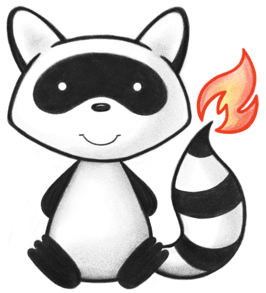
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3EntityClass { 041 042 /** 043 * Corresponds to the Entity class 044 */ 045 ENT, 046 /** 047 * A health chart included to serve as a document receiving entity in the management of medical records. 048 */ 049 HCE, 050 /** 051 * Anything that essentially has the property of life, independent of current state (a dead human corpse is still essentially a living subject). 052 */ 053 LIV, 054 /** 055 * A subtype of living subject that includes all living things except the species Homo Sapiens. 056 */ 057 NLIV, 058 /** 059 * A living subject from the animal kingdom. 060 */ 061 ANM, 062 /** 063 * All single celled living organisms including protozoa, bacteria, yeast, viruses, etc. 064 */ 065 MIC, 066 /** 067 * A living subject from the order of plants. 068 */ 069 PLNT, 070 /** 071 * A living subject of the species homo sapiens. 072 */ 073 PSN, 074 /** 075 * Any thing that has extension in space and mass, may be of living or non-living origin. 076 */ 077 MAT, 078 /** 079 * A substance that is fully defined by an organic or inorganic chemical formula, includes mixtures of other chemical substances. Refine using, e.g., IUPAC codes. 080 */ 081 CHEM, 082 /** 083 * Naturally occurring, processed or manufactured entities that are primarily used as food for humans and animals. 084 */ 085 FOOD, 086 /** 087 * Corresponds to the ManufacturedMaterial class 088 */ 089 MMAT, 090 /** 091 * A container of other entities. 092 */ 093 CONT, 094 /** 095 * A type of container that can hold other containers or other holders. 096 */ 097 HOLD, 098 /** 099 * A subtype of ManufacturedMaterial used in an activity, without being substantially changed through that activity. The kind of device is identified by the code attribute inherited from Entity. 100 101 102 Usage: This includes durable (reusable) medical equipment as well as disposable equipment. 103 */ 104 DEV, 105 /** 106 * A physical artifact that stores information about the granting of authorization. 107 */ 108 CER, 109 /** 110 * Class to contain unique attributes of diagnostic imaging equipment. 111 */ 112 MODDV, 113 /** 114 * A social or legal structure formed by human beings. 115 */ 116 ORG, 117 /** 118 * An agency of the people of a state often assuming some authority over a certain matter. Includes government, governmental agencies, associations. 119 */ 120 PUB, 121 /** 122 * A politically organized body of people bonded by territory, culture, or ethnicity, having sovereignty (to a certain extent) granted by other states (enclosing or neighboring states). This includes countries (nations), provinces (e.g., one of the United States of America or a French departement), counties or municipalities. Refine using, e.g., ISO country codes, FIPS-PUB state codes, etc. 123 */ 124 STATE, 125 /** 126 * A politically organized body of people bonded by territory and known as a nation. 127 */ 128 NAT, 129 /** 130 * A physical place or site with its containing structure. May be natural or man-made. The geographic position of a place may or may not be constant. 131 */ 132 PLC, 133 /** 134 * The territory of a city, town or other municipality. 135 */ 136 CITY, 137 /** 138 * The territory of a sovereign nation. 139 */ 140 COUNTRY, 141 /** 142 * The territory of a county, parish or other division of a state or province. 143 */ 144 COUNTY, 145 /** 146 * The territory of a state, province, department or other division of a sovereign country. 147 */ 148 PROVINCE, 149 /** 150 * A grouping of resources (personnel, material, or places) to be used for scheduling purposes. May be a pool of like-type resources, a team, or combination of personnel, material and places. 151 */ 152 RGRP, 153 /** 154 * added to help the parsers 155 */ 156 NULL; 157 public static V3EntityClass fromCode(String codeString) throws FHIRException { 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("ENT".equals(codeString)) 161 return ENT; 162 if ("HCE".equals(codeString)) 163 return HCE; 164 if ("LIV".equals(codeString)) 165 return LIV; 166 if ("NLIV".equals(codeString)) 167 return NLIV; 168 if ("ANM".equals(codeString)) 169 return ANM; 170 if ("MIC".equals(codeString)) 171 return MIC; 172 if ("PLNT".equals(codeString)) 173 return PLNT; 174 if ("PSN".equals(codeString)) 175 return PSN; 176 if ("MAT".equals(codeString)) 177 return MAT; 178 if ("CHEM".equals(codeString)) 179 return CHEM; 180 if ("FOOD".equals(codeString)) 181 return FOOD; 182 if ("MMAT".equals(codeString)) 183 return MMAT; 184 if ("CONT".equals(codeString)) 185 return CONT; 186 if ("HOLD".equals(codeString)) 187 return HOLD; 188 if ("DEV".equals(codeString)) 189 return DEV; 190 if ("CER".equals(codeString)) 191 return CER; 192 if ("MODDV".equals(codeString)) 193 return MODDV; 194 if ("ORG".equals(codeString)) 195 return ORG; 196 if ("PUB".equals(codeString)) 197 return PUB; 198 if ("STATE".equals(codeString)) 199 return STATE; 200 if ("NAT".equals(codeString)) 201 return NAT; 202 if ("PLC".equals(codeString)) 203 return PLC; 204 if ("CITY".equals(codeString)) 205 return CITY; 206 if ("COUNTRY".equals(codeString)) 207 return COUNTRY; 208 if ("COUNTY".equals(codeString)) 209 return COUNTY; 210 if ("PROVINCE".equals(codeString)) 211 return PROVINCE; 212 if ("RGRP".equals(codeString)) 213 return RGRP; 214 throw new FHIRException("Unknown V3EntityClass code '"+codeString+"'"); 215 } 216 public String toCode() { 217 switch (this) { 218 case ENT: return "ENT"; 219 case HCE: return "HCE"; 220 case LIV: return "LIV"; 221 case NLIV: return "NLIV"; 222 case ANM: return "ANM"; 223 case MIC: return "MIC"; 224 case PLNT: return "PLNT"; 225 case PSN: return "PSN"; 226 case MAT: return "MAT"; 227 case CHEM: return "CHEM"; 228 case FOOD: return "FOOD"; 229 case MMAT: return "MMAT"; 230 case CONT: return "CONT"; 231 case HOLD: return "HOLD"; 232 case DEV: return "DEV"; 233 case CER: return "CER"; 234 case MODDV: return "MODDV"; 235 case ORG: return "ORG"; 236 case PUB: return "PUB"; 237 case STATE: return "STATE"; 238 case NAT: return "NAT"; 239 case PLC: return "PLC"; 240 case CITY: return "CITY"; 241 case COUNTRY: return "COUNTRY"; 242 case COUNTY: return "COUNTY"; 243 case PROVINCE: return "PROVINCE"; 244 case RGRP: return "RGRP"; 245 case NULL: return null; 246 default: return "?"; 247 } 248 } 249 public String getSystem() { 250 return "http://hl7.org/fhir/v3/EntityClass"; 251 } 252 public String getDefinition() { 253 switch (this) { 254 case ENT: return "Corresponds to the Entity class"; 255 case HCE: return "A health chart included to serve as a document receiving entity in the management of medical records."; 256 case LIV: return "Anything that essentially has the property of life, independent of current state (a dead human corpse is still essentially a living subject)."; 257 case NLIV: return "A subtype of living subject that includes all living things except the species Homo Sapiens."; 258 case ANM: return "A living subject from the animal kingdom."; 259 case MIC: return "All single celled living organisms including protozoa, bacteria, yeast, viruses, etc."; 260 case PLNT: return "A living subject from the order of plants."; 261 case PSN: return "A living subject of the species homo sapiens."; 262 case MAT: return "Any thing that has extension in space and mass, may be of living or non-living origin."; 263 case CHEM: return "A substance that is fully defined by an organic or inorganic chemical formula, includes mixtures of other chemical substances. Refine using, e.g., IUPAC codes."; 264 case FOOD: return "Naturally occurring, processed or manufactured entities that are primarily used as food for humans and animals."; 265 case MMAT: return "Corresponds to the ManufacturedMaterial class"; 266 case CONT: return "A container of other entities."; 267 case HOLD: return "A type of container that can hold other containers or other holders."; 268 case DEV: return "A subtype of ManufacturedMaterial used in an activity, without being substantially changed through that activity. The kind of device is identified by the code attribute inherited from Entity.\r\n\n \n Usage: This includes durable (reusable) medical equipment as well as disposable equipment."; 269 case CER: return "A physical artifact that stores information about the granting of authorization."; 270 case MODDV: return "Class to contain unique attributes of diagnostic imaging equipment."; 271 case ORG: return "A social or legal structure formed by human beings."; 272 case PUB: return "An agency of the people of a state often assuming some authority over a certain matter. Includes government, governmental agencies, associations."; 273 case STATE: return "A politically organized body of people bonded by territory, culture, or ethnicity, having sovereignty (to a certain extent) granted by other states (enclosing or neighboring states). This includes countries (nations), provinces (e.g., one of the United States of America or a French departement), counties or municipalities. Refine using, e.g., ISO country codes, FIPS-PUB state codes, etc."; 274 case NAT: return "A politically organized body of people bonded by territory and known as a nation."; 275 case PLC: return "A physical place or site with its containing structure. May be natural or man-made. The geographic position of a place may or may not be constant."; 276 case CITY: return "The territory of a city, town or other municipality."; 277 case COUNTRY: return "The territory of a sovereign nation."; 278 case COUNTY: return "The territory of a county, parish or other division of a state or province."; 279 case PROVINCE: return "The territory of a state, province, department or other division of a sovereign country."; 280 case RGRP: return "A grouping of resources (personnel, material, or places) to be used for scheduling purposes. May be a pool of like-type resources, a team, or combination of personnel, material and places."; 281 case NULL: return null; 282 default: return "?"; 283 } 284 } 285 public String getDisplay() { 286 switch (this) { 287 case ENT: return "entity"; 288 case HCE: return "health chart entity"; 289 case LIV: return "living subject"; 290 case NLIV: return "non-person living subject"; 291 case ANM: return "animal"; 292 case MIC: return "microorganism"; 293 case PLNT: return "plant"; 294 case PSN: return "person"; 295 case MAT: return "material"; 296 case CHEM: return "chemical substance"; 297 case FOOD: return "food"; 298 case MMAT: return "manufactured material"; 299 case CONT: return "container"; 300 case HOLD: return "holder"; 301 case DEV: return "device"; 302 case CER: return "certificate representation"; 303 case MODDV: return "imaging modality"; 304 case ORG: return "organization"; 305 case PUB: return "public institution"; 306 case STATE: return "state"; 307 case NAT: return "Nation"; 308 case PLC: return "place"; 309 case CITY: return "city or town"; 310 case COUNTRY: return "country"; 311 case COUNTY: return "county or parish"; 312 case PROVINCE: return "state or province"; 313 case RGRP: return "group"; 314 case NULL: return null; 315 default: return "?"; 316 } 317 } 318 319 320}