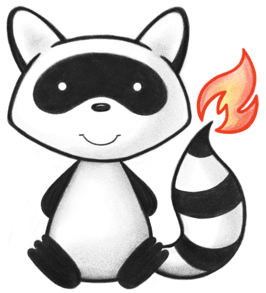
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3HL7UpdateMode { 041 042 /** 043 * Description:The item was (or is to be) added, having not been present immediately before. (If it is already present, this may be treated as an error condition.) 044 */ 045 A, 046 /** 047 * Description:The item was (or is to be) either added or replaced. 048 */ 049 AR, 050 /** 051 * Description:The item was (or is to be) removed (sometimes referred to as deleted). If the item is part of a collection, delete any matching items. 052 */ 053 D, 054 /** 055 * Description:This item is part of the identifying information for this object. 056 */ 057 K, 058 /** 059 * Description:There was (or is to be) no change to the item. This is primarily used when this element has not changed, but other attributes in the instance have changed. 060 */ 061 N, 062 /** 063 * Description:The item existed previously and has (or is to be) revised. (If an item does not already exist, this may be treated as an error condition.) 064 */ 065 R, 066 /** 067 * Description:This item provides enough information to allow a processing system to locate the full applicable record by identifying the object. 068 */ 069 REF, 070 /** 071 * Description:Description:</b>It is not specified whether or what kind of change has occurred to the item, or whether the item is present as a reference or identifying property. 072 */ 073 U, 074 /** 075 * These concepts apply when the element and/or message is updating a set of items. 076 */ 077 _SETUPDATEMODE, 078 /** 079 * Add the message element to the collection of items on the receiving system that correspond to the message element. 080 */ 081 ESA, 082 /** 083 * Change the item on the receiving system that corresponds to this message element; if a matching element does not exist, add a new one created with the values in the message. 084 */ 085 ESAC, 086 /** 087 * Change the item on the receiving system that corresponds to this message element; do not process if a matching element does not exist. 088 */ 089 ESC, 090 /** 091 * Delete the item on the receiving system that corresponds to this message element. 092 */ 093 ESD, 094 /** 095 * Description: AU: If this item exists, update it with these values. If it does not exist, create it with these values. If the item is part of the collection, update each item that matches this item, and if no items match, add a new item to the collection. 096 */ 097 AU, 098 /** 099 * Ignore this role, it is not relevant to the update. 100 */ 101 I, 102 /** 103 * Verify - this message element must match a value already in the receiving systems database in order to process the message. 104 */ 105 V, 106 /** 107 * added to help the parsers 108 */ 109 NULL; 110 public static V3HL7UpdateMode fromCode(String codeString) throws FHIRException { 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("A".equals(codeString)) 114 return A; 115 if ("AR".equals(codeString)) 116 return AR; 117 if ("D".equals(codeString)) 118 return D; 119 if ("K".equals(codeString)) 120 return K; 121 if ("N".equals(codeString)) 122 return N; 123 if ("R".equals(codeString)) 124 return R; 125 if ("REF".equals(codeString)) 126 return REF; 127 if ("U".equals(codeString)) 128 return U; 129 if ("_SetUpdateMode".equals(codeString)) 130 return _SETUPDATEMODE; 131 if ("ESA".equals(codeString)) 132 return ESA; 133 if ("ESAC".equals(codeString)) 134 return ESAC; 135 if ("ESC".equals(codeString)) 136 return ESC; 137 if ("ESD".equals(codeString)) 138 return ESD; 139 if ("AU".equals(codeString)) 140 return AU; 141 if ("I".equals(codeString)) 142 return I; 143 if ("V".equals(codeString)) 144 return V; 145 throw new FHIRException("Unknown V3HL7UpdateMode code '"+codeString+"'"); 146 } 147 public String toCode() { 148 switch (this) { 149 case A: return "A"; 150 case AR: return "AR"; 151 case D: return "D"; 152 case K: return "K"; 153 case N: return "N"; 154 case R: return "R"; 155 case REF: return "REF"; 156 case U: return "U"; 157 case _SETUPDATEMODE: return "_SetUpdateMode"; 158 case ESA: return "ESA"; 159 case ESAC: return "ESAC"; 160 case ESC: return "ESC"; 161 case ESD: return "ESD"; 162 case AU: return "AU"; 163 case I: return "I"; 164 case V: return "V"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 public String getSystem() { 170 return "http://hl7.org/fhir/v3/HL7UpdateMode"; 171 } 172 public String getDefinition() { 173 switch (this) { 174 case A: return "Description:The item was (or is to be) added, having not been present immediately before. (If it is already present, this may be treated as an error condition.)"; 175 case AR: return "Description:The item was (or is to be) either added or replaced."; 176 case D: return "Description:The item was (or is to be) removed (sometimes referred to as deleted). If the item is part of a collection, delete any matching items."; 177 case K: return "Description:This item is part of the identifying information for this object."; 178 case N: return "Description:There was (or is to be) no change to the item. This is primarily used when this element has not changed, but other attributes in the instance have changed."; 179 case R: return "Description:The item existed previously and has (or is to be) revised. (If an item does not already exist, this may be treated as an error condition.)"; 180 case REF: return "Description:This item provides enough information to allow a processing system to locate the full applicable record by identifying the object."; 181 case U: return "Description:Description:</b>It is not specified whether or what kind of change has occurred to the item, or whether the item is present as a reference or identifying property."; 182 case _SETUPDATEMODE: return "These concepts apply when the element and/or message is updating a set of items."; 183 case ESA: return "Add the message element to the collection of items on the receiving system that correspond to the message element."; 184 case ESAC: return "Change the item on the receiving system that corresponds to this message element; if a matching element does not exist, add a new one created with the values in the message."; 185 case ESC: return "Change the item on the receiving system that corresponds to this message element; do not process if a matching element does not exist."; 186 case ESD: return "Delete the item on the receiving system that corresponds to this message element."; 187 case AU: return "Description: AU: If this item exists, update it with these values. If it does not exist, create it with these values. If the item is part of the collection, update each item that matches this item, and if no items match, add a new item to the collection."; 188 case I: return "Ignore this role, it is not relevant to the update."; 189 case V: return "Verify - this message element must match a value already in the receiving systems database in order to process the message."; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getDisplay() { 195 switch (this) { 196 case A: return "Add"; 197 case AR: return "Add or Replace"; 198 case D: return "Remove"; 199 case K: return "Key"; 200 case N: return "No Change"; 201 case R: return "Replace"; 202 case REF: return "Reference"; 203 case U: return "Unknown"; 204 case _SETUPDATEMODE: return "SetUpdateMode"; 205 case ESA: return "Set Add"; 206 case ESAC: return "Set Add or Change"; 207 case ESC: return "Set Change"; 208 case ESD: return "Set Delete"; 209 case AU: return "Add or Update"; 210 case I: return "Ignore"; 211 case V: return "Verify"; 212 case NULL: return null; 213 default: return "?"; 214 } 215 } 216 217 218}