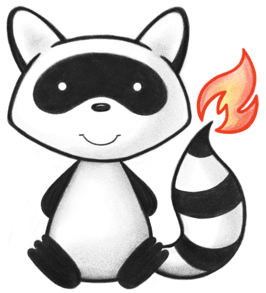
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3GTSAbbreviation { 041 042 /** 043 * Every morning at institution specified times. 044 */ 045 AM, 046 /** 047 * Two times a day at institution specified time 048 */ 049 BID, 050 /** 051 * Regular business days (Monday to Friday excluding holidays) 052 */ 053 JB, 054 /** 055 * Regular weekends (Saturday and Sunday excluding holidays) 056 */ 057 JE, 058 /** 059 * Holidays 060 */ 061 JH, 062 /** 063 * Christian Holidays (Roman/Gregorian [Western] Tradition.) 064 */ 065 _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN, 066 /** 067 * Easter Sunday. The Easter date is a rather complex calculation based on Astronomical tables describing full moon dates. Details can be found at [http://www.assa.org.au/edm.html, and http://aa.usno.navy.mil/AA/faq/docs/easter.html]. Note that the Christian Orthodox Holidays are based on the Julian calendar. 068 */ 069 JHCHREAS, 070 /** 071 * Good Friday, is the Friday right before Easter Sunday. 072 */ 073 JHCHRGFR, 074 /** 075 * New Year's Day (January 1) 076 */ 077 JHCHRNEW, 078 /** 079 * Pentecost Sunday, is seven weeks after Easter (the 50th day of Easter). 080 */ 081 JHCHRPEN, 082 /** 083 * Christmas Eve (December 24) 084 */ 085 JHCHRXME, 086 /** 087 * Christmas Day (December 25) 088 */ 089 JHCHRXMS, 090 /** 091 * Description:The Netherlands National Holidays. 092 */ 093 JHNNL, 094 /** 095 * Description:Liberation day (May 5 every five years) 096 */ 097 JHNNLLD, 098 /** 099 * Description:Queen's day (April 30) 100 */ 101 JHNNLQD, 102 /** 103 * Description:Sinterklaas (December 5) 104 */ 105 JHNNLSK, 106 /** 107 * United States National Holidays (public holidays for federal employees established by U.S. Federal law 5 U.S.C. 6103). 108 */ 109 JHNUS, 110 /** 111 * Columbus Day, the second Monday in October. 112 */ 113 JHNUSCLM, 114 /** 115 * Independence Day (4th of July) 116 */ 117 JHNUSIND, 118 /** 119 * Alternative Monday after 4th of July Weekend [5 U.S.C. 6103(b)]. 120 */ 121 JHNUSIND1, 122 /** 123 * Alternative Friday before 4th of July Weekend [5 U.S.C. 6103(b)]. 124 */ 125 JHNUSIND5, 126 /** 127 * Labor Day, the first Monday in September. 128 */ 129 JHNUSLBR, 130 /** 131 * Memorial Day, the last Monday in May. 132 */ 133 JHNUSMEM, 134 /** 135 * Friday before Memorial Day Weekend 136 */ 137 JHNUSMEM5, 138 /** 139 * Saturday of Memorial Day Weekend 140 */ 141 JHNUSMEM6, 142 /** 143 * Dr. Martin Luther King, Jr. Day, the third Monday in January. 144 */ 145 JHNUSMLK, 146 /** 147 * Washington's Birthday (Presidential Day) the third Monday in February. 148 */ 149 JHNUSPRE, 150 /** 151 * Thanksgiving Day, the fourth Thursday in November. 152 */ 153 JHNUSTKS, 154 /** 155 * Friday after Thanksgiving. 156 */ 157 JHNUSTKS5, 158 /** 159 * Veteran's Day, November 11. 160 */ 161 JHNUSVET, 162 /** 163 * Every afternoon at institution specified times. 164 */ 165 PM, 166 /** 167 * Every 4 hours at institution specified time 168 */ 169 Q4H, 170 /** 171 * Every 6 hours at institution specified time 172 */ 173 Q6H, 174 /** 175 * Every day at institution specified times. 176 */ 177 QD, 178 /** 179 * Four times a day at institution specified time 180 */ 181 QID, 182 /** 183 * Every other day at institution specified times. 184 */ 185 QOD, 186 /** 187 * Three times a day at institution specified time 188 */ 189 TID, 190 /** 191 * added to help the parsers 192 */ 193 NULL; 194 public static V3GTSAbbreviation fromCode(String codeString) throws FHIRException { 195 if (codeString == null || "".equals(codeString)) 196 return null; 197 if ("AM".equals(codeString)) 198 return AM; 199 if ("BID".equals(codeString)) 200 return BID; 201 if ("JB".equals(codeString)) 202 return JB; 203 if ("JE".equals(codeString)) 204 return JE; 205 if ("JH".equals(codeString)) 206 return JH; 207 if ("_GTSAbbreviationHolidaysChristianRoman".equals(codeString)) 208 return _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN; 209 if ("JHCHREAS".equals(codeString)) 210 return JHCHREAS; 211 if ("JHCHRGFR".equals(codeString)) 212 return JHCHRGFR; 213 if ("JHCHRNEW".equals(codeString)) 214 return JHCHRNEW; 215 if ("JHCHRPEN".equals(codeString)) 216 return JHCHRPEN; 217 if ("JHCHRXME".equals(codeString)) 218 return JHCHRXME; 219 if ("JHCHRXMS".equals(codeString)) 220 return JHCHRXMS; 221 if ("JHNNL".equals(codeString)) 222 return JHNNL; 223 if ("JHNNLLD".equals(codeString)) 224 return JHNNLLD; 225 if ("JHNNLQD".equals(codeString)) 226 return JHNNLQD; 227 if ("JHNNLSK".equals(codeString)) 228 return JHNNLSK; 229 if ("JHNUS".equals(codeString)) 230 return JHNUS; 231 if ("JHNUSCLM".equals(codeString)) 232 return JHNUSCLM; 233 if ("JHNUSIND".equals(codeString)) 234 return JHNUSIND; 235 if ("JHNUSIND1".equals(codeString)) 236 return JHNUSIND1; 237 if ("JHNUSIND5".equals(codeString)) 238 return JHNUSIND5; 239 if ("JHNUSLBR".equals(codeString)) 240 return JHNUSLBR; 241 if ("JHNUSMEM".equals(codeString)) 242 return JHNUSMEM; 243 if ("JHNUSMEM5".equals(codeString)) 244 return JHNUSMEM5; 245 if ("JHNUSMEM6".equals(codeString)) 246 return JHNUSMEM6; 247 if ("JHNUSMLK".equals(codeString)) 248 return JHNUSMLK; 249 if ("JHNUSPRE".equals(codeString)) 250 return JHNUSPRE; 251 if ("JHNUSTKS".equals(codeString)) 252 return JHNUSTKS; 253 if ("JHNUSTKS5".equals(codeString)) 254 return JHNUSTKS5; 255 if ("JHNUSVET".equals(codeString)) 256 return JHNUSVET; 257 if ("PM".equals(codeString)) 258 return PM; 259 if ("Q4H".equals(codeString)) 260 return Q4H; 261 if ("Q6H".equals(codeString)) 262 return Q6H; 263 if ("QD".equals(codeString)) 264 return QD; 265 if ("QID".equals(codeString)) 266 return QID; 267 if ("QOD".equals(codeString)) 268 return QOD; 269 if ("TID".equals(codeString)) 270 return TID; 271 throw new FHIRException("Unknown V3GTSAbbreviation code '"+codeString+"'"); 272 } 273 public String toCode() { 274 switch (this) { 275 case AM: return "AM"; 276 case BID: return "BID"; 277 case JB: return "JB"; 278 case JE: return "JE"; 279 case JH: return "JH"; 280 case _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN: return "_GTSAbbreviationHolidaysChristianRoman"; 281 case JHCHREAS: return "JHCHREAS"; 282 case JHCHRGFR: return "JHCHRGFR"; 283 case JHCHRNEW: return "JHCHRNEW"; 284 case JHCHRPEN: return "JHCHRPEN"; 285 case JHCHRXME: return "JHCHRXME"; 286 case JHCHRXMS: return "JHCHRXMS"; 287 case JHNNL: return "JHNNL"; 288 case JHNNLLD: return "JHNNLLD"; 289 case JHNNLQD: return "JHNNLQD"; 290 case JHNNLSK: return "JHNNLSK"; 291 case JHNUS: return "JHNUS"; 292 case JHNUSCLM: return "JHNUSCLM"; 293 case JHNUSIND: return "JHNUSIND"; 294 case JHNUSIND1: return "JHNUSIND1"; 295 case JHNUSIND5: return "JHNUSIND5"; 296 case JHNUSLBR: return "JHNUSLBR"; 297 case JHNUSMEM: return "JHNUSMEM"; 298 case JHNUSMEM5: return "JHNUSMEM5"; 299 case JHNUSMEM6: return "JHNUSMEM6"; 300 case JHNUSMLK: return "JHNUSMLK"; 301 case JHNUSPRE: return "JHNUSPRE"; 302 case JHNUSTKS: return "JHNUSTKS"; 303 case JHNUSTKS5: return "JHNUSTKS5"; 304 case JHNUSVET: return "JHNUSVET"; 305 case PM: return "PM"; 306 case Q4H: return "Q4H"; 307 case Q6H: return "Q6H"; 308 case QD: return "QD"; 309 case QID: return "QID"; 310 case QOD: return "QOD"; 311 case TID: return "TID"; 312 case NULL: return null; 313 default: return "?"; 314 } 315 } 316 public String getSystem() { 317 return "http://hl7.org/fhir/v3/GTSAbbreviation"; 318 } 319 public String getDefinition() { 320 switch (this) { 321 case AM: return "Every morning at institution specified times."; 322 case BID: return "Two times a day at institution specified time"; 323 case JB: return "Regular business days (Monday to Friday excluding holidays)"; 324 case JE: return "Regular weekends (Saturday and Sunday excluding holidays)"; 325 case JH: return "Holidays"; 326 case _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN: return "Christian Holidays (Roman/Gregorian [Western] Tradition.)"; 327 case JHCHREAS: return "Easter Sunday. The Easter date is a rather complex calculation based on Astronomical tables describing full moon dates. Details can be found at [http://www.assa.org.au/edm.html, and http://aa.usno.navy.mil/AA/faq/docs/easter.html]. Note that the Christian Orthodox Holidays are based on the Julian calendar."; 328 case JHCHRGFR: return "Good Friday, is the Friday right before Easter Sunday."; 329 case JHCHRNEW: return "New Year's Day (January 1)"; 330 case JHCHRPEN: return "Pentecost Sunday, is seven weeks after Easter (the 50th day of Easter)."; 331 case JHCHRXME: return "Christmas Eve (December 24)"; 332 case JHCHRXMS: return "Christmas Day (December 25)"; 333 case JHNNL: return "Description:The Netherlands National Holidays."; 334 case JHNNLLD: return "Description:Liberation day (May 5 every five years)"; 335 case JHNNLQD: return "Description:Queen's day (April 30)"; 336 case JHNNLSK: return "Description:Sinterklaas (December 5)"; 337 case JHNUS: return "United States National Holidays (public holidays for federal employees established by U.S. Federal law 5 U.S.C. 6103)."; 338 case JHNUSCLM: return "Columbus Day, the second Monday in October."; 339 case JHNUSIND: return "Independence Day (4th of July)"; 340 case JHNUSIND1: return "Alternative Monday after 4th of July Weekend [5 U.S.C. 6103(b)]."; 341 case JHNUSIND5: return "Alternative Friday before 4th of July Weekend [5 U.S.C. 6103(b)]."; 342 case JHNUSLBR: return "Labor Day, the first Monday in September."; 343 case JHNUSMEM: return "Memorial Day, the last Monday in May."; 344 case JHNUSMEM5: return "Friday before Memorial Day Weekend"; 345 case JHNUSMEM6: return "Saturday of Memorial Day Weekend"; 346 case JHNUSMLK: return "Dr. Martin Luther King, Jr. Day, the third Monday in January."; 347 case JHNUSPRE: return "Washington's Birthday (Presidential Day) the third Monday in February."; 348 case JHNUSTKS: return "Thanksgiving Day, the fourth Thursday in November."; 349 case JHNUSTKS5: return "Friday after Thanksgiving."; 350 case JHNUSVET: return "Veteran's Day, November 11."; 351 case PM: return "Every afternoon at institution specified times."; 352 case Q4H: return "Every 4 hours at institution specified time"; 353 case Q6H: return "Every 6 hours at institution specified time"; 354 case QD: return "Every day at institution specified times."; 355 case QID: return "Four times a day at institution specified time"; 356 case QOD: return "Every other day at institution specified times."; 357 case TID: return "Three times a day at institution specified time"; 358 case NULL: return null; 359 default: return "?"; 360 } 361 } 362 public String getDisplay() { 363 switch (this) { 364 case AM: return "AM"; 365 case BID: return "BID"; 366 case JB: return "JB"; 367 case JE: return "JE"; 368 case JH: return "GTSAbbreviationHolidays"; 369 case _GTSABBREVIATIONHOLIDAYSCHRISTIANROMAN: return "GTSAbbreviationHolidaysChristianRoman"; 370 case JHCHREAS: return "JHCHREAS"; 371 case JHCHRGFR: return "JHCHRGFR"; 372 case JHCHRNEW: return "JHCHRNEW"; 373 case JHCHRPEN: return "JHCHRPEN"; 374 case JHCHRXME: return "JHCHRXME"; 375 case JHCHRXMS: return "JHCHRXMS"; 376 case JHNNL: return "The Netherlands National Holidays"; 377 case JHNNLLD: return "Liberation day (May 5 every five years)"; 378 case JHNNLQD: return "Queen's day (April 30)"; 379 case JHNNLSK: return "Sinterklaas (December 5)"; 380 case JHNUS: return "GTSAbbreviationHolidaysUSNational"; 381 case JHNUSCLM: return "JHNUSCLM"; 382 case JHNUSIND: return "JHNUSIND"; 383 case JHNUSIND1: return "JHNUSIND1"; 384 case JHNUSIND5: return "JHNUSIND5"; 385 case JHNUSLBR: return "JHNUSLBR"; 386 case JHNUSMEM: return "JHNUSMEM"; 387 case JHNUSMEM5: return "JHNUSMEM5"; 388 case JHNUSMEM6: return "JHNUSMEM6"; 389 case JHNUSMLK: return "JHNUSMLK"; 390 case JHNUSPRE: return "JHNUSPRE"; 391 case JHNUSTKS: return "JHNUSTKS"; 392 case JHNUSTKS5: return "JHNUSTKS5"; 393 case JHNUSVET: return "JHNUSVET"; 394 case PM: return "PM"; 395 case Q4H: return "Q4H"; 396 case Q6H: return "Q6H"; 397 case QD: return "QD"; 398 case QID: return "QID"; 399 case QOD: return "QOD"; 400 case TID: return "TID"; 401 case NULL: return null; 402 default: return "?"; 403 } 404 } 405 406 407}