return "As soon as possible, next highest priority after stat."; 168 case CR: return "Filler should contact the placer as soon as results are available, even for preliminary results. (Was \"C\" in HL7 version 2.3's reporting priority.)"; 169 case CS: return "Filler should contact the placer (or target) to schedule the service. (Was \"C\" in HL7 version 2.3's TQ-priority component.)"; 170 case CSP: return "Filler should contact the placer to schedule the service. (Was \"C\" in HL7 version 2.3's TQ-priority component.)"; 171 case CSR: return "Filler should contact the service recipient (target) to schedule the service. (Was \"C\" in HL7 version 2.3's TQ-priority component.)"; 172 case EL: return "Beneficial to the patient but not essential for survival."; 173 case EM: return "An unforeseen combination of circumstances or the resulting state that calls for immediate action."; 174 case P: return "Used to indicate that a service is to be performed prior to a scheduled surgery. When ordering a service and using the pre-op priority, a check is done to see the amount of time that must be allowed for performance of the service. When the order is placed, a message can be generated indicating the time needed for the service so that it is not ordered in conflict with a scheduled operation."; 175 case PRN: return "An \"as needed\" order should be accompanied by a description of what constitutes a need. This description is represented by an observation service predicate as a precondition."; 176 case R: return "Routine service, do at usual work hours."; 177 case RR: return "A report should be prepared and sent as quickly as possible."; 178 case S: return "With highest priority (e.g., emergency)."; 179 case T: return "It is critical to come as close as possible to the requested time (e.g., for a through antimicrobial level)."; 180 case UD: return "Drug is to be used as directed by the prescriber."; 181 case UR: return "Calls for prompt action."; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case A: return "ASAP"; 189 case CR: return "callback results"; 190 case CS: return "callback for scheduling"; 191 case CSP: return "callback placer for scheduling"; 192 case CSR: return "contact recipient for scheduling"; 193 case EL: return "elective"; 194 case EM: return "emergency"; 195 case P: return "preop"; 196 case PRN: return "as needed"; 197 case R: return "routine"; 198 case RR: return "rush reporting"; 199 case S: return "stat"; 200 case T: return "timing critical"; 201 case UD: return "use as directed"; 202 case UR: return "urgent"; 203 case NULL: return null; 204 default: return "?"; 205 } 206 } 207 208 209}