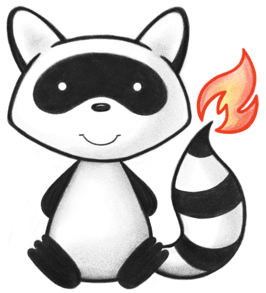
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3AddressPartType { 041 042 /** 043 * This can be a unit designator, such as apartment number, suite number, or floor. There may be several unit designators in an address (e.g., "3rd floor, Appt. 342"). This can also be a designator pointing away from the location, rather than specifying a smaller location within some larger one (e.g., Dutch "t.o." means "opposite to" for house boats located across the street facing houses). 044 */ 045 ADL, 046 /** 047 * Description: An address line is for either an additional locator, a delivery address or a street address. 048 */ 049 AL, 050 /** 051 * A delivery address line is frequently used instead of breaking out delivery mode, delivery installation, etc. An address generally has only a delivery address line or a street address line, but not both. 052 */ 053 DAL, 054 /** 055 * Description: A street address line is frequently used instead of breaking out build number, street name, street type, etc. An address generally has only a delivery address line or a street address line, but not both. 056 */ 057 SAL, 058 /** 059 * The numeric portion of a building number 060 */ 061 BNN, 062 /** 063 * The number of a building, house or lot alongside the street. Also known as "primary street number". This does not number the street but rather the building. 064 */ 065 BNR, 066 /** 067 * Any alphabetic character, fraction or other text that may appear after the numeric portion of a building number 068 */ 069 BNS, 070 /** 071 * The name of the party who will take receipt at the specified address, and will take on responsibility for ensuring delivery to the target recipient 072 */ 073 CAR, 074 /** 075 * A geographic sub-unit delineated for demographic purposes. 076 */ 077 CEN, 078 /** 079 * Country 080 */ 081 CNT, 082 /** 083 * A sub-unit of a state or province. (49 of the United States of America use the term "county;" Louisiana uses the term "parish".) 084 */ 085 CPA, 086 /** 087 * The name of the city, town, village, or other community or delivery center 088 */ 089 CTY, 090 /** 091 * Delimiters are printed without framing white space. If no value component is provided, the delimiter appears as a line break. 092 */ 093 DEL, 094 /** 095 * Indicates the type of delivery installation (the facility to which the mail will be delivered prior to final shipping via the delivery mode.) Example: post office, letter carrier depot, community mail center, station, etc. 096 */ 097 DINST, 098 /** 099 * The location of the delivery installation, usually a town or city, and is only required if the area is different from the municipality. Area to which mail delivery service is provided from any postal facility or service such as an individual letter carrier, rural route, or postal route. 100 */ 101 DINSTA, 102 /** 103 * A number, letter or name identifying a delivery installation. E.g., for Station A, the delivery installation qualifier would be 'A'. 104 */ 105 DINSTQ, 106 /** 107 * Direction (e.g., N, S, W, E) 108 */ 109 DIR, 110 /** 111 * Indicates the type of service offered, method of delivery. For example: post office box, rural route, general delivery, etc. 112 */ 113 DMOD, 114 /** 115 * Represents the routing information such as a letter carrier route number. It is the identifying number of the designator (the box number or rural route number). 116 */ 117 DMODID, 118 /** 119 * A value that uniquely identifies the postal address. 120 */ 121 DPID, 122 /** 123 * Description:An intersection denotes that the actual address is located AT or CLOSE TO the intersection OF two or more streets. 124 */ 125 INT, 126 /** 127 * A numbered box located in a post station. 128 */ 129 POB, 130 /** 131 * A subsection of a municipality 132 */ 133 PRE, 134 /** 135 * A sub-unit of a country with limited sovereignty in a federally organized country. 136 */ 137 STA, 138 /** 139 * The base name of a roadway or artery recognized by a municipality (excluding street type and direction) 140 */ 141 STB, 142 /** 143 * street name 144 */ 145 STR, 146 /** 147 * The designation given to the street. (e.g. Street, Avenue, Crescent, etc.) 148 */ 149 STTYP, 150 /** 151 * The number or name of a specific unit contained within a building or complex, as assigned by that building or complex. 152 */ 153 UNID, 154 /** 155 * Indicates the type of specific unit contained within a building or complex. E.g. Appartment, Floor 156 */ 157 UNIT, 158 /** 159 * A postal code designating a region defined by the postal service. 160 */ 161 ZIP, 162 /** 163 * added to help the parsers 164 */ 165 NULL; 166 public static V3AddressPartType fromCode(String codeString) throws FHIRException { 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("ADL".equals(codeString)) 170 return ADL; 171 if ("AL".equals(codeString)) 172 return AL; 173 if ("DAL".equals(codeString)) 174 return DAL; 175 if ("SAL".equals(codeString)) 176 return SAL; 177 if ("BNN".equals(codeString)) 178 return BNN; 179 if ("BNR".equals(codeString)) 180 return BNR; 181 if ("BNS".equals(codeString)) 182 return BNS; 183 if ("CAR".equals(codeString)) 184 return CAR; 185 if ("CEN".equals(codeString)) 186 return CEN; 187 if ("CNT".equals(codeString)) 188 return CNT; 189 if ("CPA".equals(codeString)) 190 return CPA; 191 if ("CTY".equals(codeString)) 192 return CTY; 193 if ("DEL".equals(codeString)) 194 return DEL; 195 if ("DINST".equals(codeString)) 196 return DINST; 197 if ("DINSTA".equals(codeString)) 198 return DINSTA; 199 if ("DINSTQ".equals(codeString)) 200 return DINSTQ; 201 if ("DIR".equals(codeString)) 202 return DIR; 203 if ("DMOD".equals(codeString)) 204 return DMOD; 205 if ("DMODID".equals(codeString)) 206 return DMODID; 207 if ("DPID".equals(codeString)) 208 return DPID; 209 if ("INT".equals(codeString)) 210 return INT; 211 if ("POB".equals(codeString)) 212 return POB; 213 if ("PRE".equals(codeString)) 214 return PRE; 215 if ("STA".equals(codeString)) 216 return STA; 217 if ("STB".equals(codeString)) 218 return STB; 219 if ("STR".equals(codeString)) 220 return STR; 221 if ("STTYP".equals(codeString)) 222 return STTYP; 223 if ("UNID".equals(codeString)) 224 return UNID; 225 if ("UNIT".equals(codeString)) 226 return UNIT; 227 if ("ZIP".equals(codeString)) 228 return ZIP; 229 throw new FHIRException("Unknown V3AddressPartType code '"+codeString+"'"); 230 } 231 public String toCode() { 232 switch (this) { 233 case ADL: return "ADL"; 234 case AL: return "AL"; 235 case DAL: return "DAL"; 236 case SAL: return "SAL"; 237 case BNN: return "BNN"; 238 case BNR: return "BNR"; 239 case BNS: return "BNS"; 240 case CAR: return "CAR"; 241 case CEN: return "CEN"; 242 case CNT: return "CNT"; 243 case CPA: return "CPA"; 244 case CTY: return "CTY"; 245 case DEL: return "DEL"; 246 case DINST: return "DINST"; 247 case DINSTA: return "DINSTA"; 248 case DINSTQ: return "DINSTQ"; 249 case DIR: return "DIR"; 250 case DMOD: return "DMOD"; 251 case DMODID: return "DMODID"; 252 case DPID: return "DPID"; 253 case INT: return "INT"; 254 case POB: return "POB"; 255 case PRE: return "PRE"; 256 case STA: return "STA"; 257 case STB: return "STB"; 258 case STR: return "STR"; 259 case STTYP: return "STTYP"; 260 case UNID: return "UNID"; 261 case UNIT: return "UNIT"; 262 case ZIP: return "ZIP"; 263 case NULL: return null; 264 default: return "?"; 265 } 266 } 267 public String getSystem() { 268 return "http://hl7.org/fhir/v3/AddressPartType"; 269 } 270 public String getDefinition() { 271 switch (this) { 272 case ADL: return "This can be a unit designator, such as apartment number, suite number, or floor. There may be several unit designators in an address (e.g., \"3rd floor, Appt. 342\"). This can also be a designator pointing away from the location, rather than specifying a smaller location within some larger one (e.g., Dutch \"t.o.\" means \"opposite to\" for house boats located across the street facing houses)."; 273 case AL: return "Description: An address line is for either an additional locator, a delivery address or a street address."; 274 case DAL: return "A delivery address line is frequently used instead of breaking out delivery mode, delivery installation, etc. An address generally has only a delivery address line or a street address line, but not both."; 275 case SAL: return "Description: A street address line is frequently used instead of breaking out build number, street name, street type, etc. An address generally has only a delivery address line or a street address line, but not both."; 276 case BNN: return "The numeric portion of a building number"; 277 case BNR: return "The number of a building, house or lot alongside the street. Also known as \"primary street number\". This does not number the street but rather the building."; 278 case BNS: return "Any alphabetic character, fraction or other text that may appear after the numeric portion of a building number"; 279 case CAR: return "The name of the party who will take receipt at the specified address, and will take on responsibility for ensuring delivery to the target recipient"; 280 case CEN: return "A geographic sub-unit delineated for demographic purposes."; 281 case CNT: return "Country"; 282 case CPA: return "A sub-unit of a state or province. (49 of the United States of America use the term \"county;\" Louisiana uses the term \"parish\".)"; 283 case CTY: return "The name of the city, town, village, or other community or delivery center"; 284 case DEL: return "Delimiters are printed without framing white space. If no value component is provided, the delimiter appears as a line break."; 285 case DINST: return "Indicates the type of delivery installation (the facility to which the mail will be delivered prior to final shipping via the delivery mode.) Example: post office, letter carrier depot, community mail center, station, etc."; 286 case DINSTA: return "The location of the delivery installation, usually a town or city, and is only required if the area is different from the municipality. Area to which mail delivery service is provided from any postal facility or service such as an individual letter carrier, rural route, or postal route."; 287 case DINSTQ: return "A number, letter or name identifying a delivery installation. E.g., for Station A, the delivery installation qualifier would be 'A'."; 288 case DIR: return "Direction (e.g., N, S, W, E)"; 289 case DMOD: return "Indicates the type of service offered, method of delivery. For example: post office box, rural route, general delivery, etc."; 290 case DMODID: return "Represents the routing information such as a letter carrier route number. It is the identifying number of the designator (the box number or rural route number)."; 291 case DPID: return "A value that uniquely identifies the postal address."; 292 case INT: return "Description:An intersection denotes that the actual address is located AT or CLOSE TO the intersection OF two or more streets."; 293 case POB: return "A numbered box located in a post station."; 294 case PRE: return "A subsection of a municipality"; 295 case STA: return "A sub-unit of a country with limited sovereignty in a federally organized country."; 296 case STB: return "The base name of a roadway or artery recognized by a municipality (excluding street type and direction)"; 297 case STR: return "street name"; 298 case STTYP: return "The designation given to the street. (e.g. Street, Avenue, Crescent, etc.)"; 299 case UNID: return "The number or name of a specific unit contained within a building or complex, as assigned by that building or complex."; 300 case UNIT: return "Indicates the type of specific unit contained within a building or complex. E.g. Appartment, Floor"; 301 case ZIP: return "A postal code designating a region defined by the postal service."; 302 case NULL: return null; 303 default: return "?"; 304 } 305 } 306 public String getDisplay() { 307 switch (this) { 308 case ADL: return "additional locator"; 309 case AL: return "address line"; 310 case DAL: return "delivery address line"; 311 case SAL: return "street address line"; 312 case BNN: return "building number numeric"; 313 case BNR: return "building number"; 314 case BNS: return "building number suffix"; 315 case CAR: return "care of"; 316 case CEN: return "census tract"; 317 case CNT: return "country"; 318 case CPA: return "county or parish"; 319 case CTY: return "municipality"; 320 case DEL: return "delimiter"; 321 case DINST: return "delivery installation type"; 322 case DINSTA: return "delivery installation area"; 323 case DINSTQ: return "delivery installation qualifier"; 324 case DIR: return "direction"; 325 case DMOD: return "delivery mode"; 326 case DMODID: return "delivery mode identifier"; 327 case DPID: return "delivery point identifier"; 328 case INT: return "intersection"; 329 case POB: return "post box"; 330 case PRE: return "precinct"; 331 case STA: return "state or province"; 332 case STB: return "street name base"; 333 case STR: return "street name"; 334 case STTYP: return "street type"; 335 case UNID: return "unit identifier"; 336 case UNIT: return "unit designator"; 337 case ZIP: return "postal code"; 338 case NULL: return null; 339 default: return "?"; 340 } 341 } 342 343 344}