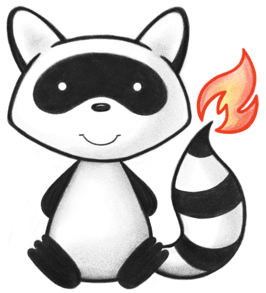
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3DataOperation { 041 042 /** 043 * Description:Act on an object or objects. 044 */ 045 OPERATE, 046 /** 047 * Description:Fundamental operation in an Information System (IS) that results only in the act of bringing an object into existence. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 048 */ 049 CREATE, 050 /** 051 * Description:Fundamental operation in an Information System (IS) that results only in the removal of information about an object from memory or storage. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. 052 */ 053 DELETE, 054 /** 055 * Description:Fundamental operation in an IS that results only in initiating performance of a single or set of programs (i.e., software objects). Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. 056 */ 057 EXECUTE, 058 /** 059 * Description:Fundamental operation in an Information System (IS) that results only in the flow of information about an object to a subject. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. 060 */ 061 READ, 062 /** 063 * Definition:Fundamental operation in an Information System (IS) that results only in the revision or alteration of an object. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. 064 */ 065 UPDATE, 066 /** 067 * Description:Fundamental operation in an Information System (IS) that results only in the addition of information to an object already in existence. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. 068 */ 069 APPEND, 070 /** 071 * Description:Change the status of an object representing an Act. 072 */ 073 MODIFYSTATUS, 074 /** 075 * Description:Change the status of an object representing an Act to "aborted", i.e., terminated prior to the originally intended completion. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 076 */ 077 ABORT, 078 /** 079 * Description:Change the status of an object representing an Act to "active", i.e., so it can be performed or is being performed, for the first time. (Contrast with REACTIVATE.) For an HL7 Act, the state transitions per the HL7 Reference Information Model. 080 */ 081 ACTIVATE, 082 /** 083 * Description:Change the status of an object representing an Act to "cancelled", i.e., abandoned before activation. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 084 */ 085 CANCEL, 086 /** 087 * Description:Change the status of an object representing an Act to "completed", i.e., terminated normally after all of its constituents have been performed. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 088 */ 089 COMPLETE, 090 /** 091 * Description:Change the status of an object representing an Act to "held", i.e., put aside an Act that is still in preparatory stages. No action can occur until the Act is released. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 092 */ 093 HOLD, 094 /** 095 * Description:Change the status of an object representing an Act to a normal state. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 096 */ 097 JUMP, 098 /** 099 * Description:Change the status of an object representing an Act to "nullified", i.e., treat as though it never existed. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 100 */ 101 NULLIFY, 102 /** 103 * Description:Change the status of an object representing an Act to "obsolete" when it has been replaced by a new instance. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 104 */ 105 OBSOLETE, 106 /** 107 * Description:Change the status of a formerly active object representing an Act to "active", i.e., so it can again be performed or is being performed. (Contrast with ACTIVATE.) For an HL7 Act, the state transitions per the HL7 Reference Information Model. 108 */ 109 REACTIVATE, 110 /** 111 * Description:Change the status of an object representing an Act so it is no longer "held", i.e., allow action to occur. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 112 */ 113 RELEASE, 114 /** 115 * Description:Change the status of a suspended object representing an Act to "active", i.e., so it can be performed or is being performed. For an HL7 Act, the state transitions per the HL7 Reference Information Model. 116 */ 117 RESUME, 118 /** 119 * Definition:Change the status of an object representing an Act to suspended, i.e., so it is temporarily not in service. 120 */ 121 SUSPEND, 122 /** 123 * added to help the parsers 124 */ 125 NULL; 126 public static V3DataOperation fromCode(String codeString) throws FHIRException { 127 if (codeString == null || "".equals(codeString)) 128 return null; 129 if ("OPERATE".equals(codeString)) 130 return OPERATE; 131 if ("CREATE".equals(codeString)) 132 return CREATE; 133 if ("DELETE".equals(codeString)) 134 return DELETE; 135 if ("EXECUTE".equals(codeString)) 136 return EXECUTE; 137 if ("READ".equals(codeString)) 138 return READ; 139 if ("UPDATE".equals(codeString)) 140 return UPDATE; 141 if ("APPEND".equals(codeString)) 142 return APPEND; 143 if ("MODIFYSTATUS".equals(codeString)) 144 return MODIFYSTATUS; 145 if ("ABORT".equals(codeString)) 146 return ABORT; 147 if ("ACTIVATE".equals(codeString)) 148 return ACTIVATE; 149 if ("CANCEL".equals(codeString)) 150 return CANCEL; 151 if ("COMPLETE".equals(codeString)) 152 return COMPLETE; 153 if ("HOLD".equals(codeString)) 154 return HOLD; 155 if ("JUMP".equals(codeString)) 156 return JUMP; 157 if ("NULLIFY".equals(codeString)) 158 return NULLIFY; 159 if ("OBSOLETE".equals(codeString)) 160 return OBSOLETE; 161 if ("REACTIVATE".equals(codeString)) 162 return REACTIVATE; 163 if ("RELEASE".equals(codeString)) 164 return RELEASE; 165 if ("RESUME".equals(codeString)) 166 return RESUME; 167 if ("SUSPEND".equals(codeString)) 168 return SUSPEND; 169 throw new FHIRException("Unknown V3DataOperation code '"+codeString+"'"); 170 } 171 public String toCode() { 172 switch (this) { 173 case OPERATE: return "OPERATE"; 174 case CREATE: return "CREATE"; 175 case DELETE: return "DELETE"; 176 case EXECUTE: return "EXECUTE"; 177 case READ: return "READ"; 178 case UPDATE: return "UPDATE"; 179 case APPEND: return "APPEND"; 180 case MODIFYSTATUS: return "MODIFYSTATUS"; 181 case ABORT: return "ABORT"; 182 case ACTIVATE: return "ACTIVATE"; 183 case CANCEL: return "CANCEL"; 184 case COMPLETE: return "COMPLETE"; 185 case HOLD: return "HOLD"; 186 case JUMP: return "JUMP"; 187 case NULLIFY: return "NULLIFY"; 188 case OBSOLETE: return "OBSOLETE"; 189 case REACTIVATE: return "REACTIVATE"; 190 case RELEASE: return "RELEASE"; 191 case RESUME: return "RESUME"; 192 case SUSPEND: return "SUSPEND"; 193 case NULL: return null; 194 default: return "?"; 195 } 196 } 197 public String getSystem() { 198 return "http://hl7.org/fhir/v3/DataOperation"; 199 } 200 public String getDefinition() { 201 switch (this) { 202 case OPERATE: return "Description:Act on an object or objects."; 203 case CREATE: return "Description:Fundamental operation in an Information System (IS) that results only in the act of bringing an object into existence. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 204 case DELETE: return "Description:Fundamental operation in an Information System (IS) that results only in the removal of information about an object from memory or storage. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 205 case EXECUTE: return "Description:Fundamental operation in an IS that results only in initiating performance of a single or set of programs (i.e., software objects). Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 206 case READ: return "Description:Fundamental operation in an Information System (IS) that results only in the flow of information about an object to a subject. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 207 case UPDATE: return "Definition:Fundamental operation in an Information System (IS) that results only in the revision or alteration of an object. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 208 case APPEND: return "Description:Fundamental operation in an Information System (IS) that results only in the addition of information to an object already in existence. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 209 case MODIFYSTATUS: return "Description:Change the status of an object representing an Act."; 210 case ABORT: return "Description:Change the status of an object representing an Act to \"aborted\", i.e., terminated prior to the originally intended completion. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 211 case ACTIVATE: return "Description:Change the status of an object representing an Act to \"active\", i.e., so it can be performed or is being performed, for the first time. (Contrast with REACTIVATE.) For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 212 case CANCEL: return "Description:Change the status of an object representing an Act to \"cancelled\", i.e., abandoned before activation. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 213 case COMPLETE: return "Description:Change the status of an object representing an Act to \"completed\", i.e., terminated normally after all of its constituents have been performed. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 214 case HOLD: return "Description:Change the status of an object representing an Act to \"held\", i.e., put aside an Act that is still in preparatory stages. No action can occur until the Act is released. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 215 case JUMP: return "Description:Change the status of an object representing an Act to a normal state. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 216 case NULLIFY: return "Description:Change the status of an object representing an Act to \"nullified\", i.e., treat as though it never existed. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 217 case OBSOLETE: return "Description:Change the status of an object representing an Act to \"obsolete\" when it has been replaced by a new instance. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 218 case REACTIVATE: return "Description:Change the status of a formerly active object representing an Act to \"active\", i.e., so it can again be performed or is being performed. (Contrast with ACTIVATE.) For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 219 case RELEASE: return "Description:Change the status of an object representing an Act so it is no longer \"held\", i.e., allow action to occur. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 220 case RESUME: return "Description:Change the status of a suspended object representing an Act to \"active\", i.e., so it can be performed or is being performed. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 221 case SUSPEND: return "Definition:Change the status of an object representing an Act to suspended, i.e., so it is temporarily not in service."; 222 case NULL: return null; 223 default: return "?"; 224 } 225 } 226 public String getDisplay() { 227 switch (this) { 228 case OPERATE: return "operate"; 229 case CREATE: return "create"; 230 case DELETE: return "delete"; 231 case EXECUTE: return "execute"; 232 case READ: return "read"; 233 case UPDATE: return "revise"; 234 case APPEND: return "append"; 235 case MODIFYSTATUS: return "modify status"; 236 case ABORT: return "abort"; 237 case ACTIVATE: return "activate"; 238 case CANCEL: return "cancel"; 239 case COMPLETE: return "complete"; 240 case HOLD: return "hold"; 241 case JUMP: return "jump"; 242 case NULLIFY: return "nullify"; 243 case OBSOLETE: return "obsolete"; 244 case REACTIVATE: return "reactivate"; 245 case RELEASE: return "release"; 246 case RESUME: return "resume"; 247 case SUSPEND: return "suspend"; 248 case NULL: return null; 249 default: return "?"; 250 } 251 } 252 253 254}