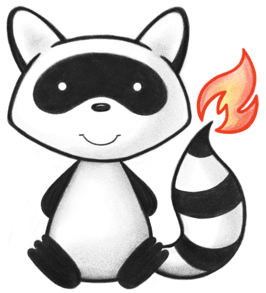
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3CalendarCycle { 041 042 /** 043 * CalendarCycleOneLetter 044 */ 045 _CALENDARCYCLEONELETTER, 046 /** 047 * week (continuous) 048 */ 049 CW, 050 /** 051 * year 052 */ 053 CY, 054 /** 055 * day of the month 056 */ 057 D, 058 /** 059 * day of the week (begins with Monday) 060 */ 061 DW, 062 /** 063 * hour of the day 064 */ 065 H, 066 /** 067 * month of the year 068 */ 069 M, 070 /** 071 * minute of the hour 072 */ 073 N, 074 /** 075 * second of the minute 076 */ 077 S, 078 /** 079 * CalendarCycleTwoLetter 080 */ 081 _CALENDARCYCLETWOLETTER, 082 /** 083 * day (continuous) 084 */ 085 CD, 086 /** 087 * hour (continuous) 088 */ 089 CH, 090 /** 091 * month (continuous) 092 */ 093 CM, 094 /** 095 * minute (continuous) 096 */ 097 CN, 098 /** 099 * second (continuous) 100 */ 101 CS, 102 /** 103 * day of the year 104 */ 105 DY, 106 /** 107 * week of the year 108 */ 109 WY, 110 /** 111 * The week with the month's first Thursday in it (analagous to the ISO 8601 definition for week of the year). 112 */ 113 WM, 114 /** 115 * added to help the parsers 116 */ 117 NULL; 118 public static V3CalendarCycle fromCode(String codeString) throws FHIRException { 119 if (codeString == null || "".equals(codeString)) 120 return null; 121 if ("_CalendarCycleOneLetter".equals(codeString)) 122 return _CALENDARCYCLEONELETTER; 123 if ("CW".equals(codeString)) 124 return CW; 125 if ("CY".equals(codeString)) 126 return CY; 127 if ("D".equals(codeString)) 128 return D; 129 if ("DW".equals(codeString)) 130 return DW; 131 if ("H".equals(codeString)) 132 return H; 133 if ("M".equals(codeString)) 134 return M; 135 if ("N".equals(codeString)) 136 return N; 137 if ("S".equals(codeString)) 138 return S; 139 if ("_CalendarCycleTwoLetter".equals(codeString)) 140 return _CALENDARCYCLETWOLETTER; 141 if ("CD".equals(codeString)) 142 return CD; 143 if ("CH".equals(codeString)) 144 return CH; 145 if ("CM".equals(codeString)) 146 return CM; 147 if ("CN".equals(codeString)) 148 return CN; 149 if ("CS".equals(codeString)) 150 return CS; 151 if ("DY".equals(codeString)) 152 return DY; 153 if ("WY".equals(codeString)) 154 return WY; 155 if ("WM".equals(codeString)) 156 return WM; 157 throw new FHIRException("Unknown V3CalendarCycle code '"+codeString+"'"); 158 } 159 public String toCode() { 160 switch (this) { 161 case _CALENDARCYCLEONELETTER: return "_CalendarCycleOneLetter"; 162 case CW: return "CW"; 163 case CY: return "CY"; 164 case D: return "D"; 165 case DW: return "DW"; 166 case H: return "H"; 167 case M: return "M"; 168 case N: return "N"; 169 case S: return "S"; 170 case _CALENDARCYCLETWOLETTER: return "_CalendarCycleTwoLetter"; 171 case CD: return "CD"; 172 case CH: return "CH"; 173 case CM: return "CM"; 174 case CN: return "CN"; 175 case CS: return "CS"; 176 case DY: return "DY"; 177 case WY: return "WY"; 178 case WM: return "WM"; 179 case NULL: return null; 180 default: return "?"; 181 } 182 } 183 public String getSystem() { 184 return "http://hl7.org/fhir/v3/CalendarCycle"; 185 } 186 public String getDefinition() { 187 switch (this) { 188 case _CALENDARCYCLEONELETTER: return "CalendarCycleOneLetter"; 189 case CW: return "week (continuous)"; 190 case CY: return "year"; 191 case D: return "day of the month"; 192 case DW: return "day of the week (begins with Monday)"; 193 case H: return "hour of the day"; 194 case M: return "month of the year"; 195 case N: return "minute of the hour"; 196 case S: return "second of the minute"; 197 case _CALENDARCYCLETWOLETTER: return "CalendarCycleTwoLetter"; 198 case CD: return "day (continuous)"; 199 case CH: return "hour (continuous)"; 200 case CM: return "month (continuous)"; 201 case CN: return "minute (continuous)"; 202 case CS: return "second (continuous)"; 203 case DY: return "day of the year"; 204 case WY: return "week of the year"; 205 case WM: return "The week with the month's first Thursday in it (analagous to the ISO 8601 definition for week of the year)."; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 public String getDisplay() { 211 switch (this) { 212 case _CALENDARCYCLEONELETTER: return "CalendarCycleOneLetter"; 213 case CW: return "week (continuous)"; 214 case CY: return "year"; 215 case D: return "day of the month"; 216 case DW: return "day of the week (begins with Monday)"; 217 case H: return "hour of the day"; 218 case M: return "month of the year"; 219 case N: return "minute of the hour"; 220 case S: return "second of the minute"; 221 case _CALENDARCYCLETWOLETTER: return "CalendarCycleTwoLetter"; 222 case CD: return "day (continuous)"; 223 case CH: return "hour (continuous)"; 224 case CM: return "month (continuous)"; 225 case CN: return "minute (continuous)"; 226 case CS: return "second (continuous)"; 227 case DY: return "day of the year"; 228 case WY: return "week of the year"; 229 case WM: return "week of the month"; 230 case NULL: return null; 231 default: return "?"; 232 } 233 } 234 235 236}