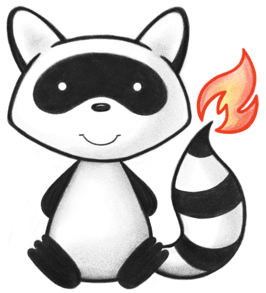
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3ActRelationshipSubset { 041 042 /** 043 * Used to indicate that the participation is a filtered subset of the total participations of the same type owned by the Act. 044 045 Used when there is a need to limit the participations to the first, the last, the next or some other filtered subset. 046 */ 047 _PARTICIPATIONSUBSET, 048 /** 049 * An occurrence that is scheduled to occur in the future. An Act whose effective time is greater than 'now', where 'now' is the time the instance is authored. 050 */ 051 FUTURE, 052 /** 053 * Represents a 'summary' of all acts that are scheduled to occur in the future (whose effective time is greater than 'now' where is the time the instance is authored.). The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc. 054 */ 055 FUTSUM, 056 /** 057 * Restricted to the latest known occurrence that is scheduled to occur. The Act with the highest known effective time. 058 */ 059 LAST, 060 /** 061 * Restricted to the nearest recent known occurrence scheduled to occur in the future. The Act with the lowest effective time, still greater than 'now'. ('now' is the time the instance is authored.) 062 */ 063 NEXT, 064 /** 065 * An occurrence that occurred or was scheduled to occur in the past. An Act whose effective time is less than 'now'. ('now' is the time the instance is authored.) 066 */ 067 PAST, 068 /** 069 * Restricted to the earliest known occurrence that occurred or was scheduled to occur in the past. The Act with the lowest effective time. ('now' is the time the instance is authored.) 070 */ 071 FIRST, 072 /** 073 * Represents a 'summary' of all acts that previously occurred or were scheduled to occur. The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc. ('now' is the time the instance is authored.) 074 */ 075 PREVSUM, 076 /** 077 * Restricted to the most recent known occurrence that occurred or was scheduled to occur in the past. The Act with the most recent effective time, still less than 'now'. ('now' is the time the instance is authored.) 078 */ 079 RECENT, 080 /** 081 * Represents a 'summary' of all acts that have occurred or were scheduled to occur and which are scheduled to occur in the future. The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc. 082 */ 083 SUM, 084 /** 085 * ActRelationshipExpectedSubset 086 */ 087 ACTRELATIONSHIPEXPECTEDSUBSET, 088 /** 089 * ActRelationshipPastSubset 090 */ 091 ACTRELATIONSHIPPASTSUBSET, 092 /** 093 * The occurrence whose value attribute is greater than all other occurrences at the time the instance is created. 094 */ 095 MAX, 096 /** 097 * The occurrence whose value attribute is less than all other occurrences at the time the instance is created. 098 */ 099 MIN, 100 /** 101 * added to help the parsers 102 */ 103 NULL; 104 public static V3ActRelationshipSubset fromCode(String codeString) throws FHIRException { 105 if (codeString == null || "".equals(codeString)) 106 return null; 107 if ("_ParticipationSubset".equals(codeString)) 108 return _PARTICIPATIONSUBSET; 109 if ("FUTURE".equals(codeString)) 110 return FUTURE; 111 if ("FUTSUM".equals(codeString)) 112 return FUTSUM; 113 if ("LAST".equals(codeString)) 114 return LAST; 115 if ("NEXT".equals(codeString)) 116 return NEXT; 117 if ("PAST".equals(codeString)) 118 return PAST; 119 if ("FIRST".equals(codeString)) 120 return FIRST; 121 if ("PREVSUM".equals(codeString)) 122 return PREVSUM; 123 if ("RECENT".equals(codeString)) 124 return RECENT; 125 if ("SUM".equals(codeString)) 126 return SUM; 127 if ("ActRelationshipExpectedSubset".equals(codeString)) 128 return ACTRELATIONSHIPEXPECTEDSUBSET; 129 if ("ActRelationshipPastSubset".equals(codeString)) 130 return ACTRELATIONSHIPPASTSUBSET; 131 if ("MAX".equals(codeString)) 132 return MAX; 133 if ("MIN".equals(codeString)) 134 return MIN; 135 throw new FHIRException("Unknown V3ActRelationshipSubset code '"+codeString+"'"); 136 } 137 public String toCode() { 138 switch (this) { 139 case _PARTICIPATIONSUBSET: return "_ParticipationSubset"; 140 case FUTURE: return "FUTURE"; 141 case FUTSUM: return "FUTSUM"; 142 case LAST: return "LAST"; 143 case NEXT: return "NEXT"; 144 case PAST: return "PAST"; 145 case FIRST: return "FIRST"; 146 case PREVSUM: return "PREVSUM"; 147 case RECENT: return "RECENT"; 148 case SUM: return "SUM"; 149 case ACTRELATIONSHIPEXPECTEDSUBSET: return "ActRelationshipExpectedSubset"; 150 case ACTRELATIONSHIPPASTSUBSET: return "ActRelationshipPastSubset"; 151 case MAX: return "MAX"; 152 case MIN: return "MIN"; 153 case NULL: return null; 154 default: return "?"; 155 } 156 } 157 public String getSystem() { 158 return "http://hl7.org/fhir/v3/ActRelationshipSubset"; 159 } 160 public String getDefinition() { 161 switch (this) { 162 case _PARTICIPATIONSUBSET: return "Used to indicate that the participation is a filtered subset of the total participations of the same type owned by the Act. \r\n\n Used when there is a need to limit the participations to the first, the last, the next or some other filtered subset."; 163 case FUTURE: return "An occurrence that is scheduled to occur in the future. An Act whose effective time is greater than 'now', where 'now' is the time the instance is authored."; 164 case FUTSUM: return "Represents a 'summary' of all acts that are scheduled to occur in the future (whose effective time is greater than 'now' where is the time the instance is authored.). The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc."; 165 case LAST: return "Restricted to the latest known occurrence that is scheduled to occur. The Act with the highest known effective time."; 166 case NEXT: return "Restricted to the nearest recent known occurrence scheduled to occur in the future. The Act with the lowest effective time, still greater than 'now'. ('now' is the time the instance is authored.)"; 167 case PAST: return "An occurrence that occurred or was scheduled to occur in the past. An Act whose effective time is less than 'now'. ('now' is the time the instance is authored.)"; 168 case FIRST: return "Restricted to the earliest known occurrence that occurred or was scheduled to occur in the past. The Act with the lowest effective time. ('now' is the time the instance is authored.)"; 169 case PREVSUM: return "Represents a 'summary' of all acts that previously occurred or were scheduled to occur. The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc. ('now' is the time the instance is authored.)"; 170 case RECENT: return "Restricted to the most recent known occurrence that occurred or was scheduled to occur in the past. The Act with the most recent effective time, still less than 'now'. ('now' is the time the instance is authored.)"; 171 case SUM: return "Represents a 'summary' of all acts that have occurred or were scheduled to occur and which are scheduled to occur in the future. The effectiveTime represents the outer boundary of all occurrences, repeatNumber represents the total number of repetitions, etc."; 172 case ACTRELATIONSHIPEXPECTEDSUBSET: return "ActRelationshipExpectedSubset"; 173 case ACTRELATIONSHIPPASTSUBSET: return "ActRelationshipPastSubset"; 174 case MAX: return "The occurrence whose value attribute is greater than all other occurrences at the time the instance is created."; 175 case MIN: return "The occurrence whose value attribute is less than all other occurrences at the time the instance is created."; 176 case NULL: return null; 177 default: return "?"; 178 } 179 } 180 public String getDisplay() { 181 switch (this) { 182 case _PARTICIPATIONSUBSET: return "ParticipationSubset"; 183 case FUTURE: return "expected future"; 184 case FUTSUM: return "future summary"; 185 case LAST: return "expected last"; 186 case NEXT: return "expected next"; 187 case PAST: return "previous"; 188 case FIRST: return "first known"; 189 case PREVSUM: return "previous summary"; 190 case RECENT: return "most recent"; 191 case SUM: return "summary"; 192 case ACTRELATIONSHIPEXPECTEDSUBSET: return "ActRelationshipExpectedSubset"; 193 case ACTRELATIONSHIPPASTSUBSET: return "ActRelationshipPastSubset"; 194 case MAX: return "maximum"; 195 case MIN: return "minimum"; 196 case NULL: return null; 197 default: return "?"; 198 } 199 } 200 201 202}