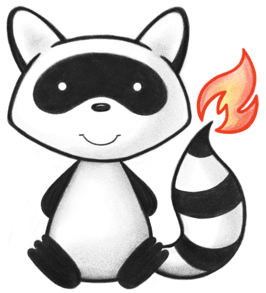
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3Hl7V3Conformance { 041 042 /** 043 * Description: Implementers receiving this property must not raise an error if the data is received, but will not perform any useful function with the data. This conformance level is not used in profiles or other artifacts that are specific to the "sender" or "initiator" of a communication. 044 */ 045 I, 046 /** 047 * Description: All implementers are prohibited from transmitting this content, and may raise an error if they receive it. 048 */ 049 NP, 050 /** 051 * Description: All implementers must support this property. I.e. they must be able to transmit, or to receive and usefully handle the concept. 052 */ 053 R, 054 /** 055 * Description: The element is considered "required" (i.e. must be supported) from the perspective of systems that consume instances, but is "undetermined" for systems that generate instances. Used only as part of specifications that define both initiator and consumer expectations. 056 */ 057 RC, 058 /** 059 * Description: The element is considered "required" (i.e. must be supported) from the perspective of systems that generate instances, but is "undetermined" for systems that consume instances. Used only as part of specifications that define both initiator and consumer expectations. 060 */ 061 RI, 062 /** 063 * Description: The conformance expectations for this element have not yet been determined. 064 */ 065 U, 066 /** 067 * added to help the parsers 068 */ 069 NULL; 070 public static V3Hl7V3Conformance fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("I".equals(codeString)) 074 return I; 075 if ("NP".equals(codeString)) 076 return NP; 077 if ("R".equals(codeString)) 078 return R; 079 if ("RC".equals(codeString)) 080 return RC; 081 if ("RI".equals(codeString)) 082 return RI; 083 if ("U".equals(codeString)) 084 return U; 085 throw new FHIRException("Unknown V3Hl7V3Conformance code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case I: return "I"; 090 case NP: return "NP"; 091 case R: return "R"; 092 case RC: return "RC"; 093 case RI: return "RI"; 094 case U: return "U"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getSystem() { 100 return "http://hl7.org/fhir/v3/hl7V3Conformance"; 101 } 102 public String getDefinition() { 103 switch (this) { 104 case I: return "Description: Implementers receiving this property must not raise an error if the data is received, but will not perform any useful function with the data. This conformance level is not used in profiles or other artifacts that are specific to the \"sender\" or \"initiator\" of a communication."; 105 case NP: return "Description: All implementers are prohibited from transmitting this content, and may raise an error if they receive it."; 106 case R: return "Description: All implementers must support this property. I.e. they must be able to transmit, or to receive and usefully handle the concept."; 107 case RC: return "Description: The element is considered \"required\" (i.e. must be supported) from the perspective of systems that consume instances, but is \"undetermined\" for systems that generate instances. Used only as part of specifications that define both initiator and consumer expectations."; 108 case RI: return "Description: The element is considered \"required\" (i.e. must be supported) from the perspective of systems that generate instances, but is \"undetermined\" for systems that consume instances. Used only as part of specifications that define both initiator and consumer expectations."; 109 case U: return "Description: The conformance expectations for this element have not yet been determined."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case I: return "ignored"; 117 case NP: return "not permitted"; 118 case R: return "required"; 119 case RC: return "required for consumer"; 120 case RI: return "required for initiator"; 121 case U: return "undetermined"; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 127 128}