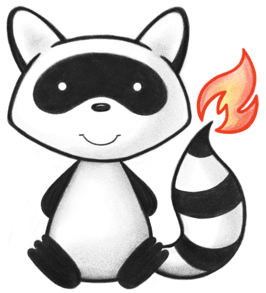
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3HtmlLinkType { 041 042 /** 043 * Designates substitute versions for the document in which the link occurs. When used together with the lang attribute, it implies a translated version of the document. When used together with the media attribute, it implies a version designed for a different medium (or media). 044 */ 045 ALTERNATE, 046 /** 047 * Refers to a document serving as an appendix in a collection of documents. 048 */ 049 APPENDIX, 050 /** 051 * Refers to a bookmark. A bookmark is a link to a key entry point within an extended document. The title attribute may be used, for example, to label the bookmark. Note that several bookmarks may be defined in each document. 052 */ 053 BOOKMARK, 054 /** 055 * Refers to a document serving as a chapter in a collection of documents. 056 */ 057 CHAPTER, 058 /** 059 * Refers to a document serving as a table of contents. Some user agents also support the synonym ToC (from "Table of Contents"). 060 */ 061 CONTENTS, 062 /** 063 * Refers to a copyright statement for the current document. 064 */ 065 COPYRIGHT, 066 /** 067 * Refers to a document providing a glossary of terms that pertain to the current document. 068 */ 069 GLOSSARY, 070 /** 071 * Refers to a document offering help (more information, links to other sources of information, etc.). 072 */ 073 HELP, 074 /** 075 * Refers to a document providing an index for the current document. 076 */ 077 INDEX, 078 /** 079 * Refers to the next document in a linear sequence of documents. User agents may choose to preload the "next" document, to reduce the perceived load time. 080 */ 081 NEXT, 082 /** 083 * Refers to the previous document in an ordered series of documents. Some user agents also support the synonym "Previous". 084 */ 085 PREV, 086 /** 087 * Refers to a document serving as a section in a collection of documents. 088 */ 089 SECTION, 090 /** 091 * Refers to the first document in a collection of documents. This link type tells search engines which document is considered by the author to be the starting point of the collection. 092 */ 093 START, 094 /** 095 * Refers to an external style sheet. See the section on external style sheets for details. This is used together with the link type "Alternate" for user-selectable alternate style sheets. 096 */ 097 STYLESHEET, 098 /** 099 * Refers to a document serving as a subsection in a collection of documents. 100 */ 101 SUBSECTION, 102 /** 103 * added to help the parsers 104 */ 105 NULL; 106 public static V3HtmlLinkType fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("alternate".equals(codeString)) 110 return ALTERNATE; 111 if ("appendix".equals(codeString)) 112 return APPENDIX; 113 if ("bookmark".equals(codeString)) 114 return BOOKMARK; 115 if ("chapter".equals(codeString)) 116 return CHAPTER; 117 if ("contents".equals(codeString)) 118 return CONTENTS; 119 if ("copyright".equals(codeString)) 120 return COPYRIGHT; 121 if ("glossary".equals(codeString)) 122 return GLOSSARY; 123 if ("help".equals(codeString)) 124 return HELP; 125 if ("index".equals(codeString)) 126 return INDEX; 127 if ("next".equals(codeString)) 128 return NEXT; 129 if ("prev".equals(codeString)) 130 return PREV; 131 if ("section".equals(codeString)) 132 return SECTION; 133 if ("start".equals(codeString)) 134 return START; 135 if ("stylesheet".equals(codeString)) 136 return STYLESHEET; 137 if ("subsection".equals(codeString)) 138 return SUBSECTION; 139 throw new FHIRException("Unknown V3HtmlLinkType code '"+codeString+"'"); 140 } 141 public String toCode() { 142 switch (this) { 143 case ALTERNATE: return "alternate"; 144 case APPENDIX: return "appendix"; 145 case BOOKMARK: return "bookmark"; 146 case CHAPTER: return "chapter"; 147 case CONTENTS: return "contents"; 148 case COPYRIGHT: return "copyright"; 149 case GLOSSARY: return "glossary"; 150 case HELP: return "help"; 151 case INDEX: return "index"; 152 case NEXT: return "next"; 153 case PREV: return "prev"; 154 case SECTION: return "section"; 155 case START: return "start"; 156 case STYLESHEET: return "stylesheet"; 157 case SUBSECTION: return "subsection"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getSystem() { 163 return "http://hl7.org/fhir/v3/HtmlLinkType"; 164 } 165 public String getDefinition() { 166 switch (this) { 167 case ALTERNATE: return "Designates substitute versions for the document in which the link occurs. When used together with the lang attribute, it implies a translated version of the document. When used together with the media attribute, it implies a version designed for a different medium (or media)."; 168 case APPENDIX: return "Refers to a document serving as an appendix in a collection of documents."; 169 case BOOKMARK: return "Refers to a bookmark. A bookmark is a link to a key entry point within an extended document. The title attribute may be used, for example, to label the bookmark. Note that several bookmarks may be defined in each document."; 170 case CHAPTER: return "Refers to a document serving as a chapter in a collection of documents."; 171 case CONTENTS: return "Refers to a document serving as a table of contents. Some user agents also support the synonym ToC (from \"Table of Contents\")."; 172 case COPYRIGHT: return "Refers to a copyright statement for the current document."; 173 case GLOSSARY: return "Refers to a document providing a glossary of terms that pertain to the current document."; 174 case HELP: return "Refers to a document offering help (more information, links to other sources of information, etc.)."; 175 case INDEX: return "Refers to a document providing an index for the current document."; 176 case NEXT: return "Refers to the next document in a linear sequence of documents. User agents may choose to preload the \"next\" document, to reduce the perceived load time."; 177 case PREV: return "Refers to the previous document in an ordered series of documents. Some user agents also support the synonym \"Previous\"."; 178 case SECTION: return "Refers to a document serving as a section in a collection of documents."; 179 case START: return "Refers to the first document in a collection of documents. This link type tells search engines which document is considered by the author to be the starting point of the collection."; 180 case STYLESHEET: return "Refers to an external style sheet. See the section on external style sheets for details. This is used together with the link type \"Alternate\" for user-selectable alternate style sheets."; 181 case SUBSECTION: return "Refers to a document serving as a subsection in a collection of documents."; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case ALTERNATE: return "alternate"; 189 case APPENDIX: return "appendix"; 190 case BOOKMARK: return "bookmark"; 191 case CHAPTER: return "chapter"; 192 case CONTENTS: return "contents"; 193 case COPYRIGHT: return "copyright"; 194 case GLOSSARY: return "glossary"; 195 case HELP: return "help"; 196 case INDEX: return "index"; 197 case NEXT: return "next"; 198 case PREV: return "prev"; 199 case SECTION: return "section"; 200 case START: return "start"; 201 case STYLESHEET: return "stylesheet"; 202 case SUBSECTION: return "subsection"; 203 case NULL: return null; 204 default: return "?"; 205 } 206 } 207 208 209}