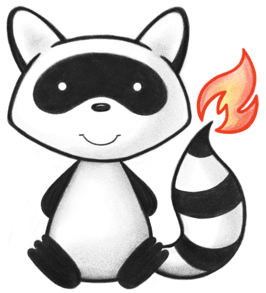
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3LivingArrangement { 041 042 /** 043 * Definition: Living arrangements lacking a permanent residence. 044 */ 045 HL, 046 /** 047 * Nomadic 048 */ 049 M, 050 /** 051 * Transient 052 */ 053 T, 054 /** 055 * Institution 056 */ 057 I, 058 /** 059 * Definition: A group living arrangement specifically for the care of those in need of temporary and crisis housing assistance. Examples include domestic violence shelters, shelters for displaced or homeless individuals, Salvation Army, Jesus House, etc. Community based services may be provided. 060 */ 061 CS, 062 /** 063 * Group Home 064 */ 065 G, 066 /** 067 * Nursing Home 068 */ 069 N, 070 /** 071 * Extended care facility 072 */ 073 X, 074 /** 075 * Definition: A living arrangement within a private residence for single family. 076 */ 077 PR, 078 /** 079 * Independent Household 080 */ 081 H, 082 /** 083 * Retirement Community 084 */ 085 R, 086 /** 087 * Definition: Assisted living in a single family residence for persons with physical, behavioral, or functional health, or socio-economic challenges. There may or may not be on-site supervision but the housing is designed to assist the client with developing independent living skills. Community based services may be provided. 088 */ 089 SL, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 public static V3LivingArrangement fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("HL".equals(codeString)) 098 return HL; 099 if ("M".equals(codeString)) 100 return M; 101 if ("T".equals(codeString)) 102 return T; 103 if ("I".equals(codeString)) 104 return I; 105 if ("CS".equals(codeString)) 106 return CS; 107 if ("G".equals(codeString)) 108 return G; 109 if ("N".equals(codeString)) 110 return N; 111 if ("X".equals(codeString)) 112 return X; 113 if ("PR".equals(codeString)) 114 return PR; 115 if ("H".equals(codeString)) 116 return H; 117 if ("R".equals(codeString)) 118 return R; 119 if ("SL".equals(codeString)) 120 return SL; 121 throw new FHIRException("Unknown V3LivingArrangement code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case HL: return "HL"; 126 case M: return "M"; 127 case T: return "T"; 128 case I: return "I"; 129 case CS: return "CS"; 130 case G: return "G"; 131 case N: return "N"; 132 case X: return "X"; 133 case PR: return "PR"; 134 case H: return "H"; 135 case R: return "R"; 136 case SL: return "SL"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getSystem() { 142 return "http://hl7.org/fhir/v3/LivingArrangement"; 143 } 144 public String getDefinition() { 145 switch (this) { 146 case HL: return "Definition: Living arrangements lacking a permanent residence."; 147 case M: return "Nomadic"; 148 case T: return "Transient"; 149 case I: return "Institution"; 150 case CS: return "Definition: A group living arrangement specifically for the care of those in need of temporary and crisis housing assistance. Examples include domestic violence shelters, shelters for displaced or homeless individuals, Salvation Army, Jesus House, etc. Community based services may be provided."; 151 case G: return "Group Home"; 152 case N: return "Nursing Home"; 153 case X: return "Extended care facility"; 154 case PR: return "Definition: A living arrangement within a private residence for single family."; 155 case H: return "Independent Household"; 156 case R: return "Retirement Community"; 157 case SL: return "Definition: Assisted living in a single family residence for persons with physical, behavioral, or functional health, or socio-economic challenges. There may or may not be on-site supervision but the housing is designed to assist the client with developing independent living skills. Community based services may be provided."; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getDisplay() { 163 switch (this) { 164 case HL: return "homeless"; 165 case M: return "Nomadic"; 166 case T: return "Transient"; 167 case I: return "Institution"; 168 case CS: return "community shelter"; 169 case G: return "Group Home"; 170 case N: return "Nursing Home"; 171 case X: return "Extended care facility"; 172 case PR: return "private residence"; 173 case H: return "Independent Household"; 174 case R: return "Retirement Community"; 175 case SL: return "supported living"; 176 case NULL: return null; 177 default: return "?"; 178 } 179 } 180 181 182}