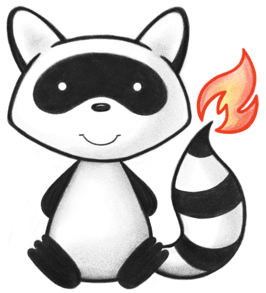
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3ContextControl { 041 042 /** 043 * The association adds to the existing context associated with the Act. Both this association and any associations propagated from ancestor Acts are interpreted as being related to this Act. 044 */ 045 _CONTEXTCONTROLADDITIVE, 046 /** 047 * The association adds to the existing context associated with the Act, but will not propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as "Additive, Non-Propagating" it means that the author will be added to the set of author participations that have propagated from ancestor Acts for the purpose of this Act. However only the previously propagated authors will propagate to any child Acts that allow context to be propagated. 048 */ 049 AN, 050 /** 051 * The association adds to the existing context associated with the Act, and will propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as "Additive, Propagating" it means that the author will be added to the set of author participations that have propagated from ancestor Acts, and will itself propagate with the other authors to any child Acts that allow context to be propagated. 052 */ 053 AP, 054 /** 055 * The association applies only to the current Act and will not propagate to any child Acts that are related via a conducting ActRelationship (refer to contextConductionInd). 056 */ 057 _CONTEXTCONTROLNONPROPAGATING, 058 /** 059 * The association is added to the existing context associated with the Act, but overrides an association with the same typeCode. However, this overriding association will not propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as "Overriding, Non-Propagating" it means that the author will replace the set of author participations that have propagated from ancestor Acts. Furthermore, no author participations whatsoever will propagate to any child Acts that allow context to be propagated. 060 */ 061 ON, 062 /** 063 * The association adds to the existing context associated with the Act, but replaces associations propagated from ancestor Acts whose typeCodes are the same or more specific. 064 */ 065 _CONTEXTCONTROLOVERRIDING, 066 /** 067 * The association is added to the existing context associated with the Act, but overrides an association with the same typeCode. This overriding association will propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as "Overriding, Propagating" it means that the author will replace the set of author participations that have propagated from ancestor Acts, and will itself be the only author to propagate to any child Acts that allow context to be propagated. 068 */ 069 OP, 070 /** 071 * The association propagates to any child Acts that are related via a conducting ActRelationship (refer to contextConductionInd). 072 */ 073 _CONTEXTCONTROLPROPAGATING, 074 /** 075 * added to help the parsers 076 */ 077 NULL; 078 public static V3ContextControl fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("_ContextControlAdditive".equals(codeString)) 082 return _CONTEXTCONTROLADDITIVE; 083 if ("AN".equals(codeString)) 084 return AN; 085 if ("AP".equals(codeString)) 086 return AP; 087 if ("_ContextControlNonPropagating".equals(codeString)) 088 return _CONTEXTCONTROLNONPROPAGATING; 089 if ("ON".equals(codeString)) 090 return ON; 091 if ("_ContextControlOverriding".equals(codeString)) 092 return _CONTEXTCONTROLOVERRIDING; 093 if ("OP".equals(codeString)) 094 return OP; 095 if ("_ContextControlPropagating".equals(codeString)) 096 return _CONTEXTCONTROLPROPAGATING; 097 throw new FHIRException("Unknown V3ContextControl code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case _CONTEXTCONTROLADDITIVE: return "_ContextControlAdditive"; 102 case AN: return "AN"; 103 case AP: return "AP"; 104 case _CONTEXTCONTROLNONPROPAGATING: return "_ContextControlNonPropagating"; 105 case ON: return "ON"; 106 case _CONTEXTCONTROLOVERRIDING: return "_ContextControlOverriding"; 107 case OP: return "OP"; 108 case _CONTEXTCONTROLPROPAGATING: return "_ContextControlPropagating"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getSystem() { 114 return "http://hl7.org/fhir/v3/ContextControl"; 115 } 116 public String getDefinition() { 117 switch (this) { 118 case _CONTEXTCONTROLADDITIVE: return "The association adds to the existing context associated with the Act. Both this association and any associations propagated from ancestor Acts are interpreted as being related to this Act."; 119 case AN: return "The association adds to the existing context associated with the Act, but will not propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Additive, Non-Propagating\" it means that the author will be added to the set of author participations that have propagated from ancestor Acts for the purpose of this Act. However only the previously propagated authors will propagate to any child Acts that allow context to be propagated."; 120 case AP: return "The association adds to the existing context associated with the Act, and will propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Additive, Propagating\" it means that the author will be added to the set of author participations that have propagated from ancestor Acts, and will itself propagate with the other authors to any child Acts that allow context to be propagated."; 121 case _CONTEXTCONTROLNONPROPAGATING: return "The association applies only to the current Act and will not propagate to any child Acts that are related via a conducting ActRelationship (refer to contextConductionInd)."; 122 case ON: return "The association is added to the existing context associated with the Act, but overrides an association with the same typeCode. However, this overriding association will not propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Overriding, Non-Propagating\" it means that the author will replace the set of author participations that have propagated from ancestor Acts. Furthermore, no author participations whatsoever will propagate to any child Acts that allow context to be propagated."; 123 case _CONTEXTCONTROLOVERRIDING: return "The association adds to the existing context associated with the Act, but replaces associations propagated from ancestor Acts whose typeCodes are the same or more specific."; 124 case OP: return "The association is added to the existing context associated with the Act, but overrides an association with the same typeCode. This overriding association will propagate to any descendant Acts reached by conducting ActRelationships (see contextControlCode). Examples: If an 'Author' Participation were marked as \"Overriding, Propagating\" it means that the author will replace the set of author participations that have propagated from ancestor Acts, and will itself be the only author to propagate to any child Acts that allow context to be propagated."; 125 case _CONTEXTCONTROLPROPAGATING: return "The association propagates to any child Acts that are related via a conducting ActRelationship (refer to contextConductionInd)."; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case _CONTEXTCONTROLADDITIVE: return "ContextControlAdditive"; 133 case AN: return "additive, non-propagating"; 134 case AP: return "additive, propagating"; 135 case _CONTEXTCONTROLNONPROPAGATING: return "ContextControlNonPropagating"; 136 case ON: return "overriding, non-propagating"; 137 case _CONTEXTCONTROLOVERRIDING: return "ContextControlOverriding"; 138 case OP: return "overriding, propagating"; 139 case _CONTEXTCONTROLPROPAGATING: return "ContextControlPropagating"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 145 146}