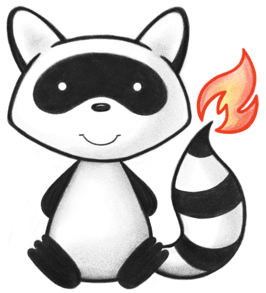
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3ObservationMethod { 041 042 /** 043 * Provides codes for decision methods, initially for assessing the causality of events. 044 */ 045 _DECISIONOBSERVATIONMETHOD, 046 /** 047 * Reaching a decision through the application of an algorithm designed to weigh the different factors involved. 048 */ 049 ALGM, 050 /** 051 * Reaching a decision through the use of Bayesian statistical analysis. 052 */ 053 BYCL, 054 /** 055 * Reaching a decision by consideration of the totality of factors involved in order to reach a judgement. 056 */ 057 GINT, 058 /** 059 * A code that provides additional detail about the means or technique used to ascertain the genetic analysis. Example, PCR, Micro Array 060 */ 061 _GENETICOBSERVATIONMETHOD, 062 /** 063 * Description: Polymerase Chain Reaction 064 */ 065 PCR, 066 /** 067 * Provides additional detail about the aggregation methods used to compute the aggregated values for an observation. This is an abstract code. 068 */ 069 _OBSERVATIONMETHODAGGREGATE, 070 /** 071 * Average of non-null values in the referenced set of values 072 */ 073 AVERAGE, 074 /** 075 * Count of non-null values in the referenced set of values 076 */ 077 COUNT, 078 /** 079 * Largest of all non-null values in the referenced set of values. 080 */ 081 MAX, 082 /** 083 * The median of all non-null values in the referenced set of values. 084 */ 085 MEDIAN, 086 /** 087 * Smallest of all non-null values in the referenced set of values. 088 */ 089 MIN, 090 /** 091 * The most common value of all non-null values in the referenced set of values. 092 */ 093 MODE, 094 /** 095 * Standard Deviation of the values in the referenced set of values, computed over the population. 096 */ 097 STDEV_P, 098 /** 099 * Standard Deviation of the values in the referenced set of values, computed over a sample of the population. 100 */ 101 STDEV_S, 102 /** 103 * Sum of non-null values in the referenced set of values 104 */ 105 SUM, 106 /** 107 * Variance of the values in the referenced set of values, computed over the population. 108 */ 109 VARIANCE_P, 110 /** 111 * Variance of the values in the referenced set of values, computed over a sample of the population. 112 */ 113 VARIANCE_S, 114 /** 115 * VerificationMethod 116 */ 117 _VERIFICATIONMETHOD, 118 /** 119 * Verification by means of document. 120 121 122 Example: Fax, letter, attachment to e-mail. 123 */ 124 VDOC, 125 /** 126 * verification by means of a response to an electronic query 127 128 129 Example: query message to a Covered Party registry application or Coverage Administrator. 130 */ 131 VREG, 132 /** 133 * Verification by means of electronic token. 134 135 136 Example: smartcard, magnetic swipe card, RFID device. 137 */ 138 VTOKEN, 139 /** 140 * Verification by means of voice. 141 142 143 Example: By speaking with or calling the Coverage Administrator or Covered Party 144 */ 145 VVOICE, 146 /** 147 * Complement fixation 148 */ 149 _0001, 150 /** 151 * Computed axial tomography 152 */ 153 _0002, 154 /** 155 * Susceptibility, High Level Aminoglycoside Resistance agar test 156 */ 157 _0003, 158 /** 159 * Visual, Macroscopic observation 160 */ 161 _0004, 162 /** 163 * Computed, Magnetic resonance 164 */ 165 _0005, 166 /** 167 * Computed, Morphometry 168 */ 169 _0006, 170 /** 171 * Computed, Positron emission tomography 172 */ 173 _0007, 174 /** 175 * SAMHSA drug assay confirmation 176 */ 177 _0008, 178 /** 179 * SAMHSA drug assay screening 180 */ 181 _0009, 182 /** 183 * Serum Neutralization 184 */ 185 _0010, 186 /** 187 * Titration 188 */ 189 _0011, 190 /** 191 * Ultrasound 192 */ 193 _0012, 194 /** 195 * X-ray crystallography 196 */ 197 _0013, 198 /** 199 * Agglutination 200 */ 201 _0014, 202 /** 203 * Agglutination, Buffered acidified plate 204 */ 205 _0015, 206 /** 207 * Agglutination, Card 208 */ 209 _0016, 210 /** 211 * Agglutination, Hemagglutination 212 */ 213 _0017, 214 /** 215 * Agglutination, Hemagglutination inhibition 216 */ 217 _0018, 218 /** 219 * Agglutination, Latex 220 */ 221 _0019, 222 /** 223 * Agglutination, Plate 224 */ 225 _0020, 226 /** 227 * Agglutination, Rapid Plate 228 */ 229 _0021, 230 /** 231 * Agglutination, RBC 232 */ 233 _0022, 234 /** 235 * Agglutination, Rivanol 236 */ 237 _0023, 238 /** 239 * Agglutination, Tube 240 */ 241 _0024, 242 /** 243 * Bioassay 244 */ 245 _0025, 246 /** 247 * Bioassay, Animal Inoculation 248 */ 249 _0026, 250 /** 251 * Bioassay, Cytotoxicity 252 */ 253 _0027, 254 /** 255 * Bioassay, Embryo Infective Dose 50 256 */ 257 _0028, 258 /** 259 * Bioassay, Embryo Lethal Dose 50 260 */ 261 _0029, 262 /** 263 * Bioassay, Mouse intercerebral inoculation 264 */ 265 _0030, 266 /** 267 * Bioassay, qualitative 268 */ 269 _0031, 270 /** 271 * Bioassay, quantitative 272 */ 273 _0032, 274 /** 275 * Chemical 276 */ 277 _0033, 278 /** 279 * Chemical, Differential light absorption 280 */ 281 _0034, 282 /** 283 * Chemical, Dipstick 284 */ 285 _0035, 286 /** 287 * Chemical, Dipstick colorimetric laboratory test 288 */ 289 _0036, 290 /** 291 * Chemical, Test strip 292 */ 293 _0037, 294 /** 295 * Chromatography 296 */ 297 _0038, 298 /** 299 * Chromatography, Affinity 300 */ 301 _0039, 302 /** 303 * Chromatography, Gas liquid 304 */ 305 _0040, 306 /** 307 * Chromatography, High performance liquid 308 */ 309 _0041, 310 /** 311 * Chromatography, Liquid 312 */ 313 _0042, 314 /** 315 * Chromatography, Protein A affinity 316 */ 317 _0043, 318 /** 319 * Coagulation 320 */ 321 _0044, 322 /** 323 * Coagulation, Tilt tube 324 */ 325 _0045, 326 /** 327 * Coagulation, Tilt tube reptilase induced 328 */ 329 _0046, 330 /** 331 * Count, Automated 332 */ 333 _0047, 334 /** 335 * Count, Manual 336 */ 337 _0048, 338 /** 339 * Count, Platelet, Rees-Ecker 340 */ 341 _0049, 342 /** 343 * Culture, Aerobic 344 */ 345 _0050, 346 /** 347 * Culture, Anaerobic 348 */ 349 _0051, 350 /** 351 * Culture, Chicken Embryo 352 */ 353 _0052, 354 /** 355 * Culture, Delayed secondary enrichment 356 */ 357 _0053, 358 /** 359 * Culture, Microaerophilic 360 */ 361 _0054, 362 /** 363 * Culture, Quantitative microbial, cup 364 */ 365 _0055, 366 /** 367 * Culture, Quantitative microbial, droplet 368 */ 369 _0056, 370 /** 371 * Culture, Quantitative microbial, filter paper 372 */ 373 _0057, 374 /** 375 * Culture, Quantitative microbial, pad 376 */ 377 _0058, 378 /** 379 * Culture, Quantitative microbial, pour plate 380 */ 381 _0059, 382 /** 383 * Culture, Quantitative microbial, surface streak 384 */ 385 _0060, 386 /** 387 * Culture, Somatic Cell 388 */ 389 _0061, 390 /** 391 * Diffusion, Agar 392 */ 393 _0062, 394 /** 395 * Diffusion, Agar Gel Immunodiffusion 396 */ 397 _0063, 398 /** 399 * Electrophoresis 400 */ 401 _0064, 402 /** 403 * Electrophoresis, Agaorse gel 404 */ 405 _0065, 406 /** 407 * Electrophoresis, citrate agar 408 */ 409 _0066, 410 /** 411 * Electrophoresis, Immuno 412 */ 413 _0067, 414 /** 415 * Electrophoresis, Polyacrylamide gel 416 */ 417 _0068, 418 /** 419 * Electrophoresis, Starch gel 420 */ 421 _0069, 422 /** 423 * ELISA 424 */ 425 _0070, 426 /** 427 * ELISA, antigen capture 428 */ 429 _0071, 430 /** 431 * ELISA, avidin biotin peroxidase complex 432 */ 433 _0072, 434 /** 435 * ELISA, Kinetic 436 */ 437 _0073, 438 /** 439 * ELISA, peroxidase-antiperoxidase 440 */ 441 _0074, 442 /** 443 * Identification, API 20 Strep 444 */ 445 _0075, 446 /** 447 * Identification, API 20A 448 */ 449 _0076, 450 /** 451 * Identification, API 20C AUX 452 */ 453 _0077, 454 /** 455 * Identification, API 20E 456 */ 457 _0078, 458 /** 459 * Identification, API 20NE 460 */ 461 _0079, 462 /** 463 * Identification, API 50 CH 464 */ 465 _0080, 466 /** 467 * Identification, API An-IDENT 468 */ 469 _0081, 470 /** 471 * Identification, API Coryne 472 */ 473 _0082, 474 /** 475 * Identification, API Rapid 20E 476 */ 477 _0083, 478 /** 479 * Identification, API Staph 480 */ 481 _0084, 482 /** 483 * Identification, API ZYM 484 */ 485 _0085, 486 /** 487 * Identification, Bacterial 488 */ 489 _0086, 490 /** 491 * Identification, mini VIDAS 492 */ 493 _0087, 494 /** 495 * Identification, Phage susceptibility typing 496 */ 497 _0088, 498 /** 499 * Identification, Quad-FERM+ 500 */ 501 _0089, 502 /** 503 * Identification, RAPIDEC Staph 504 */ 505 _0090, 506 /** 507 * Identification, Staphaurex 508 */ 509 _0091, 510 /** 511 * Identification, VIDAS 512 */ 513 _0092, 514 /** 515 * Identification, Vitek 516 */ 517 _0093, 518 /** 519 * Identification, VITEK 2 520 */ 521 _0094, 522 /** 523 * Immune stain 524 */ 525 _0095, 526 /** 527 * Immune stain, Immunofluorescent antibody, direct 528 */ 529 _0096, 530 /** 531 * Immune stain, Immunofluorescent antibody, indirect 532 */ 533 _0097, 534 /** 535 * Immune stain, Immunoperoxidase, Avidin-Biotin Complex 536 */ 537 _0098, 538 /** 539 * Immune stain, Immunoperoxidase, Peroxidase anti-peroxidase complex 540 */ 541 _0099, 542 /** 543 * Immune stain, Immunoperoxidase, Protein A-peroxidase complex 544 */ 545 _0100, 546 /** 547 * Immunoassay 548 */ 549 _0101, 550 /** 551 * Immunoassay, qualitative, multiple step 552 */ 553 _0102, 554 /** 555 * Immunoassay, qualitative, single step 556 */ 557 _0103, 558 /** 559 * Immunoassay, Radioimmunoassay 560 */ 561 _0104, 562 /** 563 * Immunoassay, semi-quantitative, multiple step 564 */ 565 _0105, 566 /** 567 * Immunoassay, semi-quantitative, single step 568 */ 569 _0106, 570 /** 571 * Microscopy 572 */ 573 _0107, 574 /** 575 * Microscopy, Darkfield 576 */ 577 _0108, 578 /** 579 * Microscopy, Electron 580 */ 581 _0109, 582 /** 583 * Microscopy, Electron microscopy tomography 584 */ 585 _0110, 586 /** 587 * Microscopy, Electron, negative stain 588 */ 589 _0111, 590 /** 591 * Microscopy, Electron, thick section transmission 592 */ 593 _0112, 594 /** 595 * Microscopy, Electron, thin section transmission 596 */ 597 _0113, 598 /** 599 * Microscopy, Light 600 */ 601 _0114, 602 /** 603 * Microscopy, Polarized light 604 */ 605 _0115, 606 /** 607 * Microscopy, Scanning electron 608 */ 609 _0116, 610 /** 611 * Microscopy, Transmission electron 612 */ 613 _0117, 614 /** 615 * Microscopy, Transparent tape direct examination 616 */ 617 _0118, 618 /** 619 * Molecular, 3 Self-Sustaining Sequence Replication 620 */ 621 _0119, 622 /** 623 * Molecular, Branched Chain DNA 624 */ 625 _0120, 626 /** 627 * Molecular, Hybridization Protection Assay 628 */ 629 _0121, 630 /** 631 * Molecular, Immune blot 632 */ 633 _0122, 634 /** 635 * Molecular, In-situ hybridization 636 */ 637 _0123, 638 /** 639 * Molecular, Ligase Chain Reaction 640 */ 641 _0124, 642 /** 643 * Molecular, Ligation Activated Transcription 644 */ 645 _0125, 646 /** 647 * Molecular, Nucleic Acid Probe 648 */ 649 _0126, 650 /** 651 * Molecular, Nucleic acid probe with amplification 652 653 654 655 Rationale: Duplicate of code 0126. Use code 0126 instead. 656 */ 657 _0128, 658 /** 659 * Molecular, Nucleic acid probe with target amplification 660 */ 661 _0129, 662 /** 663 * Molecular, Nucleic acid reverse transcription 664 */ 665 _0130, 666 /** 667 * Molecular, Nucleic Acid Sequence Based Analysis 668 */ 669 _0131, 670 /** 671 * Molecular, Polymerase chain reaction 672 */ 673 _0132, 674 /** 675 * Molecular, Q-Beta Replicase or probe amplification category method 676 */ 677 _0133, 678 /** 679 * Molecular, Restriction Fragment Length Polymorphism 680 */ 681 _0134, 682 /** 683 * Molecular, Southern Blot 684 */ 685 _0135, 686 /** 687 * Molecular, Strand Displacement Amplification 688 */ 689 _0136, 690 /** 691 * Molecular, Transcription Mediated Amplification 692 */ 693 _0137, 694 /** 695 * Molecular, Western Blot 696 */ 697 _0138, 698 /** 699 * Precipitation, Flocculation 700 */ 701 _0139, 702 /** 703 * Precipitation, Immune precipitation 704 */ 705 _0140, 706 /** 707 * Precipitation, Milk ring test 708 */ 709 _0141, 710 /** 711 * Precipitation, Precipitin 712 */ 713 _0142, 714 /** 715 * Stain, Acid fast 716 */ 717 _0143, 718 /** 719 * Stain, Acid fast, fluorochrome 720 */ 721 _0144, 722 /** 723 * Stain, Acid fast, Kinyoun's cold carbolfuchsin 724 */ 725 _0145, 726 /** 727 * Stain, Acid fast, Ziehl-Neelsen 728 */ 729 _0146, 730 /** 731 * Stain, Acid phosphatase 732 */ 733 _0147, 734 /** 735 * Stain, Acridine orange 736 */ 737 _0148, 738 /** 739 * Stain, Active brilliant orange KH 740 */ 741 _0149, 742 /** 743 * Stain, Alazarin red S 744 */ 745 _0150, 746 /** 747 * Stain, Alcian blue 748 */ 749 _0151, 750 /** 751 * Stain, Alcian blue with Periodic acid Schiff 752 */ 753 _0152, 754 /** 755 * Stain, Argentaffin 756 */ 757 _0153, 758 /** 759 * Stain, Argentaffin silver 760 */ 761 _0154, 762 /** 763 * Stain, Azure-eosin 764 */ 765 _0155, 766 /** 767 * Stain, Basic Fuschin 768 */ 769 _0156, 770 /** 771 * Stain, Bennhold 772 */ 773 _0157, 774 /** 775 * Stain, Bennhold's Congo red 776 */ 777 _0158, 778 /** 779 * Stain, Bielschowsky 780 */ 781 _0159, 782 /** 783 * Stain, Bielschowsky's silver 784 */ 785 _0160, 786 /** 787 * Stain, Bleach 788 */ 789 _0161, 790 /** 791 * Stain, Bodian 792 */ 793 _0162, 794 /** 795 * Stain, Brown-Brenn 796 */ 797 _0163, 798 /** 799 * Stain, Butyrate-esterase 800 */ 801 _0164, 802 /** 803 * Stain, Calcofluor white fluorescent 804 */ 805 _0165, 806 /** 807 * Stain, Carbol-fuchsin 808 */ 809 _0166, 810 /** 811 * Stain, Carmine 812 */ 813 _0167, 814 /** 815 * Stain, Churukian-Schenk 816 */ 817 _0168, 818 /** 819 * Stain, Congo red 820 */ 821 _0169, 822 /** 823 * Stain, Cresyl echt violet 824 */ 825 _0170, 826 /** 827 * Stain, Crystal violet 828 */ 829 _0171, 830 /** 831 * Stain, De Galantha 832 */ 833 _0172, 834 /** 835 * Stain, Dieterle silver impregnation 836 */ 837 _0173, 838 /** 839 * Stain, Fite-Farco 840 */ 841 _0174, 842 /** 843 * Stain, Fontana-Masson silver 844 */ 845 _0175, 846 /** 847 * Stain, Fouchet 848 */ 849 _0176, 850 /** 851 * Stain, Gomori 852 */ 853 _0177, 854 /** 855 * Stain, Gomori methenamine silver 856 */ 857 _0178, 858 /** 859 * Stain, Gomori-Wheatly trichrome 860 */ 861 _0179, 862 /** 863 * Stain, Gridley 864 */ 865 _0180, 866 /** 867 * Stain, Grimelius silver 868 */ 869 _0181, 870 /** 871 * Stain, Grocott 872 */ 873 _0182, 874 /** 875 * Stain, Grocott methenamine silver 876 */ 877 _0183, 878 /** 879 * Stain, Hale's colloidal ferric oxide 880 */ 881 _0184, 882 /** 883 * Stain, Hale's colloidal iron 884 */ 885 _0185, 886 /** 887 * Stain, Hansel 888 */ 889 _0186, 890 /** 891 * Stain, Harris regressive hematoxylin and eosin 892 */ 893 _0187, 894 /** 895 * Stain, Hematoxylin and eosin 896 */ 897 _0188, 898 /** 899 * Stain, Highman 900 */ 901 _0189, 902 /** 903 * Stain, Holzer 904 */ 905 _0190, 906 /** 907 * Stain, Iron hematoxylin 908 */ 909 _0191, 910 /** 911 * Stain, Jones 912 */ 913 _0192, 914 /** 915 * Stain, Jones methenamine silver 916 */ 917 _0193, 918 /** 919 * Stain, Kossa 920 */ 921 _0194, 922 /** 923 * Stain, Lawson-Van Gieson 924 */ 925 _0195, 926 /** 927 * Stain, Loeffler methylene blue 928 */ 929 _0196, 930 /** 931 * Stain, Luxol fast blue with cresyl violet 932 */ 933 _0197, 934 /** 935 * Stain, Luxol fast blue with Periodic acid-Schiff 936 */ 937 _0198, 938 /** 939 * Stain, MacNeal's tetrachrome blood 940 */ 941 _0199, 942 /** 943 * Stain, Mallory-Heidenhain 944 */ 945 _0200, 946 /** 947 * Stain, Masson trichrome 948 */ 949 _0201, 950 /** 951 * Stain, Mayer mucicarmine 952 */ 953 _0202, 954 /** 955 * Stain, Mayers progressive hematoxylin and eosin 956 */ 957 _0203, 958 /** 959 * Stain, May-Grunwald Giemsa 960 */ 961 _0204, 962 /** 963 * Stain, Methyl green 964 */ 965 _0205, 966 /** 967 * Stain, Methyl green pyronin 968 */ 969 _0206, 970 /** 971 * Stain, Modified Gomori-Wheatly trichrome 972 */ 973 _0207, 974 /** 975 * Stain, Modified Masson trichrome 976 */ 977 _0208, 978 /** 979 * Stain, Modified trichrome 980 */ 981 _0209, 982 /** 983 * Stain, Movat pentachrome 984 */ 985 _0210, 986 /** 987 * Stain, Mucicarmine 988 */ 989 _0211, 990 /** 991 * Stain, Neutral red 992 */ 993 _0212, 994 /** 995 * Stain, Night blue 996 */ 997 _0213, 998 /** 999 * Stain, Non-specific esterase 1000 */ 1001 _0214, 1002 /** 1003 * Stain, Oil red-O 1004 */ 1005 _0215, 1006 /** 1007 * Stain, Orcein 1008 */ 1009 _0216, 1010 /** 1011 * Stain, Perls' 1012 */ 1013 _0217, 1014 /** 1015 * Stain, Phosphotungstic acid-hematoxylin 1016 */ 1017 _0218, 1018 /** 1019 * Stain, Potassium ferrocyanide 1020 */ 1021 _0219, 1022 /** 1023 * Stain, Prussian blue 1024 */ 1025 _0220, 1026 /** 1027 * Stain, Putchler modified Bennhold 1028 */ 1029 _0221, 1030 /** 1031 * Stain, Quinacrine fluorescent 1032 */ 1033 _0222, 1034 /** 1035 * Stain, Reticulin 1036 */ 1037 _0223, 1038 /** 1039 * Stain, Rhodamine 1040 */ 1041 _0224, 1042 /** 1043 * Stain, Safranin 1044 */ 1045 _0225, 1046 /** 1047 * Stain, Schmorl 1048 */ 1049 _0226, 1050 /** 1051 * Stain, Seiver-Munger 1052 */ 1053 _0227, 1054 /** 1055 * Stain, Silver 1056 */ 1057 _0228, 1058 /** 1059 * Stain, Specific esterase 1060 */ 1061 _0229, 1062 /** 1063 * Stain, Steiner silver 1064 */ 1065 _0230, 1066 /** 1067 * Stain, Sudan III 1068 */ 1069 _0231, 1070 /** 1071 * Stain, Sudan IVI 1072 */ 1073 _0232, 1074 /** 1075 * Stain, Sulfated alcian blue 1076 */ 1077 _0233, 1078 /** 1079 * Stain, Supravital 1080 */ 1081 _0234, 1082 /** 1083 * Stain, Thioflavine-S 1084 */ 1085 _0235, 1086 /** 1087 * Stain, Three micron Giemsa 1088 */ 1089 _0236, 1090 /** 1091 * Stain, Vassar-Culling 1092 */ 1093 _0237, 1094 /** 1095 * Stain, Vital 1096 */ 1097 _0238, 1098 /** 1099 * Stain, von Kossa 1100 */ 1101 _0239, 1102 /** 1103 * Susceptibility, Minimum bactericidal concentration, macrodilution 1104 */ 1105 _0243, 1106 /** 1107 * Susceptibility, Minimum bactericidal concentration, microdilution 1108 */ 1109 _0244, 1110 /** 1111 * Turbidometric 1112 */ 1113 _0247, 1114 /** 1115 * Turbidometric, Refractometric 1116 */ 1117 _0248, 1118 /** 1119 * Chromatography, Thin Layer 1120 */ 1121 _0249, 1122 /** 1123 * Immunoassay, enzyme-multiplied technique (EMIT) 1124 */ 1125 _0250, 1126 /** 1127 * Flow Cytometry 1128 */ 1129 _0251, 1130 /** 1131 * Radial Immunodiffusion 1132 */ 1133 _0252, 1134 /** 1135 * Immunoassay, Fluorescence Polarization 1136 */ 1137 _0253, 1138 /** 1139 * Electrophoresis, Immunofixation 1140 */ 1141 _0254, 1142 /** 1143 * Dialysis, Direct Equilibrium 1144 */ 1145 _0255, 1146 /** 1147 * Acid Elution, Kleihauer-Betke Method 1148 */ 1149 _0256, 1150 /** 1151 * Immunofluorescence, Anti-Complement 1152 */ 1153 _0257, 1154 /** 1155 * Gas Chromatography/Mass Spectroscopy 1156 */ 1157 _0258, 1158 /** 1159 * Light Scatter, Nephelometry 1160 */ 1161 _0259, 1162 /** 1163 * Immunoassay, IgE Antibody Test 1164 */ 1165 _0260, 1166 /** 1167 * Lymphocyte Microcytotoxicity Assay 1168 */ 1169 _0261, 1170 /** 1171 * Spectrophotometry 1172 */ 1173 _0262, 1174 /** 1175 * Spectrophotometry, Atomic Absorption 1176 */ 1177 _0263, 1178 /** 1179 * Electrochemical, Ion Selective Electrode 1180 */ 1181 _0264, 1182 /** 1183 * Chromatography, Gas 1184 */ 1185 _0265, 1186 /** 1187 * Isoelectric Focusing 1188 */ 1189 _0266, 1190 /** 1191 * Immunoassay, Chemiluminescent 1192 */ 1193 _0267, 1194 /** 1195 * Immunoassay, Microparticle Enzyme 1196 */ 1197 _0268, 1198 /** 1199 * Inductively-Coupled Plasma/Mass Spectrometry 1200 */ 1201 _0269, 1202 /** 1203 * Immunoassay, Immunoradiometric Assay 1204 */ 1205 _0270, 1206 /** 1207 * Coagulation, Photo Optical Clot Detection 1208 */ 1209 _0271, 1210 /** 1211 * Test methods designed to determine a microorganismaTMs susceptibility to being killed by an antibiotic. 1212 */ 1213 _0280, 1214 /** 1215 * Susceptibility, Antibiotic sensitivity, disk 1216 */ 1217 _0240, 1218 /** 1219 * Susceptibility, BACTEC susceptibility test 1220 */ 1221 _0241, 1222 /** 1223 * Susceptibility, Disk dilution 1224 */ 1225 _0242, 1226 /** 1227 * Testing to measure the minimum concentration of the antibacterial agent in a given culture medium below which bacterial growth is not inhibited. 1228 */ 1229 _0272, 1230 /** 1231 * Susceptibility, Minimum Inhibitory concentration, macrodilution 1232 */ 1233 _0245, 1234 /** 1235 * Susceptibility, Minimum Inhibitory concentration, microdilution 1236 */ 1237 _0246, 1238 /** 1239 * Viral Genotype Susceptibility 1240 */ 1241 _0273, 1242 /** 1243 * Viral Phenotype Susceptibility 1244 */ 1245 _0274, 1246 /** 1247 * Gradient Strip 1248 */ 1249 _0275, 1250 /** 1251 * Minimum Lethal Concentration (MLC) 1252 */ 1253 _0275A, 1254 /** 1255 * Testing to measure the minimum concentration of the antibacterial agent in a given culture medium below which bacterial growth is not inhibited. 1256 */ 1257 _0276, 1258 /** 1259 * Serum bactericidal titer 1260 */ 1261 _0277, 1262 /** 1263 * Agar screen 1264 */ 1265 _0278, 1266 /** 1267 * Disk induction 1268 */ 1269 _0279, 1270 /** 1271 * Molecular, Nucleic acid probe 1272 */ 1273 _0127, 1274 /** 1275 * added to help the parsers 1276 */ 1277 NULL; 1278 public static V3ObservationMethod fromCode(String codeString) throws FHIRException { 1279 if (codeString == null || "".equals(codeString)) 1280 return null; 1281 if ("_DecisionObservationMethod".equals(codeString)) 1282 return _DECISIONOBSERVATIONMETHOD; 1283 if ("ALGM".equals(codeString)) 1284 return ALGM; 1285 if ("BYCL".equals(codeString)) 1286 return BYCL; 1287 if ("GINT".equals(codeString)) 1288 return GINT; 1289 if ("_GeneticObservationMethod".equals(codeString)) 1290 return _GENETICOBSERVATIONMETHOD; 1291 if ("PCR".equals(codeString)) 1292 return PCR; 1293 if ("_ObservationMethodAggregate".equals(codeString)) 1294 return _OBSERVATIONMETHODAGGREGATE; 1295 if ("AVERAGE".equals(codeString)) 1296 return AVERAGE; 1297 if ("COUNT".equals(codeString)) 1298 return COUNT; 1299 if ("MAX".equals(codeString)) 1300 return MAX; 1301 if ("MEDIAN".equals(codeString)) 1302 return MEDIAN; 1303 if ("MIN".equals(codeString)) 1304 return MIN; 1305 if ("MODE".equals(codeString)) 1306 return MODE; 1307 if ("STDEV.P".equals(codeString)) 1308 return STDEV_P; 1309 if ("STDEV.S".equals(codeString)) 1310 return STDEV_S; 1311 if ("SUM".equals(codeString)) 1312 return SUM; 1313 if ("VARIANCE.P".equals(codeString)) 1314 return VARIANCE_P; 1315 if ("VARIANCE.S".equals(codeString)) 1316 return VARIANCE_S; 1317 if ("_VerificationMethod".equals(codeString)) 1318 return _VERIFICATIONMETHOD; 1319 if ("VDOC".equals(codeString)) 1320 return VDOC; 1321 if ("VREG".equals(codeString)) 1322 return VREG; 1323 if ("VTOKEN".equals(codeString)) 1324 return VTOKEN; 1325 if ("VVOICE".equals(codeString)) 1326 return VVOICE; 1327 if ("0001".equals(codeString)) 1328 return _0001; 1329 if ("0002".equals(codeString)) 1330 return _0002; 1331 if ("0003".equals(codeString)) 1332 return _0003; 1333 if ("0004".equals(codeString)) 1334 return _0004; 1335 if ("0005".equals(codeString)) 1336 return _0005; 1337 if ("0006".equals(codeString)) 1338 return _0006; 1339 if ("0007".equals(codeString)) 1340 return _0007; 1341 if ("0008".equals(codeString)) 1342 return _0008; 1343 if ("0009".equals(codeString)) 1344 return _0009; 1345 if ("0010".equals(codeString)) 1346 return _0010; 1347 if ("0011".equals(codeString)) 1348 return _0011; 1349 if ("0012".equals(codeString)) 1350 return _0012; 1351 if ("0013".equals(codeString)) 1352 return _0013; 1353 if ("0014".equals(codeString)) 1354 return _0014; 1355 if ("0015".equals(codeString)) 1356 return _0015; 1357 if ("0016".equals(codeString)) 1358 return _0016; 1359 if ("0017".equals(codeString)) 1360 return _0017; 1361 if ("0018".equals(codeString)) 1362 return _0018; 1363 if ("0019".equals(codeString)) 1364 return _0019; 1365 if ("0020".equals(codeString)) 1366 return _0020; 1367 if ("0021".equals(codeString)) 1368 return _0021; 1369 if ("0022".equals(codeString)) 1370 return _0022; 1371 if ("0023".equals(codeString)) 1372 return _0023; 1373 if ("0024".equals(codeString)) 1374 return _0024; 1375 if ("0025".equals(codeString)) 1376 return _0025; 1377 if ("0026".equals(codeString)) 1378 return _0026; 1379 if ("0027".equals(codeString)) 1380 return _0027; 1381 if ("0028".equals(codeString)) 1382 return _0028; 1383 if ("0029".equals(codeString)) 1384 return _0029; 1385 if ("0030".equals(codeString)) 1386 return _0030; 1387 if ("0031".equals(codeString)) 1388 return _0031; 1389 if ("0032".equals(codeString)) 1390 return _0032; 1391 if ("0033".equals(codeString)) 1392 return _0033; 1393 if ("0034".equals(codeString)) 1394 return _0034; 1395 if ("0035".equals(codeString)) 1396 return _0035; 1397 if ("0036".equals(codeString)) 1398 return _0036; 1399 if ("0037".equals(codeString)) 1400 return _0037; 1401 if ("0038".equals(codeString)) 1402 return _0038; 1403 if ("0039".equals(codeString)) 1404 return _0039; 1405 if ("0040".equals(codeString)) 1406 return _0040; 1407 if ("0041".equals(codeString)) 1408 return _0041; 1409 if ("0042".equals(codeString)) 1410 return _0042; 1411 if ("0043".equals(codeString)) 1412 return _0043; 1413 if ("0044".equals(codeString)) 1414 return _0044; 1415 if ("0045".equals(codeString)) 1416 return _0045; 1417 if ("0046".equals(codeString)) 1418 return _0046; 1419 if ("0047".equals(codeString)) 1420 return _0047; 1421 if ("0048".equals(codeString)) 1422 return _0048; 1423 if ("0049".equals(codeString)) 1424 return _0049; 1425 if ("0050".equals(codeString)) 1426 return _0050; 1427 if ("0051".equals(codeString)) 1428 return _0051; 1429 if ("0052".equals(codeString)) 1430 return _0052; 1431 if ("0053".equals(codeString)) 1432 return _0053; 1433 if ("0054".equals(codeString)) 1434 return _0054; 1435 if ("0055".equals(codeString)) 1436 return _0055; 1437 if ("0056".equals(codeString)) 1438 return _0056; 1439 if ("0057".equals(codeString)) 1440 return _0057; 1441 if ("0058".equals(codeString)) 1442 return _0058; 1443 if ("0059".equals(codeString)) 1444 return _0059; 1445 if ("0060".equals(codeString)) 1446 return _0060; 1447 if ("0061".equals(codeString)) 1448 return _0061; 1449 if ("0062".equals(codeString)) 1450 return _0062; 1451 if ("0063".equals(codeString)) 1452 return _0063; 1453 if ("0064".equals(codeString)) 1454 return _0064; 1455 if ("0065".equals(codeString)) 1456 return _0065; 1457 if ("0066".equals(codeString)) 1458 return _0066; 1459 if ("0067".equals(codeString)) 1460 return _0067; 1461 if ("0068".equals(codeString)) 1462 return _0068; 1463 if ("0069".equals(codeString)) 1464 return _0069; 1465 if ("0070".equals(codeString)) 1466 return _0070; 1467 if ("0071".equals(codeString)) 1468 return _0071; 1469 if ("0072".equals(codeString)) 1470 return _0072; 1471 if ("0073".equals(codeString)) 1472 return _0073; 1473 if ("0074".equals(codeString)) 1474 return _0074; 1475 if ("0075".equals(codeString)) 1476 return _0075; 1477 if ("0076".equals(codeString)) 1478 return _0076; 1479 if ("0077".equals(codeString)) 1480 return _0077; 1481 if ("0078".equals(codeString)) 1482 return _0078; 1483 if ("0079".equals(codeString)) 1484 return _0079; 1485 if ("0080".equals(codeString)) 1486 return _0080; 1487 if ("0081".equals(codeString)) 1488 return _0081; 1489 if ("0082".equals(codeString)) 1490 return _0082; 1491 if ("0083".equals(codeString)) 1492 return _0083; 1493 if ("0084".equals(codeString)) 1494 return _0084; 1495 if ("0085".equals(codeString)) 1496 return _0085; 1497 if ("0086".equals(codeString)) 1498 return _0086; 1499 if ("0087".equals(codeString)) 1500 return _0087; 1501 if ("0088".equals(codeString)) 1502 return _0088; 1503 if ("0089".equals(codeString)) 1504 return _0089; 1505 if ("0090".equals(codeString)) 1506 return _0090; 1507 if ("0091".equals(codeString)) 1508 return _0091; 1509 if ("0092".equals(codeString)) 1510 return _0092; 1511 if ("0093".equals(codeString)) 1512 return _0093; 1513 if ("0094".equals(codeString)) 1514 return _0094; 1515 if ("0095".equals(codeString)) 1516 return _0095; 1517 if ("0096".equals(codeString)) 1518 return _0096; 1519 if ("0097".equals(codeString)) 1520 return _0097; 1521 if ("0098".equals(codeString)) 1522 return _0098; 1523 if ("0099".equals(codeString)) 1524 return _0099; 1525 if ("0100".equals(codeString)) 1526 return _0100; 1527 if ("0101".equals(codeString)) 1528 return _0101; 1529 if ("0102".equals(codeString)) 1530 return _0102; 1531 if ("0103".equals(codeString)) 1532 return _0103; 1533 if ("0104".equals(codeString)) 1534 return _0104; 1535 if ("0105".equals(codeString)) 1536 return _0105; 1537 if ("0106".equals(codeString)) 1538 return _0106; 1539 if ("0107".equals(codeString)) 1540 return _0107; 1541 if ("0108".equals(codeString)) 1542 return _0108; 1543 if ("0109".equals(codeString)) 1544 return _0109; 1545 if ("0110".equals(codeString)) 1546 return _0110; 1547 if ("0111".equals(codeString)) 1548 return _0111; 1549 if ("0112".equals(codeString)) 1550 return _0112; 1551 if ("0113".equals(codeString)) 1552 return _0113; 1553 if ("0114".equals(codeString)) 1554 return _0114; 1555 if ("0115".equals(codeString)) 1556 return _0115; 1557 if ("0116".equals(codeString)) 1558 return _0116; 1559 if ("0117".equals(codeString)) 1560 return _0117; 1561 if ("0118".equals(codeString)) 1562 return _0118; 1563 if ("0119".equals(codeString)) 1564 return _0119; 1565 if ("0120".equals(codeString)) 1566 return _0120; 1567 if ("0121".equals(codeString)) 1568 return _0121; 1569 if ("0122".equals(codeString)) 1570 return _0122; 1571 if ("0123".equals(codeString)) 1572 return _0123; 1573 if ("0124".equals(codeString)) 1574 return _0124; 1575 if ("0125".equals(codeString)) 1576 return _0125; 1577 if ("0126".equals(codeString)) 1578 return _0126; 1579 if ("0128".equals(codeString)) 1580 return _0128; 1581 if ("0129".equals(codeString)) 1582 return _0129; 1583 if ("0130".equals(codeString)) 1584 return _0130; 1585 if ("0131".equals(codeString)) 1586 return _0131; 1587 if ("0132".equals(codeString)) 1588 return _0132; 1589 if ("0133".equals(codeString)) 1590 return _0133; 1591 if ("0134".equals(codeString)) 1592 return _0134; 1593 if ("0135".equals(codeString)) 1594 return _0135; 1595 if ("0136".equals(codeString)) 1596 return _0136; 1597 if ("0137".equals(codeString)) 1598 return _0137; 1599 if ("0138".equals(codeString)) 1600 return _0138; 1601 if ("0139".equals(codeString)) 1602 return _0139; 1603 if ("0140".equals(codeString)) 1604 return _0140; 1605 if ("0141".equals(codeString)) 1606 return _0141; 1607 if ("0142".equals(codeString)) 1608 return _0142; 1609 if ("0143".equals(codeString)) 1610 return _0143; 1611 if ("0144".equals(codeString)) 1612 return _0144; 1613 if ("0145".equals(codeString)) 1614 return _0145; 1615 if ("0146".equals(codeString)) 1616 return _0146; 1617 if ("0147".equals(codeString)) 1618 return _0147; 1619 if ("0148".equals(codeString)) 1620 return _0148; 1621 if ("0149".equals(codeString)) 1622 return _0149; 1623 if ("0150".equals(codeString)) 1624 return _0150; 1625 if ("0151".equals(codeString)) 1626 return _0151; 1627 if ("0152".equals(codeString)) 1628 return _0152; 1629 if ("0153".equals(codeString)) 1630 return _0153; 1631 if ("0154".equals(codeString)) 1632 return _0154; 1633 if ("0155".equals(codeString)) 1634 return _0155; 1635 if ("0156".equals(codeString)) 1636 return _0156; 1637 if ("0157".equals(codeString)) 1638 return _0157; 1639 if ("0158".equals(codeString)) 1640 return _0158; 1641 if ("0159".equals(codeString)) 1642 return _0159; 1643 if ("0160".equals(codeString)) 1644 return _0160; 1645 if ("0161".equals(codeString)) 1646 return _0161; 1647 if ("0162".equals(codeString)) 1648 return _0162; 1649 if ("0163".equals(codeString)) 1650 return _0163; 1651 if ("0164".equals(codeString)) 1652 return _0164; 1653 if ("0165".equals(codeString)) 1654 return _0165; 1655 if ("0166".equals(codeString)) 1656 return _0166; 1657 if ("0167".equals(codeString)) 1658 return _0167; 1659 if ("0168".equals(codeString)) 1660 return _0168; 1661 if ("0169".equals(codeString)) 1662 return _0169; 1663 if ("0170".equals(codeString)) 1664 return _0170; 1665 if ("0171".equals(codeString)) 1666 return _0171; 1667 if ("0172".equals(codeString)) 1668 return _0172; 1669 if ("0173".equals(codeString)) 1670 return _0173; 1671 if ("0174".equals(codeString)) 1672 return _0174; 1673 if ("0175".equals(codeString)) 1674 return _0175; 1675 if ("0176".equals(codeString)) 1676 return _0176; 1677 if ("0177".equals(codeString)) 1678 return _0177; 1679 if ("0178".equals(codeString)) 1680 return _0178; 1681 if ("0179".equals(codeString)) 1682 return _0179; 1683 if ("0180".equals(codeString)) 1684 return _0180; 1685 if ("0181".equals(codeString)) 1686 return _0181; 1687 if ("0182".equals(codeString)) 1688 return _0182; 1689 if ("0183".equals(codeString)) 1690 return _0183; 1691 if ("0184".equals(codeString)) 1692 return _0184; 1693 if ("0185".equals(codeString)) 1694 return _0185; 1695 if ("0186".equals(codeString)) 1696 return _0186; 1697 if ("0187".equals(codeString)) 1698 return _0187; 1699 if ("0188".equals(codeString)) 1700 return _0188; 1701 if ("0189".equals(codeString)) 1702 return _0189; 1703 if ("0190".equals(codeString)) 1704 return _0190; 1705 if ("0191".equals(codeString)) 1706 return _0191; 1707 if ("0192".equals(codeString)) 1708 return _0192; 1709 if ("0193".equals(codeString)) 1710 return _0193; 1711 if ("0194".equals(codeString)) 1712 return _0194; 1713 if ("0195".equals(codeString)) 1714 return _0195; 1715 if ("0196".equals(codeString)) 1716 return _0196; 1717 if ("0197".equals(codeString)) 1718 return _0197; 1719 if ("0198".equals(codeString)) 1720 return _0198; 1721 if ("0199".equals(codeString)) 1722 return _0199; 1723 if ("0200".equals(codeString)) 1724 return _0200; 1725 if ("0201".equals(codeString)) 1726 return _0201; 1727 if ("0202".equals(codeString)) 1728 return _0202; 1729 if ("0203".equals(codeString)) 1730 return _0203; 1731 if ("0204".equals(codeString)) 1732 return _0204; 1733 if ("0205".equals(codeString)) 1734 return _0205; 1735 if ("0206".equals(codeString)) 1736 return _0206; 1737 if ("0207".equals(codeString)) 1738 return _0207; 1739 if ("0208".equals(codeString)) 1740 return _0208; 1741 if ("0209".equals(codeString)) 1742 return _0209; 1743 if ("0210".equals(codeString)) 1744 return _0210; 1745 if ("0211".equals(codeString)) 1746 return _0211; 1747 if ("0212".equals(codeString)) 1748 return _0212; 1749 if ("0213".equals(codeString)) 1750 return _0213; 1751 if ("0214".equals(codeString)) 1752 return _0214; 1753 if ("0215".equals(codeString)) 1754 return _0215; 1755 if ("0216".equals(codeString)) 1756 return _0216; 1757 if ("0217".equals(codeString)) 1758 return _0217; 1759 if ("0218".equals(codeString)) 1760 return _0218; 1761 if ("0219".equals(codeString)) 1762 return _0219; 1763 if ("0220".equals(codeString)) 1764 return _0220; 1765 if ("0221".equals(codeString)) 1766 return _0221; 1767 if ("0222".equals(codeString)) 1768 return _0222; 1769 if ("0223".equals(codeString)) 1770 return _0223; 1771 if ("0224".equals(codeString)) 1772 return _0224; 1773 if ("0225".equals(codeString)) 1774 return _0225; 1775 if ("0226".equals(codeString)) 1776 return _0226; 1777 if ("0227".equals(codeString)) 1778 return _0227; 1779 if ("0228".equals(codeString)) 1780 return _0228; 1781 if ("0229".equals(codeString)) 1782 return _0229; 1783 if ("0230".equals(codeString)) 1784 return _0230; 1785 if ("0231".equals(codeString)) 1786 return _0231; 1787 if ("0232".equals(codeString)) 1788 return _0232; 1789 if ("0233".equals(codeString)) 1790 return _0233; 1791 if ("0234".equals(codeString)) 1792 return _0234; 1793 if ("0235".equals(codeString)) 1794 return _0235; 1795 if ("0236".equals(codeString)) 1796 return _0236; 1797 if ("0237".equals(codeString)) 1798 return _0237; 1799 if ("0238".equals(codeString)) 1800 return _0238; 1801 if ("0239".equals(codeString)) 1802 return _0239; 1803 if ("0243".equals(codeString)) 1804 return _0243; 1805 if ("0244".equals(codeString)) 1806 return _0244; 1807 if ("0247".equals(codeString)) 1808 return _0247; 1809 if ("0248".equals(codeString)) 1810 return _0248; 1811 if ("0249".equals(codeString)) 1812 return _0249; 1813 if ("0250".equals(codeString)) 1814 return _0250; 1815 if ("0251".equals(codeString)) 1816 return _0251; 1817 if ("0252".equals(codeString)) 1818 return _0252; 1819 if ("0253".equals(codeString)) 1820 return _0253; 1821 if ("0254".equals(codeString)) 1822 return _0254; 1823 if ("0255".equals(codeString)) 1824 return _0255; 1825 if ("0256".equals(codeString)) 1826 return _0256; 1827 if ("0257".equals(codeString)) 1828 return _0257; 1829 if ("0258".equals(codeString)) 1830 return _0258; 1831 if ("0259".equals(codeString)) 1832 return _0259; 1833 if ("0260".equals(codeString)) 1834 return _0260; 1835 if ("0261".equals(codeString)) 1836 return _0261; 1837 if ("0262".equals(codeString)) 1838 return _0262; 1839 if ("0263".equals(codeString)) 1840 return _0263; 1841 if ("0264".equals(codeString)) 1842 return _0264; 1843 if ("0265".equals(codeString)) 1844 return _0265; 1845 if ("0266".equals(codeString)) 1846 return _0266; 1847 if ("0267".equals(codeString)) 1848 return _0267; 1849 if ("0268".equals(codeString)) 1850 return _0268; 1851 if ("0269".equals(codeString)) 1852 return _0269; 1853 if ("0270".equals(codeString)) 1854 return _0270; 1855 if ("0271".equals(codeString)) 1856 return _0271; 1857 if ("0280".equals(codeString)) 1858 return _0280; 1859 if ("0240".equals(codeString)) 1860 return _0240; 1861 if ("0241".equals(codeString)) 1862 return _0241; 1863 if ("0242".equals(codeString)) 1864 return _0242; 1865 if ("0272".equals(codeString)) 1866 return _0272; 1867 if ("0245".equals(codeString)) 1868 return _0245; 1869 if ("0246".equals(codeString)) 1870 return _0246; 1871 if ("0273".equals(codeString)) 1872 return _0273; 1873 if ("0274".equals(codeString)) 1874 return _0274; 1875 if ("0275".equals(codeString)) 1876 return _0275; 1877 if ("0275a".equals(codeString)) 1878 return _0275A; 1879 if ("0276".equals(codeString)) 1880 return _0276; 1881 if ("0277".equals(codeString)) 1882 return _0277; 1883 if ("0278".equals(codeString)) 1884 return _0278; 1885 if ("0279".equals(codeString)) 1886 return _0279; 1887 if ("0127".equals(codeString)) 1888 return _0127; 1889 throw new FHIRException("Unknown V3ObservationMethod code '"+codeString+"'"); 1890 } 1891 public String toCode() { 1892 switch (this) { 1893 case _DECISIONOBSERVATIONMETHOD: return "_DecisionObservationMethod"; 1894 case ALGM: return "ALGM"; 1895 case BYCL: return "BYCL"; 1896 case GINT: return "GINT"; 1897 case _GENETICOBSERVATIONMETHOD: return "_GeneticObservationMethod"; 1898 case PCR: return "PCR"; 1899 case _OBSERVATIONMETHODAGGREGATE: return "_ObservationMethodAggregate"; 1900 case AVERAGE: return "AVERAGE"; 1901 case COUNT: return "COUNT"; 1902 case MAX: return "MAX"; 1903 case MEDIAN: return "MEDIAN"; 1904 case MIN: return "MIN"; 1905 case MODE: return "MODE"; 1906 case STDEV_P: return "STDEV.P"; 1907 case STDEV_S: return "STDEV.S"; 1908 case SUM: return "SUM"; 1909 case VARIANCE_P: return "VARIANCE.P"; 1910 case VARIANCE_S: return "VARIANCE.S"; 1911 case _VERIFICATIONMETHOD: return "_VerificationMethod"; 1912 case VDOC: return "VDOC"; 1913 case VREG: return "VREG"; 1914 case VTOKEN: return "VTOKEN"; 1915 case VVOICE: return "VVOICE"; 1916 case _0001: return "0001"; 1917 case _0002: return "0002"; 1918 case _0003: return "0003"; 1919 case _0004: return "0004"; 1920 case _0005: return "0005"; 1921 case _0006: return "0006"; 1922 case _0007: return "0007"; 1923 case _0008: return "0008"; 1924 case _0009: return "0009"; 1925 case _0010: return "0010"; 1926 case _0011: return "0011"; 1927 case _0012: return "0012"; 1928 case _0013: return "0013"; 1929 case _0014: return "0014"; 1930 case _0015: return "0015"; 1931 case _0016: return "0016"; 1932 case _0017: return "0017"; 1933 case _0018: return "0018"; 1934 case _0019: return "0019"; 1935 case _0020: return "0020"; 1936 case _0021: return "0021"; 1937 case _0022: return "0022"; 1938 case _0023: return "0023"; 1939 case _0024: return "0024"; 1940 case _0025: return "0025"; 1941 case _0026: return "0026"; 1942 case _0027: return "0027"; 1943 case _0028: return "0028"; 1944 case _0029: return "0029"; 1945 case _0030: return "0030"; 1946 case _0031: return "0031"; 1947 case _0032: return "0032"; 1948 case _0033: return "0033"; 1949 case _0034: return "0034"; 1950 case _0035: return "0035"; 1951 case _0036: return "0036"; 1952 case _0037: return "0037"; 1953 case _0038: return "0038"; 1954 case _0039: return "0039"; 1955 case _0040: return "0040"; 1956 case _0041: return "0041"; 1957 case _0042: return "0042"; 1958 case _0043: return "0043"; 1959 case _0044: return "0044"; 1960 case _0045: return "0045"; 1961 case _0046: return "0046"; 1962 case _0047: return "0047"; 1963 case _0048: return "0048"; 1964 case _0049: return "0049"; 1965 case _0050: return "0050"; 1966 case _0051: return "0051"; 1967 case _0052: return "0052"; 1968 case _0053: return "0053"; 1969 case _0054: return "0054"; 1970 case _0055: return "0055"; 1971 case _0056: return "0056"; 1972 case _0057: return "0057"; 1973 case _0058: return "0058"; 1974 case _0059: return "0059"; 1975 case _0060: return "0060"; 1976 case _0061: return "0061"; 1977 case _0062: return "0062"; 1978 case _0063: return "0063"; 1979 case _0064: return "0064"; 1980 case _0065: return "0065"; 1981 case _0066: return "0066"; 1982 case _0067: return "0067"; 1983 case _0068: return "0068"; 1984 case _0069: return "0069"; 1985 case _0070: return "0070"; 1986 case _0071: return "0071"; 1987 case _0072: return "0072"; 1988 case _0073: return "0073"; 1989 case _0074: return "0074"; 1990 case _0075: return "0075"; 1991 case _0076: return "0076"; 1992 case _0077: return "0077"; 1993 case _0078: return "0078"; 1994 case _0079: return "0079"; 1995 case _0080: return "0080"; 1996 case _0081: return "0081"; 1997 case _0082: return "0082"; 1998 case _0083: return "0083"; 1999 case _0084: return "0084"; 2000 case _0085: return "0085"; 2001 case _0086: return "0086"; 2002 case _0087: return "0087"; 2003 case _0088: return "0088"; 2004 case _0089: return "0089"; 2005 case _0090: return "0090"; 2006 case _0091: return "0091"; 2007 case _0092: return "0092"; 2008 case _0093: return "0093"; 2009 case _0094: return "0094"; 2010 case _0095: return "0095"; 2011 case _0096: return "0096"; 2012 case _0097: return "0097"; 2013 case _0098: return "0098"; 2014 case _0099: return "0099"; 2015 case _0100: return "0100"; 2016 case _0101: return "0101"; 2017 case _0102: return "0102"; 2018 case _0103: return "0103"; 2019 case _0104: return "0104"; 2020 case _0105: return "0105"; 2021 case _0106: return "0106"; 2022 case _0107: return "0107"; 2023 case _0108: return "0108"; 2024 case _0109: return "0109"; 2025 case _0110: return "0110"; 2026 case _0111: return "0111"; 2027 case _0112: return "0112"; 2028 case _0113: return "0113"; 2029 case _0114: return "0114"; 2030 case _0115: return "0115"; 2031 case _0116: return "0116"; 2032 case _0117: return "0117"; 2033 case _0118: return "0118"; 2034 case _0119: return "0119"; 2035 case _0120: return "0120"; 2036 case _0121: return "0121"; 2037 case _0122: return "0122"; 2038 case _0123: return "0123"; 2039 case _0124: return "0124"; 2040 case _0125: return "0125"; 2041 case _0126: return "0126"; 2042 case _0128: return "0128"; 2043 case _0129: return "0129"; 2044 case _0130: return "0130"; 2045 case _0131: return "0131"; 2046 case _0132: return "0132"; 2047 case _0133: return "0133"; 2048 case _0134: return "0134"; 2049 case _0135: return "0135"; 2050 case _0136: return "0136"; 2051 case _0137: return "0137"; 2052 case _0138: return "0138"; 2053 case _0139: return "0139"; 2054 case _0140: return "0140"; 2055 case _0141: return "0141"; 2056 case _0142: return "0142"; 2057 case _0143: return "0143"; 2058 case _0144: return "0144"; 2059 case _0145: return "0145"; 2060 case _0146: return "0146"; 2061 case _0147: return "0147"; 2062 case _0148: return "0148"; 2063 case _0149: return "0149"; 2064 case _0150: return "0150"; 2065 case _0151: return "0151"; 2066 case _0152: return "0152"; 2067 case _0153: return "0153"; 2068 case _0154: return "0154"; 2069 case _0155: return "0155"; 2070 case _0156: return "0156"; 2071 case _0157: return "0157"; 2072 case _0158: return "0158"; 2073 case _0159: return "0159"; 2074 case _0160: return "0160"; 2075 case _0161: return "0161"; 2076 case _0162: return "0162"; 2077 case _0163: return "0163"; 2078 case _0164: return "0164"; 2079 case _0165: return "0165"; 2080 case _0166: return "0166"; 2081 case _0167: return "0167"; 2082 case _0168: return "0168"; 2083 case _0169: return "0169"; 2084 case _0170: return "0170"; 2085 case _0171: return "0171"; 2086 case _0172: return "0172"; 2087 case _0173: return "0173"; 2088 case _0174: return "0174"; 2089 case _0175: return "0175"; 2090 case _0176: return "0176"; 2091 case _0177: return "0177"; 2092 case _0178: return "0178"; 2093 case _0179: return "0179"; 2094 case _0180: return "0180"; 2095 case _0181: return "0181"; 2096 case _0182: return "0182"; 2097 case _0183: return "0183"; 2098 case _0184: return "0184"; 2099 case _0185: return "0185"; 2100 case _0186: return "0186"; 2101 case _0187: return "0187"; 2102 case _0188: return "0188"; 2103 case _0189: return "0189"; 2104 case _0190: return "0190"; 2105 case _0191: return "0191"; 2106 case _0192: return "0192"; 2107 case _0193: return "0193"; 2108 case _0194: return "0194"; 2109 case _0195: return "0195"; 2110 case _0196: return "0196"; 2111 case _0197: return "0197"; 2112 case _0198: return "0198"; 2113 case _0199: return "0199"; 2114 case _0200: return "0200"; 2115 case _0201: return "0201"; 2116 case _0202: return "0202"; 2117 case _0203: return "0203"; 2118 case _0204: return "0204"; 2119 case _0205: return "0205"; 2120 case _0206: return "0206"; 2121 case _0207: return "0207"; 2122 case _0208: return "0208"; 2123 case _0209: return "0209"; 2124 case _0210: return "0210"; 2125 case _0211: return "0211"; 2126 case _0212: return "0212"; 2127 case _0213: return "0213"; 2128 case _0214: return "0214"; 2129 case _0215: return "0215"; 2130 case _0216: return "0216"; 2131 case _0217: return "0217"; 2132 case _0218: return "0218"; 2133 case _0219: return "0219"; 2134 case _0220: return "0220"; 2135 case _0221: return "0221"; 2136 case _0222: return "0222"; 2137 case _0223: return "0223"; 2138 case _0224: return "0224"; 2139 case _0225: return "0225"; 2140 case _0226: return "0226"; 2141 case _0227: return "0227"; 2142 case _0228: return "0228"; 2143 case _0229: return "0229"; 2144 case _0230: return "0230"; 2145 case _0231: return "0231"; 2146 case _0232: return "0232"; 2147 case _0233: return "0233"; 2148 case _0234: return "0234"; 2149 case _0235: return "0235"; 2150 case _0236: return "0236"; 2151 case _0237: return "0237"; 2152 case _0238: return "0238"; 2153 case _0239: return "0239"; 2154 case _0243: return "0243"; 2155 case _0244: return "0244"; 2156 case _0247: return "0247"; 2157 case _0248: return "0248"; 2158 case _0249: return "0249"; 2159 case _0250: return "0250"; 2160 case _0251: return "0251"; 2161 case _0252: return "0252"; 2162 case _0253: return "0253"; 2163 case _0254: return "0254"; 2164 case _0255: return "0255"; 2165 case _0256: return "0256"; 2166 case _0257: return "0257"; 2167 case _0258: return "0258"; 2168 case _0259: return "0259"; 2169 case _0260: return "0260"; 2170 case _0261: return "0261"; 2171 case _0262: return "0262"; 2172 case _0263: return "0263"; 2173 case _0264: return "0264"; 2174 case _0265: return "0265"; 2175 case _0266: return "0266"; 2176 case _0267: return "0267"; 2177 case _0268: return "0268"; 2178 case _0269: return "0269"; 2179 case _0270: return "0270"; 2180 case _0271: return "0271"; 2181 case _0280: return "0280"; 2182 case _0240: return "0240"; 2183 case _0241: return "0241"; 2184 case _0242: return "0242"; 2185 case _0272: return "0272"; 2186 case _0245: return "0245"; 2187 case _0246: return "0246"; 2188 case _0273: return "0273"; 2189 case _0274: return "0274"; 2190 case _0275: return "0275"; 2191 case _0275A: return "0275a"; 2192 case _0276: return "0276"; 2193 case _0277: return "0277"; 2194 case _0278: return "0278"; 2195 case _0279: return "0279"; 2196 case _0127: return "0127"; 2197 case NULL: return null; 2198 default: return "?"; 2199 } 2200 } 2201 public String getSystem() { 2202 return "http://hl7.org/fhir/v3/ObservationMethod"; 2203 } 2204 public String getDefinition() { 2205 switch (this) { 2206 case _DECISIONOBSERVATIONMETHOD: return "Provides codes for decision methods, initially for assessing the causality of events."; 2207 case ALGM: return "Reaching a decision through the application of an algorithm designed to weigh the different factors involved."; 2208 case BYCL: return "Reaching a decision through the use of Bayesian statistical analysis."; 2209 case GINT: return "Reaching a decision by consideration of the totality of factors involved in order to reach a judgement."; 2210 case _GENETICOBSERVATIONMETHOD: return "A code that provides additional detail about the means or technique used to ascertain the genetic analysis. Example, PCR, Micro Array"; 2211 case PCR: return "Description: Polymerase Chain Reaction"; 2212 case _OBSERVATIONMETHODAGGREGATE: return "Provides additional detail about the aggregation methods used to compute the aggregated values for an observation. This is an abstract code."; 2213 case AVERAGE: return "Average of non-null values in the referenced set of values"; 2214 case COUNT: return "Count of non-null values in the referenced set of values"; 2215 case MAX: return "Largest of all non-null values in the referenced set of values."; 2216 case MEDIAN: return "The median of all non-null values in the referenced set of values."; 2217 case MIN: return "Smallest of all non-null values in the referenced set of values."; 2218 case MODE: return "The most common value of all non-null values in the referenced set of values."; 2219 case STDEV_P: return "Standard Deviation of the values in the referenced set of values, computed over the population."; 2220 case STDEV_S: return "Standard Deviation of the values in the referenced set of values, computed over a sample of the population."; 2221 case SUM: return "Sum of non-null values in the referenced set of values"; 2222 case VARIANCE_P: return "Variance of the values in the referenced set of values, computed over the population."; 2223 case VARIANCE_S: return "Variance of the values in the referenced set of values, computed over a sample of the population."; 2224 case _VERIFICATIONMETHOD: return "VerificationMethod"; 2225 case VDOC: return "Verification by means of document.\r\n\n \n Example: Fax, letter, attachment to e-mail."; 2226 case VREG: return "verification by means of a response to an electronic query\r\n\n \n Example: query message to a Covered Party registry application or Coverage Administrator."; 2227 case VTOKEN: return "Verification by means of electronic token.\r\n\n \n Example: smartcard, magnetic swipe card, RFID device."; 2228 case VVOICE: return "Verification by means of voice.\r\n\n \n Example: By speaking with or calling the Coverage Administrator or Covered Party"; 2229 case _0001: return "Complement fixation"; 2230 case _0002: return "Computed axial tomography"; 2231 case _0003: return "Susceptibility, High Level Aminoglycoside Resistance agar test"; 2232 case _0004: return "Visual, Macroscopic observation"; 2233 case _0005: return "Computed, Magnetic resonance"; 2234 case _0006: return "Computed, Morphometry"; 2235 case _0007: return "Computed, Positron emission tomography"; 2236 case _0008: return "SAMHSA drug assay confirmation"; 2237 case _0009: return "SAMHSA drug assay screening"; 2238 case _0010: return "Serum Neutralization"; 2239 case _0011: return "Titration"; 2240 case _0012: return "Ultrasound"; 2241 case _0013: return "X-ray crystallography"; 2242 case _0014: return "Agglutination"; 2243 case _0015: return "Agglutination, Buffered acidified plate"; 2244 case _0016: return "Agglutination, Card"; 2245 case _0017: return "Agglutination, Hemagglutination"; 2246 case _0018: return "Agglutination, Hemagglutination inhibition"; 2247 case _0019: return "Agglutination, Latex"; 2248 case _0020: return "Agglutination, Plate"; 2249 case _0021: return "Agglutination, Rapid Plate"; 2250 case _0022: return "Agglutination, RBC"; 2251 case _0023: return "Agglutination, Rivanol"; 2252 case _0024: return "Agglutination, Tube"; 2253 case _0025: return "Bioassay"; 2254 case _0026: return "Bioassay, Animal Inoculation"; 2255 case _0027: return "Bioassay, Cytotoxicity"; 2256 case _0028: return "Bioassay, Embryo Infective Dose 50"; 2257 case _0029: return "Bioassay, Embryo Lethal Dose 50"; 2258 case _0030: return "Bioassay, Mouse intercerebral inoculation"; 2259 case _0031: return "Bioassay, qualitative"; 2260 case _0032: return "Bioassay, quantitative"; 2261 case _0033: return "Chemical"; 2262 case _0034: return "Chemical, Differential light absorption"; 2263 case _0035: return "Chemical, Dipstick"; 2264 case _0036: return "Chemical, Dipstick colorimetric laboratory test"; 2265 case _0037: return "Chemical, Test strip"; 2266 case _0038: return "Chromatography"; 2267 case _0039: return "Chromatography, Affinity"; 2268 case _0040: return "Chromatography, Gas liquid"; 2269 case _0041: return "Chromatography, High performance liquid"; 2270 case _0042: return "Chromatography, Liquid"; 2271 case _0043: return "Chromatography, Protein A affinity"; 2272 case _0044: return "Coagulation"; 2273 case _0045: return "Coagulation, Tilt tube"; 2274 case _0046: return "Coagulation, Tilt tube reptilase induced"; 2275 case _0047: return "Count, Automated"; 2276 case _0048: return "Count, Manual"; 2277 case _0049: return "Count, Platelet, Rees-Ecker"; 2278 case _0050: return "Culture, Aerobic"; 2279 case _0051: return "Culture, Anaerobic"; 2280 case _0052: return "Culture, Chicken Embryo"; 2281 case _0053: return "Culture, Delayed secondary enrichment"; 2282 case _0054: return "Culture, Microaerophilic"; 2283 case _0055: return "Culture, Quantitative microbial, cup"; 2284 case _0056: return "Culture, Quantitative microbial, droplet"; 2285 case _0057: return "Culture, Quantitative microbial, filter paper"; 2286 case _0058: return "Culture, Quantitative microbial, pad"; 2287 case _0059: return "Culture, Quantitative microbial, pour plate"; 2288 case _0060: return "Culture, Quantitative microbial, surface streak"; 2289 case _0061: return "Culture, Somatic Cell"; 2290 case _0062: return "Diffusion, Agar"; 2291 case _0063: return "Diffusion, Agar Gel Immunodiffusion"; 2292 case _0064: return "Electrophoresis"; 2293 case _0065: return "Electrophoresis, Agaorse gel"; 2294 case _0066: return "Electrophoresis, citrate agar"; 2295 case _0067: return "Electrophoresis, Immuno"; 2296 case _0068: return "Electrophoresis, Polyacrylamide gel"; 2297 case _0069: return "Electrophoresis, Starch gel"; 2298 case _0070: return "ELISA"; 2299 case _0071: return "ELISA, antigen capture"; 2300 case _0072: return "ELISA, avidin biotin peroxidase complex"; 2301 case _0073: return "ELISA, Kinetic"; 2302 case _0074: return "ELISA, peroxidase-antiperoxidase"; 2303 case _0075: return "Identification, API 20 Strep"; 2304 case _0076: return "Identification, API 20A"; 2305 case _0077: return "Identification, API 20C AUX"; 2306 case _0078: return "Identification, API 20E"; 2307 case _0079: return "Identification, API 20NE"; 2308 case _0080: return "Identification, API 50 CH"; 2309 case _0081: return "Identification, API An-IDENT"; 2310 case _0082: return "Identification, API Coryne"; 2311 case _0083: return "Identification, API Rapid 20E"; 2312 case _0084: return "Identification, API Staph"; 2313 case _0085: return "Identification, API ZYM"; 2314 case _0086: return "Identification, Bacterial"; 2315 case _0087: return "Identification, mini VIDAS"; 2316 case _0088: return "Identification, Phage susceptibility typing"; 2317 case _0089: return "Identification, Quad-FERM+"; 2318 case _0090: return "Identification, RAPIDEC Staph"; 2319 case _0091: return "Identification, Staphaurex"; 2320 case _0092: return "Identification, VIDAS"; 2321 case _0093: return "Identification, Vitek"; 2322 case _0094: return "Identification, VITEK 2"; 2323 case _0095: return "Immune stain"; 2324 case _0096: return "Immune stain, Immunofluorescent antibody, direct"; 2325 case _0097: return "Immune stain, Immunofluorescent antibody, indirect"; 2326 case _0098: return "Immune stain, Immunoperoxidase, Avidin-Biotin Complex"; 2327 case _0099: return "Immune stain, Immunoperoxidase, Peroxidase anti-peroxidase complex"; 2328 case _0100: return "Immune stain, Immunoperoxidase, Protein A-peroxidase complex"; 2329 case _0101: return "Immunoassay"; 2330 case _0102: return "Immunoassay, qualitative, multiple step"; 2331 case _0103: return "Immunoassay, qualitative, single step"; 2332 case _0104: return "Immunoassay, Radioimmunoassay"; 2333 case _0105: return "Immunoassay, semi-quantitative, multiple step"; 2334 case _0106: return "Immunoassay, semi-quantitative, single step"; 2335 case _0107: return "Microscopy"; 2336 case _0108: return "Microscopy, Darkfield"; 2337 case _0109: return "Microscopy, Electron"; 2338 case _0110: return "Microscopy, Electron microscopy tomography"; 2339 case _0111: return "Microscopy, Electron, negative stain"; 2340 case _0112: return "Microscopy, Electron, thick section transmission"; 2341 case _0113: return "Microscopy, Electron, thin section transmission"; 2342 case _0114: return "Microscopy, Light"; 2343 case _0115: return "Microscopy, Polarized light"; 2344 case _0116: return "Microscopy, Scanning electron"; 2345 case _0117: return "Microscopy, Transmission electron"; 2346 case _0118: return "Microscopy, Transparent tape direct examination"; 2347 case _0119: return "Molecular, 3 Self-Sustaining Sequence Replication"; 2348 case _0120: return "Molecular, Branched Chain DNA"; 2349 case _0121: return "Molecular, Hybridization Protection Assay"; 2350 case _0122: return "Molecular, Immune blot"; 2351 case _0123: return "Molecular, In-situ hybridization"; 2352 case _0124: return "Molecular, Ligase Chain Reaction"; 2353 case _0125: return "Molecular, Ligation Activated Transcription"; 2354 case _0126: return "Molecular, Nucleic Acid Probe"; 2355 case _0128: return "Molecular, Nucleic acid probe with amplification\r\n\n \r\n\n Rationale: Duplicate of code 0126. Use code 0126 instead."; 2356 case _0129: return "Molecular, Nucleic acid probe with target amplification"; 2357 case _0130: return "Molecular, Nucleic acid reverse transcription"; 2358 case _0131: return "Molecular, Nucleic Acid Sequence Based Analysis"; 2359 case _0132: return "Molecular, Polymerase chain reaction"; 2360 case _0133: return "Molecular, Q-Beta Replicase or probe amplification category method"; 2361 case _0134: return "Molecular, Restriction Fragment Length Polymorphism"; 2362 case _0135: return "Molecular, Southern Blot"; 2363 case _0136: return "Molecular, Strand Displacement Amplification"; 2364 case _0137: return "Molecular, Transcription Mediated Amplification"; 2365 case _0138: return "Molecular, Western Blot"; 2366 case _0139: return "Precipitation, Flocculation"; 2367 case _0140: return "Precipitation, Immune precipitation"; 2368 case _0141: return "Precipitation, Milk ring test"; 2369 case _0142: return "Precipitation, Precipitin"; 2370 case _0143: return "Stain, Acid fast"; 2371 case _0144: return "Stain, Acid fast, fluorochrome"; 2372 case _0145: return "Stain, Acid fast, Kinyoun's cold carbolfuchsin"; 2373 case _0146: return "Stain, Acid fast, Ziehl-Neelsen"; 2374 case _0147: return "Stain, Acid phosphatase"; 2375 case _0148: return "Stain, Acridine orange"; 2376 case _0149: return "Stain, Active brilliant orange KH"; 2377 case _0150: return "Stain, Alazarin red S"; 2378 case _0151: return "Stain, Alcian blue"; 2379 case _0152: return "Stain, Alcian blue with Periodic acid Schiff"; 2380 case _0153: return "Stain, Argentaffin"; 2381 case _0154: return "Stain, Argentaffin silver"; 2382 case _0155: return "Stain, Azure-eosin"; 2383 case _0156: return "Stain, Basic Fuschin"; 2384 case _0157: return "Stain, Bennhold"; 2385 case _0158: return "Stain, Bennhold's Congo red"; 2386 case _0159: return "Stain, Bielschowsky"; 2387 case _0160: return "Stain, Bielschowsky's silver"; 2388 case _0161: return "Stain, Bleach"; 2389 case _0162: return "Stain, Bodian"; 2390 case _0163: return "Stain, Brown-Brenn"; 2391 case _0164: return "Stain, Butyrate-esterase"; 2392 case _0165: return "Stain, Calcofluor white fluorescent"; 2393 case _0166: return "Stain, Carbol-fuchsin"; 2394 case _0167: return "Stain, Carmine"; 2395 case _0168: return "Stain, Churukian-Schenk"; 2396 case _0169: return "Stain, Congo red"; 2397 case _0170: return "Stain, Cresyl echt violet"; 2398 case _0171: return "Stain, Crystal violet"; 2399 case _0172: return "Stain, De Galantha"; 2400 case _0173: return "Stain, Dieterle silver impregnation"; 2401 case _0174: return "Stain, Fite-Farco"; 2402 case _0175: return "Stain, Fontana-Masson silver"; 2403 case _0176: return "Stain, Fouchet"; 2404 case _0177: return "Stain, Gomori"; 2405 case _0178: return "Stain, Gomori methenamine silver"; 2406 case _0179: return "Stain, Gomori-Wheatly trichrome"; 2407 case _0180: return "Stain, Gridley"; 2408 case _0181: return "Stain, Grimelius silver"; 2409 case _0182: return "Stain, Grocott"; 2410 case _0183: return "Stain, Grocott methenamine silver"; 2411 case _0184: return "Stain, Hale's colloidal ferric oxide"; 2412 case _0185: return "Stain, Hale's colloidal iron"; 2413 case _0186: return "Stain, Hansel"; 2414 case _0187: return "Stain, Harris regressive hematoxylin and eosin"; 2415 case _0188: return "Stain, Hematoxylin and eosin"; 2416 case _0189: return "Stain, Highman"; 2417 case _0190: return "Stain, Holzer"; 2418 case _0191: return "Stain, Iron hematoxylin"; 2419 case _0192: return "Stain, Jones"; 2420 case _0193: return "Stain, Jones methenamine silver"; 2421 case _0194: return "Stain, Kossa"; 2422 case _0195: return "Stain, Lawson-Van Gieson"; 2423 case _0196: return "Stain, Loeffler methylene blue"; 2424 case _0197: return "Stain, Luxol fast blue with cresyl violet"; 2425 case _0198: return "Stain, Luxol fast blue with Periodic acid-Schiff"; 2426 case _0199: return "Stain, MacNeal's tetrachrome blood"; 2427 case _0200: return "Stain, Mallory-Heidenhain"; 2428 case _0201: return "Stain, Masson trichrome"; 2429 case _0202: return "Stain, Mayer mucicarmine"; 2430 case _0203: return "Stain, Mayers progressive hematoxylin and eosin"; 2431 case _0204: return "Stain, May-Grunwald Giemsa"; 2432 case _0205: return "Stain, Methyl green"; 2433 case _0206: return "Stain, Methyl green pyronin"; 2434 case _0207: return "Stain, Modified Gomori-Wheatly trichrome"; 2435 case _0208: return "Stain, Modified Masson trichrome"; 2436 case _0209: return "Stain, Modified trichrome"; 2437 case _0210: return "Stain, Movat pentachrome"; 2438 case _0211: return "Stain, Mucicarmine"; 2439 case _0212: return "Stain, Neutral red"; 2440 case _0213: return "Stain, Night blue"; 2441 case _0214: return "Stain, Non-specific esterase"; 2442 case _0215: return "Stain, Oil red-O"; 2443 case _0216: return "Stain, Orcein"; 2444 case _0217: return "Stain, Perls'"; 2445 case _0218: return "Stain, Phosphotungstic acid-hematoxylin"; 2446 case _0219: return "Stain, Potassium ferrocyanide"; 2447 case _0220: return "Stain, Prussian blue"; 2448 case _0221: return "Stain, Putchler modified Bennhold"; 2449 case _0222: return "Stain, Quinacrine fluorescent"; 2450 case _0223: return "Stain, Reticulin"; 2451 case _0224: return "Stain, Rhodamine"; 2452 case _0225: return "Stain, Safranin"; 2453 case _0226: return "Stain, Schmorl"; 2454 case _0227: return "Stain, Seiver-Munger"; 2455 case _0228: return "Stain, Silver"; 2456 case _0229: return "Stain, Specific esterase"; 2457 case _0230: return "Stain, Steiner silver"; 2458 case _0231: return "Stain, Sudan III"; 2459 case _0232: return "Stain, Sudan IVI"; 2460 case _0233: return "Stain, Sulfated alcian blue"; 2461 case _0234: return "Stain, Supravital"; 2462 case _0235: return "Stain, Thioflavine-S"; 2463 case _0236: return "Stain, Three micron Giemsa"; 2464 case _0237: return "Stain, Vassar-Culling"; 2465 case _0238: return "Stain, Vital"; 2466 case _0239: return "Stain, von Kossa"; 2467 case _0243: return "Susceptibility, Minimum bactericidal concentration, macrodilution"; 2468 case _0244: return "Susceptibility, Minimum bactericidal concentration, microdilution"; 2469 case _0247: return "Turbidometric"; 2470 case _0248: return "Turbidometric, Refractometric"; 2471 case _0249: return "Chromatography, Thin Layer"; 2472 case _0250: return "Immunoassay, enzyme-multiplied technique (EMIT)"; 2473 case _0251: return "Flow Cytometry"; 2474 case _0252: return "Radial Immunodiffusion"; 2475 case _0253: return "Immunoassay, Fluorescence Polarization"; 2476 case _0254: return "Electrophoresis, Immunofixation"; 2477 case _0255: return "Dialysis, Direct Equilibrium"; 2478 case _0256: return "Acid Elution, Kleihauer-Betke Method"; 2479 case _0257: return "Immunofluorescence, Anti-Complement"; 2480 case _0258: return "Gas Chromatography/Mass Spectroscopy"; 2481 case _0259: return "Light Scatter, Nephelometry"; 2482 case _0260: return "Immunoassay, IgE Antibody Test"; 2483 case _0261: return "Lymphocyte Microcytotoxicity Assay"; 2484 case _0262: return "Spectrophotometry"; 2485 case _0263: return "Spectrophotometry, Atomic Absorption"; 2486 case _0264: return "Electrochemical, Ion Selective Electrode"; 2487 case _0265: return "Chromatography, Gas"; 2488 case _0266: return "Isoelectric Focusing"; 2489 case _0267: return "Immunoassay, Chemiluminescent"; 2490 case _0268: return "Immunoassay, Microparticle Enzyme"; 2491 case _0269: return "Inductively-Coupled Plasma/Mass Spectrometry"; 2492 case _0270: return "Immunoassay, Immunoradiometric Assay"; 2493 case _0271: return "Coagulation, Photo Optical Clot Detection"; 2494 case _0280: return "Test methods designed to determine a microorganismaTMs susceptibility to being killed by an antibiotic."; 2495 case _0240: return "Susceptibility, Antibiotic sensitivity, disk"; 2496 case _0241: return "Susceptibility, BACTEC susceptibility test"; 2497 case _0242: return "Susceptibility, Disk dilution"; 2498 case _0272: return "Testing to measure the minimum concentration of the antibacterial agent in a given culture medium below which bacterial growth is not inhibited."; 2499 case _0245: return "Susceptibility, Minimum Inhibitory concentration, macrodilution"; 2500 case _0246: return "Susceptibility, Minimum Inhibitory concentration, microdilution"; 2501 case _0273: return "Viral Genotype Susceptibility"; 2502 case _0274: return "Viral Phenotype Susceptibility"; 2503 case _0275: return "Gradient Strip"; 2504 case _0275A: return "Minimum Lethal Concentration (MLC)"; 2505 case _0276: return "Testing to measure the minimum concentration of the antibacterial agent in a given culture medium below which bacterial growth is not inhibited."; 2506 case _0277: return "Serum bactericidal titer"; 2507 case _0278: return "Agar screen"; 2508 case _0279: return "Disk induction"; 2509 case _0127: return "Molecular, Nucleic acid probe"; 2510 case NULL: return null; 2511 default: return "?"; 2512 } 2513 } 2514 public String getDisplay() { 2515 switch (this) { 2516 case _DECISIONOBSERVATIONMETHOD: return "DecisionObservationMethod"; 2517 case ALGM: return "algorithm"; 2518 case BYCL: return "bayesian calculation"; 2519 case GINT: return "global introspection"; 2520 case _GENETICOBSERVATIONMETHOD: return "GeneticObservationMethod"; 2521 case PCR: return "PCR"; 2522 case _OBSERVATIONMETHODAGGREGATE: return "observation method aggregate"; 2523 case AVERAGE: return "average"; 2524 case COUNT: return "count"; 2525 case MAX: return "maxima"; 2526 case MEDIAN: return "median"; 2527 case MIN: return "minima"; 2528 case MODE: return "mode"; 2529 case STDEV_P: return "population standard deviation"; 2530 case STDEV_S: return "sample standard deviation"; 2531 case SUM: return "sum"; 2532 case VARIANCE_P: return "population variance"; 2533 case VARIANCE_S: return "sample variance"; 2534 case _VERIFICATIONMETHOD: return "VerificationMethod"; 2535 case VDOC: return "document verification"; 2536 case VREG: return "registry verification"; 2537 case VTOKEN: return "electronic token verification"; 2538 case VVOICE: return "voice-based verification"; 2539 case _0001: return "Complement fixation"; 2540 case _0002: return "Computed axial tomography"; 2541 case _0003: return "HLAR agar test"; 2542 case _0004: return "Macroscopic observation"; 2543 case _0005: return "Magnetic resonance"; 2544 case _0006: return "Morphometry"; 2545 case _0007: return "Positron emission tomography"; 2546 case _0008: return "SAMHSA confirmation"; 2547 case _0009: return "SAMHSA screening"; 2548 case _0010: return "Serum Neutralization"; 2549 case _0011: return "Titration"; 2550 case _0012: return "Ultrasound"; 2551 case _0013: return "X-ray crystallography"; 2552 case _0014: return "Agglutination"; 2553 case _0015: return "Buffered acidified plate agglutination"; 2554 case _0016: return "Card agglutination"; 2555 case _0017: return "Hemagglutination"; 2556 case _0018: return "Hemagglutination inhibition"; 2557 case _0019: return "Latex agglutination"; 2558 case _0020: return "Plate agglutination"; 2559 case _0021: return "Rapid agglutination"; 2560 case _0022: return "RBC agglutination"; 2561 case _0023: return "Rivanol agglutination"; 2562 case _0024: return "Tube agglutination"; 2563 case _0025: return "Bioassay"; 2564 case _0026: return "Animal Inoculation"; 2565 case _0027: return "Cytotoxicity"; 2566 case _0028: return "Embryo infective dose 50"; 2567 case _0029: return "Embryo lethal dose 50"; 2568 case _0030: return "Mouse intercerebral inoculation"; 2569 case _0031: return "Bioassay, qualitative"; 2570 case _0032: return "Bioassay, quantitative"; 2571 case _0033: return "Chemical method"; 2572 case _0034: return "Differential light absorption chemical test"; 2573 case _0035: return "Dipstick"; 2574 case _0036: return "Dipstick colorimetric laboratory test"; 2575 case _0037: return "Test strip"; 2576 case _0038: return "Chromatography"; 2577 case _0039: return "Affinity chromatography"; 2578 case _0040: return "Gas liquid chromatography"; 2579 case _0041: return "High performance liquid chromatography"; 2580 case _0042: return "Liquid Chromatography"; 2581 case _0043: return "Protein A affinity chromatography"; 2582 case _0044: return "Coagulation"; 2583 case _0045: return "Tilt tube coagulation time"; 2584 case _0046: return "Tilt tube reptilase induced coagulation"; 2585 case _0047: return "Automated count"; 2586 case _0048: return "Manual cell count"; 2587 case _0049: return "Platelet count, Rees-Ecker"; 2588 case _0050: return "Aerobic Culture"; 2589 case _0051: return "Anaerobic Culture"; 2590 case _0052: return "Chicken embryo culture"; 2591 case _0053: return "Delayed secondary enrichment"; 2592 case _0054: return "Microaerophilic Culture"; 2593 case _0055: return "Quantitative microbial culture, cup"; 2594 case _0056: return "Quantitative microbial culture, droplet"; 2595 case _0057: return "Quantitative microbial culture, filter paper"; 2596 case _0058: return "Quantitative microbial culture, pad culture"; 2597 case _0059: return "Quantitative microbial culture, pour plate"; 2598 case _0060: return "Quantitative microbial culture, surface streak"; 2599 case _0061: return "Somatic Cell culture"; 2600 case _0062: return "Agar diffusion"; 2601 case _0063: return "Agar Gel Immunodiffusion"; 2602 case _0064: return "Electrophoresis"; 2603 case _0065: return "Agaorse gel electrophoresis"; 2604 case _0066: return "Electrophoresis, citrate agar"; 2605 case _0067: return "Immunoelectrophoresis"; 2606 case _0068: return "Polyacrylamide gel electrophoresis"; 2607 case _0069: return "Starch gel electrophoresis"; 2608 case _0070: return "ELISA"; 2609 case _0071: return "ELISA, antigen capture"; 2610 case _0072: return "ELISA, avidin biotin peroxidase complex"; 2611 case _0073: return "Kinetic ELISA"; 2612 case _0074: return "ELISA, peroxidase-antiperoxidase"; 2613 case _0075: return "API 20 Strep"; 2614 case _0076: return "API 20A"; 2615 case _0077: return "API 20C AUX"; 2616 case _0078: return "API 20E"; 2617 case _0079: return "API 20NE"; 2618 case _0080: return "API 50 CH"; 2619 case _0081: return "API An-IDENT"; 2620 case _0082: return "API Coryne"; 2621 case _0083: return "API Rapid 20E"; 2622 case _0084: return "API Staph"; 2623 case _0085: return "API ZYM"; 2624 case _0086: return "Bacterial identification"; 2625 case _0087: return "mini VIDAS"; 2626 case _0088: return "Phage susceptibility typing"; 2627 case _0089: return "Quad-FERM+"; 2628 case _0090: return "RAPIDEC Staph"; 2629 case _0091: return "Staphaurex"; 2630 case _0092: return "VIDAS"; 2631 case _0093: return "Vitek"; 2632 case _0094: return "VITEK 2"; 2633 case _0095: return "Immune stain"; 2634 case _0096: return "Immunofluorescent antibody, direct"; 2635 case _0097: return "Immunofluorescent antibody, indirect"; 2636 case _0098: return "Immunoperoxidase, Avidin-Biotin Complex"; 2637 case _0099: return "Immunoperoxidase, Peroxidase anti-peroxidase complex"; 2638 case _0100: return "Immunoperoxidase, Protein A-peroxidase complex"; 2639 case _0101: return "Immunoassay"; 2640 case _0102: return "Immunoassay, qualitative, multiple step"; 2641 case _0103: return "Immunoassay, qualitative, single step"; 2642 case _0104: return "Radioimmunoassay"; 2643 case _0105: return "Immunoassay, semi-quantitative, multiple step"; 2644 case _0106: return "Immunoassay, semi-quantitative, single step"; 2645 case _0107: return "Microscopy"; 2646 case _0108: return "Darkfield microscopy"; 2647 case _0109: return "Electron microscopy"; 2648 case _0110: return "Electron microscopy tomography"; 2649 case _0111: return "Electron microscopy, negative stain"; 2650 case _0112: return "Electron microscopy, thick section"; 2651 case _0113: return "Electron microscopy, thin section"; 2652 case _0114: return "Microscopy, Light"; 2653 case _0115: return "Polarizing light microscopy"; 2654 case _0116: return "Scanning electron microscopy"; 2655 case _0117: return "Transmission electron microscopy"; 2656 case _0118: return "Transparent tape direct examination"; 2657 case _0119: return "3 Self-Sustaining Sequence Replication"; 2658 case _0120: return "Branched Chain DNA"; 2659 case _0121: return "Hybridization Protection Assay"; 2660 case _0122: return "Immune blot"; 2661 case _0123: return "In-situ hybridization"; 2662 case _0124: return "Ligase Chain Reaction"; 2663 case _0125: return "Ligation Activated Transcription"; 2664 case _0126: return "Nucleic Acid Probe"; 2665 case _0128: return "Nucleic acid probe with amplification"; 2666 case _0129: return "Nucleic acid probe with target amplification"; 2667 case _0130: return "Nucleic acid reverse transcription"; 2668 case _0131: return "Nucleic Acid Sequence Based Analysis"; 2669 case _0132: return "Polymerase chain reaction"; 2670 case _0133: return "Q-Beta Replicase or probe amplification category method"; 2671 case _0134: return "Restriction Fragment Length Polymorphism"; 2672 case _0135: return "Southern Blot"; 2673 case _0136: return "Strand Displacement Amplification"; 2674 case _0137: return "Transcription Mediated Amplification"; 2675 case _0138: return "Western Blot"; 2676 case _0139: return "Flocculation"; 2677 case _0140: return "Immune precipitation"; 2678 case _0141: return "Milk ring test"; 2679 case _0142: return "Precipitin"; 2680 case _0143: return "Acid fast stain"; 2681 case _0144: return "Acid fast stain, fluorochrome"; 2682 case _0145: return "Acid fast stain, Kinyoun's cold carbolfuchsin"; 2683 case _0146: return "Acid fast stain, Ziehl-Neelsen"; 2684 case _0147: return "Acid phosphatase stain"; 2685 case _0148: return "Acridine orange stain"; 2686 case _0149: return "Active brilliant orange KH stain"; 2687 case _0150: return "Alazarin red S stain"; 2688 case _0151: return "Alcian blue stain"; 2689 case _0152: return "Alcian blue with Periodic acid Schiff stain"; 2690 case _0153: return "Argentaffin stain"; 2691 case _0154: return "Argentaffin silver stain"; 2692 case _0155: return "Azure-eosin stain"; 2693 case _0156: return "Basic Fuschin stain"; 2694 case _0157: return "Bennhold stain"; 2695 case _0158: return "Bennhold's Congo red stain"; 2696 case _0159: return "Bielschowsky stain"; 2697 case _0160: return "Bielschowsky's silver stain"; 2698 case _0161: return "Bleach stain"; 2699 case _0162: return "Bodian stain"; 2700 case _0163: return "Brown-Brenn stain"; 2701 case _0164: return "Butyrate-esterase stain"; 2702 case _0165: return "Calcofluor white fluorescent stain"; 2703 case _0166: return "Carbol-fuchsin stain"; 2704 case _0167: return "Carmine stain"; 2705 case _0168: return "Churukian-Schenk stain"; 2706 case _0169: return "Congo red stain"; 2707 case _0170: return "Cresyl echt violet stain"; 2708 case _0171: return "Crystal violet stain"; 2709 case _0172: return "De Galantha stain"; 2710 case _0173: return "Dieterle silver impregnation stain"; 2711 case _0174: return "Fite-Farco stain"; 2712 case _0175: return "Fontana-Masson silver stain"; 2713 case _0176: return "Fouchet stain"; 2714 case _0177: return "Gomori stain"; 2715 case _0178: return "Gomori methenamine silver stain"; 2716 case _0179: return "Gomori-Wheatly trichrome stain"; 2717 case _0180: return "Gridley stain"; 2718 case _0181: return "Grimelius silver stain"; 2719 case _0182: return "Grocott stain"; 2720 case _0183: return "Grocott methenamine silver stain"; 2721 case _0184: return "Hale's colloidal ferric oxide stain"; 2722 case _0185: return "Hale's colloidal iron stain"; 2723 case _0186: return "Hansel stain"; 2724 case _0187: return "Harris regressive hematoxylin and eosin stain"; 2725 case _0188: return "Hematoxylin and eosin stain"; 2726 case _0189: return "Highman stain"; 2727 case _0190: return "Holzer stain"; 2728 case _0191: return "Iron hematoxylin stain"; 2729 case _0192: return "Jones stain"; 2730 case _0193: return "Jones methenamine silver stain"; 2731 case _0194: return "Kossa stain"; 2732 case _0195: return "Lawson-Van Gieson stain"; 2733 case _0196: return "Loeffler methylene blue stain"; 2734 case _0197: return "Luxol fast blue with cresyl violet stain"; 2735 case _0198: return "Luxol fast blue with Periodic acid-Schiff stain"; 2736 case _0199: return "MacNeal's tetrachrome blood stain"; 2737 case _0200: return "Mallory-Heidenhain stain"; 2738 case _0201: return "Masson trichrome stain"; 2739 case _0202: return "Mayer mucicarmine stain"; 2740 case _0203: return "Mayers progressive hematoxylin and eosin stain"; 2741 case _0204: return "May-Grunwald Giemsa stain"; 2742 case _0205: return "Methyl green stain"; 2743 case _0206: return "Methyl green pyronin stain"; 2744 case _0207: return "Modified Gomori-Wheatly trichrome stain"; 2745 case _0208: return "Modified Masson trichrome stain"; 2746 case _0209: return "Modified trichrome stain"; 2747 case _0210: return "Movat pentachrome stain"; 2748 case _0211: return "Mucicarmine stain"; 2749 case _0212: return "Neutral red stain"; 2750 case _0213: return "Night blue stain"; 2751 case _0214: return "Non-specific esterase stain"; 2752 case _0215: return "Oil red-O stain"; 2753 case _0216: return "Orcein stain"; 2754 case _0217: return "Perls' stain"; 2755 case _0218: return "Phosphotungstic acid-hematoxylin stain"; 2756 case _0219: return "Potassium ferrocyanide stain"; 2757 case _0220: return "Prussian blue stain"; 2758 case _0221: return "Putchler modified Bennhold stain"; 2759 case _0222: return "Quinacrine fluorescent stain"; 2760 case _0223: return "Reticulin stain"; 2761 case _0224: return "Rhodamine stain"; 2762 case _0225: return "Safranin stain"; 2763 case _0226: return "Schmorl stain"; 2764 case _0227: return "Seiver-Munger stain"; 2765 case _0228: return "Silver stain"; 2766 case _0229: return "Specific esterase stain"; 2767 case _0230: return "Steiner silver stain"; 2768 case _0231: return "Sudan III stain"; 2769 case _0232: return "Sudan IVI stain"; 2770 case _0233: return "Sulfated alcian blue stain"; 2771 case _0234: return "Supravital stain"; 2772 case _0235: return "Thioflavine-S stain"; 2773 case _0236: return "Three micron Giemsa stain"; 2774 case _0237: return "Vassar-Culling stain"; 2775 case _0238: return "Vital Stain"; 2776 case _0239: return "von Kossa stain"; 2777 case _0243: return "Minimum bactericidal concentration test, macrodilution"; 2778 case _0244: return "Minimum bactericidal concentration test, microdilution"; 2779 case _0247: return "Turbidometric"; 2780 case _0248: return "Refractometric"; 2781 case _0249: return "Thin layer chromatography (TLC)"; 2782 case _0250: return "EMIT"; 2783 case _0251: return "Flow cytometry (FC)"; 2784 case _0252: return "Radial immunodiffusion (RID)"; 2785 case _0253: return "Fluorescence polarization immunoassay (FPIA)"; 2786 case _0254: return "Immunofixation electrophoresis (IFE)"; 2787 case _0255: return "Equilibrium dialysis"; 2788 case _0256: return "Kleihauer-Betke acid elution"; 2789 case _0257: return "Anti-complement immunofluorescence (ACIF)"; 2790 case _0258: return "GC/MS"; 2791 case _0259: return "Nephelometry"; 2792 case _0260: return "IgE immunoassay antibody"; 2793 case _0261: return "Lymphocyte Microcytotoxicity Assay"; 2794 case _0262: return "Spectrophotometry"; 2795 case _0263: return "Atomic absorption spectrophotometry (AAS)"; 2796 case _0264: return "Ion selective electrode (ISE)"; 2797 case _0265: return "Gas chromatography (GC)"; 2798 case _0266: return "Isoelectric focusing (IEF)"; 2799 case _0267: return "Immunochemiluminescence"; 2800 case _0268: return "Microparticle enzyme immunoassay (MEIA)"; 2801 case _0269: return "ICP/MS"; 2802 case _0270: return "Immunoradiometric assay (IRMA)"; 2803 case _0271: return "Photo optical clot detection"; 2804 case _0280: return "Susceptibility Testing"; 2805 case _0240: return "Antibiotic sensitivity, disk"; 2806 case _0241: return "BACTEC susceptibility test"; 2807 case _0242: return "Disk dilution"; 2808 case _0272: return "Minimum Inhibitory Concentration"; 2809 case _0245: return "Minimum Inhibitory Concentration, macrodilution"; 2810 case _0246: return "Minimum Inhibitory Concentration, microdilution"; 2811 case _0273: return "Viral Genotype Susceptibility"; 2812 case _0274: return "Viral Phenotype Susceptibility"; 2813 case _0275: return "Gradient Strip"; 2814 case _0275A: return "Minimum Lethal Concentration (MLC)"; 2815 case _0276: return "Slow Mycobacteria Susceptibility"; 2816 case _0277: return "Serum bactericidal titer"; 2817 case _0278: return "Agar screen"; 2818 case _0279: return "Disk induction"; 2819 case _0127: return "Nucleic acid probe"; 2820 case NULL: return null; 2821 default: return "?"; 2822 } 2823 } 2824 2825 2826}