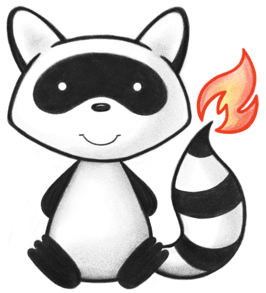
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3EntityNameUse { 041 042 /** 043 * Identifies the different representations of a name. The representation may affect how the name is used. (E.g. use of Ideographic for formal communications.) 044 */ 045 _NAMEREPRESENTATIONUSE, 046 /** 047 * Alphabetic transcription of name (Japanese: romaji) 048 */ 049 ABC, 050 /** 051 * Ideographic representation of name (e.g., Japanese kanji, Chinese characters) 052 */ 053 IDE, 054 /** 055 * Syllabic transcription of name (e.g., Japanese kana, Korean hangul) 056 */ 057 SYL, 058 /** 059 * A name assigned to a person. Reasons some organizations assign alternate names may include not knowing the person's name, or to maintain anonymity. Some, but not necessarily all, of the name types that people call "alias" may fit into this category. 060 */ 061 ASGN, 062 /** 063 * As recorded on a license, record, certificate, etc. (only if different from legal name) 064 */ 065 C, 066 /** 067 * e.g. Chief Red Cloud 068 */ 069 I, 070 /** 071 * Known as/conventional/the one you use 072 */ 073 L, 074 /** 075 * Definition:The formal name as registered in an official (government) registry, but which name might not be commonly used. Particularly used in countries with a law system based on Napoleonic law. 076 */ 077 OR, 078 /** 079 * A self asserted name that the person is using or has used. 080 */ 081 P, 082 /** 083 * Includes writer's pseudonym, stage name, etc 084 */ 085 A, 086 /** 087 * e.g. Sister Mary Francis, Brother John 088 */ 089 R, 090 /** 091 * A name intended for use in searching or matching. 092 */ 093 SRCH, 094 /** 095 * A name spelled phonetically. 096 097 There are a variety of phonetic spelling algorithms. This code value does not distinguish between these.Discussion: 098 */ 099 PHON, 100 /** 101 * A name spelled according to the SoundEx algorithm. 102 */ 103 SNDX, 104 /** 105 * added to help the parsers 106 */ 107 NULL; 108 public static V3EntityNameUse fromCode(String codeString) throws FHIRException { 109 if (codeString == null || "".equals(codeString)) 110 return null; 111 if ("_NameRepresentationUse".equals(codeString)) 112 return _NAMEREPRESENTATIONUSE; 113 if ("ABC".equals(codeString)) 114 return ABC; 115 if ("IDE".equals(codeString)) 116 return IDE; 117 if ("SYL".equals(codeString)) 118 return SYL; 119 if ("ASGN".equals(codeString)) 120 return ASGN; 121 if ("C".equals(codeString)) 122 return C; 123 if ("I".equals(codeString)) 124 return I; 125 if ("L".equals(codeString)) 126 return L; 127 if ("OR".equals(codeString)) 128 return OR; 129 if ("P".equals(codeString)) 130 return P; 131 if ("A".equals(codeString)) 132 return A; 133 if ("R".equals(codeString)) 134 return R; 135 if ("SRCH".equals(codeString)) 136 return SRCH; 137 if ("PHON".equals(codeString)) 138 return PHON; 139 if ("SNDX".equals(codeString)) 140 return SNDX; 141 throw new FHIRException("Unknown V3EntityNameUse code '"+codeString+"'"); 142 } 143 public String toCode() { 144 switch (this) { 145 case _NAMEREPRESENTATIONUSE: return "_NameRepresentationUse"; 146 case ABC: return "ABC"; 147 case IDE: return "IDE"; 148 case SYL: return "SYL"; 149 case ASGN: return "ASGN"; 150 case C: return "C"; 151 case I: return "I"; 152 case L: return "L"; 153 case OR: return "OR"; 154 case P: return "P"; 155 case A: return "A"; 156 case R: return "R"; 157 case SRCH: return "SRCH"; 158 case PHON: return "PHON"; 159 case SNDX: return "SNDX"; 160 case NULL: return null; 161 default: return "?"; 162 } 163 } 164 public String getSystem() { 165 return "http://hl7.org/fhir/v3/EntityNameUse"; 166 } 167 public String getDefinition() { 168 switch (this) { 169 case _NAMEREPRESENTATIONUSE: return "Identifies the different representations of a name. The representation may affect how the name is used. (E.g. use of Ideographic for formal communications.)"; 170 case ABC: return "Alphabetic transcription of name (Japanese: romaji)"; 171 case IDE: return "Ideographic representation of name (e.g., Japanese kanji, Chinese characters)"; 172 case SYL: return "Syllabic transcription of name (e.g., Japanese kana, Korean hangul)"; 173 case ASGN: return "A name assigned to a person. Reasons some organizations assign alternate names may include not knowing the person's name, or to maintain anonymity. Some, but not necessarily all, of the name types that people call \"alias\" may fit into this category."; 174 case C: return "As recorded on a license, record, certificate, etc. (only if different from legal name)"; 175 case I: return "e.g. Chief Red Cloud"; 176 case L: return "Known as/conventional/the one you use"; 177 case OR: return "Definition:The formal name as registered in an official (government) registry, but which name might not be commonly used. Particularly used in countries with a law system based on Napoleonic law."; 178 case P: return "A self asserted name that the person is using or has used."; 179 case A: return "Includes writer's pseudonym, stage name, etc"; 180 case R: return "e.g. Sister Mary Francis, Brother John"; 181 case SRCH: return "A name intended for use in searching or matching."; 182 case PHON: return "A name spelled phonetically.\r\n\n There are a variety of phonetic spelling algorithms. This code value does not distinguish between these.Discussion:"; 183 case SNDX: return "A name spelled according to the SoundEx algorithm."; 184 case NULL: return null; 185 default: return "?"; 186 } 187 } 188 public String getDisplay() { 189 switch (this) { 190 case _NAMEREPRESENTATIONUSE: return "NameRepresentationUse"; 191 case ABC: return "Alphabetic"; 192 case IDE: return "Ideographic"; 193 case SYL: return "Syllabic"; 194 case ASGN: return "assigned"; 195 case C: return "License"; 196 case I: return "Indigenous/Tribal"; 197 case L: return "Legal"; 198 case OR: return "official registry"; 199 case P: return "pseudonym"; 200 case A: return "Artist/Stage"; 201 case R: return "Religious"; 202 case SRCH: return "search"; 203 case PHON: return "phonetic"; 204 case SNDX: return "Soundex"; 205 case NULL: return null; 206 default: return "?"; 207 } 208 } 209 210 211}