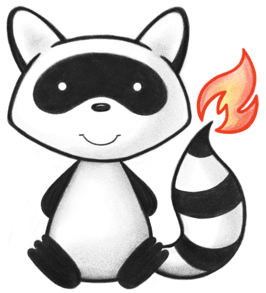
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3MaritalStatus { 041 042 /** 043 * Marriage contract has been declared null and to not have existed 044 */ 045 A, 046 /** 047 * Marriage contract has been declared dissolved and inactive 048 */ 049 D, 050 /** 051 * Subject to an Interlocutory Decree. 052 */ 053 I, 054 /** 055 * Legally Separated 056 */ 057 L, 058 /** 059 * A current marriage contract is active 060 */ 061 M, 062 /** 063 * More than 1 current spouse 064 */ 065 P, 066 /** 067 * No marriage contract has ever been entered 068 */ 069 S, 070 /** 071 * Person declares that a domestic partner relationship exists. 072 */ 073 T, 074 /** 075 * Currently not in a marriage contract. 076 */ 077 U, 078 /** 079 * The spouse has died 080 */ 081 W, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 public static V3MaritalStatus fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("A".equals(codeString)) 090 return A; 091 if ("D".equals(codeString)) 092 return D; 093 if ("I".equals(codeString)) 094 return I; 095 if ("L".equals(codeString)) 096 return L; 097 if ("M".equals(codeString)) 098 return M; 099 if ("P".equals(codeString)) 100 return P; 101 if ("S".equals(codeString)) 102 return S; 103 if ("T".equals(codeString)) 104 return T; 105 if ("U".equals(codeString)) 106 return U; 107 if ("W".equals(codeString)) 108 return W; 109 throw new FHIRException("Unknown V3MaritalStatus code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case A: return "A"; 114 case D: return "D"; 115 case I: return "I"; 116 case L: return "L"; 117 case M: return "M"; 118 case P: return "P"; 119 case S: return "S"; 120 case T: return "T"; 121 case U: return "U"; 122 case W: return "W"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getSystem() { 128 return "http://hl7.org/fhir/v3/MaritalStatus"; 129 } 130 public String getDefinition() { 131 switch (this) { 132 case A: return "Marriage contract has been declared null and to not have existed"; 133 case D: return "Marriage contract has been declared dissolved and inactive"; 134 case I: return "Subject to an Interlocutory Decree."; 135 case L: return "Legally Separated"; 136 case M: return "A current marriage contract is active"; 137 case P: return "More than 1 current spouse"; 138 case S: return "No marriage contract has ever been entered"; 139 case T: return "Person declares that a domestic partner relationship exists."; 140 case U: return "Currently not in a marriage contract."; 141 case W: return "The spouse has died"; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDisplay() { 147 switch (this) { 148 case A: return "Annulled"; 149 case D: return "Divorced"; 150 case I: return "Interlocutory"; 151 case L: return "Legally Separated"; 152 case M: return "Married"; 153 case P: return "Polygamous"; 154 case S: return "Never Married"; 155 case T: return "Domestic partner"; 156 case U: return "unmarried"; 157 case W: return "Widowed"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 163 164}