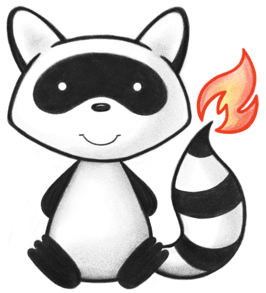
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3DocumentCompletion { 041 042 /** 043 * A completion status in which a document has been signed manually or electronically by one or more individuals who attest to its accuracy. No explicit determination is made that the assigned individual has performed the authentication. While the standard allows multiple instances of authentication, it would be typical to have a single instance of authentication, usually by the assigned individual. 044 */ 045 AU, 046 /** 047 * A completion status in which information has been orally recorded but not yet transcribed. 048 */ 049 DI, 050 /** 051 * A completion status in which document content, other than dictation, has been received but has not been translated into the final electronic format. Examples include paper documents, whether hand-written or typewritten, and intermediate electronic forms, such as voice to text. 052 */ 053 DO, 054 /** 055 * A completion status in which information is known to be missing from a transcribed document. 056 */ 057 IN, 058 /** 059 * A workflow status where the material has been assigned to personnel to perform the task of transcription. The document remains in this state until the document is transcribed. 060 */ 061 IP, 062 /** 063 * A completion status in which a document has been signed manually or electronically by the individual who is legally responsible for that document. This is the most mature state in the workflow progression. 064 */ 065 LA, 066 /** 067 * A completion status in which a document was created in error or was placed in the wrong chart. The document is no longer available. 068 */ 069 NU, 070 /** 071 * A completion status in which a document is transcribed but not authenticated. 072 */ 073 PA, 074 /** 075 * A completion status where the document is complete and there is no expectation that the document will be signed. 076 */ 077 UC, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 public static V3DocumentCompletion fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("AU".equals(codeString)) 086 return AU; 087 if ("DI".equals(codeString)) 088 return DI; 089 if ("DO".equals(codeString)) 090 return DO; 091 if ("IN".equals(codeString)) 092 return IN; 093 if ("IP".equals(codeString)) 094 return IP; 095 if ("LA".equals(codeString)) 096 return LA; 097 if ("NU".equals(codeString)) 098 return NU; 099 if ("PA".equals(codeString)) 100 return PA; 101 if ("UC".equals(codeString)) 102 return UC; 103 throw new FHIRException("Unknown V3DocumentCompletion code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case AU: return "AU"; 108 case DI: return "DI"; 109 case DO: return "DO"; 110 case IN: return "IN"; 111 case IP: return "IP"; 112 case LA: return "LA"; 113 case NU: return "NU"; 114 case PA: return "PA"; 115 case UC: return "UC"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 return "http://hl7.org/fhir/v3/DocumentCompletion"; 122 } 123 public String getDefinition() { 124 switch (this) { 125 case AU: return "A completion status in which a document has been signed manually or electronically by one or more individuals who attest to its accuracy. No explicit determination is made that the assigned individual has performed the authentication. While the standard allows multiple instances of authentication, it would be typical to have a single instance of authentication, usually by the assigned individual."; 126 case DI: return "A completion status in which information has been orally recorded but not yet transcribed."; 127 case DO: return "A completion status in which document content, other than dictation, has been received but has not been translated into the final electronic format. Examples include paper documents, whether hand-written or typewritten, and intermediate electronic forms, such as voice to text."; 128 case IN: return "A completion status in which information is known to be missing from a transcribed document."; 129 case IP: return "A workflow status where the material has been assigned to personnel to perform the task of transcription. The document remains in this state until the document is transcribed."; 130 case LA: return "A completion status in which a document has been signed manually or electronically by the individual who is legally responsible for that document. This is the most mature state in the workflow progression."; 131 case NU: return "A completion status in which a document was created in error or was placed in the wrong chart. The document is no longer available."; 132 case PA: return "A completion status in which a document is transcribed but not authenticated."; 133 case UC: return "A completion status where the document is complete and there is no expectation that the document will be signed."; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case AU: return "authenticated"; 141 case DI: return "dictated"; 142 case DO: return "documented"; 143 case IN: return "incomplete"; 144 case IP: return "in progress"; 145 case LA: return "legally authenticated"; 146 case NU: return "nullified document"; 147 case PA: return "pre-authenticated"; 148 case UC: return "unsigned completed document"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 154 155}