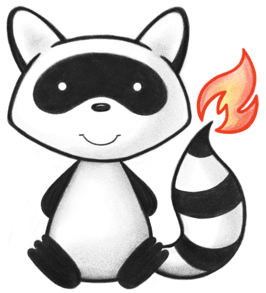
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3EntityRisk { 041 042 /** 043 * A danger that can be associated with certain living subjects, including humans. 044 */ 045 AGG, 046 /** 047 * The dangers associated with normal biological materials. I.e. potential risk of unknown infections. Routine biological materials from living subjects. 048 */ 049 BIO, 050 /** 051 * Material is corrosive and may cause severe injury to skin, mucous membranes and eyes. Avoid any unprotected contact. 052 */ 053 COR, 054 /** 055 * The entity is at risk for escaping from containment or control. 056 */ 057 ESC, 058 /** 059 * Material is highly inflammable and in certain mixtures (with air) may lead to explosions. Keep away from fire, sparks and excessive heat. 060 */ 061 IFL, 062 /** 063 * Material is an explosive mixture. Keep away from fire, sparks, and heat. 064 */ 065 EXP, 066 /** 067 * Material known to be infectious with human pathogenic microorganisms. Those who handle this material must take precautions for their protection. 068 */ 069 INF, 070 /** 071 * Material contains microorganisms that is an environmental hazard. Must be handled with special care. 072 */ 073 BHZ, 074 /** 075 * Material is solid and sharp (e.g., cannulas). Dispose in hard container. 076 */ 077 INJ, 078 /** 079 * Material is poisonous to humans and/or animals. Special care must be taken to avoid incorporation, even of small amounts. 080 */ 081 POI, 082 /** 083 * Material is a source for ionizing radiation and must be handled with special care to avoid injury of those who handle it and to avoid environmental hazards. 084 */ 085 RAD, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 public static V3EntityRisk fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("AGG".equals(codeString)) 094 return AGG; 095 if ("BIO".equals(codeString)) 096 return BIO; 097 if ("COR".equals(codeString)) 098 return COR; 099 if ("ESC".equals(codeString)) 100 return ESC; 101 if ("IFL".equals(codeString)) 102 return IFL; 103 if ("EXP".equals(codeString)) 104 return EXP; 105 if ("INF".equals(codeString)) 106 return INF; 107 if ("BHZ".equals(codeString)) 108 return BHZ; 109 if ("INJ".equals(codeString)) 110 return INJ; 111 if ("POI".equals(codeString)) 112 return POI; 113 if ("RAD".equals(codeString)) 114 return RAD; 115 throw new FHIRException("Unknown V3EntityRisk code '"+codeString+"'"); 116 } 117 public String toCode() { 118 switch (this) { 119 case AGG: return "AGG"; 120 case BIO: return "BIO"; 121 case COR: return "COR"; 122 case ESC: return "ESC"; 123 case IFL: return "IFL"; 124 case EXP: return "EXP"; 125 case INF: return "INF"; 126 case BHZ: return "BHZ"; 127 case INJ: return "INJ"; 128 case POI: return "POI"; 129 case RAD: return "RAD"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getSystem() { 135 return "http://hl7.org/fhir/v3/EntityRisk"; 136 } 137 public String getDefinition() { 138 switch (this) { 139 case AGG: return "A danger that can be associated with certain living subjects, including humans."; 140 case BIO: return "The dangers associated with normal biological materials. I.e. potential risk of unknown infections. Routine biological materials from living subjects."; 141 case COR: return "Material is corrosive and may cause severe injury to skin, mucous membranes and eyes. Avoid any unprotected contact."; 142 case ESC: return "The entity is at risk for escaping from containment or control."; 143 case IFL: return "Material is highly inflammable and in certain mixtures (with air) may lead to explosions. Keep away from fire, sparks and excessive heat."; 144 case EXP: return "Material is an explosive mixture. Keep away from fire, sparks, and heat."; 145 case INF: return "Material known to be infectious with human pathogenic microorganisms. Those who handle this material must take precautions for their protection."; 146 case BHZ: return "Material contains microorganisms that is an environmental hazard. Must be handled with special care."; 147 case INJ: return "Material is solid and sharp (e.g., cannulas). Dispose in hard container."; 148 case POI: return "Material is poisonous to humans and/or animals. Special care must be taken to avoid incorporation, even of small amounts."; 149 case RAD: return "Material is a source for ionizing radiation and must be handled with special care to avoid injury of those who handle it and to avoid environmental hazards."; 150 case NULL: return null; 151 default: return "?"; 152 } 153 } 154 public String getDisplay() { 155 switch (this) { 156 case AGG: return "aggressive"; 157 case BIO: return "Biological"; 158 case COR: return "Corrosive"; 159 case ESC: return "Escape Risk"; 160 case IFL: return "inflammable"; 161 case EXP: return "explosive"; 162 case INF: return "infectious"; 163 case BHZ: return "biohazard"; 164 case INJ: return "injury hazard"; 165 case POI: return "poison"; 166 case RAD: return "radioactive"; 167 case NULL: return null; 168 default: return "?"; 169 } 170 } 171 172 173}