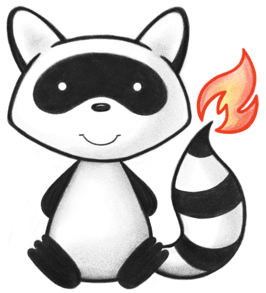
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3Charset { 041 042 /** 043 * HL7 is indifferent to the use of this Charset. 044 */ 045 EBCDIC, 046 /** 047 * Deprecated for HL7 use. 048 */ 049 ISO10646UCS2, 050 /** 051 * Deprecated for HL7 use. 052 */ 053 ISO10646UCS4, 054 /** 055 * HL7 is indifferent to the use of this Charset. 056 */ 057 ISO88591, 058 /** 059 * HL7 is indifferent to the use of this Charset. 060 */ 061 ISO88592, 062 /** 063 * HL7 is indifferent to the use of this Charset. 064 */ 065 ISO88595, 066 /** 067 * HL7 is indifferent to the use of this Charset. 068 */ 069 JIS2022JP, 070 /** 071 * Required for HL7 use. 072 */ 073 USASCII, 074 /** 075 * HL7 is indifferent to the use of this Charset. 076 */ 077 UTF7, 078 /** 079 * Required for Unicode support. 080 */ 081 UTF8, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 public static V3Charset fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("EBCDIC".equals(codeString)) 090 return EBCDIC; 091 if ("ISO-10646-UCS-2".equals(codeString)) 092 return ISO10646UCS2; 093 if ("ISO-10646-UCS-4".equals(codeString)) 094 return ISO10646UCS4; 095 if ("ISO-8859-1".equals(codeString)) 096 return ISO88591; 097 if ("ISO-8859-2".equals(codeString)) 098 return ISO88592; 099 if ("ISO-8859-5".equals(codeString)) 100 return ISO88595; 101 if ("JIS-2022-JP".equals(codeString)) 102 return JIS2022JP; 103 if ("US-ASCII".equals(codeString)) 104 return USASCII; 105 if ("UTF-7".equals(codeString)) 106 return UTF7; 107 if ("UTF-8".equals(codeString)) 108 return UTF8; 109 throw new FHIRException("Unknown V3Charset code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case EBCDIC: return "EBCDIC"; 114 case ISO10646UCS2: return "ISO-10646-UCS-2"; 115 case ISO10646UCS4: return "ISO-10646-UCS-4"; 116 case ISO88591: return "ISO-8859-1"; 117 case ISO88592: return "ISO-8859-2"; 118 case ISO88595: return "ISO-8859-5"; 119 case JIS2022JP: return "JIS-2022-JP"; 120 case USASCII: return "US-ASCII"; 121 case UTF7: return "UTF-7"; 122 case UTF8: return "UTF-8"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getSystem() { 128 return "http://hl7.org/fhir/v3/Charset"; 129 } 130 public String getDefinition() { 131 switch (this) { 132 case EBCDIC: return "HL7 is indifferent to the use of this Charset."; 133 case ISO10646UCS2: return "Deprecated for HL7 use."; 134 case ISO10646UCS4: return "Deprecated for HL7 use."; 135 case ISO88591: return "HL7 is indifferent to the use of this Charset."; 136 case ISO88592: return "HL7 is indifferent to the use of this Charset."; 137 case ISO88595: return "HL7 is indifferent to the use of this Charset."; 138 case JIS2022JP: return "HL7 is indifferent to the use of this Charset."; 139 case USASCII: return "Required for HL7 use."; 140 case UTF7: return "HL7 is indifferent to the use of this Charset."; 141 case UTF8: return "Required for Unicode support."; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDisplay() { 147 switch (this) { 148 case EBCDIC: return "EBCDIC"; 149 case ISO10646UCS2: return "ISO-10646-UCS-2"; 150 case ISO10646UCS4: return "ISO-10646-UCS-4"; 151 case ISO88591: return "ISO-8859-1"; 152 case ISO88592: return "ISO-8859-2"; 153 case ISO88595: return "ISO-8859-5"; 154 case JIS2022JP: return "JIS-2022-JP"; 155 case USASCII: return "US-ASCII"; 156 case UTF7: return "UTF-7"; 157 case UTF8: return "UTF-8"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 163 164}