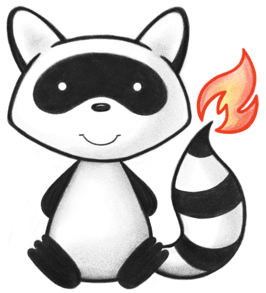
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3AddressUse { 041 042 /** 043 * Description: Address uses that can apply to both postal and telecommunication addresses. 044 */ 045 _GENERALADDRESSUSE, 046 /** 047 * Description: A flag indicating that the address is bad, in fact, useless. 048 */ 049 BAD, 050 /** 051 * Description: Indicates that the address is considered sensitive and should only be shared or published in accordance with organizational controls governing patient demographic information with increased sensitivity. Uses of Addresses. Lloyd to supply more complete description. 052 */ 053 CONF, 054 /** 055 * Description: A communication address at a home, attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available. 056 */ 057 H, 058 /** 059 * Description: The primary home, to reach a person after business hours. 060 */ 061 HP, 062 /** 063 * Description: A vacation home, to reach a person while on vacation. 064 */ 065 HV, 066 /** 067 * This address is no longer in use. 068 069 070 Usage Note: Address may also carry valid time ranges. This code is used to cover the situations where it is known that the address is no longer valid, but no particular time range for its use is known. 071 */ 072 OLD, 073 /** 074 * Description: A temporary address, may be good for visit or mailing. Note that an address history can provide more detailed information. 075 */ 076 TMP, 077 /** 078 * Description: An office address. First choice for business related contacts during business hours. 079 */ 080 WP, 081 /** 082 * Description: Indicates a work place address or telecommunication address that reaches the individual or organization directly without intermediaries. For phones, often referred to as a 'private line'. 083 */ 084 DIR, 085 /** 086 * Description: Indicates a work place address or telecommunication address that is a 'standard' address which may reach a reception service, mail-room, or other intermediary prior to the target entity. 087 */ 088 PUB, 089 /** 090 * Description: Address uses that only apply to postal addresses, not telecommunication addresses. 091 */ 092 _POSTALADDRESSUSE, 093 /** 094 * Description: Used primarily to visit an address. 095 */ 096 PHYS, 097 /** 098 * Description: Used to send mail. 099 */ 100 PST, 101 /** 102 * Description: Address uses that only apply to telecommunication addresses, not postal addresses. 103 */ 104 _TELECOMMUNICATIONADDRESSUSE, 105 /** 106 * Description: An automated answering machine used for less urgent cases and if the main purpose of contact is to leave a message or access an automated announcement. 107 */ 108 AS, 109 /** 110 * Description: A contact specifically designated to be used for emergencies. This is the first choice in emergencies, independent of any other use codes. 111 */ 112 EC, 113 /** 114 * Description: A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business. 115 */ 116 MC, 117 /** 118 * Description: A paging device suitable to solicit a callback or to leave a very short message. 119 */ 120 PG, 121 /** 122 * added to help the parsers 123 */ 124 NULL; 125 public static V3AddressUse fromCode(String codeString) throws FHIRException { 126 if (codeString == null || "".equals(codeString)) 127 return null; 128 if ("_GeneralAddressUse".equals(codeString)) 129 return _GENERALADDRESSUSE; 130 if ("BAD".equals(codeString)) 131 return BAD; 132 if ("CONF".equals(codeString)) 133 return CONF; 134 if ("H".equals(codeString)) 135 return H; 136 if ("HP".equals(codeString)) 137 return HP; 138 if ("HV".equals(codeString)) 139 return HV; 140 if ("OLD".equals(codeString)) 141 return OLD; 142 if ("TMP".equals(codeString)) 143 return TMP; 144 if ("WP".equals(codeString)) 145 return WP; 146 if ("DIR".equals(codeString)) 147 return DIR; 148 if ("PUB".equals(codeString)) 149 return PUB; 150 if ("_PostalAddressUse".equals(codeString)) 151 return _POSTALADDRESSUSE; 152 if ("PHYS".equals(codeString)) 153 return PHYS; 154 if ("PST".equals(codeString)) 155 return PST; 156 if ("_TelecommunicationAddressUse".equals(codeString)) 157 return _TELECOMMUNICATIONADDRESSUSE; 158 if ("AS".equals(codeString)) 159 return AS; 160 if ("EC".equals(codeString)) 161 return EC; 162 if ("MC".equals(codeString)) 163 return MC; 164 if ("PG".equals(codeString)) 165 return PG; 166 throw new FHIRException("Unknown V3AddressUse code '"+codeString+"'"); 167 } 168 public String toCode() { 169 switch (this) { 170 case _GENERALADDRESSUSE: return "_GeneralAddressUse"; 171 case BAD: return "BAD"; 172 case CONF: return "CONF"; 173 case H: return "H"; 174 case HP: return "HP"; 175 case HV: return "HV"; 176 case OLD: return "OLD"; 177 case TMP: return "TMP"; 178 case WP: return "WP"; 179 case DIR: return "DIR"; 180 case PUB: return "PUB"; 181 case _POSTALADDRESSUSE: return "_PostalAddressUse"; 182 case PHYS: return "PHYS"; 183 case PST: return "PST"; 184 case _TELECOMMUNICATIONADDRESSUSE: return "_TelecommunicationAddressUse"; 185 case AS: return "AS"; 186 case EC: return "EC"; 187 case MC: return "MC"; 188 case PG: return "PG"; 189 case NULL: return null; 190 default: return "?"; 191 } 192 } 193 public String getSystem() { 194 return "http://hl7.org/fhir/v3/AddressUse"; 195 } 196 public String getDefinition() { 197 switch (this) { 198 case _GENERALADDRESSUSE: return "Description: Address uses that can apply to both postal and telecommunication addresses."; 199 case BAD: return "Description: A flag indicating that the address is bad, in fact, useless."; 200 case CONF: return "Description: Indicates that the address is considered sensitive and should only be shared or published in accordance with organizational controls governing patient demographic information with increased sensitivity. Uses of Addresses. Lloyd to supply more complete description."; 201 case H: return "Description: A communication address at a home, attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available."; 202 case HP: return "Description: The primary home, to reach a person after business hours."; 203 case HV: return "Description: A vacation home, to reach a person while on vacation."; 204 case OLD: return "This address is no longer in use.\r\n\n \n Usage Note: Address may also carry valid time ranges. This code is used to cover the situations where it is known that the address is no longer valid, but no particular time range for its use is known."; 205 case TMP: return "Description: A temporary address, may be good for visit or mailing. Note that an address history can provide more detailed information."; 206 case WP: return "Description: An office address. First choice for business related contacts during business hours."; 207 case DIR: return "Description: Indicates a work place address or telecommunication address that reaches the individual or organization directly without intermediaries. For phones, often referred to as a 'private line'."; 208 case PUB: return "Description: Indicates a work place address or telecommunication address that is a 'standard' address which may reach a reception service, mail-room, or other intermediary prior to the target entity."; 209 case _POSTALADDRESSUSE: return "Description: Address uses that only apply to postal addresses, not telecommunication addresses."; 210 case PHYS: return "Description: Used primarily to visit an address."; 211 case PST: return "Description: Used to send mail."; 212 case _TELECOMMUNICATIONADDRESSUSE: return "Description: Address uses that only apply to telecommunication addresses, not postal addresses."; 213 case AS: return "Description: An automated answering machine used for less urgent cases and if the main purpose of contact is to leave a message or access an automated announcement."; 214 case EC: return "Description: A contact specifically designated to be used for emergencies. This is the first choice in emergencies, independent of any other use codes."; 215 case MC: return "Description: A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business."; 216 case PG: return "Description: A paging device suitable to solicit a callback or to leave a very short message."; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 public String getDisplay() { 222 switch (this) { 223 case _GENERALADDRESSUSE: return "_GeneralAddressUse"; 224 case BAD: return "bad address"; 225 case CONF: return "confidential address"; 226 case H: return "home address"; 227 case HP: return "primary home"; 228 case HV: return "vacation home"; 229 case OLD: return "no longer in use"; 230 case TMP: return "temporary address"; 231 case WP: return "work place"; 232 case DIR: return "direct"; 233 case PUB: return "public"; 234 case _POSTALADDRESSUSE: return "_PostalAddressUse"; 235 case PHYS: return "physical visit address"; 236 case PST: return "postal address"; 237 case _TELECOMMUNICATIONADDRESSUSE: return "_TelecommunicationAddressUse"; 238 case AS: return "answering service"; 239 case EC: return "emergency contact"; 240 case MC: return "mobile contact)"; 241 case PG: return "pager"; 242 case NULL: return null; 243 default: return "?"; 244 } 245 } 246 247 248}