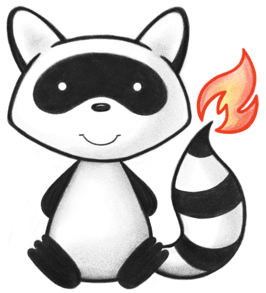
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3NullFlavor { 041 042 /** 043 * Description:The value is exceptional (missing, omitted, incomplete, improper). No information as to the reason for being an exceptional value is provided. This is the most general exceptional value. It is also the default exceptional value. 044 */ 045 NI, 046 /** 047 * Description:The value as represented in the instance is not a member of the set of permitted data values in the constrained value domain of a variable. 048 */ 049 INV, 050 /** 051 * Description:An actual value may exist, but it must be derived from the provided information (usually an EXPR generic data type extension will be used to convey the derivation expressionexpression . 052 */ 053 DER, 054 /** 055 * Description:The actual value is not a member of the set of permitted data values in the constrained value domain of a variable. (e.g., concept not provided by required code system). 056 057 058 Usage Notes: This flavor and its specializations are most commonly used with the CD datatype and its flavors. However, it may apply to *any* datatype where the constraints of the type are tighter than can be conveyed. For example, a PQ that is for a true measured amount whose units are not supported in UCUM, a need to convey a REAL when the type has been constrained to INT, etc. 059 060 With coded datatypes, this null flavor may only be used if the vocabulary binding has a coding strength of CNE. By definition, all local codes and original text are part of the value set if the coding strength is CWE. 061 */ 062 OTH, 063 /** 064 * Negative infinity of numbers. 065 */ 066 NINF, 067 /** 068 * Positive infinity of numbers. 069 */ 070 PINF, 071 /** 072 * Description: The actual value has not yet been encoded within the approved value domain. 073 074 075 Example: Original text or a local code has been specified but translation or encoding to the approved value set has not yet occurred due to limitations of the sending system. Original text has been captured for a PQ, but not attempt has been made to split the value and unit or to encode the unit in UCUM. 076 077 078 Usage Notes: If it is known that it is not possible to encode the concept, OTH should be used instead. However, use of UNC does not necessarily guarantee the concept will be encodable, only that encoding has not been attempted. 079 080 Data type properties such as original text and translations may be present when this null flavor is included. 081 */ 082 UNC, 083 /** 084 * There is information on this item available but it has not been provided by the sender due to security, privacy or other reasons. There may be an alternate mechanism for gaining access to this information. 085 086 Note: using this null flavor does provide information that may be a breach of confidentiality, even though no detail data is provided. Its primary purpose is for those circumstances where it is necessary to inform the receiver that the information does exist without providing any detail. 087 */ 088 MSK, 089 /** 090 * Known to have no proper value (e.g., last menstrual period for a male). 091 */ 092 NA, 093 /** 094 * Description:A proper value is applicable, but not known. 095 096 097 Usage Notes: This means the actual value is not known. If the only thing that is unknown is how to properly express the value in the necessary constraints (value set, datatype, etc.), then the OTH or UNC flavor should be used. No properties should be included for a datatype with this property unless: 098 099 100 Those properties themselves directly translate to a semantic of "unknown". (E.g. a local code sent as a translation that conveys 'unknown') 101 Those properties further qualify the nature of what is unknown. (E.g. specifying a use code of "H" and a URL prefix of "tel:" to convey that it is the home phone number that is unknown.) 102 */ 103 UNK, 104 /** 105 * Information was sought but not found (e.g., patient was asked but didn't know) 106 */ 107 ASKU, 108 /** 109 * Information is not available at this time but it is expected that it will be available later. 110 */ 111 NAV, 112 /** 113 * This information has not been sought (e.g., patient was not asked) 114 */ 115 NASK, 116 /** 117 * Information is not available at this time (with no expectation regarding whether it will or will not be available in the future). 118 */ 119 NAVU, 120 /** 121 * Description:The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material. e.g. 'Add 10mg of ingredient X, 50mg of ingredient Y, and sufficient quantity of water to 100mL.' The null flavor would be used to express the quantity of water. 122 */ 123 QS, 124 /** 125 * The content is greater than zero, but too small to be quantified. 126 */ 127 TRC, 128 /** 129 * Value is not present in a message. This is only defined in messages, never in application data! All values not present in the message must be replaced by the applicable default, or no-information (NI) as the default of all defaults. 130 */ 131 NP, 132 /** 133 * added to help the parsers 134 */ 135 NULL; 136 public static V3NullFlavor fromCode(String codeString) throws FHIRException { 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("NI".equals(codeString)) 140 return NI; 141 if ("INV".equals(codeString)) 142 return INV; 143 if ("DER".equals(codeString)) 144 return DER; 145 if ("OTH".equals(codeString)) 146 return OTH; 147 if ("NINF".equals(codeString)) 148 return NINF; 149 if ("PINF".equals(codeString)) 150 return PINF; 151 if ("UNC".equals(codeString)) 152 return UNC; 153 if ("MSK".equals(codeString)) 154 return MSK; 155 if ("NA".equals(codeString)) 156 return NA; 157 if ("UNK".equals(codeString)) 158 return UNK; 159 if ("ASKU".equals(codeString)) 160 return ASKU; 161 if ("NAV".equals(codeString)) 162 return NAV; 163 if ("NASK".equals(codeString)) 164 return NASK; 165 if ("NAVU".equals(codeString)) 166 return NAVU; 167 if ("QS".equals(codeString)) 168 return QS; 169 if ("TRC".equals(codeString)) 170 return TRC; 171 if ("NP".equals(codeString)) 172 return NP; 173 throw new FHIRException("Unknown V3NullFlavor code '"+codeString+"'"); 174 } 175 public String toCode() { 176 switch (this) { 177 case NI: return "NI"; 178 case INV: return "INV"; 179 case DER: return "DER"; 180 case OTH: return "OTH"; 181 case NINF: return "NINF"; 182 case PINF: return "PINF"; 183 case UNC: return "UNC"; 184 case MSK: return "MSK"; 185 case NA: return "NA"; 186 case UNK: return "UNK"; 187 case ASKU: return "ASKU"; 188 case NAV: return "NAV"; 189 case NASK: return "NASK"; 190 case NAVU: return "NAVU"; 191 case QS: return "QS"; 192 case TRC: return "TRC"; 193 case NP: return "NP"; 194 case NULL: return null; 195 default: return "?"; 196 } 197 } 198 public String getSystem() { 199 return "http://hl7.org/fhir/v3/NullFlavor"; 200 } 201 public String getDefinition() { 202 switch (this) { 203 case NI: return "Description:The value is exceptional (missing, omitted, incomplete, improper). No information as to the reason for being an exceptional value is provided. This is the most general exceptional value. It is also the default exceptional value."; 204 case INV: return "Description:The value as represented in the instance is not a member of the set of permitted data values in the constrained value domain of a variable."; 205 case DER: return "Description:An actual value may exist, but it must be derived from the provided information (usually an EXPR generic data type extension will be used to convey the derivation expressionexpression ."; 206 case OTH: return "Description:The actual value is not a member of the set of permitted data values in the constrained value domain of a variable. (e.g., concept not provided by required code system).\r\n\n \n Usage Notes: This flavor and its specializations are most commonly used with the CD datatype and its flavors. However, it may apply to *any* datatype where the constraints of the type are tighter than can be conveyed. For example, a PQ that is for a true measured amount whose units are not supported in UCUM, a need to convey a REAL when the type has been constrained to INT, etc.\r\n\n With coded datatypes, this null flavor may only be used if the vocabulary binding has a coding strength of CNE. By definition, all local codes and original text are part of the value set if the coding strength is CWE."; 207 case NINF: return "Negative infinity of numbers."; 208 case PINF: return "Positive infinity of numbers."; 209 case UNC: return "Description: The actual value has not yet been encoded within the approved value domain.\r\n\n \n Example: Original text or a local code has been specified but translation or encoding to the approved value set has not yet occurred due to limitations of the sending system. Original text has been captured for a PQ, but not attempt has been made to split the value and unit or to encode the unit in UCUM.\r\n\n \n Usage Notes: If it is known that it is not possible to encode the concept, OTH should be used instead. However, use of UNC does not necessarily guarantee the concept will be encodable, only that encoding has not been attempted.\r\n\n Data type properties such as original text and translations may be present when this null flavor is included."; 210 case MSK: return "There is information on this item available but it has not been provided by the sender due to security, privacy or other reasons. There may be an alternate mechanism for gaining access to this information.\r\n\n Note: using this null flavor does provide information that may be a breach of confidentiality, even though no detail data is provided. Its primary purpose is for those circumstances where it is necessary to inform the receiver that the information does exist without providing any detail."; 211 case NA: return "Known to have no proper value (e.g., last menstrual period for a male)."; 212 case UNK: return "Description:A proper value is applicable, but not known.\r\n\n \n Usage Notes: This means the actual value is not known. If the only thing that is unknown is how to properly express the value in the necessary constraints (value set, datatype, etc.), then the OTH or UNC flavor should be used. No properties should be included for a datatype with this property unless:\r\n\n \n Those properties themselves directly translate to a semantic of \"unknown\". (E.g. a local code sent as a translation that conveys 'unknown')\n Those properties further qualify the nature of what is unknown. (E.g. specifying a use code of \"H\" and a URL prefix of \"tel:\" to convey that it is the home phone number that is unknown.)"; 213 case ASKU: return "Information was sought but not found (e.g., patient was asked but didn't know)"; 214 case NAV: return "Information is not available at this time but it is expected that it will be available later."; 215 case NASK: return "This information has not been sought (e.g., patient was not asked)"; 216 case NAVU: return "Information is not available at this time (with no expectation regarding whether it will or will not be available in the future)."; 217 case QS: return "Description:The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material. e.g. 'Add 10mg of ingredient X, 50mg of ingredient Y, and sufficient quantity of water to 100mL.' The null flavor would be used to express the quantity of water."; 218 case TRC: return "The content is greater than zero, but too small to be quantified."; 219 case NP: return "Value is not present in a message. This is only defined in messages, never in application data! All values not present in the message must be replaced by the applicable default, or no-information (NI) as the default of all defaults."; 220 case NULL: return null; 221 default: return "?"; 222 } 223 } 224 public String getDisplay() { 225 switch (this) { 226 case NI: return "NoInformation"; 227 case INV: return "invalid"; 228 case DER: return "derived"; 229 case OTH: return "other"; 230 case NINF: return "negative infinity"; 231 case PINF: return "positive infinity"; 232 case UNC: return "un-encoded"; 233 case MSK: return "masked"; 234 case NA: return "not applicable"; 235 case UNK: return "unknown"; 236 case ASKU: return "asked but unknown"; 237 case NAV: return "temporarily unavailable"; 238 case NASK: return "not asked"; 239 case NAVU: return "Not available"; 240 case QS: return "Sufficient Quantity"; 241 case TRC: return "trace"; 242 case NP: return "not present"; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 248 249}