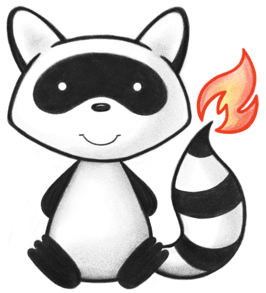
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3EntityNameUseR2 { 041 042 /** 043 * Description:A name that a person has assumed or has been assumed to them. 044 */ 045 ASSUMED, 046 /** 047 * Description:A name used in a Professional or Business context . Examples: Continuing to use a maiden name in a professional context, or using a stage performing name (some of these names are also pseudonyms) 048 */ 049 A, 050 /** 051 * Description:Anonymous assigned name (used to protect a persons identity for privacy reasons) 052 */ 053 ANON, 054 /** 055 * Description:e.g . Chief Red Cloud 056 */ 057 I, 058 /** 059 * Description:A non-official name by which the person is sometimes known. (This may also be used to record informal names such as a nickname) 060 */ 061 P, 062 /** 063 * Description:A name assumed as part of a religious vocation . e.g . Sister Mary Francis, Brother John 064 */ 065 R, 066 /** 067 * Description:Known as/conventional/the one you normally use 068 */ 069 C, 070 /** 071 * Description:A name used prior to marriage.Note that marriage naming customs vary greatly around the world. This name use is for use by applications that collect and store maiden names. Though the concept of maiden name is often gender specific, the use of this term is not gender specific. The use of this term does not imply any particular history for a person's name, nor should the maiden name be determined algorithmically 072 */ 073 M, 074 /** 075 * Description:Identifies the different representations of a name . The representation may affect how the name is used . (E.g . use of Ideographic for formal communications.) 076 */ 077 NAMEREPRESENTATIONUSE, 078 /** 079 * Description:Alphabetic transcription of name in latin alphabet (Japanese: romaji) 080 */ 081 ABC, 082 /** 083 * Description:Ideographic representation of name (e.g., Japanese kanji, Chinese characters) 084 */ 085 IDE, 086 /** 087 * Description:Syllabic transcription of name (e.g., Japanese kana, Korean hangul) 088 */ 089 SYL, 090 /** 091 * Description:This name is no longer in use (note: Names may also carry valid time ranges . This code is used to cover the situations where it is known that the name is no longer valid, but no particular time range for its use is known) 092 */ 093 OLD, 094 /** 095 * Description:This name should no longer be used when interacting with the person (i.e . in addition to no longer being used, the name should not be even mentioned when interacting with the person)Note: applications are not required to compare names labeled "Do Not Use" and other names in order to eliminate name parts that are common between the other name and a name labeled "Do Not Use". 096 */ 097 DN, 098 /** 099 * Description:The formal name as registered in an official (government) registry, but which name might not be commonly used . May correspond to the concept of legal name 100 */ 101 OR, 102 /** 103 * Description:The name as understood by the data enterer, i.e. a close approximation of a phonetic spelling of the name, not based on a phonetic algorithm. 104 */ 105 PHON, 106 /** 107 * Description:A name intended for use in searching or matching. This is used when the name is incomplete and contains enough details for search matching, but not enough for other uses. 108 */ 109 SRCH, 110 /** 111 * Description:A temporary name. Note that a name valid time can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations. 112 */ 113 T, 114 /** 115 * added to help the parsers 116 */ 117 NULL; 118 public static V3EntityNameUseR2 fromCode(String codeString) throws FHIRException { 119 if (codeString == null || "".equals(codeString)) 120 return null; 121 if ("Assumed".equals(codeString)) 122 return ASSUMED; 123 if ("A".equals(codeString)) 124 return A; 125 if ("ANON".equals(codeString)) 126 return ANON; 127 if ("I".equals(codeString)) 128 return I; 129 if ("P".equals(codeString)) 130 return P; 131 if ("R".equals(codeString)) 132 return R; 133 if ("C".equals(codeString)) 134 return C; 135 if ("M".equals(codeString)) 136 return M; 137 if ("NameRepresentationUse".equals(codeString)) 138 return NAMEREPRESENTATIONUSE; 139 if ("ABC".equals(codeString)) 140 return ABC; 141 if ("IDE".equals(codeString)) 142 return IDE; 143 if ("SYL".equals(codeString)) 144 return SYL; 145 if ("OLD".equals(codeString)) 146 return OLD; 147 if ("DN".equals(codeString)) 148 return DN; 149 if ("OR".equals(codeString)) 150 return OR; 151 if ("PHON".equals(codeString)) 152 return PHON; 153 if ("SRCH".equals(codeString)) 154 return SRCH; 155 if ("T".equals(codeString)) 156 return T; 157 throw new FHIRException("Unknown V3EntityNameUseR2 code '"+codeString+"'"); 158 } 159 public String toCode() { 160 switch (this) { 161 case ASSUMED: return "Assumed"; 162 case A: return "A"; 163 case ANON: return "ANON"; 164 case I: return "I"; 165 case P: return "P"; 166 case R: return "R"; 167 case C: return "C"; 168 case M: return "M"; 169 case NAMEREPRESENTATIONUSE: return "NameRepresentationUse"; 170 case ABC: return "ABC"; 171 case IDE: return "IDE"; 172 case SYL: return "SYL"; 173 case OLD: return "OLD"; 174 case DN: return "DN"; 175 case OR: return "OR"; 176 case PHON: return "PHON"; 177 case SRCH: return "SRCH"; 178 case T: return "T"; 179 case NULL: return null; 180 default: return "?"; 181 } 182 } 183 public String getSystem() { 184 return "http://hl7.org/fhir/v3/EntityNameUseR2"; 185 } 186 public String getDefinition() { 187 switch (this) { 188 case ASSUMED: return "Description:A name that a person has assumed or has been assumed to them."; 189 case A: return "Description:A name used in a Professional or Business context . Examples: Continuing to use a maiden name in a professional context, or using a stage performing name (some of these names are also pseudonyms)"; 190 case ANON: return "Description:Anonymous assigned name (used to protect a persons identity for privacy reasons)"; 191 case I: return "Description:e.g . Chief Red Cloud"; 192 case P: return "Description:A non-official name by which the person is sometimes known. (This may also be used to record informal names such as a nickname)"; 193 case R: return "Description:A name assumed as part of a religious vocation . e.g . Sister Mary Francis, Brother John"; 194 case C: return "Description:Known as/conventional/the one you normally use"; 195 case M: return "Description:A name used prior to marriage.Note that marriage naming customs vary greatly around the world. This name use is for use by applications that collect and store maiden names. Though the concept of maiden name is often gender specific, the use of this term is not gender specific. The use of this term does not imply any particular history for a person's name, nor should the maiden name be determined algorithmically"; 196 case NAMEREPRESENTATIONUSE: return "Description:Identifies the different representations of a name . The representation may affect how the name is used . (E.g . use of Ideographic for formal communications.)"; 197 case ABC: return "Description:Alphabetic transcription of name in latin alphabet (Japanese: romaji)"; 198 case IDE: return "Description:Ideographic representation of name (e.g., Japanese kanji, Chinese characters)"; 199 case SYL: return "Description:Syllabic transcription of name (e.g., Japanese kana, Korean hangul)"; 200 case OLD: return "Description:This name is no longer in use (note: Names may also carry valid time ranges . This code is used to cover the situations where it is known that the name is no longer valid, but no particular time range for its use is known)"; 201 case DN: return "Description:This name should no longer be used when interacting with the person (i.e . in addition to no longer being used, the name should not be even mentioned when interacting with the person)Note: applications are not required to compare names labeled \"Do Not Use\" and other names in order to eliminate name parts that are common between the other name and a name labeled \"Do Not Use\"."; 202 case OR: return "Description:The formal name as registered in an official (government) registry, but which name might not be commonly used . May correspond to the concept of legal name"; 203 case PHON: return "Description:The name as understood by the data enterer, i.e. a close approximation of a phonetic spelling of the name, not based on a phonetic algorithm."; 204 case SRCH: return "Description:A name intended for use in searching or matching. This is used when the name is incomplete and contains enough details for search matching, but not enough for other uses."; 205 case T: return "Description:A temporary name. Note that a name valid time can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 public String getDisplay() { 211 switch (this) { 212 case ASSUMED: return "Assumed"; 213 case A: return "business name"; 214 case ANON: return "Anonymous"; 215 case I: return "Indigenous/Tribal"; 216 case P: return "Other/Pseudonym/Alias"; 217 case R: return "religious"; 218 case C: return "customary"; 219 case M: return "maiden name"; 220 case NAMEREPRESENTATIONUSE: return "NameRepresentationUse"; 221 case ABC: return "alphabetic"; 222 case IDE: return "ideographic"; 223 case SYL: return "syllabic"; 224 case OLD: return "no longer in use"; 225 case DN: return "do not use"; 226 case OR: return "official registry name"; 227 case PHON: return "phonetic"; 228 case SRCH: return "search"; 229 case T: return "temporary"; 230 case NULL: return null; 231 default: return "?"; 232 } 233 } 234 235 236}