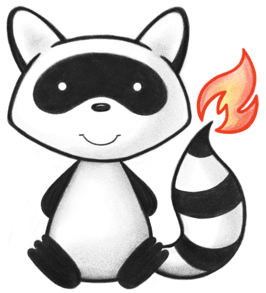
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3ActStatus { 041 042 /** 043 * Encompasses the expected states of an Act, but excludes "nullified" and "obsolete" which represent unusual terminal states for the life-cycle. 044 */ 045 NORMAL, 046 /** 047 * The Act has been terminated prior to the originally intended completion. 048 */ 049 ABORTED, 050 /** 051 * The Act can be performed or is being performed 052 */ 053 ACTIVE, 054 /** 055 * The Act has been abandoned before activation. 056 */ 057 CANCELLED, 058 /** 059 * An Act that has terminated normally after all of its constituents have been performed. 060 */ 061 COMPLETED, 062 /** 063 * An Act that is still in the preparatory stages has been put aside. No action can occur until the Act is released. 064 */ 065 HELD, 066 /** 067 * An Act that is in the preparatory stages and may not yet be acted upon 068 */ 069 NEW, 070 /** 071 * An Act that has been activated (actions could or have been performed against it), but has been temporarily disabled. No further action should be taken against it until it is released 072 */ 073 SUSPENDED, 074 /** 075 * This Act instance was created in error and has been 'removed' and is treated as though it never existed. A record is retained for audit purposes only. 076 */ 077 NULLIFIED, 078 /** 079 * This Act instance has been replaced by a new instance. 080 */ 081 OBSOLETE, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 public static V3ActStatus fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("normal".equals(codeString)) 090 return NORMAL; 091 if ("aborted".equals(codeString)) 092 return ABORTED; 093 if ("active".equals(codeString)) 094 return ACTIVE; 095 if ("cancelled".equals(codeString)) 096 return CANCELLED; 097 if ("completed".equals(codeString)) 098 return COMPLETED; 099 if ("held".equals(codeString)) 100 return HELD; 101 if ("new".equals(codeString)) 102 return NEW; 103 if ("suspended".equals(codeString)) 104 return SUSPENDED; 105 if ("nullified".equals(codeString)) 106 return NULLIFIED; 107 if ("obsolete".equals(codeString)) 108 return OBSOLETE; 109 throw new FHIRException("Unknown V3ActStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case NORMAL: return "normal"; 114 case ABORTED: return "aborted"; 115 case ACTIVE: return "active"; 116 case CANCELLED: return "cancelled"; 117 case COMPLETED: return "completed"; 118 case HELD: return "held"; 119 case NEW: return "new"; 120 case SUSPENDED: return "suspended"; 121 case NULLIFIED: return "nullified"; 122 case OBSOLETE: return "obsolete"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getSystem() { 128 return "http://hl7.org/fhir/v3/ActStatus"; 129 } 130 public String getDefinition() { 131 switch (this) { 132 case NORMAL: return "Encompasses the expected states of an Act, but excludes \"nullified\" and \"obsolete\" which represent unusual terminal states for the life-cycle."; 133 case ABORTED: return "The Act has been terminated prior to the originally intended completion."; 134 case ACTIVE: return "The Act can be performed or is being performed"; 135 case CANCELLED: return "The Act has been abandoned before activation."; 136 case COMPLETED: return "An Act that has terminated normally after all of its constituents have been performed."; 137 case HELD: return "An Act that is still in the preparatory stages has been put aside. No action can occur until the Act is released."; 138 case NEW: return "An Act that is in the preparatory stages and may not yet be acted upon"; 139 case SUSPENDED: return "An Act that has been activated (actions could or have been performed against it), but has been temporarily disabled. No further action should be taken against it until it is released"; 140 case NULLIFIED: return "This Act instance was created in error and has been 'removed' and is treated as though it never existed. A record is retained for audit purposes only."; 141 case OBSOLETE: return "This Act instance has been replaced by a new instance."; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDisplay() { 147 switch (this) { 148 case NORMAL: return "normal"; 149 case ABORTED: return "aborted"; 150 case ACTIVE: return "active"; 151 case CANCELLED: return "cancelled"; 152 case COMPLETED: return "completed"; 153 case HELD: return "held"; 154 case NEW: return "new"; 155 case SUSPENDED: return "suspended"; 156 case NULLIFIED: return "nullified"; 157 case OBSOLETE: return "obsolete"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 163 164}